Common Pitfalls in Building a Jersey Hello World Service
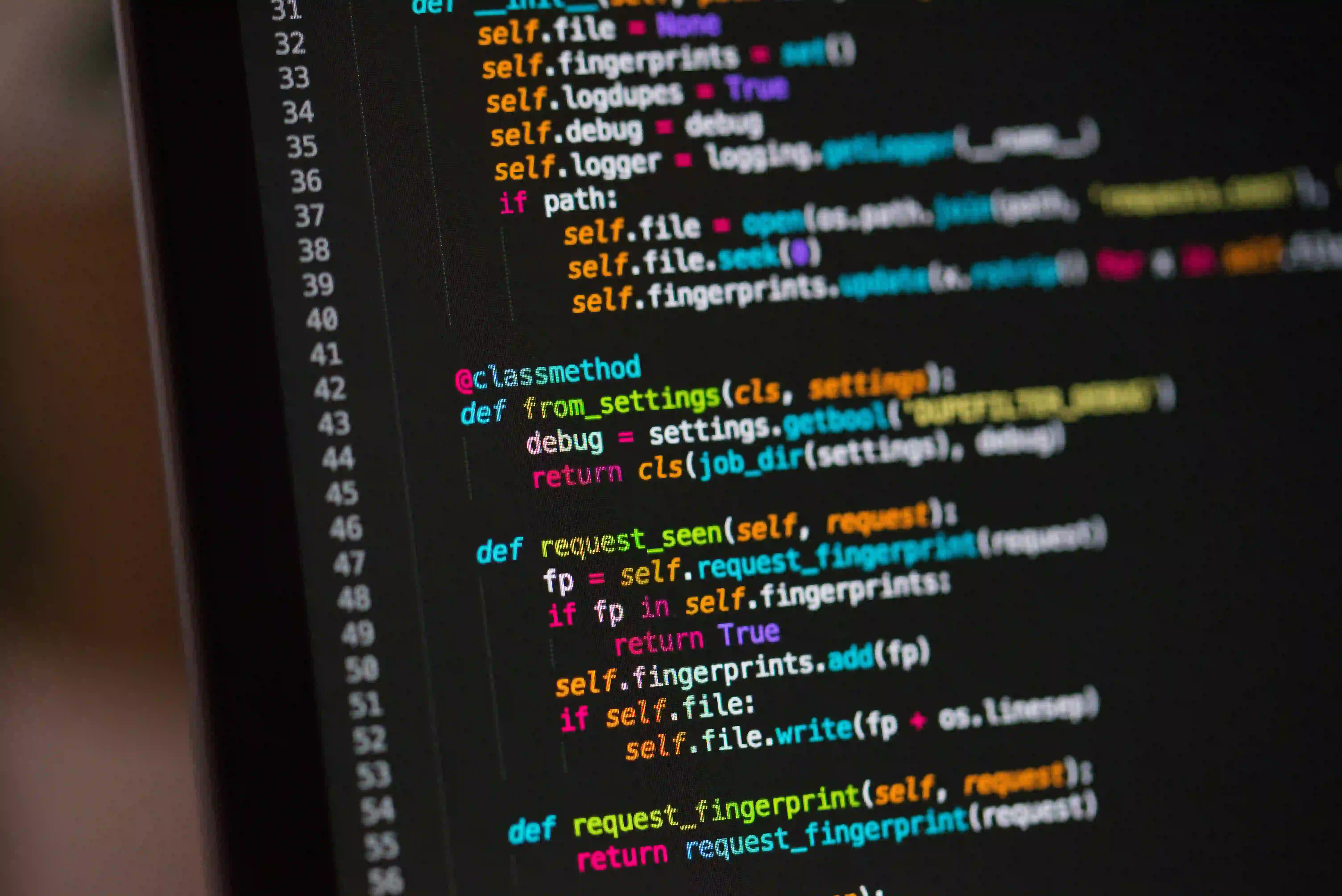
Common Pitfalls in Building a Jersey Hello World Service
Starting Off
Java developers often use the Jersey framework when building RESTful web services. Jersey is an open-source project from Oracle that provides a powerful, flexible way to build, consume, and test REST APIs. While creating a simple "Hello World" service seems straightforward, there are common pitfalls that developers frequently encounter.
In this blog post, we'll explore these pitfalls, illustrate them with code snippets, and provide strategies to avoid these issues. By the end, you'll have a clearer insight into building a Jersey service effectively.
Setting Up Your Project
Before diving into the pitfalls, let's set up a minimal project structure. We'll include the necessary dependencies for Jersey. If you're using Maven, your pom.xml
might look like this:
<dependencies>
<dependency>
<groupId>org.glassfish.jersey.containers</groupId>
<artifactId>jersey-container-servlet-core</artifactId>
<version>2.35</version>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.inject</groupId>
<artifactId>jersey-hk2</artifactId>
<version>2.35</version>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>4.0.1</version>
<scope>provided</scope>
</dependency>
</dependencies>
Pitfall 1: Incorrect Application Configuration
The first and most frequent misstep is failing to configure your Jersey application correctly. This includes using the right class to extend and ensuring correct annotation usage.
Example:
import org.glassfish.jersey.server.ResourceConfig;
import javax.ws.rs.ApplicationPath;
@ApplicationPath("api")
public class MyApplication extends ResourceConfig {
public MyApplication() {
packages("com.example");
}
}
Why it matters:
Failing to set the @ApplicationPath
annotation can lead to a 404 error when accessing your service. If Jersey doesn't know the base URI for your endpoints, it cannot route requests properly.
Creating a Basic Resource Class
Next, you will create your resource class that handles HTTP requests.
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Path("hello")
public class HelloWorldResource {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String sayHello() {
return "Hello, World!";
}
}
Pitfall 2: Forgetting Media Type Annotations
It's crucial to specify the media type for the responses using the @Produces
annotation. Failing to do so can confuse clients about the response format.
Why it matters: If your response format isn't explicitly stated, clients might misinterpret the data, leading to unexpected behavior in their applications.
Testing Your Service
Once your service is configured, testing ensures your "Hello World" function works as expected. Use tools like Postman or simply curl for quick testing.
curl http://localhost:8080/api/hello
Pitfall 3: Server Misconfiguration
Developers often encounter issues where the service is not accessible due to misconfigured server settings.
Example:
If you're using embedded Jetty, ensure you correctly bind it to the desired port:
import org.eclipse.jetty.server.Server;
import org.eclipse.jetty.servlet.ServletContextHandler;
import org.glassfish.jersey.servlet.ServletContainer;
public class Main {
public static void main(String[] args) throws Exception {
Server server = new Server(8080);
ServletContextHandler context = new ServletContextHandler(ServletContextHandler.SESSIONS);
context.setContextPath("/api");
server.setHandler(context);
context.addServlet(new ServletHolder(new ServletContainer(new MyApplication())), "/*");
server.start();
server.join();
}
}
Why it matters: If your server doesn't run on the expected port or cannot bind properly, your application will be inaccessible.
Pitfall 4: Handling Exceptions Poorly
When building RESTful services, proper exception handling is critical for a good user experience.
Example:
You can use an exception mapper to return meaningful error responses:
import javax.ws.rs.NotFoundException;
import javax.ws.rs.core.Response;
import javax.ws.rs.ext.ExceptionMapper;
import javax.ws.rs.ext.Provider;
@Provider
public class MyExceptionMapper implements ExceptionMapper<NotFoundException> {
@Override
public Response toResponse(NotFoundException exception) {
return Response.status(Response.Status.NOT_FOUND)
.entity("Resource not found.")
.build();
}
}
Why it matters: Improper exception handling can lead to poor user experience or expose sensitive information. Providing meaningful error messages enhances the API usability.
Understanding Dependency Injection
With Jersey, you can also leverage dependency injection to make your services cleaner and facilitate testing.
Pitfall 5: Poor Management of Dependencies
Using DI tools like HK2 is essential but can create complexity if you're unaware of lifecycle management.
Example:
import javax.inject.Inject;
public class HelloWorldService {
private final SomeDependency someDependency;
@Inject
public HelloWorldService(SomeDependency someDependency) {
this.someDependency = someDependency;
}
public String getGreeting() {
return someDependency.getDefaultGreeting();
}
}
Why it matters: Not managing dependencies well can lead to memory leaks or unexpected behavior during service calls.
To Wrap Things Up
Building a Jersey Hello World service should be a straightforward experience, but as we've discussed, several common pitfalls can derail your efforts. Whether it's misconfigurations, poor exception handling, or dependency mismanagement, these issues can complicate the development process.
By following best practices and being mindful of these pitfalls, you'll be better prepared to build responsive, robust RESTful services with Java and Jersey.
Be sure to stay updated with Jersey's evolving documentation at Jersey Documentation and explore various community resources to expand your understanding further.
Additional Resources
- RESTful Web Services with Jersey
- Best Practices for Designing a RESTful API
Feel free to reach out with questions or comments below! Happy coding!