10 Kotlin Features Java Developers Can't Resist
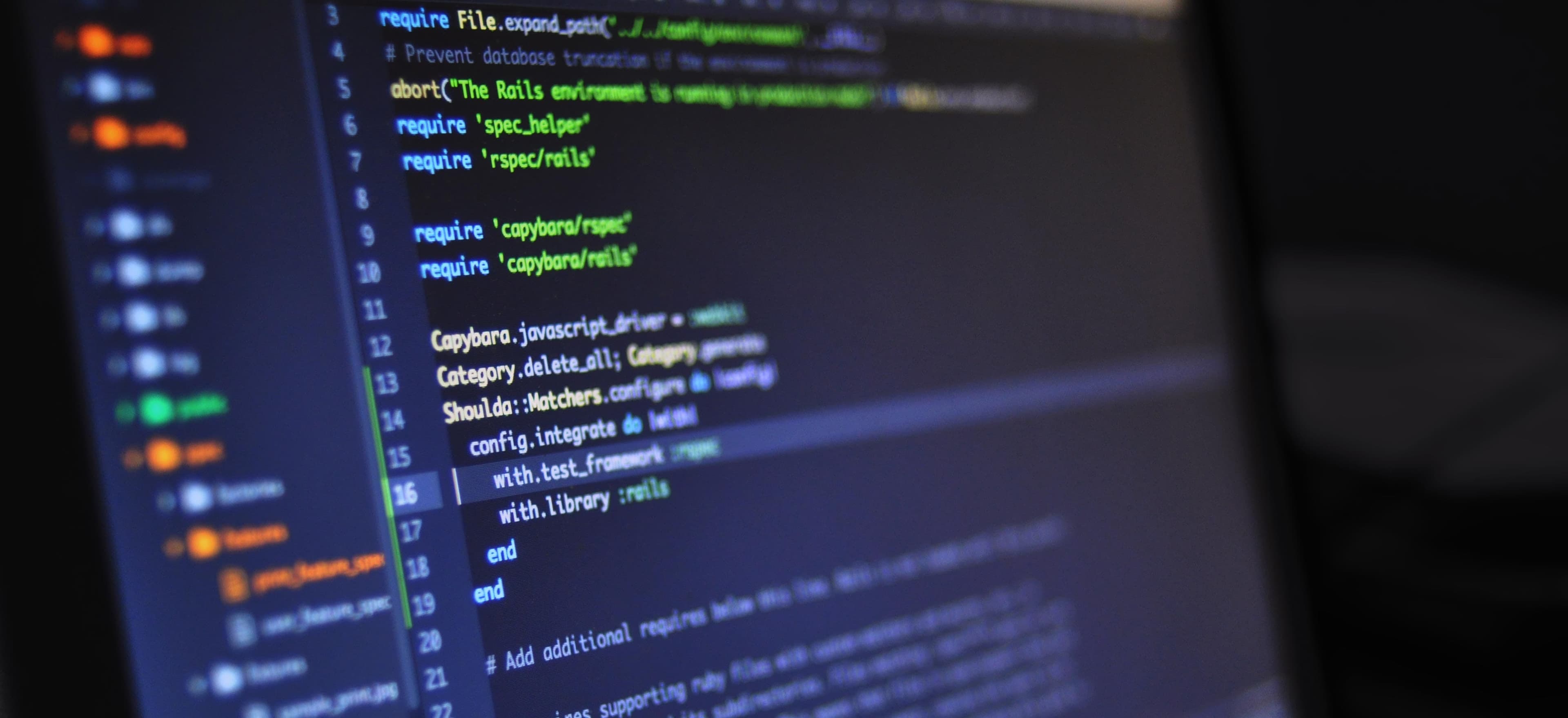
- Published on
10 Kotlin Features Java Developers Can't Resist
As the tech landscape evolves, Java developers are increasingly looking for alternatives to enhance productivity and simplify coding tasks. Kotlin, a statically typed programming language developed by JetBrains, has emerged as an excellent choice. Not only is Kotlin fully interoperable with Java, but it also brings forth a suite of modern features that enhance functionality and ease of use. In this blog post, we will explore ten compelling features of Kotlin that Java developers will find irresistible.
1. Interoperability with Java
One of the standout features of Kotlin is its seamless interoperability with Java.
fun greet(name: String) {
println("Hello, $name!")
}
With this simple function, Kotlin allows developers to call Java methods and use Java libraries without any special configuration. This means you can gradually migrate to Kotlin while still leveraging your existing Java codebase, making the transition smooth and efficient. More on interoperability.
2. Null Safety
Kotlin takes null pointer exceptions seriously by providing built-in null safety. In Java, developers have to perform redundant null checks. In Kotlin, however, the type system distinguishes between nullable and non-nullable types.
fun printLength(str: String?) {
println("Length: ${str?.length ?: "Not provided"}")
}
In this example, the safe call operator ?.
checks if str
is null before accessing length
. This reduces the risk of crashes caused by null pointer exceptions. With !:
, developers can even throw exceptions if a null value is encountered.
3. Extension Functions
Kotlin introduces the concept of extension functions, allowing developers to add new functionality to existing classes without altering their code.
fun String.addExclamation() = this + "!"
fun main() {
println("Hello".addExclamation())
}
In this code snippet, the addExclamation
function extends the String
class, demonstrating how Kotlin can empower you to enhance libraries or frameworks. This feature significantly improves code readability and maintainability.
4. Coroutines for Asynchronous Programming
Managing asynchronous programming in Java can lead to cumbersome callback hell. Kotlin simplifies this process with coroutines, which allow developers to write asynchronous code sequentially.
import kotlinx.coroutines.*
fun main() = runBlocking {
launch {
delay(1000L)
println("World!")
}
println("Hello,")
}
The code above shows how coroutines can help manage asynchronous tasks much more cleanly. With less boilerplate and better readability, developers can focus on solving problems rather than managing application states.
5. Data Classes
In Java, creating a simple data holder requires writing several boilerplate methods such as equals()
, hashCode()
, and toString()
. In Kotlin, the data class
eliminates this redundancy.
data class User(val name: String, val age: Int)
fun main() {
val user = User("Alice", 30)
println(user) // User(name=Alice, age=30)
}
Here, Kotlin automatically generates the toString()
, equals()
, and other methods based on the properties defined in the data class
. This focus on immutability and simplicity allows developers to create clearer and more concise code.
6. Smart Casts
Java requires explicit casting in many situations, which often clutters the codebase. Kotlin simplifies this through smart casts, allowing developers to work with types more fluidly.
fun handleSomeInput(input: Any) {
if (input is String) {
println(input.length) // No explicit cast needed here
}
}
In this example, once we check that input
is of type String
, Kotlin smartly casts it for us. This feature enhances code readability while maintaining type safety.
7. Properties and Getters/Setters
Kotlin simplifies the way you create fields and their accompanying accessor methods (getters/setters) compared to Java.
class Person(var name: String)
fun main() {
val person = Person("Bob")
person.name = "Alice"
println(person.name) // Alice
}
Kotlin allows you to declare properties within the class header, making your code more concise. Furthermore, it allows for easy default getter/setter definitions or custom implementations as needed.
8. First-Class Delegation
Delegation is a powerful design pattern in object-oriented programming, and Kotlin supports it natively. This enables code reuse and separation of concerns effectively.
interface Base {
fun print()
}
class BaseImpl(val x: Int) : Base {
override fun print() {
println(x)
}
}
class Derived(b: Base) : Base by b
fun main() {
val b = BaseImpl(10)
Derived(b).print() // 10
}
In the above example, Derived
delegates the print
function to an instance of Base
, reducing boilerplate and promoting composition over inheritance.
9. Higher-Order Functions and Lambdas
Kotlin embraces functional programming, allowing for concise and powerful manipulation of collections with higher-order functions.
val numbers = listOf(1, 2, 3, 4, 5)
val doubledNumbers = numbers.map { it * 2 }
println(doubledNumbers) // [2, 4, 6, 8, 10]
By using higher-order functions like map
, developers can easily transform data sets. Lambdas in Kotlin are clean and intuitive, resulting in less verbose code compared to Java’s anonymous inner classes.
10. Named Parameters and Default Arguments
The ability to use named parameters and default arguments makes Kotlin functions easier to read and use.
fun displayMessage(message: String, prefix: String = "Info") {
println("$prefix: $message")
}
fun main() {
displayMessage("Hello") // Using default prefix
displayMessage("Hello", "Alert") // Custom prefix
}
Here, the displayMessage
function has a default argument for prefix
. This flexibility allows developers to call the function in a more intuitive way without being forced to stick to all parameters.
Closing Remarks
Kotlin provides an array of features that enhance development efficiency and improve code quality. From seamless interoperability with Java to modern constructs like coroutines and delegation, Kotlin empowers developers to write clean, concise, and maintainable code.
If you are a Java developer looking to step into the Kotlin world, these features can be powerful allies. Embrace Kotlin and harness its modern programming paradigms to elevate your software projects.
For further reading on Kotlin and how to integrate it into your projects, you can check out the official Kotlin documentation or explore resources on Kotlin for Java Developers.
Happy coding!
Checkout our other articles