Mastering Spring Cloud Config: Common Pitfalls to Avoid
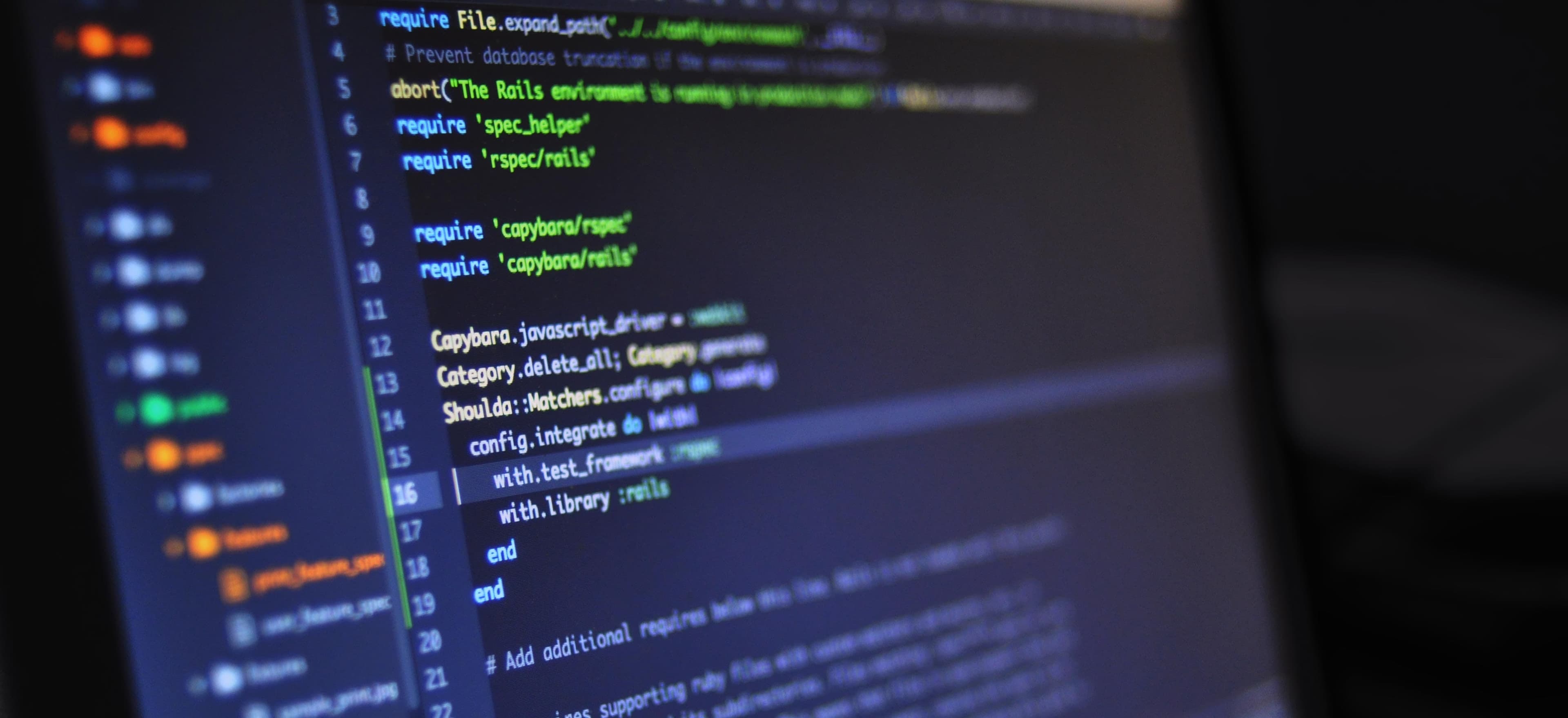
- Published on
Mastering Spring Cloud Config: Common Pitfalls to Avoid
As the microservices architecture trend continues to grow, managing configuration across various services has become a critical task. Spring Cloud Config provides a powerful way to manage external configurations for applications across all environments. However, like any technology, there are common pitfalls developers might encounter. This blog post will guide you through these pitfalls and provide solutions, ensuring you can leverage Spring Cloud Config effectively.
What is Spring Cloud Config?
Spring Cloud Config is a server-side and client-side solution that allows you to manage application settings and configurations in a centralized manner. By maintaining configurations in a Git repository or a local filesystem, you can control the way configurations are loaded based on specific profiles (like dev, test, prod).
Benefits of Using Spring Cloud Config
- Centralized Configuration: Manage all configurations in one place.
- Version Control: Use Git for tracking changes to configurations.
- Dynamic Updates: Change configurations without needing to redeploy the application.
Common Pitfalls to Avoid
Now that we have an understanding of what Spring Cloud Config offers, let's explore frequent mistakes that developers typically make while using it and the solutions to avoid these pitfalls.
1. Misconfiguring the Server URL
One of the most common mistakes is failing to correctly set the Spring Cloud Config server URL in your application properties. The spring.cloud.config.uri
should point to the correct server URL.
spring.cloud.config.uri=http://localhost:8888
Why This Matters: If the URL is incorrect, your application will fail to fetch configurations and will start with default values, potentially causing unexpected behavior.
Solution: Always verify that the Config Server is running, and the URL is accessible. It’s also a good practice to write integration tests that check the availability of the Config Server before deploying your services.
2. Ignoring Security Configurations
Security is often an afterthought in microservices architecture, and the Spring Cloud Config server is no different. By default, your configurations might be publicly accessible.
Why This Matters: Sensitive information, like database passwords and API keys, should never be exposed to unauthorized access.
Solution: Always configure security settings. Here’s an example of how you might secure your Config Server using Spring Security:
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.anyRequest().authenticated()
.and()
.httpBasic();
}
}
This configuration requires HTTP Basic authentication, meaning only authenticated users can access the configurations.
3. Not Using Profiles Effectively
Spring supports multiple environments through profiles. A common pitfall is not utilizing profiles effectively, which can lead to configuration mismatches across environments.
Why This Matters: Different environments (development, staging, production) often require different configurations. Ignoring profiles can result in the propagation of erroneous settings.
Solution: Use the application-{profile}.yml
format to manage environment-specific configurations. For example:
# application-dev.yml
server:
port: 8080
# application-prod.yml
server:
port: 80
Use the following property to specify which profile to activate when starting your application:
spring.profiles.active=dev
4. Lack of Version Control
While many teams utilize Git for their application code, they often neglect to apply the same principle to configuration files.
Why This Matters: Managing configuration without version control can lead to confusion and conflicts when multiple team members make changes.
Solution: Store configurations in a Git repository. Spring Cloud Config supports versioning out of the box. By referencing a Git repository, you can roll back to previous versions of your configurations with ease.
5. Inefficient Configuration Refresh
Spring Cloud Config allows dynamic configuration refresh without the need to restart your application. However, some developers may overlook how to implement this feature.
Why This Matters: Without enabling refresh, applications will not automatically update their configurations, potentially leading to inconsistencies.
Solution: To enable dynamic refresh, use the @RefreshScope
annotation in your Spring Beans. Here’s an example:
@RefreshScope
@RestController
public class MyController {
@Value("${some.config.property}")
private String configProperty;
@GetMapping("/property")
public String getProperty() {
return configProperty;
}
}
With @RefreshScope
, when the configuration changes in the Config Server, the bean will be re-instantiated, applying the latest configuration dynamically.
6. Overcomplicating Configuration Files
While Spring Cloud Config provides a robust way to handle configurations, it’s easy to overcomplicate the configuration files.
Why This Matters: Overcomplication can lead to maintenance difficulties and confusion among developers about which properties are necessary.
Solution: Keep configuration files clean and straightforward. Avoid unnecessary properties and try to use defaults wherever possible. Focus on key configurations that define the behavior of your application.
7. Not Testing Configurations
Finally, some developers fail to adequately test their configurations when running in different environments.
Why This Matters: Failing to test configurations can lead to unexpected issues during runtime, affecting the integrity of your application.
Solution: Make it a best practice to include integration tests that validate configurations before deploying to production. You can leverage tools like JUnit and Spring’s testing framework to achieve this.
@SpringBootTest
public class ConfigTest {
@Value("${some.config.property}")
private String configProperty;
@Test
public void testConfigProperty() {
assertNotNull(configProperty);
}
}
Closing Remarks
Using Spring Cloud Config effectively requires attention to detail and consideration of best practices. By being mindful of common pitfalls, such as misconfiguring the server URL, ignoring security, and not utilizing profiles, developers can ensure a smoother experience.
For further reading on Spring Cloud Config, check out the official documentation and guides.
By avoiding these pitfalls and implementing the right strategies, you’ll be well on your way to mastering Spring Cloud Config and setting your microservices up for success. Happy coding!
Checkout our other articles