Handling Java 8 Date-Time Serialization Issues with Gson
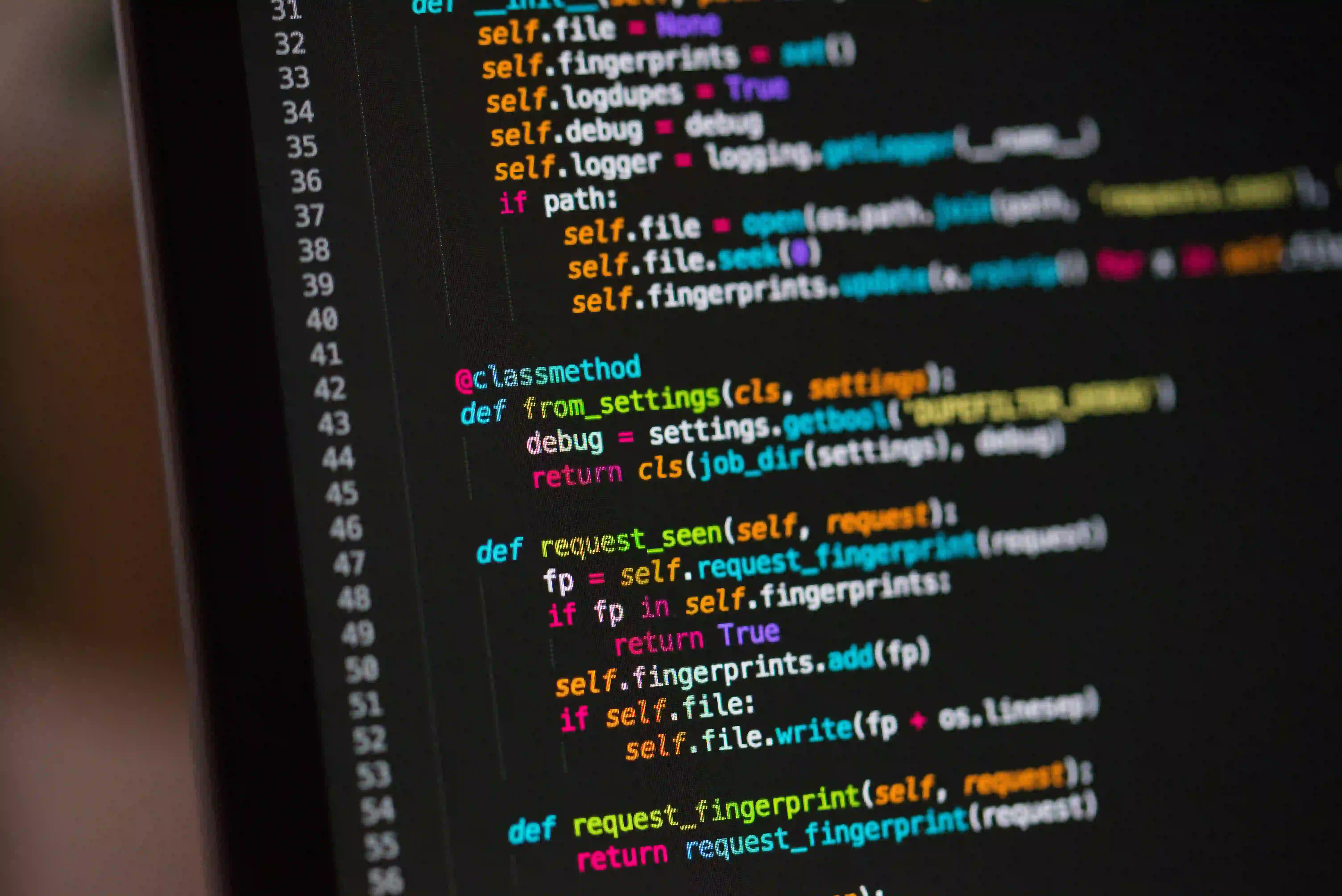
Handling Java 8 Date-Time Serialization Issues with Gson
In the ever-evolving world of software development, managing date and time data is essential. Java 8 introduced a powerful date-time API that greatly improved how developers handle date and time. However, with this improvement comes the challenge of serializing these date-time objects when using libraries such as Gson. In this blog post, we will delve into how to serialize and deserialize Java 8's date-time objects using Gson effectively and discuss some common pitfalls and solutions.
Why Use Java 8 Date-Time API?
Before diving into serialization issues, it's important to understand why you should consider using Java 8's Date-Time API. Here are three reasons:
- Clarity and Simplicity: The API is intuitive, allowing you to work with date and time types in a way that feels more natural.
- Immutability: Objects such as
LocalDate
andLocalDateTime
are immutable, which means they can’t be changed after creation. This leads to safer code, especially in concurrent applications. - Timezone Support: The Java 8 Date-Time API supports ISO-8601 standards and offers built-in classes, such as
ZonedDateTime
, to handle timezones effectively.
For more details, see Java 8 Date-Time.
Common Serialization Issues with Gson
Gson is a popular Java library for converting Java objects into their JSON representation and vice versa. However, when dealing with Java 8 date-time objects, you might run into serialization issues, as Gson does not support these types out of the box. For instance:
LocalDate today = LocalDate.now();
String json = new Gson().toJson(today);
System.out.println(json); // Output: "2023-10-08" (works)
However, if you attempt to serialize and deserialize a LocalDateTime
, the output may not be what you expect. This leads us to explore how to resolve these issues effectively.
Custom Serializers and Deserializers
One way to tackle the serialization problem is by creating a custom serializer and deserializer for each date-time type you need to handle.
Step 1: Creating a Custom Serializer
Let's take LocalDateTime
as an example:
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonSerializationContext;
import com.google.gson.JsonSerializer;
import java.lang.reflect.Type;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class LocalDateTimeSerializer implements JsonSerializer<LocalDateTime> {
private static final DateTimeFormatter formatter = DateTimeFormatter.ISO_LOCAL_DATE_TIME;
@Override
public JsonElement serialize(LocalDateTime dateTime, Type typeOfSrc, JsonSerializationContext context) {
return new JsonObject().addProperty("dateTime", dateTime.format(formatter));
}
}
Why This Works: Here, we format the LocalDateTime
to a readable string format while keeping the ISO standard. This ensures easy human reading and interoperability with other systems using the same standard.
Step 2: Creating a Custom Deserializer
Next, we create a corresponding deserializer:
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import java.lang.reflect.Type;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class LocalDateTimeDeserializer implements JsonDeserializer<LocalDateTime> {
private static final DateTimeFormatter formatter = DateTimeFormatter.ISO_LOCAL_DATE_TIME;
@Override
public LocalDateTime deserialize(JsonElement json, Type typeOfT, JsonDeserializationContext context) {
return LocalDateTime.parse(json.getAsJsonObject().get("dateTime").getAsString(), formatter);
}
}
Why This Works: We parse the formatted string back to a LocalDateTime
object, ensuring our deserialization process is as seamless as serialization.
Step 3: Registering Custom Serializers/Deserializers
Now that we have our custom serializer and deserializer, we need to register them into our Gson instance:
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
public class GsonConfig {
public static Gson getGson() {
return new GsonBuilder()
.registerTypeAdapter(LocalDateTime.class, new LocalDateTimeSerializer())
.registerTypeAdapter(LocalDateTime.class, new LocalDateTimeDeserializer())
.create();
}
}
Why This Works: We create a singleton Gson instance equipped with our custom serialization logic, making it easy to reuse throughout our application.
Example Usage
Now let’s put this all together in an example:
import java.time.LocalDateTime;
public class Example {
public static void main(String[] args) {
Gson gson = GsonConfig.getGson();
// Sample LocalDateTime
LocalDateTime now = LocalDateTime.now();
String json = gson.toJson(now);
System.out.println("Serialized JSON: " + json);
LocalDateTime parsedDateTime = gson.fromJson(json, LocalDateTime.class);
System.out.println("Deserialized LocalDateTime: " + parsedDateTime);
}
}
When you run this, you'll see that the LocalDateTime
is serialized and deserialized correctly.
Additional Considerations
- Null Handling: If you anticipate null values, ensure your serializer and deserializer can handle them gracefully.
- Other Date-Time Types: Similar custom approaches can be applied for
LocalDate
,ZonedDateTime
, etc. Just adjust the formatter accordingly. - Error Handling: Invest in proper error handling in deserialization so that any malformatted JSON does not lead to application crashes.
In Conclusion, Here is What Matters
Handling Java 8 Date-Time serialization issues with Gson is crucial for modern applications. By establishing custom serializers and deserializers, you can leverage the full power of Java 8's date-time API while ensuring data integrity during JSON serialization and deserialization.
With these techniques, your application can handle date-time data more effectively, providing better clarity and standards compliance. Stay updated with the latest in Java and Gson to continue improving your development practices.
For further reading, you might find the following links helpful:
- Gson User Guide - A comprehensive guide on using Gson.
- Java 8 Date and Time - Official Java documentation on the new Date and Time API.
Feel free to explore and implement these practices to improve your application's handling of date and time data! Happy coding!