Ensuring Reliable Data Exchange in Microservices Architecture
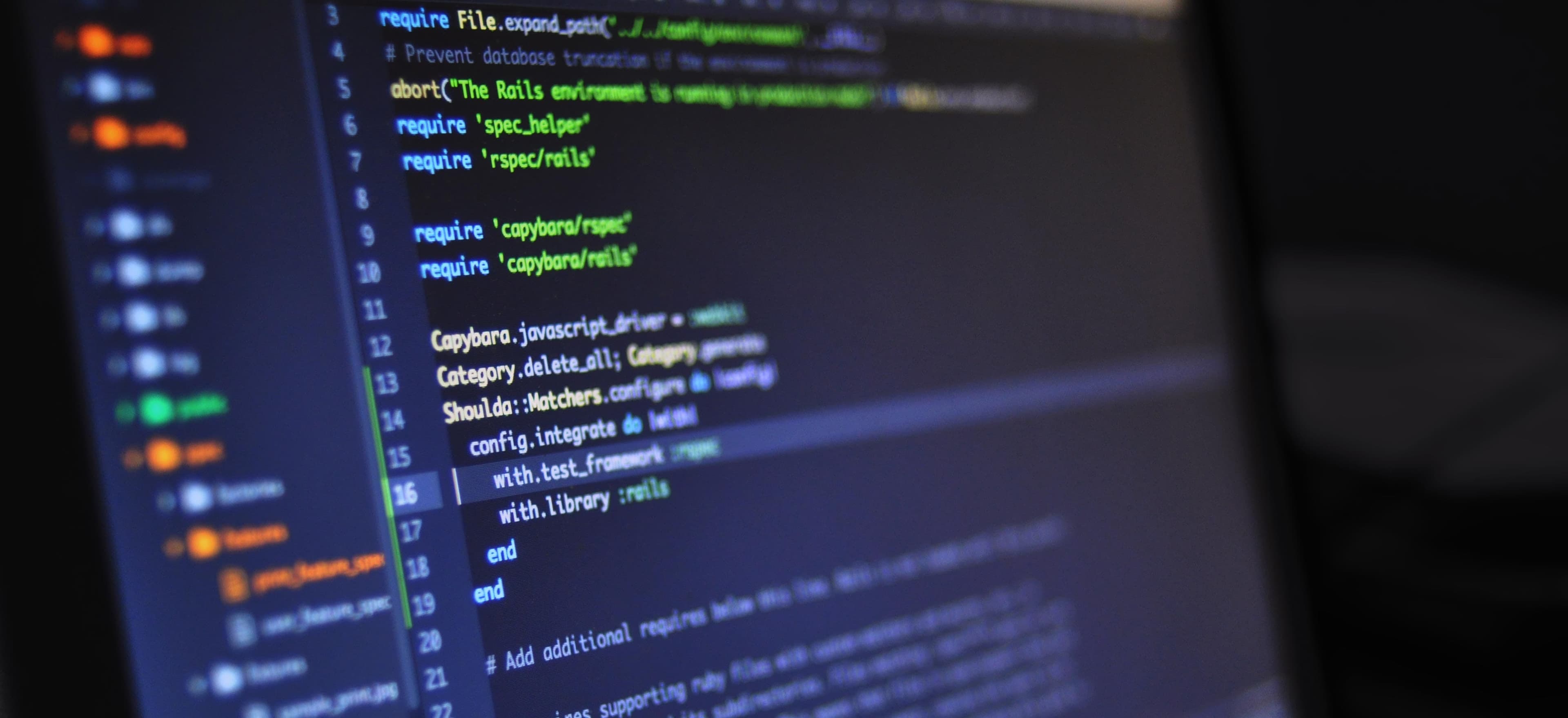
- Published on
Ensuring Reliable Data Exchange in Microservices Architecture
Microservices architecture has become a popular approach for developing complex applications due to its modularity, scalability, and flexibility. However, one of the critical challenges that arise in a microservices environment is ensuring reliable data exchange between services. In this blog post, we will explore the various strategies and best practices for achieving effective communication and data integrity in a microservices architecture.
What is Microservices Architecture?
Before diving into the nuances of reliable data exchange, it's crucial to understand what microservices architecture entails. Microservices architecture is an architectural style where an application is composed of multiple, loosely coupled services. Each service is responsible for a specific business function and can communicate with other services over a network, typically using RESTful APIs or messaging systems.
Advantages of Microservices
- Scalability: Individual services can be scaled independently based on demand.
- Flexibility: Different services can be built using different technologies and programming languages.
- Resilience: Failure in one service does not adversely affect the entire system.
However, these benefits come with the complexity of managing inter-service communication and ensuring data consistency.
Challenges in Data Exchange
Microservices face several challenges in data exchange:
- Network Latency: Communication over a network can introduce delays.
- Data Consistency: Ensuring that all services have a consistent view of the data.
- Failure Handling: Dealing with service downtimes and ensuring data is not lost.
To tackle these challenges, developers must adopt various strategies.
Strategies for Reliable Data Exchange
1. Use of API Gateways
An API Gateway is a server that acts as an entry point for clients to interact with the microservices. It provides a unified interface for multiple services and can handle various tasks such as authentication, routing, and rate limiting.
Code Example:
@RestController
@RequestMapping("/api/gateway")
public class ApiGatewayController {
@Autowired
private RestTemplate restTemplate;
@GetMapping("/serviceA")
public ResponseEntity<String> callServiceA() {
String serviceAUrl = "http://serviceA/endpoint";
return restTemplate.getForEntity(serviceAUrl, String.class);
}
}
Why: Here, we are using RestTemplate
to make a call to another service. The API Gateway abstracts the complexity of service communication, making it easier for clients to interact with multiple microservices seamlessly.
2. Event-Driven Architecture
Using event-driven architecture can help decouple services and enhance data exchange reliability. In this approach, services communicate by producing and consuming events instead of making direct API calls.
Code Example:
@Service
public class UserService {
@Autowired
private KafkaTemplate<String, String> kafkaTemplate;
public void createUser(User user) {
// Save user to database
// ...
// Publish an event
kafkaTemplate.send("user-topic", "User created: " + user.getId());
}
}
Why: This code snippet demonstrates how a service can produce an event to a message broker (like Kafka) after creating a user. Other services can subscribe to this event and react accordingly. This decoupling reduces direct dependencies between services and enhances resilience.
3. Distributed Transactions
Managing transactions across microservices can become challenging. One common approach to ensure data consistency is implementing the Saga pattern. In this pattern, each service participates in a series of transactions that can either be committed or compensated if a failure occurs.
Code Example:
@Saga
public class OrderSaga {
@Autowired
private PaymentService paymentService;
@Autowired
private InventoryService inventoryService;
public void createOrder(Order order) {
// Step 1: Reserve inventory
boolean isInventoryReserved = inventoryService.reserve(order);
if (!isInventoryReserved) {
throw new RuntimeException("Inventory reservation failed");
}
// Step 2: Process payment
boolean isPaymentProcessed = paymentService.processPayment(order.getPaymentDetails());
if (!isPaymentProcessed) {
// Step 3: Compensate inventory
inventoryService.release(order.getInventoryDetails());
throw new RuntimeException("Payment processing failed");
}
// Order created successfully
}
}
Why: The above code illustrates how the saga pattern manages a series of operations. If one part of the transaction fails, compensating actions (like releasing inventory) are initiated. This ensures that even in case of failure, the system maintains data consistency across services.
4. Circuit Breaker Pattern
When microservices communicate over a network, the risk of service unavailability increases. The Circuit Breaker pattern can be employed to detect failures and provide fallback solutions.
Code Example:
@Component
public class UserServiceFallback implements UserService {
@Override
public User getUser(Long userId) {
return new User("Fallback User", "No details available");
}
}
@RestController
@RequestMapping("/api/users")
public class UserController {
@Autowired
private CircuitBreakerFactory circuitBreakerFactory;
@GetMapping("/{userId}")
public User getUser(@PathVariable Long userId) {
return circuitBreakerFactory.create("userServiceCircuitBreaker")
.run(() -> userService.getUser(userId),
throwable -> new User("Fallback User", "Service unavailable"));
}
}
Why: This code implements the Circuit Breaker pattern using Spring Cloud. If the getUser
service fails, the circuit breaker prevents further calls and returns a fallback response, improving resilience and user experience.
Best Practices for Data Exchange
-
Use Strongly Typed Contracts: Define clear API contracts using tools like Swagger or OpenAPI. This ensures all services understand how to communicate effectively.
-
Implement Logging and Monitoring: Use centralized logging and monitoring tools (like ELK Stack or Prometheus) to track requests between services and detect issues promptly.
-
Maintain Idempotency: Ensure that operations are idempotent, meaning that repeating the same operation will have the same effect. This is crucial for retrying failed requests.
-
Choose the Right Communication Style: Use synchronous communication (like REST) for immediate responses, while asynchronous communication (like messaging queues) is suitable for decoupling services.
-
Test Thoroughly: Regularly test the communication pathways between microservices to spot potential issues early.
Final Considerations
Ensuring reliable data exchange in microservices architecture is a multifaceted challenge that requires a thoughtful combination of strategies and patterns. By employing techniques such as event-driven architecture, the Saga pattern, and the Circuit Breaker pattern, developers can build resilient microservices systems that handle failures gracefully and maintain the integrity of data.
Adopting the best practices outlined in this post will help you navigate the complexities of microservices while ensuring that your application performs seamlessly under varying conditions.
For further reading on microservices architecture, consider checking out Microservices.io and Spring Cloud Documentation.
By implementing these strategies, you can build a robust microservices architecture that keeps communication between services reliable and efficient. Happy coding!
Checkout our other articles