Debugging Kivakit Microservices: Common Pitfalls to Avoid
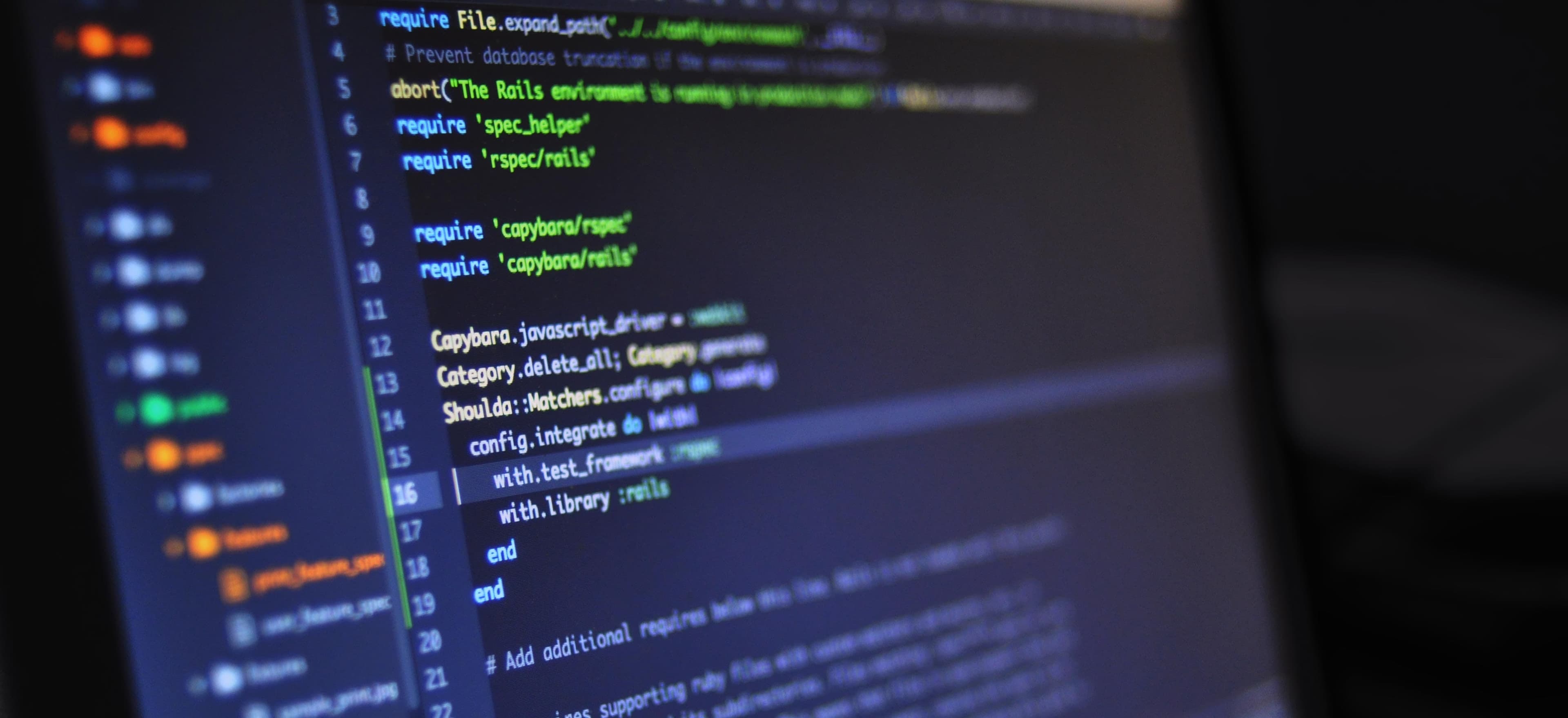
- Published on
Debugging Kivakit Microservices: Common Pitfalls to Avoid
The rise of microservices architecture has transformed how we develop applications. Kivakit, a powerful framework for building microservices in Java, provides developers with a plethora of tools to simplify their tasks. However, with these advancements come significant challenges—especially in debugging. This article will delve into common pitfalls when debugging Kivakit microservices and offer practical solutions to these issues.
Understanding the Kivakit Framework
Before diving into debugging, let's establish a fundamental understanding of the Kivakit framework. Kivakit offers:
- Simplified microservices construction: It abstracts many complexities inherent in distributed systems.
- Rich APIs: Kivakit provides a comprehensive set of APIs for logging, networking, and data handling.
- Move toward simplicity: It promotes clean, maintainable code, contributing to reduced technical debt.
Being familiar with these features can significantly ease debugging efforts, making it essential to understand them before addressing pitfalls.
Common Pitfalls in Debugging Kivakit Microservices
1. Inadequate Logging
Logging is crucial in microservices. Lack of detailed logs can obscure what happens in different services. In Kivakit, proper logging can be implemented using Logger
.
import static com.kivakit.core.logging.LoggerFactory.logger;
public class MyService {
private static final Logger LOGGER = logger(MyService.class);
public void performService() {
LOGGER.info("Service execution started");
try {
// Service logic
LOGGER.info("Service executed successfully");
} catch (Exception e) {
LOGGER.error("Error executing service", e);
}
}
}
Why This Matters: The above code not only logs the success but also catches exceptions and logs errors—all of which can give you context when things go wrong. Ensure your logs are granular enough to provide insights without overwhelming you with information.
2. Ignoring Service Dependencies
Microservices don't operate in isolation. They often depend on one another, and overlooking these dependencies can lead to confusing issues. For instance, if a service relies on a database service that is down, it may cause cascading failures across your microservices.
Solution: Implement circuit breakers using libraries like Hystrix or Resilience4j to handle failures gracefully.
3. Neglecting Configuration Management
Configuration errors are a common pitfall. Inconsistent configurations across environments can lead to unexpected behavior and bugs that are challenging to trace.
import com.kivakit.configuration.Config;
import com.kivakit.configuration.ConfigurationLoader;
public class ConfigReader {
private final Config config;
public ConfigReader() {
this.config = ConfigurationLoader.load("application.properties");
}
public String getDatabaseUrl() {
return config.getProperty("database.url");
}
}
Why This Approach: Using a centralized configuration management system can significantly reduce errors associated with misconfigurations. A sound practice is to separate configuration files for development, testing, and production environments.
4. Overestimating Tooling
There are many tools available for monitoring and debugging microservices. However, relying too heavily on automated tools can be misleading if not used wisely.
- Use tools intentionally.
- Combine automated health checks with manual intervention to gain a broader view.
5. Neglecting Response Handling
In Kivakit, ensuring that your services can handle different response scenarios (success, failure, and error responses) is critical.
public ResponseEntity<String> getUser(Long userId) {
try {
User user = userService.getUserById(userId);
return ResponseEntity.ok(user);
} catch (UserNotFoundException e) {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body("User not found");
} catch (Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("An error occurred");
}
}
Why Handle It This Way? This structure ensures that your microservice provides meaningful feedback instead of vague error messages. It allows the developer (and users) to understand where the fault lies.
6. Failing to Profile Performance
Performance issues in microservices can often be ignored until they become pressing problems. Kivakit can profile and provide metrics to analyze performance across various services.
- Use profiling tools to examine memory usage and response times.
- Sometimes memory leaks can result from poor resource management.
7. Skipping Local Development Testing
While it is essential to test your services in production-like environments, don’t skip local development testing. Unit tests and integration tests can catch many issues before they reach production.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class ConfigReaderTest {
@Test
public void testGetDatabaseUrl() {
ConfigReader configReader = new ConfigReader();
assertEquals("jdbc:mysql://localhost:3306/testdb", configReader.getDatabaseUrl());
}
}
Why Unit Testing? Unit tests enhance confidence in your service's functionality. Writing tests for both positive and negative scenarios ensures that your service behaves as expected.
8. Failure to Scale Tests with Microservices
Unlike traditional monolithic applications, microservices can introduce complexities such as inter-service communication and state management. It’s vital to simulate realistic traffic during tests to reveal potential bottlenecks.
Closing Remarks
Navigating the complexities of debugging Kivakit microservices can be challenging. By recognizing and addressing common pitfalls, you can significantly enhance the robustness of your services. Remember that thorough logging, efficient dependency management, diligent configuration practices, and rigorous testing are paramount.
To delve deeper into debugging and managing Kivakit microservices, you might find the following resources helpful:
- Kivakit Documentation
- Spring Microservices in Action
Equipped with this understanding and best practices, you’ll be better prepared to tackle the challenges of debugging in a microservices architecture. Happy coding!
Checkout our other articles