Conquer GC Overhead: 5 Hacks to Boost Performance
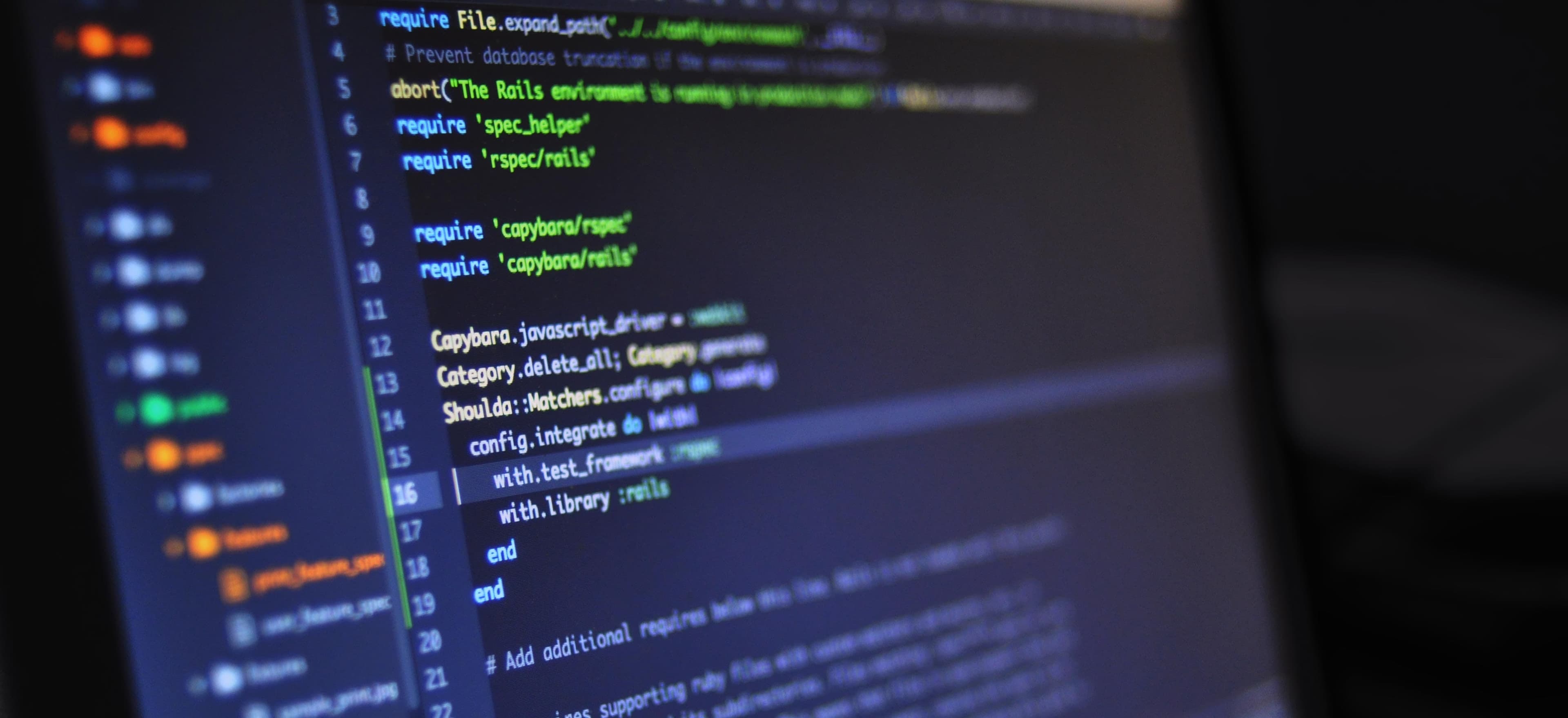
- Published on
Conquer GC Overhead: 5 Hacks to Boost Performance
Java is a powerful, versatile language widely used in enterprise applications. However, one of the common challenges developers face is Garbage Collection (GC) overhead. GC helps manage memory by automatically freeing up unused memory, but it can also lead to performance bottlenecks if not managed properly.
In this blog post, we will explore five effective hacks to help you reduce GC overhead, enhance your application’s performance, and improve overall efficiency. Alongside, we will include actionable code snippets and insights into why these strategies are effective.
What is Garbage Collection?
Garbage Collection in Java is a process that automatically frees memory by reclaiming objects that are no longer in use. Although it alleviates the burden of manual memory management, excessive GC can lead to latency spikes or application slowdowns, adversely affecting user experience.
Understanding how garbage collection works is vital. The Java Virtual Machine (JVM) performs different types of garbage collections (Young Generation, Old Generation, and Full GC) based on the application's memory usage pattern. Knowing how to manage these generations proactively can have lasting impacts.
1. Optimize Object Creation
One of the most effective hacks to manage GC overhead is optimizing object creation. Excessive instantiation of objects leads to more objects collected, causing increased GC activity.
Code Snippet: Object Pooling
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
class DatabaseConnection {
// Simulated Database Connection
}
// A simple object pool implementation
class ConnectionPool {
private final ExecutorService executorService;
public ConnectionPool(int poolSize) {
this.executorService = Executors.newFixedThreadPool(poolSize);
}
public DatabaseConnection getConnection() {
// Fetch from pool instead of creating a new object
return new DatabaseConnection();
}
// Other pool management functions...
}
Why?: Using an object pool helps to reuse existing objects instead of creating new ones. This reduces memory pressure and minimizes GC overhead, especially in applications with high object instantiation rates.
2. Tune the JVM Options
The JVM offers various options that can allow you to fine-tune the garbage collection behavior. These settings can lead to significant performance improvements.
Key JVM Options:
-Xms
and-Xmx
: Set the initial and maximum heap size (e.g.,-Xms512m -Xmx2g
).-XX:NewRatio
: Controls the ratio between the young and old generation.-XX:+UseG1GC
: Enables the Garbage-First (G1) garbage collector, which is ideal for applications with large datasets.
Code Snippet: Running Your Program with Specific JVM Flags
java -Xms512m -Xmx2g -XX:+UseG1GC -XX:NewRatio=3 -jar your-app.jar
Why?: Tailoring your JVM settings based on your application's needs will help balance memory use, reduce GC times, and enhance overall responsiveness. Always benchmark your application before and after to ensure the changes have the intended effect.
3. Use Immutable Objects
Creating immutable objects can drastically decrease the number of live objects in memory, which simplifies the garbage collection process. Immutable objects are inherently thread-safe, leading to fewer synchronization issues in multi-threaded applications.
Code Snippet: Creating an Immutable Class
public final class User {
private final String name;
private final int age;
public User(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
Why?: Because immutable objects cannot change state, they often can be reused, resulting in less frequent allocation of new objects. This can help reduce memory fragmentation and optimize GC times.
4. Minimize the Use of Finalizers
Finalizers are methods that execute just before an object is garbage collected. However, they're notorious for causing latency and should be avoided if possible.
Alternative: Implementing AutoCloseable
class Resource implements AutoCloseable {
public void useResource() {
// Logic for using the resource
}
@Override
public void close() {
// Cleanup code
}
}
// Using try-with-resources
try (Resource resource = new Resource()) {
resource.useResource();
}
Why?: Using AutoCloseable
with try-with-resources ensures that resources are managed safely and efficiently without relying on the garbage collector for cleanup, minimizing overhead during GC cycles.
5. Leverage Profiling Tools
Profiling tools help you identify memory usage patterns and potential bottlenecks in your application. Tools like VisualVM, Eclipse Memory Analyzer (MAT), and Java Flight Recorder provide insights into memory allocation and garbage collection.
Using VisualVM
- Download and install VisualVM.
- Attach it to your running Java application.
- Monitor memory usage and look for heavy object allocation patterns.
Why?: Regularly profiling your application allows you to make informed optimizations instead of relying on guesswork, directly targeting GC overhead and memory leaks.
In Conclusion, Here is What Matters
By combining these five hacks—optimizing object creation, tuning JVM options, using immutable objects, minimizing finalizers, and leveraging profiling tools—you can significantly reduce GC overhead in your Java applications. This will not only improve performance but also enhance the overall user experience.
We all know that Java's automatic memory management is a powerful feature. However, understanding and managing garbage collection can take your application's performance to the next level.
Additional Resources
- Understanding Java Garbage Collection
- Exploring the New Garbage Collectors in Java
- Java Performance Tuning Tips
By deploying these techniques effectively, you will be well-equipped to conquer GC overhead and enhance your Java applications significantly. Happy coding!
Checkout our other articles