Feign vs RestTemplate: Choosing the Right Spring Client
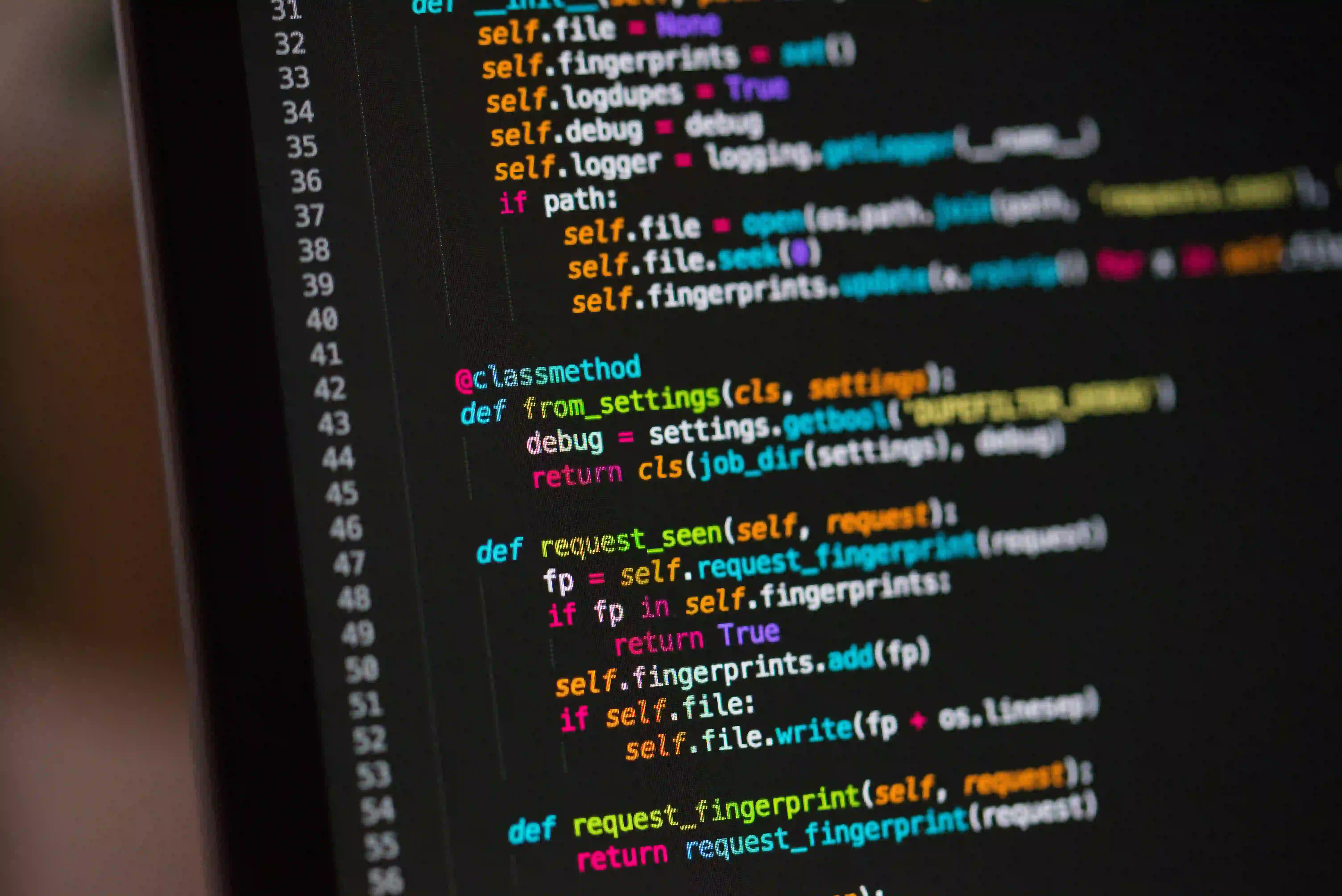
Feign vs RestTemplate: Choosing the Right Spring Client
When working with microservices, a common requirement is to make HTTP calls between services. In the Spring ecosystem, there are a few options to facilitate these requests, with Feign and RestTemplate being among the most popular choices. Each has its own strengths and weaknesses, making it crucial to understand the differences to choose the right tool for your project.
This article delves into Feign and RestTemplate, examining their features, use cases, and how to implement them in your applications. By the end of this post, you will have a clearer understanding of when to use each of these Spring clients.
Table of Contents
- What is RestTemplate?
- What is Feign?
- Key Differences Between Feign and RestTemplate
- Use Cases
- Conclusion
What is RestTemplate?
RestTemplate is a synchronous client provided by Spring for making RESTful HTTP requests. It offers several methods to make HTTP calls like GET
, POST
, PUT
, and DELETE
.
Key Features
- Request/Response Handling: Handles serialization and deserialization internally, allowing you to focus on your application logic.
- Customizable Interceptors: You can easily create custom interceptors to add headers, logging, etc.
- Error Handling: Built-in exception handling with the
ResponseErrorHandler
interface.
Basic Example
Here’s a simple example of using RestTemplate:
import org.springframework.web.client.RestTemplate;
public class RestTemplateExample {
public static void main(String[] args) {
RestTemplate restTemplate = new RestTemplate();
String url = "https://api.example.com/data";
// GET request
MyData response = restTemplate.getForObject(url, MyData.class);
System.out.println(response);
}
}
class MyData {
private String dataField;
// Getters and Setters
}
Why Use RestTemplate?
RestTemplate is perfect for simple use cases where you need to perform straightforward HTTP requests without too much overhead. It provides a straightforward, traditional Java approach to handle HTTP interactions.
What is Feign?
Feign is a declarative web service client from Netflix that simplifies the process of writing HTTP clients. It allows you to define a Java interface and automatically create a REST client implementation at runtime.
Key Features
- Declarative Approach: You can define an interface, and Feign will generate the implementation, making code cleaner and easier to read.
- Integration with Spring Cloud: Seamless compatibility with Spring Cloud for microservice architecture, providing features like load balancing and service discovery.
- Customizable: Feign supports custom configuration through annotations and a variety of different form configurations.
Basic Example
Here's a quick example of how to use Feign:
import feign.Feign;
import feign.RequestLine;
public interface ApiClient {
@RequestLine("GET /data")
MyData getData();
}
public class FeignExample {
public static void main(String[] args) {
ApiClient apiClient = Feign.builder()
.target(ApiClient.class, "https://api.example.com");
MyData response = apiClient.getData();
System.out.println(response);
}
}
class MyData {
private String dataField;
// Getters and Setters
}
Why Use Feign?
Feign is ideal for larger applications or microservices where a declarative style of writing HTTP clients can drastically reduce boilerplate code. With its integration into Spring Cloud, it becomes even more powerful.
Key Differences Between Feign and RestTemplate
| Feature | RestTemplate | Feign | |------------------|---------------------------------------|--------------------------------------| | Approach | Imperative | Declarative | | Configuration | Manually configure HTTP requests | Auto generation of implementation based on interface | | Complexity | More lines of code for HTTP handling | Less boilerplate code | | Error Handling | Built-in exception handling | Custom error handling through interfaces | | Spring Cloud Integration | Requires additional setup | Seamless integration with Spring Cloud | | Performance | Synchronous, may block | Synchronous, but can be configured for asynchronous |
Use Cases
When to Use RestTemplate
- Simple Applications: When your application is relatively straightforward and does not require extensive service-to-service communication.
- Existing Codebase: When you have an existing codebase that already uses RestTemplate, it may not be worth the effort to refactor.
- Lightweight Tasks: When making lightweight HTTP requests where performance is not critical.
When to Use Feign
- Microservices Architecture: If you are developing microservices that interact heavily, Feign’s declarative approach can reduce boilerplate.
- Integration with Spring Cloud: If your application is leveraging Spring Cloud for service discovery or load balancing, Feign can work seamlessly with Eureka and Ribbon.
- Complex API Requests: When calling APIs that require complex request structures, annotations on a defined interface can simplify your code.
Final Thoughts
Both RestTemplate and Feign have their place within the Spring ecosystem. For simple tasks, RestTemplate may be the ideal choice due to its ease of use and straightforward API. However, for more complicated microservices solutions where maintaining clean and concise code is essential, Feign could be the better alternative.
Ultimately, it comes down to the requirements of your application and the complexity of your service interactions. Evaluate your needs and choose the tool that aligns best with your project's objectives. For more detailed exploration, you might want to check out the official Spring Documentation on RestTemplate and Feigin Documentation for Feign.
Choosing the right client not only enhances readability but also influences the performance and maintainability of your code. Happy coding!