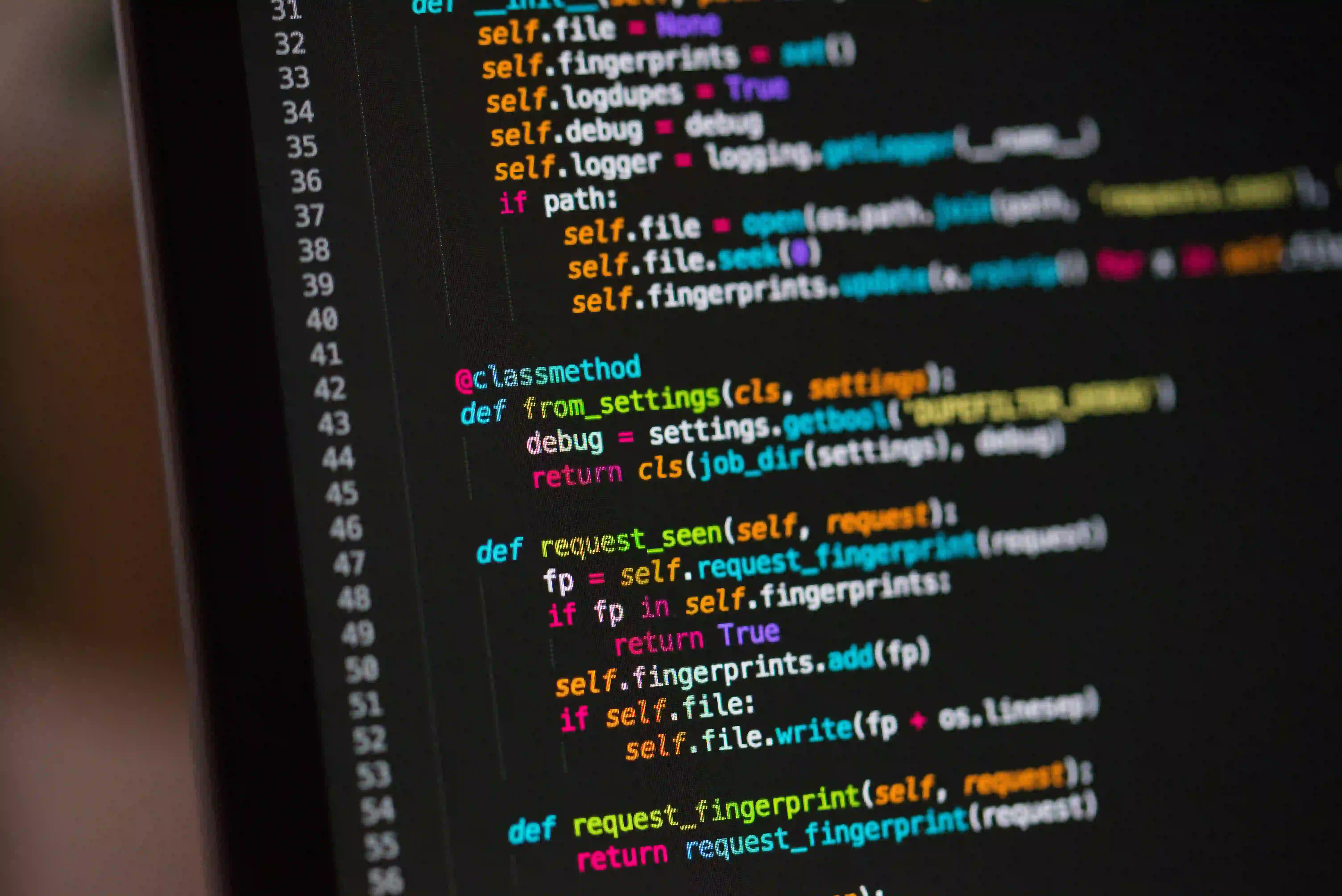
Mastering React and Relay: Overcoming Common Pitfalls
When diving into the world of modern web development, mastering frameworks like React and libraries like Relay can feel akin to navigating a vast ocean. Both tools provide immense capabilities, yet they come with their own learning curves and challenges. This article aims to guide you through overcoming common pitfalls while implementing these technologies, drawing from both foundational knowledge and practical examples.
Understanding React and Relay
What is React?
React is a popular JavaScript library for building user interfaces. Developed by Facebook, it allows developers to create single-page applications with a component-based architecture. The paradigm shift from traditional DOM manipulation to a virtual DOM in React enhances performance and allows for a seamless user experience.
What is Relay?
Relay is a JavaScript framework for managing and fetching data in React applications. Its core principle revolves around providing a powerful and efficient way to interact with GraphQL APIs. However, the learning curve associated with Relay is often cited as a barrier for many developers. As discussed in the article "Why React Relay's Learning Curve Still Frustrates Developers" on infinitejs.com, developers often struggle with Relayβs complexities, especially when trying to manage data dependencies.
Common Pitfalls in React and Relay
1. Misunderstanding Component Lifecycle
One of the most frequent challenges in React is mastering the component lifecycle. Knowing when and how components mount, update, and unmount is crucial for performance optimization.
Example: Using useEffect
import React, { useEffect, useState } from 'react';
const MyComponent = () => {
const [data, setData] = useState(null);
useEffect(() => {
fetchData();
}, []); // Run only on mount
const fetchData = async () => {
const response = await fetch('https://api.example.com/data');
const result = await response.json();
setData(result);
};
return (
<div>
{data ? <p>{data}</p> : <p>Loading...</p>}
</div>
);
};
In the above code, useEffect is utilized to fetch data when the component mounts. The empty array as a second argument ensures the effect runs only once. Not understanding this could lead to redundant API calls, affecting application performance.
2. Prop Drilling vs. Context
As applications grow, the need to pass data through various layers of components can lead to what is infamously known as "prop drilling." This occurs when props are passed down multiple levels, cluttering your components with unnecessary data.
Solution: Using React Context
import React, { createContext, useContext, useState } from 'react';
// Create context
const MyContext = createContext();
const MyProvider = ({ children }) => {
const [value, setValue] = useState('Initial Value');
return (
<MyContext.Provider value={[value, setValue]}>
{children}
</MyContext.Provider>
);
};
const ChildComponent = () => {
const [value] = useContext(MyContext);
return <div>{value}</div>;
};
In this example, instead of passing props down to ChildComponent, we utilize React's Context API. This approach simplifies data sharing across components, mitigating the issue of prop drilling.
3. Ignoring Relay's Fragment Structure
Relay emphasizes the use of fragments to manage and optimize data fetching. However, many developers overlook their potential, leading to inefficient and convoluted codebases.
Utilizing Fragments Effectively
import { graphql, useFragment } from 'react-relay';
const UserFragment = graphql`
fragment UserFragment_user on User {
id
name
email
}
`;
const UserComponent = ({ userRef }) => {
const user = useFragment(UserFragment, userRef);
return (
<div>
<h1>{user.name}</h1>
<p>{user.email}</p>
</div>
);
};
In this case, we define a fragment for the `User` data type. The `useFragment` hook allows us to efficiently fetch and utilize only the necessary fields. This results in a more modular and scalable application architecture.
4. Not Setting Optimistic Updates
In applications with complex state management, neglecting optimistic updates can lead to a broken user experience. By anticipating the state change before receiving confirmation from the server, users feel a more responsive interface.
import { commitMutation, graphql } from 'react-relay';
const AddTodoMutation = graphql`
mutation AddTodoMutation($input: AddTodoInput!) {
addTodo(input: $input) {
todo {
id
text
}
}
}
`;
const commit = (environment, input) => {
const optimisticResponse = {
addTodo: {
todo: { id: 'temp-id', text: input.text },
},
};
commitMutation(environment, {
mutation: AddTodoMutation,
variables: { input },
optimisticResponse,
updater: (store) => {
// Update the store with the new todo
},
onError: (err) => console.error(err),
});
};
This implementation provides an optimistic response, enabling a smoother experience for users. If the API call fails, you can handle this scenario gracefully.
Best Practices for Effective Usage of React and Relay
Embrace Type Safety
By integrating tools like TypeScript, you can catch errors early, enabling a more predictable development experience.
Modular Component Design
Keep components small and focused on a single responsibility. This practice enhances maintainability and facilitates easier testing.
Keep Learning
React and Relay continue to evolve. Stay updated with the official documentation, participate in community discussions, and keep an eye on the latest trends.
Final Thoughts
Mastering React and Relay requires dedication and practice. By understanding common pitfalls and implementing effective strategies, you can ultimately enhance your skills and create more robust applications. As discussed in the article "Why React Relay's Learning Curve Still Frustrates Developers" (infinitejs.com), recognizing these challenges as stepping stones rather than barriers will help you evolve into a proficient developer.
Remember, every framework and library has its quirks. Embrace them, learn from them, and grow your development prowess in this exciting ecosystem. Happy coding!