Mastering Java Debugging in Eclipse: A Step-by-Step Guide
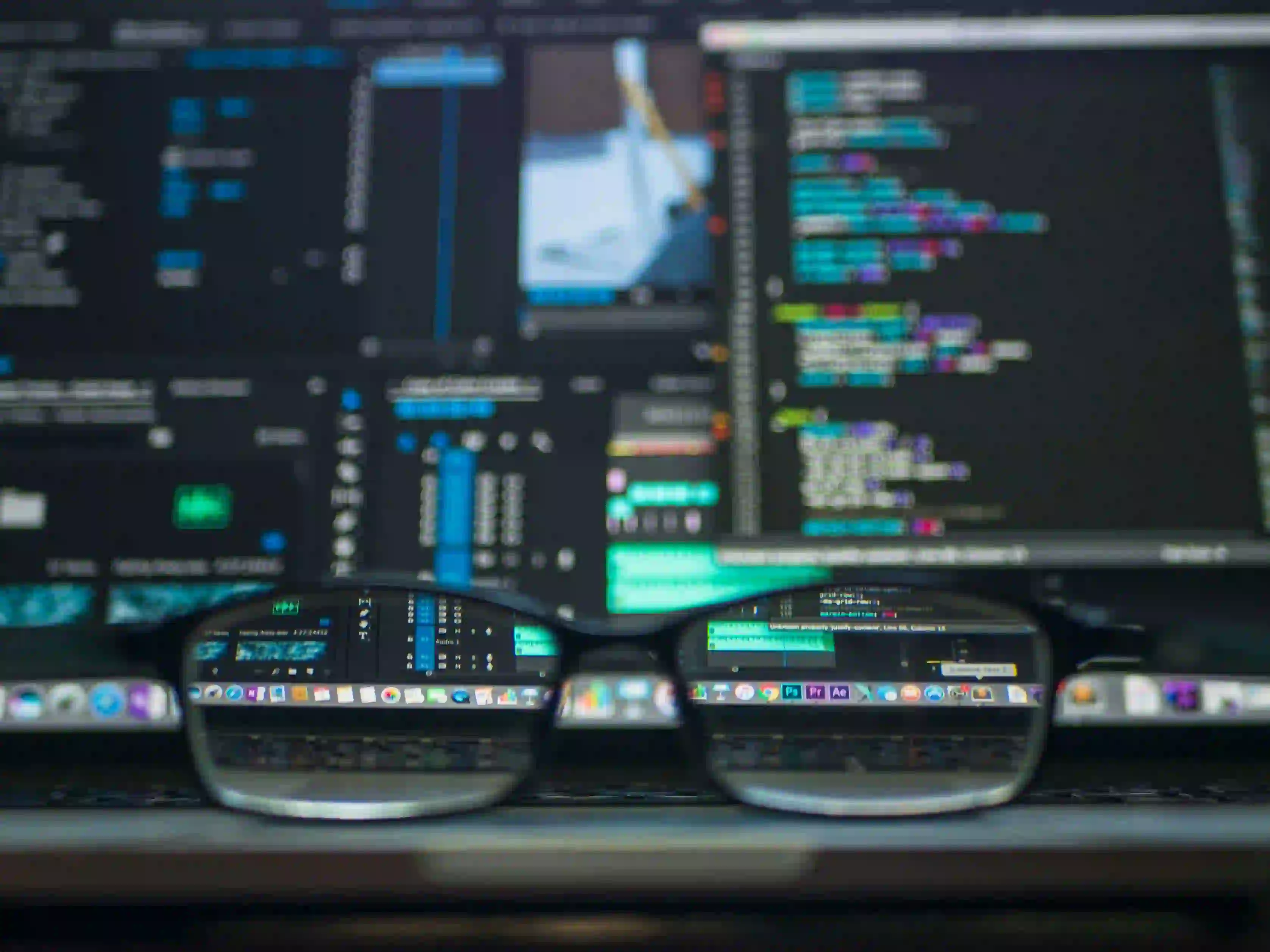
Mastering Java Debugging in Eclipse: A Step-by-Step Guide
Debugging is an essential skill for any software developer. It’s the process of identifying and rectifying errors in your code, which can be particularly challenging, especially in large applications. Eclipse, one of the most widely used Integrated Development Environments (IDEs) for Java, offers robust debugging tools that can simplify this process.
In this guide, we will discuss the various features in Eclipse that can help you debug Java applications effectively. Whether you are a seasoned developer or a beginner, mastering these techniques will significantly enhance your coding efficiency and code quality.
Table of Contents
- Understanding the Basics of Debugging
- Setting Up Your Eclipse Environment
- Using Breakpoints
- Step Through Your Code
- Inspecting Variables
- Viewing the Call Stack
- Conditional Breakpoints
- Exception Breakpoints
- Using Debug Perspectives
- Conclusion
1. Understanding the Basics of Debugging
Debugging is not just about finding bugs; it involves understanding code behavior and reasoning about its execution process. Some common types of bugs include:
- Syntax Errors
- Logical Errors
- Runtime Errors
Each type of error requires a different debugging approach. For example, syntax errors are usually simple to fix as the compiler flags them, while runtime errors can be more complex. Understanding this can help you approach debugging strategically rather than just reactively.
2. Setting Up Your Eclipse Environment
Before diving into debugging, make sure Eclipse is correctly set up for Java development. You can download Eclipse IDE for Java Developers from the official site.
Installing Eclipse:
- Visit the Eclipse Downloads page.
- Choose the version suitable for your operating system.
- Follow the installation instructions provided.
Once installed, you can create a new Java project to start debugging by navigating to:
- File > New > Java Project.
3. Using Breakpoints
Breakpoints are one of the most powerful features for debugging. They allow you to pause program execution at a specific line of code, enabling you to examine the program's behavior.
Setting a Breakpoint:
To set a breakpoint in Eclipse:
- Open your Java file in the editor.
- Click in the left margin next to the line number where you want to set the breakpoint. A blue circle will appear, indicating a breakpoint is set.
When you run your program in debug mode, execution will pause at this point.
Sample Code with Breakpoint:
public class DebugExample {
public static void main(String[] args) {
int sum = add(5, 10); // Set a breakpoint here
System.out.println("The sum is: " + sum);
}
public static int add(int a, int b) {
return a + b;
}
}
4. Step Through Your Code
Once a breakpoint is activated, you can step through your code line by line. This allows you to observe how your variables change and how your program flows.
Step Controls:
Fight bugs effectively by using the following controls:
- F5 (Step Into): Dive into methods called within your current line.
- F6 (Step Over): Execute the current line and go to the next line in the current method.
- F7 (Step Return): Execute the remaining lines of the current method and return to the calling method.
5. Inspecting Variables
While stepping through your code, inspecting variables is crucial to understand their state.
Checking Variables:
- Hover over the variable to view its current value.
- Use the "Variables" view in the Eclipse Debug Perspective to see all local variables and their values.
This helps you pinpoint if a variable is behaving as expected.
6. Viewing the Call Stack
The call stack shows the method execution path leading to the current line where the debugger has paused. Understanding the call stack is essential to trace back the flow of your program.
Using the Call Stack:
- Navigate to the "Debug" view in Eclipse.
- Click on "Debug" and observe the call stack on the left side.
7. Conditional Breakpoints
Conditional breakpoints pause execution only when specific conditions are met. This feature is particularly useful when dealing with loops or when a bug occurs under certain states.
Creating Conditional Breakpoints:
- Right-click the breakpoint.
- Select "Breakpoint Properties" and check “Enable Condition”.
- Enter a boolean condition (e.g.,
x == 10
).
for (int x = 0; x < 20; x++) {
// Set conditional breakpoint here, e.g., only when x == 10
System.out.println(x);
}
8. Exception Breakpoints
An exception breakpoint is triggered when a specific exception is thrown, allowing you to interrupt execution when an error occurs.
Setting Exception Breakpoints:
- In the "Breakpoints" view, right-click and choose "Add Java Exception Breakpoint".
- You can specify which exceptions to monitor, such as
NullPointerException
.
9. Using Debug Perspectives
The Eclipse Debug Perspective is designed to help you focus on debugging tasks. This perspective provides quick access to views like:
- Variables: Show all variables in the current context.
- Breakpoints: Manage your breakpoints.
- Console: View system outputs and errors.
Switch to Debug Perspective by clicking on Window > Perspective > Open Perspective > Debug.
10. Conclusion
Debugging in Eclipse is a powerful way to optimize your development process. By mastering breakpoints, inspecting variables, and utilizing the Eclipse Debug Perspective, you can quickly pinpoint and fix issues in your code.
Additional Resources
In summary, debugging is an art as much as it is a science. Equip yourself with these skills, and you can tackle even the most complex bugs with confidence. Happy coding!