Resolving Java Log Management Issues with ELK on Kubernetes
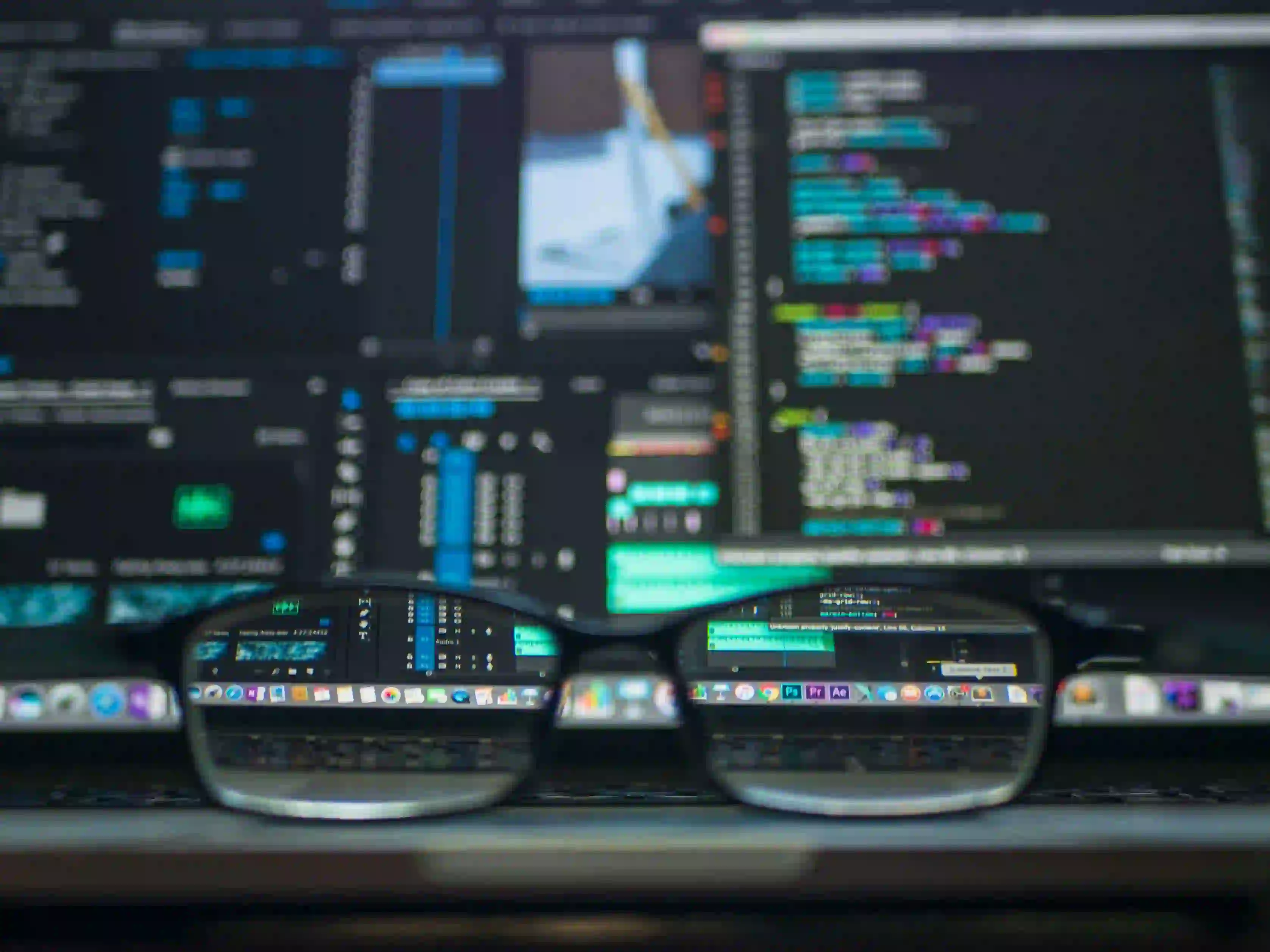
Resolving Java Log Management Issues with ELK on Kubernetes
Log management is a critical component of modern application development, especially in distributed systems. When building applications in Java, efficiently managing logs can be a challenging task. This becomes even more complex in environments like Kubernetes. Fortunately, using the ELK stack (Elasticsearch, Logstash, and Kibana) along with Kubernetes can simplify the process significantly. In this article, we will explore how to set up and troubleshoot Java log management issues using ELK in Kubernetes.
What is ELK?
Before diving into details, let's briefly summarize what ELK is.
- Elasticsearch: A distributed, RESTful search and analytics engine. It is designed for horizontal scalability and high availability.
- Logstash: A server-side data processing pipeline that ingests data from multiple sources, transforms it, and then sends it to your chosen "stash."
- Kibana: A visualization layer on top of Elasticsearch that allows users to search and visualize the data.
Using these three tools together provides a powerful solution for managing logs from Java applications running in Kubernetes.
Why Use ELK with Java on Kubernetes?
- Centralized Logging: Collect all logs from various services into a single repository.
- Scalability: ELK can handle large volumes of data efficiently, making it perfect for microservices architectures.
- Real-Time Analysis: Monitor logs in real-time, allowing you to address issues proactively.
- Flexibility: Easily configurable parameters for various environments.
To make these benefits actionable, we will outline how to resolve common Java log management issues when using the ELK stack in a Kubernetes environment.
Setting Up ELK on Kubernetes
Prerequisites
Before we start, ensure you have the following:
- A Kubernetes cluster up and running.
kubectl
configured to communicate with your cluster.- Knowledge of Docker and Kubernetes.
Step 1: Deploying Elasticsearch
Elasticsearch is the backbone of the ELK stack. It stores, searches, and analyzes log data.
Create a YAML configuration file (elasticsearch-deployment.yaml
) for deploying Elasticsearch:
apiVersion: apps/v1
kind: Deployment
metadata:
name: elasticsearch
labels:
app: elasticsearch
spec:
replicas: 1
selector:
matchLabels:
app: elasticsearch
template:
metadata:
labels:
app: elasticsearch
spec:
containers:
- name: elasticsearch
image: elasticsearch:7.10.0
env:
- name: discovery.type
value: single-node
ports:
- containerPort: 9200
---
apiVersion: v1
kind: Service
metadata:
name: elasticsearch
spec:
ports:
- port: 9200
targetPort: 9200
selector:
app: elasticsearch
Why this works: The discovery.type: single-node
configuration is essential as it allows Elasticsearch to run without requiring additional nodes, simplifying the deployment for our development environment.
Deploy the configuration using:
kubectl apply -f elasticsearch-deployment.yaml
Step 2: Deploying Logstash
Logstash will act as a data pipeline for collecting and transmitting logs from our Java applications to Elasticsearch.
Create a configuration file (logstash-configmap.yaml
) for Logstash:
apiVersion: v1
kind: ConfigMap
metadata:
name: logstash-config
data:
logstash.conf: |
input {
tcp {
port => 5044
codec => json_lines
}
}
output {
elasticsearch {
hosts => ["http://elasticsearch:9200"]
index => "java-logs-%{+YYYY.MM.dd}"
}
}
---
apiVersion: apps/v1
kind: Deployment
metadata:
name: logstash
spec:
replicas: 1
selector:
matchLabels:
app: logstash
template:
metadata:
labels:
app: logstash
spec:
containers:
- name: logstash
image: logstash:7.10.0
ports:
- containerPort: 5044
volumeMounts:
- name: logstash-config
mountPath: /usr/share/logstash/pipeline/
volumes:
- name: logstash-config
configMap:
name: logstash-config
Why this works: The TCP input receives logs from your Java application, and it outputs them in a structured format to Elasticsearch. This configuration accommodates real-time processing.
Deploy the configuration using:
kubectl apply -f logstash-configmap.yaml
Step 3: Deploying Kibana
Kibana is essential for visualizing your logs. Create a YAML configuration file (kibana-deployment.yaml
):
apiVersion: apps/v1
kind: Deployment
metadata:
name: kibana
labels:
app: kibana
spec:
replicas: 1
selector:
matchLabels:
app: kibana
template:
metadata:
labels:
app: kibana
spec:
containers:
- name: kibana
image: kibana:7.10.0
ports:
- containerPort: 5601
env:
- name: ELASTICSEARCH_HOSTS
value: "http://elasticsearch:9200"
---
apiVersion: v1
kind: Service
metadata:
name: kibana
spec:
ports:
- port: 5601
targetPort: 5601
selector:
app: kibana
Why this works: The ELASTICSEARCH_HOSTS
environment variable ensures Kibana is properly linked to Elasticsearch, allowing it to visualize the log data seamlessly.
Deploy the configuration using:
kubectl apply -f kibana-deployment.yaml
Step 4: Configuring Your Java Application
To send logs from your Java application to Logstash, you can use a logging framework such as Logback or Log4j. Below is an example of how to configure Logback for sending logs over TCP.
Logback Configuration
Add the Logback dependencies in your pom.xml
:
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-classic</artifactId>
<version>1.2.3</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.30</version>
</dependency>
Next, create a logback.xml
configuration file:
<configuration>
<appender name="TCP" class="ch.qos.logback.classic.net.SocketAppender">
<remoteHost>logstash</remoteHost>
<port>5044</port>
<encoder>
<pattern>
{
"timestamp": "%date",
"level": "%level",
"logger": "%logger",
"thread": "%thread",
"msg": "%msg"
}
</pattern>
</encoder>
</appender>
<root level="info">
<appender-ref ref="TCP"/>
</root>
</configuration>
Why this works: The SocketAppender
sends logs directly to Logstash over TCP. The JSON pattern helps ensure logs are structured, making parsing easier on the ELK side.
Deploy your Java application with the proper logging configuration, and start sending logs.
Monitoring Logs with Kibana
At this point, our ELK stack should be up and running. Access Kibana through the web browser at http://{your-kubernetes-cluster-ip}:5601
.
Add an index pattern for java-logs-*
, and you will be able to see and analyze logs in a clear and structured chart format.
Troubleshooting Common Issues
While setting up ELK on Kubernetes, you might encounter some common issues. For detailed guidelines, refer to the article titled Troubleshooting Common Issues When Setting Up ELK on Kubernetes.
-
Elasticsearch Not Starting: If you encounter issues with Elasticsearch not starting, check the logs using:
π§snippet.shkubectl logs -f $(kubectl get pods | grep elasticsearch | awk '{print $1}')
-
Logstash not receiving logs: Ensure that your Java application is correctly configured to send logs. Also, check the network connectivity between your app and Logstash.
-
Kibana not displaying logs: Verify that the index pattern matches the logs and that they are being successfully indexed in Elasticsearch.
The Last Word
Using ELK in a Kubernetes environment presents a robust solution for Java log management. It centralizes logs, enables real-time analysis, and scales with your application needs. Configuring ELK involves understanding each component's role and how they communicate, as demonstrated in the setup above.
Take the time to configure your systems correctly and make full use of the ELK stack to ensure a smooth logging experience. Embrace this powerful combination, and monitor your Java applications with confidence!