Mastering Functional FizzBuzz in Java: A Step-by-Step Guide
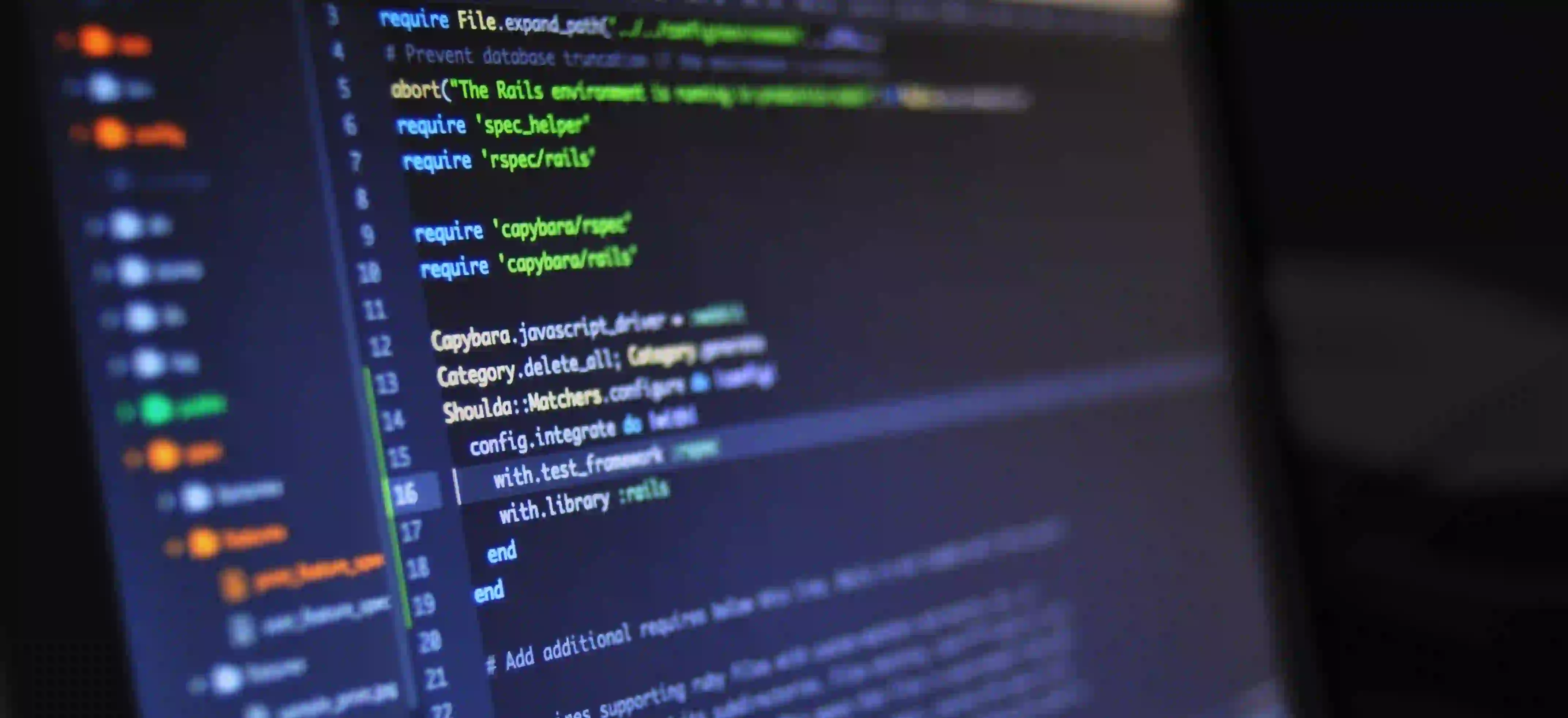
Mastering Functional FizzBuzz in Java: A Step-by-Step Guide
FizzBuzz is a classic programming challenge often used to assess algorithmic thinking and proficiency in programming languages. The conventional requirements are simple:
- Print numbers from 1 to n.
- For multiples of three, print "Fizz" instead of the number.
- For multiples of five, print "Buzz".
- For numbers that are multiples of both three and five, print "FizzBuzz".
While the traditional FizzBuzz is straightforward, we can take it a step further by leveraging Java's functional programming capabilities. In this blog post, we will explore how to implement FizzBuzz using Java Streams and Lambda expressions, providing an elegant and efficient solution.
Understanding Functional Programming in Java
Before we dive into the FizzBuzz implementation, it’s essential to understand some core principles of functional programming in Java:
-
First-Class Functions: Functions are treated as first-class citizens, meaning they can be passed as arguments, returned from other functions, and assigned to variables.
-
Immutable Data: In functional programming, we strive to avoid side effects and mutable data. This leads to cleaner and more predictable code.
-
Higher-Order Functions: Functions that can take other functions as parameters or return functions as results.
Java introduced functional programming in version 8, which includes lambda expressions and the Stream API.
Setting Up FizzBuzz with Java Streams
Let’s break down the implementation of FizzBuzz into digestible steps using Java Streams. A Stream represents a sequence of elements supporting sequential and parallel aggregate operations. Here’s how you can implement FizzBuzz functionally:
import java.util.stream.IntStream;
public class FizzBuzz {
public static void main(String[] args) {
fizzBuzz(100);
}
public static void fizzBuzz(int n) {
IntStream.rangeClosed(1, n) // Generate a stream of integers from 1 to n
.mapToObj(FizzBuzz::fizzBuzzLogic) // Map each integer to its corresponding FizzBuzz value
.forEach(System.out::println); // Print each result
}
private static String fizzBuzzLogic(int number) {
if (number % 3 == 0 && number % 5 == 0) {
return "FizzBuzz"; // Condition for multiples of both 3 and 5
}
if (number % 3 == 0) {
return "Fizz"; // Condition for multiples of 3
}
if (number % 5 == 0) {
return "Buzz"; // Condition for multiples of 5
}
return String.valueOf(number); // If none of the above, return the number itself
}
}
Explanation of the Code
-
Importing Necessary Packages: We start by importing
java.util.stream.IntStream
. This allows us to work with a stream of integers conveniently. -
Main Method: Inside the
main
method, we call thefizzBuzz
method with the desired upper limit (in this case, 100). -
Creating the Stream:
IntStream.rangeClosed(1, n)
: Creates a stream of integers from 1 to n (inclusive).mapToObj(FizzBuzz::fizzBuzzLogic)
: Each integer in the stream is passed to thefizzBuzzLogic
method, which returns a string ("Fizz", "Buzz", "FizzBuzz", or the number itself).forEach(System.out::println)
: Finally, we print each string to the console.
Functional Logic with fizzBuzzLogic
- This method encapsulates the core logic of determining whether to print "Fizz", "Buzz", "FizzBuzz", or the number itself.
- It checks the conditions for divisibility and returns the appropriate string.
Advantages of Using Java Streams
Using the Stream API and functional paradigms provide several advantages:
- Conciseness: The code is much shorter and more expressive.
- Readability: The intent of the code is clear, making it easier to understand and maintain.
- Performance: Java Streams can be processed in parallel, potentially improving performance for large datasets.
Enhancing FizzBuzz: Customization
The beauty of FizzBuzz lies in its simplicity, but it can be further customized. For instance, what if we wanted to allow dynamic customization of the words to print for multiples of three and five?
Here's how you can extend the fizzBuzz
method to accept custom strings:
public static void fizzBuzz(int n, String fizz, String buzz) {
IntStream.rangeClosed(1, n)
.mapToObj(i -> fizzBuzzCustomLogic(i, fizz, buzz))
.forEach(System.out::println);
}
private static String fizzBuzzCustomLogic(int number, String fizz, String buzz) {
if (number % 3 == 0 && number % 5 == 0) {
return fizz + buzz;
}
if (number % 3 == 0) {
return fizz;
}
if (number % 5 == 0) {
return buzz;
}
return String.valueOf(number);
}
How to Use Custom Strings
To use the customized FizzBuzz, simply call the method with your desired strings:
public static void main(String[] args) {
fizzBuzz(100, "Foo", "Bar");
}
In this case, numbers that are multiples of three will print "Foo" and multiples of five will print "Bar".
Closing the Chapter
The Functional FizzBuzz in Java exemplifies how leveraging functional programming concepts can introduce elegance and efficiency to traditional programming tasks. By using Java Streams and lambda expressions, you can create concise code that is easier to read and maintain.
In this guide, we learned not only how to implement a functional FizzBuzz but also how to extend its functionality for custom logic. Whether you're preparing for coding interviews or looking to sharpen your Java skills, mastering these principles will supercharge your programming toolkit.
Additional Resources
- Java 8 Streams Documentation
- Functional Programming Concepts
- Coding Interview Questions and Answers
Happy coding!