Mastering Complexity: Challenges with Abstract Factory Pattern
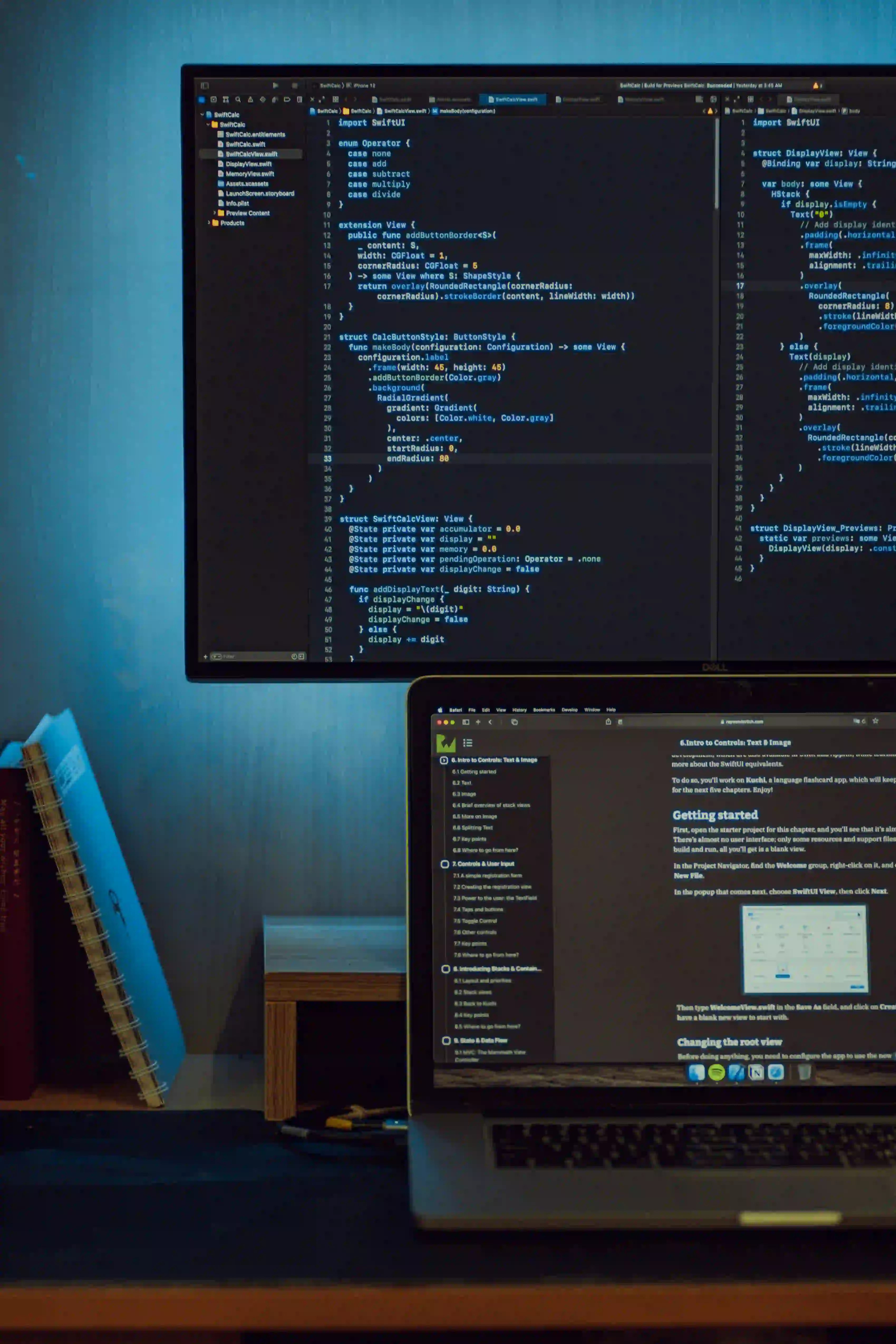
Mastering Complexity: Challenges with the Abstract Factory Pattern in Java
In software design, flexibility and maintainability often stand at odds with complexity. When using design patterns, it's crucial to balance these aspects. One such complex yet powerful design pattern is the Abstract Factory Pattern. In this article, we will delve deeply into the challenges associated with the Abstract Factory Pattern, particularly in Java, and understand why mastering this pattern can be both rewarding and tricky.
Understanding the Abstract Factory Pattern
The Abstract Factory Pattern provides an interface for creating families of related or dependent objects without specifying their concrete classes. This pattern is particularly useful when the client needs to work with multiple types of products from different families.
Key Concepts
- Abstract Factory: This is an interface that declares creation methods for each type of product.
- Concrete Factory: This implements the Abstract Factory and creates products relevant to a specific family.
- Abstract Product: This is the interface for products that the factories produce.
- Concrete Product: These are the classes that implement the Abstract Product interface and define specific variations of products.
Example Overview
Consider a scenario where we need to create a user interface for different operating systems, such as Windows and MacOS. We could define factories for each operating system that produces a set of compatible UI components.
Code Implementation
Let's discuss how the Abstract Factory Pattern looks in Java.
// Step 1: Define the Abstract Product interfaces
interface Button {
void paint();
}
interface Checkbox {
void check();
}
// Step 2: Create Concrete Products for Windows
class WinButton implements Button {
public void paint() {
System.out.println("Rendering button in Windows style.");
}
}
class WinCheckbox implements Checkbox {
public void check() {
System.out.println("Checking Windows checkbox.");
}
}
// Step 3: Create Concrete Products for MacOS
class MacButton implements Button {
public void paint() {
System.out.println("Rendering button in MacOS style.");
}
}
class MacCheckbox implements Checkbox {
public void check() {
System.out.println("Checking Mac checkbox.");
}
}
// Step 4: Define the Abstract Factory
interface GUIFactory {
Button createButton();
Checkbox createCheckbox();
}
// Step 5: Implement Concrete Factories
class WinFactory implements GUIFactory {
public Button createButton() {
return new WinButton();
}
public Checkbox createCheckbox() {
return new WinCheckbox();
}
}
class MacFactory implements GUIFactory {
public Button createButton() {
return new MacButton();
}
public Checkbox createCheckbox() {
return new MacCheckbox();
}
}
// Step 6: Use the Abstract Factory
class Application {
private Button button;
private Checkbox checkbox;
public Application(GUIFactory factory) {
button = factory.createButton();
checkbox = factory.createCheckbox();
}
public void paint() {
button.paint();
checkbox.check();
}
}
Code Commentary
In the provided code, the Abstract Factory Pattern allows us to separate the creation logic of various UI components from their usage. We can easily extend our application to support new operating systems by adding new concrete products and concrete factories without modifying existing code.
Challenges with the Abstract Factory Pattern
Although the Abstract Factory Pattern offers a robust solution for creating families of related objects, it comes with its share of challenges. Understanding these challenges is vital for effectively implementing this design pattern.
1. Increased Complexity
Each additional product type requires the declaration of an interface and its implementation. This can lead to an explosion of classes, making the codebase more complex and challenging to manage.
2. Difficulty in Maintenance
When changes are necessary—say, if a new product type is introduced—the abstract factory needs to be updated accordingly, which could cause a ripple effect throughout the codebase.
3. Rigidity
The introduction and removal of products require careful adjustments to the factory interfaces. This rigidity can lead to difficulties in scaling or evolving the system. If you decide to expand your product family, you may need to alter existing abstractions, which violates the Open-Closed Principle.
4. Testing Challenges
Unit testing classes that rely on factories can be cumbersome. The dependencies on the factory make it less straightforward to mock objects and dependencies.
5. Learning Curve
For developers new to design patterns, the Abstract Factory can be challenging to understand and implement correctly, especially if they have little experience with dependency injection and polymorphism.
Best Practices to Mitigate Challenges
To leverage the strengths of the Abstract Factory Pattern while minimizing its inherent challenges, consider the following best practices:
Favor Composition Over Inheritance
Use composition over inheritance wherever possible. Instead of creating a large hierarchy of factories, you can compose factory classes with smaller, specialized implementations that conform to single responsibilities.
Utilize Reflection
In certain scenarios, using Java Reflection can provide more dynamic control over object creation. It can reduce the amount of manual boilerplate code needed for factory methods.
Make Extensive Use of Interfaces
Interfaces should be used extensively to define product types. This not only adds flexibility to your design but also keeps your factories understandable.
Embrace Dependency Injection
Incorporating a Dependency Injection (DI) framework can simplify the management of object creation. Using frameworks like Spring allows you to keep your factories clean and organized.
Emphasize Documentation
Due to the increased complexity associated with this pattern, thorough documentation surrounding your factories and product family structures is vital for ensuring maintainability and ease of understanding.
Key Takeaways
The Abstract Factory Pattern is a powerful tool that, when used judiciously, can lead to flexible and scalable software design. However, its complexity can hinder both maintainability and adaptability. Awareness of these challenges leads to better preparation and more thoughtful architectural choices.
As you navigate these complexities, remember that the goal of software design should always be to create solutions that are both maintainable and scalable while resisting unnecessary complexity. By understanding the nuances of the Abstract Factory Pattern, you pave the way for more robust Java applications.
For those who wish to dive deeper into design patterns in Java, I recommend resources like Refactoring Guru or the classic book Design Patterns: Elements of Reusable Object-Oriented Software by the Gang of Four.
Happy coding!