Struggling with SOA Modularity? Here’s Your Solution!
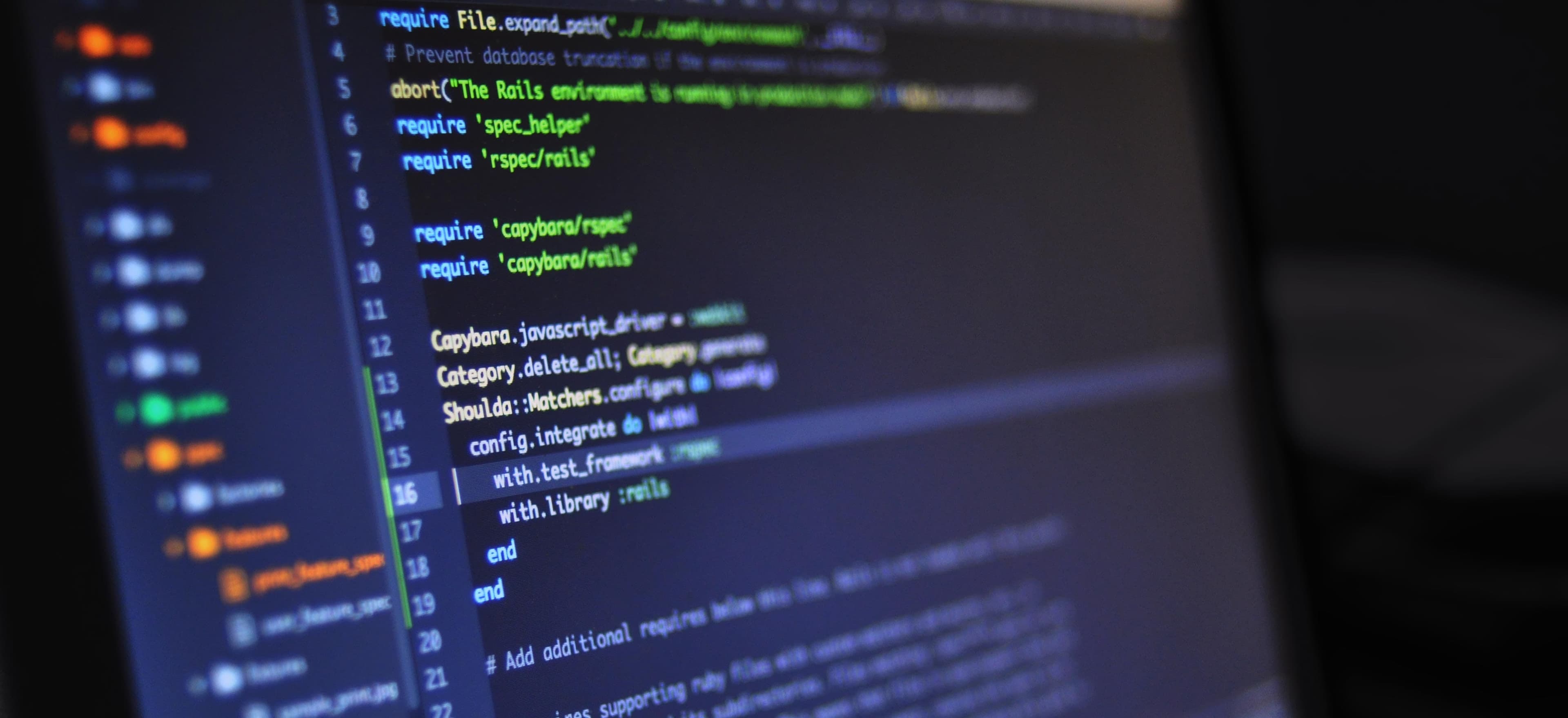
- Published on
Struggling with SOA Modularity? Here’s Your Solution!
In today's rapidly evolving software landscape, Service-Oriented Architecture (SOA) has emerged as a critical strategy for designing and building applications. However, achieving true modularity within SOA presents numerous challenges. In this post, we will delve into the intricacies of SOA modularity, understand its significance, and explore practical solutions to enhance it.
What is SOA?
Service-Oriented Architecture (SOA) is an architectural pattern that allows different services to communicate with one another, utilizing a combination of protocols and interfaces. The primary advantage of SOA lies in its ability to provide flexibility, scalability, and reusability. Through SOA, organizations can develop software systems that are easier to integrate and maintain.
Key benefits of SOA include:
- Interoperability: Services from various platforms can interact seamlessly.
- Scalability: New services can be added without disrupting existing functionality.
- Reusability: Developers can reuse existing services, reducing redundancy.
Despite these advantages, mastering modularity within SOA can be challenging, leading many to ask: How do we achieve a modular structure that fosters growth and adaptability?
The Importance of Modularity in SOA
Modularity is vital for several reasons:
- Independent Development: Modular services allow teams to work on different components simultaneously.
- Ease of Updates: Updating one service does not necessitate changes in the entire system.
- Testing Simplification: Testing becomes easier, as each module can be tested in isolation.
However, modularity within SOA often falls short due to tightly coupled services, poor interface design, and inadequate governance frameworks. To overcome these challenges, let us explore effective strategies to improve modularity in SOA.
Strategies to Enhance SOA Modularity
1. Define Clear Interfaces
The first step towards achieving modularity is establishing clear and well-defined interfaces. An interface specifies how services communicate with each other, encompassing the data formats, protocols, and operation methods.
Example: Defining an Interface in Java
Consider a simple example where we define a service interface for user management.
public interface UserService {
User createUser(String username, String password);
User getUser(String username);
void deleteUser(String username);
}
Why this matters: By defining an interface like UserService
, we create a contract for users of the service. This promotes loose coupling, as implementations can vary without affecting dependent components.
2. Adopt Asynchronous Communication
Using asynchronous communication patterns, such as message queues or event-driven architectures, can significantly decouple services.
Example of Asynchronous Messaging
Let’s consider using Apache Kafka for messaging:
import org.apache.kafka.clients.producer.KafkaProducer;
import org.apache.kafka.clients.producer.ProducerRecord;
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
KafkaProducer<String, String> producer = new KafkaProducer<>(props);
producer.send(new ProducerRecord<>("user-topic", "user-key", "user-data"));
Why use this approach? Asynchronous communication allows services to function independently. If one service is unavailable, others continue to operate, improving the overall resilience of your system.
3. Embrace Microservices
While SOA refers to a broader concept, microservices can be seen as a more granular form of SOA. By breaking down applications into smaller, independently deployable services, modularity becomes achievable.
- Services can be scaled independently.
- Each team can work on a service aligned with its expertise.
However, microservices also introduce complexity. You can learn more about the benefits of microservices here.
4. Implement Governance Frameworks
Establishing governance frameworks ensures that services conform to architectural standards. This includes enforcing rules about:
- Interface contracts
- Service naming conventions
- Security policies
By adhering to these frameworks, organizations can maintain modularity and avoid undesirable dependencies.
5. Use API Gateways
API gateways act as a single entry point for services and manage how clients interact with them. By centralizing communication, gateways can abstract away the underlying services, further promoting modularity.
Example: API Gateway Configuration
Using Spring Cloud Gateway, we can configure routing:
spring:
cloud:
gateway:
routes:
- id: user_service
uri: lb://user-service
predicates:
- Path=/user/**
Why this is essential: An API gateway can reduce the complexity of client interactions with multiple services, allowing for easier client-side logic.
Testing Your SOA Modularity
To ensure that your SOA modularity is effective, implement automated testing to verify the interactions between different services. Use testing frameworks like JUnit or TestNG to perform integration tests.
Example: Integration Test in JUnit
import static org.springframework.boot.test.context.SpringBootTest.WebEnvironment.RANDOM_PORT;
@SpringBootTest(webEnvironment = RANDOM_PORT)
public class UserServiceIntegrationTest {
@Autowired
private UserService userService;
@Test
public void testCreateUser() {
User user = userService.createUser("testuser", "password");
assertNotNull(user);
assertEquals("testuser", user.getUsername());
}
}
The importance of testing: Integration tests like this ensure that services work as expected when combined, which is critical in a modular architecture.
In Conclusion, Here is What Matters
Achieving modularity in SOA is not a one-time task but rather an ongoing journey. By defining clear interfaces, embracing asynchronous communication, adopting microservices, implementing governance, and utilizing API gateways, you can significantly enhance your SOA's modularity.
For further reading on improving SOA architecture, check out resources on Best Practices in SOA.
The road to effective modularity may be challenging, but with the right tools and principles, you can create a robust, scalable, and maintainable architecture that will stand the test of time. Embrace these changes today, and watch your SOA transform into a modular marvel!
Checkout our other articles