Creating a Fluent Design Style Toggle Switch in JavaFX
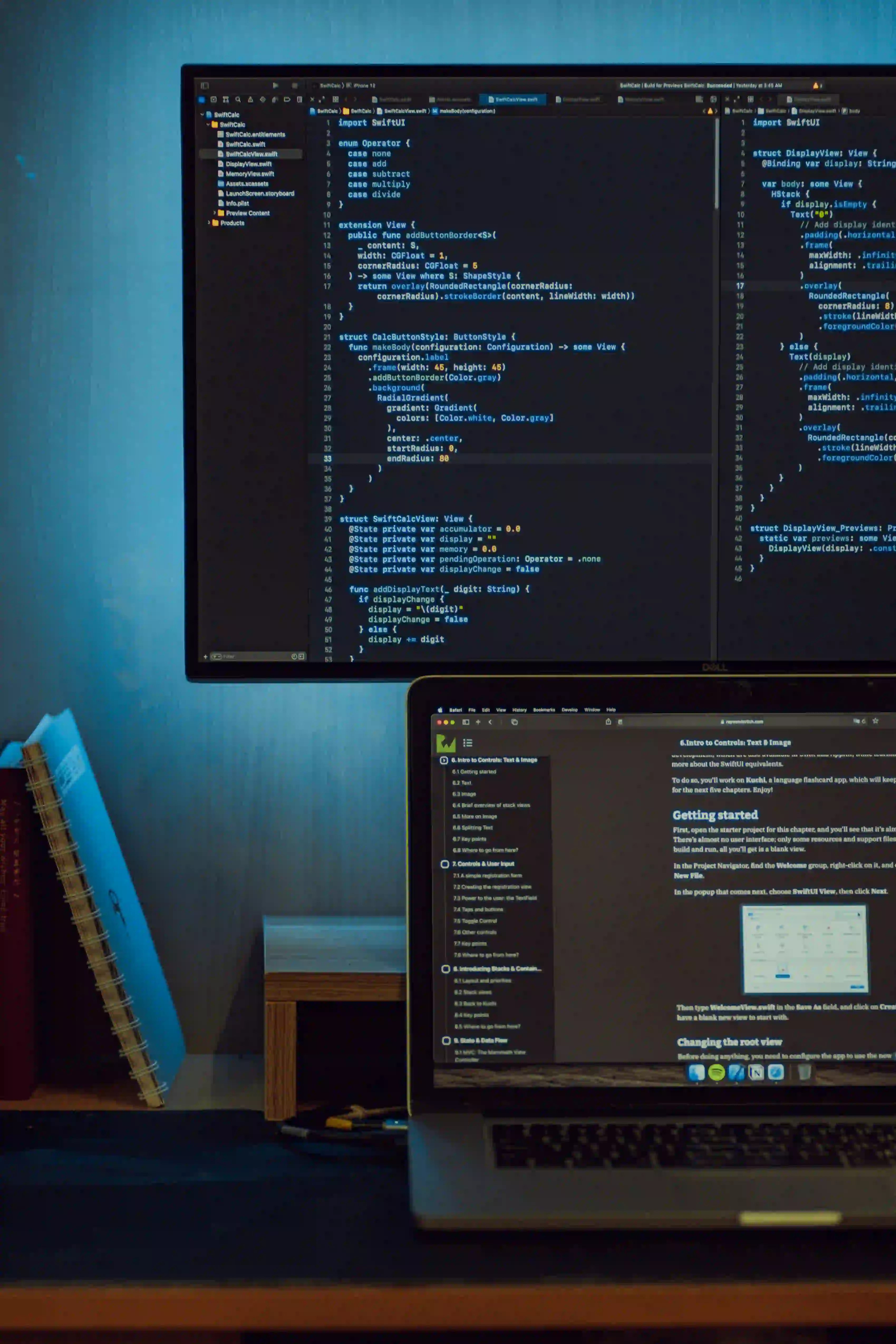
Creating a Fluent Design Style Toggle Switch in JavaFX
As the demand for modern and visually appealing applications increases, developers are constantly looking for ways to enhance the user interface of their JavaFX applications. One popular approach is to incorporate Fluent Design principles, which provide an elegant and intuitive experience. In this blog post, we will delve into the process of creating a Fluent Design style toggle switch in JavaFX, enhancing both functionality and aesthetics.
Table of Contents
- Overview of Fluent Design
- Setting Up the JavaFX Project
- Designing the Toggle Switch
- Implementing Functionality
- Conclusion and Further Reading
1. Overview of Fluent Design
Fluent Design is a design language created by Microsoft that focuses on light, depth, motion, and materials to create a cohesive and engaging interface. It emphasizes a clean aesthetic, with elements that feel dynamic and interconnected. This design principle provides a great foundation for building modern UIs in JavaFX.
Key Principles of Fluent Design:
- Light: Use light for depth and clarity.
- Depth: Create layers within the UI to provide a sense of hierarchy.
- Motion: Incorporate subtle motion to direct the user’s attention.
- Material: Utilize materials to create tactile experiences.
By integrating these principles, your JavaFX application can deliver a more engaging user experience.
2. Setting Up the JavaFX Project
Before we dive into the coding part, ensure that you have a JavaFX environment set up in your development environment. You can use an IDE such as IntelliJ IDEA or Eclipse. Here’s how to create a simple JavaFX project:
Gradle Setup
You might want to use Gradle to manage your JavaFX project dependencies easily. Here’s a simple build.gradle
configuration:
plugins {
id 'application'
}
repositories {
mavenCentral()
}
dependencies {
implementation 'org.openjfx:javafx-controls:17'
}
application {
mainClass = 'com.example.Main'
}
This configuration pulls in JavaFX controls for your user interface. After this setup, you are ready to start coding!
3. Designing the Toggle Switch
Let’s create a basic structure for our toggle switch using JavaFX's UI components. The toggle switch will consist of a rectangle and a circle to represent the on/off states.
Basic Structure
First, we will set up the main UI container and create the toggle switch components.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
public class ToggleSwitch extends Application {
@Override
public void start(Stage primaryStage) {
StackPane root = new StackPane();
Scene scene = new Scene(root, 300, 200);
// Toggle Switch Components
Rectangle background = new Rectangle(60, 30, Color.LIGHTGRAY);
Circle knob = new Circle(15, Color.WHITE);
// Position the knob
knob.setTranslateX(-15);
root.getChildren().addAll(background, knob);
primaryStage.setTitle("Fluent Design Toggle Switch");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Explanation of Code
- Rectangle background: Acts as the background of the toggle switch, providing a grey look when off.
- Circle knob: Represents the on/off toggle, which will move to the right when the switch is activated.
4. Implementing Functionality
Now that we have our UI components, we will implement the functionality to make our toggle switch interactive. We want the knob to move when clicked, and change its background color accordingly.
Adding Interactivity
We accomplish this by adding a mouse event listener to the knob:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
public class ToggleSwitch extends Application {
private boolean isOn = false;
@Override
public void start(Stage primaryStage) {
StackPane root = new StackPane();
Scene scene = new Scene(root, 300, 200);
// Toggle Switch Components
Rectangle background = new Rectangle(60, 30, Color.LIGHTGRAY);
Circle knob = new Circle(15, Color.WHITE);
knob.setTranslateX(-15);
// Knob Click Event
knob.setOnMouseClicked(event -> toggleSwitch(background, knob));
root.getChildren().addAll(background, knob);
primaryStage.setTitle("Fluent Design Toggle Switch");
primaryStage.setScene(scene);
primaryStage.show();
}
private void toggleSwitch(Rectangle background, Circle knob) {
isOn = !isOn; // Toggle the state
// Change properties based on the current state
if (isOn) {
knob.setTranslateX(15); // Move knob to the right
background.setFill(Color.GREEN); // Change background color
} else {
knob.setTranslateX(-15); // Move knob back to the left
background.setFill(Color.LIGHTGRAY); // Reset background color
}
}
public static void main(String[] args) {
launch(args);
}
}
Explanation of Interactive Code
- isOn flag: Maintains the current state of the toggle switch.
- toggleSwitch method: Adjusts the position of the knob and the background color when the switch is toggled.
- Event Handling: The
setOnMouseClicked
method allows us to interact with the knob, providing immediate feedback to the user.
5. Conclusion and Further Reading
In this blog post, we explored how to create a Fluent Design style toggle switch in JavaFX from scratch. By utilizing JavaFX's built-in components and simple event handling, we crafted an aesthetically appealing and interactive user interface element.
Next Steps:
- Enhance the toggle switch with animations to make the transitions smoother.
- Explore more about JavaFX layouts and other UI components to build complete applications.
- For more advanced topics in JavaFX, consider the JavaFX Official Documentation and JavaFX Tutorial.
By implementing the toggle switch described in this blog post, you'll not only enhance your JavaFX projects but also gain a better understanding of Fluent Design principles in modern UI development. Happy coding!