Understanding the Pitfalls of Java's Volatile Keyword
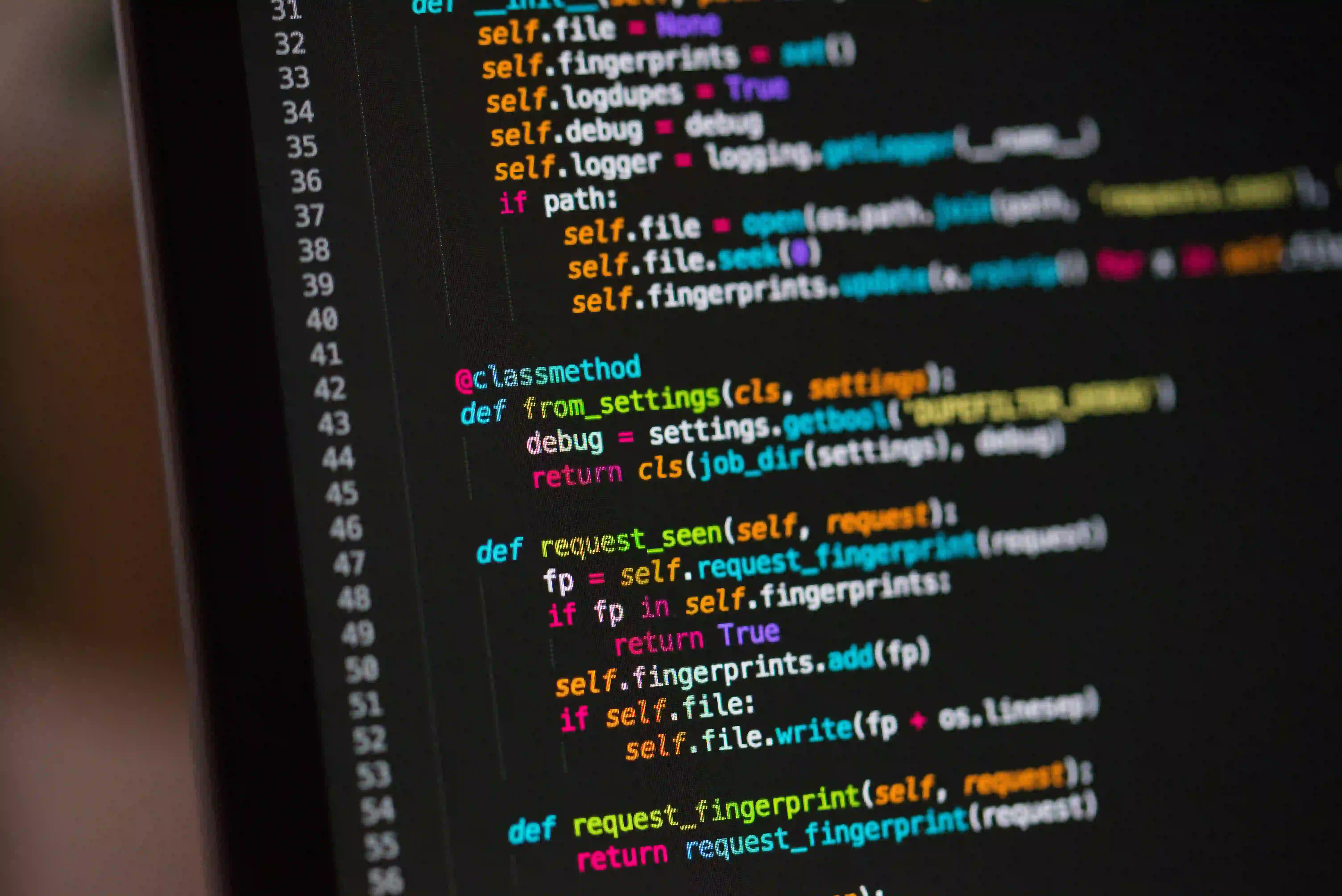
Understanding the Pitfalls of Java's Volatile Keyword
The volatile
keyword in Java is commonly used in concurrent programming. Its primary function is to ensure visibility of changes to variables across threads. However, it's essential to understand that while volatile
provides some guarantees, it is not a silver bullet. In this post, we'll delve deep into what volatile
is, the guarantees it provides, and most importantly, the pitfalls that developers often encounter.
What is the Volatile Keyword?
In Java, the volatile
keyword is a modifier for a variable. When a variable is defined as volatile
, it tells the Java Virtual Machine (JVM) that the variable can be changed unexpectedly by other threads. Here’s an example:
public class Counter {
private volatile int count = 0;
public void increment() {
count++;
}
public int getCount() {
return count;
}
}
Why Use Volatile?
The main reason to use volatile
is to ensure that changes to a variable are visible to all threads. Without volatile
, a thread may cache a variable in its local memory and not see changes made by other threads, leading to inconsistent states.
Important Guarantees of Volatile
-
Visibility: Changes made by one thread to a
volatile
variable are visible to other threads. In simple terms, if one thread updates avolatile
variable, other threads immediately see the new value. -
Atomicity of Reads and Writes: A read or write operation to a
volatile
variable is atomic. However, it’s crucial to note that operations that involve multiple steps (like incrementing a counter) are not atomic.
The Pitfalls of Using Volatile
While volatile
can be useful, it comes with its own set of caveats. Let’s explore some of the most common pitfalls:
1. No Atomicity for Compound Actions
The most significant pitfall is that volatile
does not provide atomicity for compound actions—such as check-then-act or read-modify-write sequences.
For instance, consider this incorrect approach:
public class VolatileCounter {
private volatile int count = 0;
public void increment() {
count++; // Not atomic
}
public int getCount() {
return count;
}
}
In this example, the count++
operation involves three separate actions: reading the current value, incrementing it, and storing it back. If two threads execute increment()
simultaneously, it could lead to a lost update, where both threads read the same value before either has updated it.
Solution
Use synchronization for compound actions:
public class SynchronizedCounter {
private int count = 0;
public synchronized void increment() {
count++; // Atomic due to synchronization
}
public synchronized int getCount() {
return count;
}
}
2. Not a Replacement for Synchronization
Many developers mistakenly believe that using volatile
is a way to avoid synchronization mechanisms entirely. However, while volatile
handles visibility, it does not ensure mutual exclusion. If multiple threads can modify the state of an object, you still need to use synchronization to avoid race conditions.
3. Unpredictable Behavior
The volatile
keyword might lead to unpredictable behavior when it comes to ordering of operations. The Java Memory Model guarantees visibility but does not guarantee that all operations will be ordered as they appear in the code. For instance, when using volatile
, a thread may not see the results of a prior write if that write was to a non-volatile variable.
class VolatileExample {
private volatile boolean flag = false;
private int value = 0;
public void writer() {
value = 42; // Non-volatile write
flag = true; // Volatile write
}
public void reader() {
if (flag) {
// It is possible that value is still 0 here
System.out.println(value);
}
}
}
In this example, upon checking flag
, a thread may not see the updated value of value
. This behavior often leads to subtle bugs that can be difficult to trace.
4. Misleading Performance Expectations
Some developers believe that because volatile
variables are faster than locks (due to the lack of context switching), they can use it in high-performance code. However, this is often a misunderstanding. The performance gain is typically negligible compared to the risk of introducing concurrency issues.
When to Use Volatile
Despite these pitfalls, volatile
has its rightful place in concurrent programming. Here are some scenarios where volatile
is appropriate:
- Flags: When you need a simple "on/off" flag shared between threads.
- Single Assignment: When a variable will be assigned once after construction or initialization.
- State indicators: When you have a state variable that does not require complex operations.
public class FlagExample {
private volatile boolean running = true;
public void stop() {
running = false;
}
public void run() {
while (running) {
// Do work
}
}
}
To Wrap Things Up
The volatile
keyword in Java can be a valuable tool for managing visibility between threads. However, its improper use can lead to subtle and complex bugs. Understanding the guarantees it provides—and its limitations—is crucial for effective concurrent programming.
For a deeper dive into Java memory management and concurrency, consider checking out the official Java documentation and the Java Concurrency in Practice book by Brian Goetz.
By using volatile
wisely and understanding its limitations, developers can write safer and more maintainable concurrent Java applications. Always remember: when in doubt, reach for synchronization tools like synchronized
, Lock
, or Atomic
classes to ensure safety in concurrent contexts.