Common Pitfalls When Converting String to Int in Java
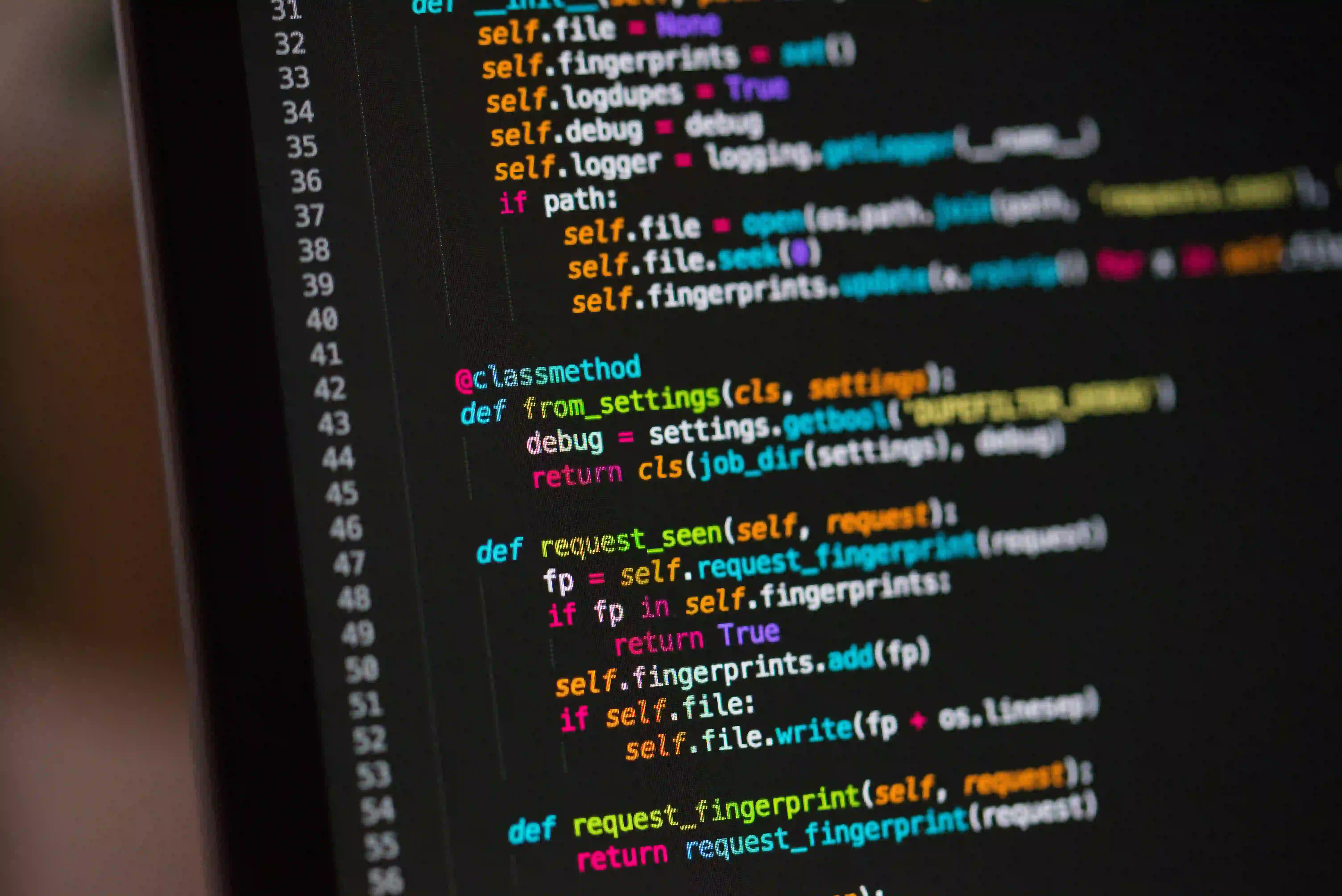
Common Pitfalls When Converting String to Int in Java
Java is a strongly typed language, which means that it requires explicit type conversions. Converting a String
to an int
can frequently lead to pitfalls that many developers, especially newcomers, occasionally overlook. This post will outline these common issues, provide code snippets to illustrate the problems, and offer solutions to mitigate errors. By understanding these caveats, you can handle type conversions robustly and efficiently.
Table of Contents
- Understanding the Basics
- Common Pitfalls
- Non-Numeric Strings
- Overflows
- Using
Integer.parseInt
Incorrectly - Handling Leading and Trailing Spaces
- Best Practices for Conversion
- Conclusion
Understanding the Basics
In Java, converting a String
to an int
is usually carried out using Integer.parseInt(String s)
. This method parses the string argument as a signed decimal integer. Here’s a simple example:
String numStr = "12345";
int number = Integer.parseInt(numStr);
System.out.println(number); // Output: 12345
This code works flawlessly for numeric strings. However, when faced with real-world scenarios, certain strings present challenges.
Common Pitfalls
Non-Numeric Strings
One of the most common pitfalls arises when the String
does not represent a valid integer. If you attempt to convert a non-numeric string, such as "abc", you will encounter a NumberFormatException
.
String invalidStr = "abc";
try {
int number = Integer.parseInt(invalidStr); // This will throw an exception
} catch (NumberFormatException e) {
System.out.println("Invalid number format: " + e.getMessage());
}
Why this matters: The ability to handle exceptions appropriately can prevent your application from crashing. Always validate your input before conversion.
Overflows
Another potential issue occurs when the numeric value in the String
exceeds the range of the int
type, which is between [-2,147,483,648, 2,147,483,647]. If the string represents a larger number, parsing it will also throw a NumberFormatException
.
String largeNumberStr = "3000000000"; // This value exceeds Integer.MAX_VALUE
try {
int number = Integer.parseInt(largeNumberStr);
} catch (NumberFormatException e) {
System.out.println("Overflow error: " + e.getMessage());
}
Solution: To handle large numbers, consider converting the string to a long
type first, then downcast if certain the value fits the int
range:
long largeNumber = Long.parseLong(largeNumberStr);
if (largeNumber <= Integer.MAX_VALUE && largeNumber >= Integer.MIN_VALUE) {
int number = (int) largeNumber;
} else {
System.out.println("Value is out of range for int.");
}
Using Integer.parseInt
Incorrectly
Using parseInt()
on an improperly formatted string, such as a string with a decimal point or other non-integer characters, will lead to an exception.
String decimalStr = "123.45";
try {
int number = Integer.parseInt(decimalStr); // Will throw an exception
} catch (NumberFormatException e) {
System.out.println("Cannot convert decimal string: " + e.getMessage());
}
Why this happens: parseInt()
is strict about input format. Only a well-formatted, non-decimal, numeric string can be successfully parsed.
Handling Leading and Trailing Spaces
Sometimes, input strings may have leading or trailing spaces. While Integer.parseInt()
does ignore leading spaces, trailing spaces may deprive you of a seamless conversion.
String spacedStr = " 12345 ";
int number = Integer.parseInt(spacedStr.trim()); // Using trim() removes surrounding spaces
System.out.println(number); // Output: 12345
Best Practice: Always use trim()
to clean your strings before attempting to parse them.
Best Practices for Conversion
- Validation: Before converting, implement a validation method to check if the string is numeric. This could be done easily using regex:
public static boolean isNumeric(String str) {
return str != null && str.matches("-?\\d+");
}
-
Use Try-Catch Blocks: Wrap your parsing calls in try-catch blocks. This safeguards against unexpected values.
-
Long Type: When anticipating a potentially large number, always use
Long
for parsing and validate whether it fits withinint
bounds. -
Utilize External Libraries: Libraries like Apache Commons Lang offer utility methods for safely converting strings to numbers, managing many pitfalls internally. Check Apache Commons Lang for more tools.
A Final Look
Converting a String
to an int
in Java may seem straightforward, yet it presents several common pitfalls that developers must navigate. Be proactive about error handling, validate input meticulously, and keep an eye on the potential for overflow. By adhering to best practices and leveraging existing libraries, you can significantly enhance the robustness of your Java applications.
For further reading on handling numbers in Java and best practices, consider exploring the official documentation on Java Integer and Handling Exceptions.