Mastering Java Code Execution: Tame Your Section Expansions
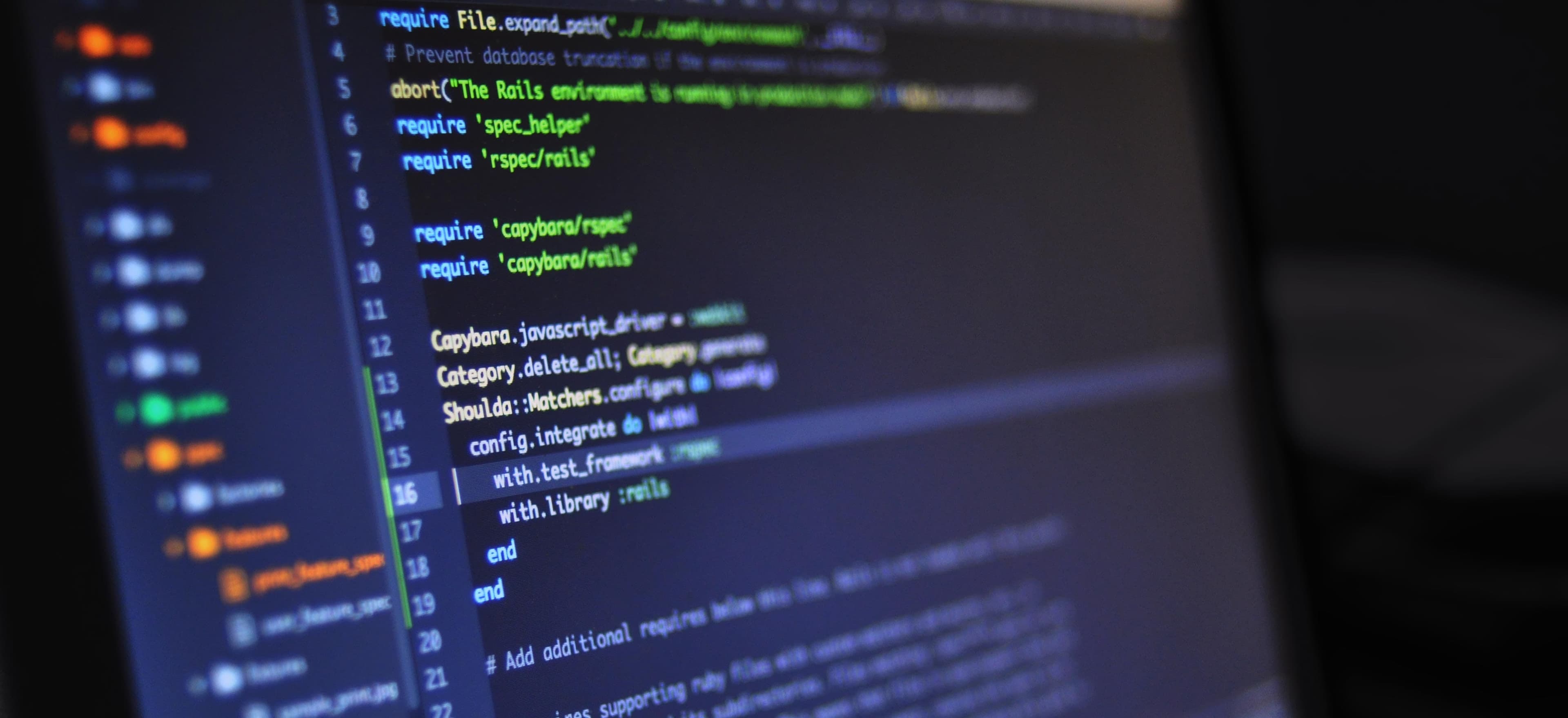
- Published on
Mastering Java Code Execution: Tame Your Section Expansions
Java is one of the most robust programming languages, enabling developers to craft high-performance applications. However, the language demands a solid understanding of how code execution works, particularly when it comes to managing sections of code. In this article, we will explore techniques to control code execution effectively, ensuring that your Java applications are efficient and maintainable. For context, be sure to check out the insightful article, Taming the Section Expansion: Controlling Code Execution.
What is Code Execution in Java?
Code execution in Java encompasses the process through which Java Virtual Machine (JVM) translates Java bytecode into machine language. This process involves several stages—compilation, class loading, and execution. Learning how to control this flow is crucial for optimizing performance and preventing runtime errors.
The Importance of Managing Code Sections
One crucial aspect of controlling code execution is managing 'sections.' A 'section' in Java might refer to various code blocks—methods, loops, or conditional statements. By effectively managing these sections, you gain better control over resource allocation, error handling, and overall performance.
Common Challenges
Before diving into solutions, it's important to understand the common challenges developers face:
- Section Overlap: Ineffective code sections can lead to performance lags.
- Uncontrolled Recursion: Recursive methods can spiral out of control if not managed.
- Ineffective Error Handling: Code sections that aren't well-defined may inadvertently swallow exceptions.
Techniques to Control Code Execution
In this section, we will explore a few effective techniques for controlling code execution in Java.
1. Usage of Conditional Statements
Conditional statements (if, switch) guide the flow based on certain conditions, making them essential for managing sections of your code. Here's a quick example:
public void performAction(int actionCode) {
switch (actionCode) {
case 1:
executeActionOne();
break;
case 2:
executeActionTwo();
break;
default:
System.out.println("Invalid action code.");
}
}
Why this code matters: By using a switch statement, you can easily manage various execution paths based on actionCode
. This creates a clear, maintainable structure while preventing section overlap and ambiguity.
2. Leveraging Exception Handling
Exception handling is a crucial component of code execution control. By using try-catch blocks, we can catch and handle exceptions gracefully without crashing the application.
public void safeDivision(int numerator, int denominator) {
try {
int result = numerator / denominator;
System.out.println("Result: " + result);
} catch (ArithmeticException e) {
System.out.println("Error: Cannot divide by zero.");
}
}
Why this code matters: The code demonstrates how a simple mistake like division by zero can be handled efficiently. By catching potential exceptions, you ensure that your application runs smoothly without unforeseen errors impacting user experience.
3. Managing Recursion Carefully
Recursion can lead to errors if not managed correctly. To prevent uncontrolled recursion, set base conditions carefully:
public int factorial(int number) {
if (number < 0) {
throw new IllegalArgumentException("Number must be non-negative");
}
if (number == 0) {
return 1; // Base case
}
return number * factorial(number - 1); // Recursive call
}
Why this code matters: By enforcing validation before the recursive calls and establishing a clear base case, the method prevents stack overflow errors. This shows how managing sections within recursive methods can improve both efficacy and safety.
4. Using Multithreading for Heavy Operations
For performance-heavy applications, you can systematize code execution by using threads to separate concerns. This can enhance responsiveness without blocking the main thread.
public void performLongTask() {
Thread task = new Thread(() -> {
// Simulating a long-running task
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
System.out.println("Task completed!");
});
task.start();
}
Why this code matters: The code snippet demonstrates how to perform a long-running task on a separate thread. This approach prevents the main thread from becoming unresponsive, allowing your application to remain user-friendly.
5. Optimizing Code Structure
Keeping your codebase organized is essential for maintaining manageable sections. One effective practice is to utilize classes and interfaces to compartmentalize functionality.
interface Action {
void execute();
}
class ActionOne implements Action {
public void execute() {
System.out.println("Executing Action One");
}
}
class ActionTwo implements Action {
public void execute() {
System.out.println("Executing Action Two");
}
}
Why this code matters: By using interfaces to define actions, you create a loosely coupled architecture that is easier to maintain and test. Each action is contained in its own class, creating clear section boundaries.
Final Considerations
Mastering Java code execution involves learning how to control code sections effectively. By employing conditional statements, leveraging exception handling, managing recursion, utilizing multithreading, and optimizing code structure, you can enhance the performance and reliability of your Java applications.
It's always beneficial to continuously develop your understanding of Java. For a deeper dive into managing code execution, I recommend checking out Taming the Section Expansion: Controlling Code Execution. This article provides practical insights into handling code sections and will enrich your skillset, making you a more proficient Java developer.
Further Reading
For additional resources, check out:
- Java Documentation for detailed information on Java's features.
- Effective Java by Joshua Bloch for best practices in coding.
By developing these skills, you'll not only tame your section expansions but also elevate your Java programming proficiency to new heights. Happy coding!
Checkout our other articles