Overcoming Common Pitfalls in Test-Driven Development
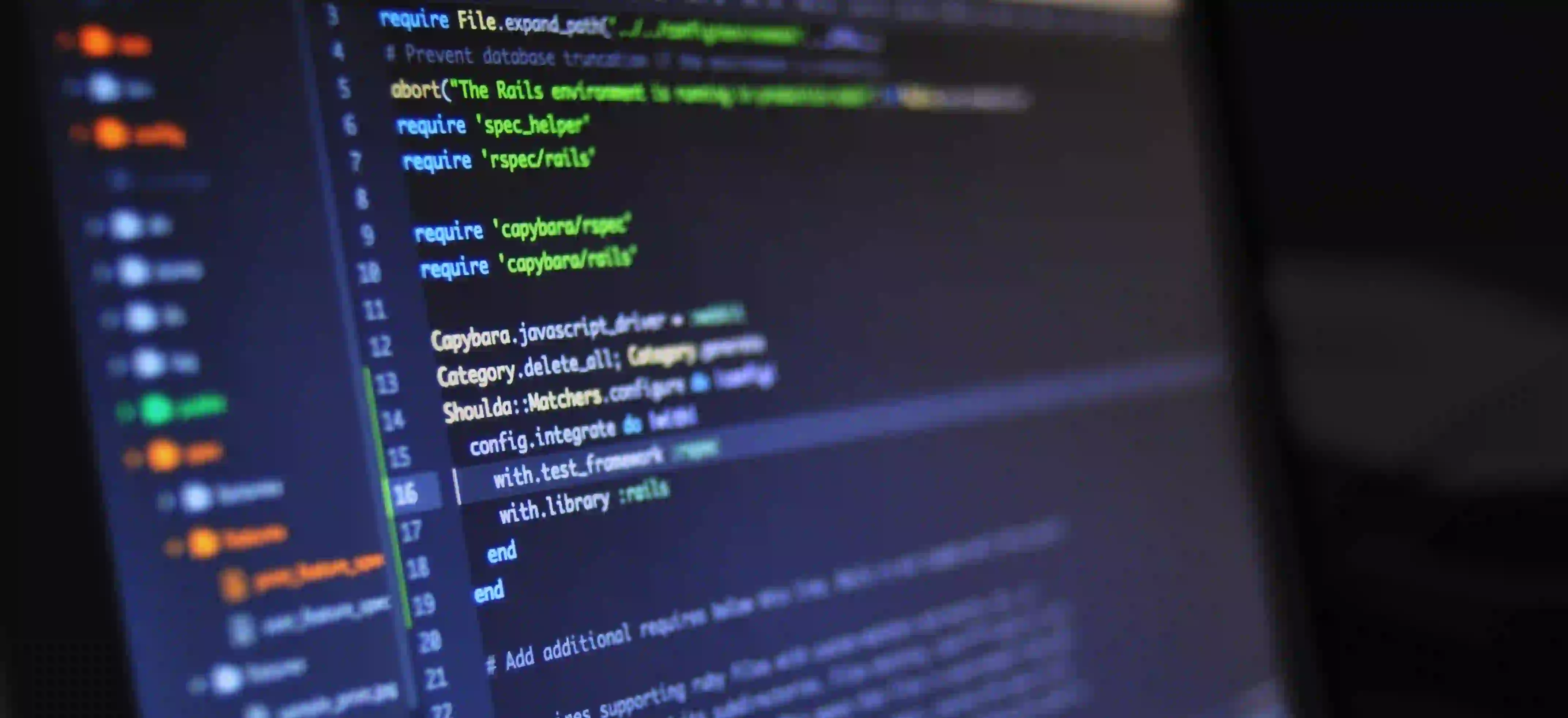
Overcoming Common Pitfalls in Test-Driven Development
Test-Driven Development (TDD) is a software development process that relies heavily on the repetition of a very short development cycle. First, the developer writes a test that defines a function or improvements of a function, then produces code to pass that test, and finally refactors the code to acceptable standards. While TDD provides numerous benefits, it also has its challenges. In this article, we’ll explore the common pitfalls in TDD and how to overcome them effectively.
What is Test-Driven Development (TDD)?
TDD is based on a simple concept: Red-Green-Refactor. This means writing a failing test (Red), implementing the minimal code necessary to pass the test (Green), and then cleaning up the code (Refactor). This approach helps ensure that automation is in place at every stage and encourages writing better, more reliable code.
// Example of writing a simple test in JUnit
import org.junit.Test;
import static org.junit.Assert.*;
public class MathUtilsTest {
@Test
public void testAdd() {
MathUtils mathUtils = new MathUtils();
assertEquals(5, mathUtils.add(2, 3)); // This test should pass
}
}
Why TDD?
TDD boosts productivity by creating a safety net of tests around your code, ensuring that changes won't unintentionally break existing functionalities. It leads to better design, improved code quality, and increased developer confidence.
Common Pitfalls in TDD
Regardless of the advantages, several pitfalls can undermine the effectiveness of TDD. Let's look at some of the most common issues developers face and how to overcome them:
1. Inadequate Understanding of Requirements
One of the most significant pitfalls in TDD is rushing to write tests without fully understanding the requirements. This can lead to tests that are misaligned with what is being developed.
Solution: Take the time to engage with stakeholders to fully comprehend the requirements. Use a collaborative approach that involves discussions and maybe user stories to clarify details.
2. Writing Tests Based on Implementation
Developers often make the mistake of writing tests that become too intertwined with the implementation details. If the implementation changes, the tests might break even if the functionality still holds.
Solution: Focus on testing the behavior of the system rather than its implementation. Techniques like Behavior-Driven Development (BDD) can help in emphasizing system behavior.
// Example of behavior-focused testing
@Test
public void testUserRegistration() {
UserRegistrationService service = new UserRegistrationService();
boolean isRegistered = service.registerUser("john.doe@example.com");
assertTrue(isRegistered);
}
3. Skipping Refactoring
After passing the tests, some developers skip the refactoring step, thinking the job is done. This not only leads to poor code quality but may also cause code bloat over time.
Solution: Always prioritize clean code through periodic refactoring. The second "R" in the TDD process is essential. Ensure your code is readable and maintainable.
4. Overemphasis on Unit Tests
While unit tests are crucial, developers sometimes lose sight of the big picture and neglect integration and end-to-end tests. This can lead to issues when components interact with each other.
Solution: Adopt a balanced testing strategy that emphasizes various test types. Use unit tests for individual components, integration tests for how parts work together, and end-to-end tests to validate workflows.
5. Not Running Tests Frequently
It is tempting to delay running tests until too many changes have accumulated. However, this is counterproductive.
Solution: Run your tests frequently—ideally, after each development cycle. This helps to catch defects early, keeps the code clean, and saves time in the long run.
6. Failing to Write Tests for Edge Cases
Developers might favor happy paths while ignoring edge cases. While these situations might not be common, they can lead to critical failures when overlooked.
Solution: Make it a practice to write tests not only for intended behavior but also for potential edge cases.
@Test
public void testAddNegativeNumbers() {
MathUtils mathUtils = new MathUtils();
assertEquals(-1, mathUtils.add(-2, 1)); // Testing an edge case
}
7. Not Understanding the Purpose of TDD
Many developers misunderstand the TDD philosophy and end up writing tests that slow them down rather than improving their workflow.
Solution: Invest time in understanding TDD principles. Embrace the idea that tests are an integral part of software design and not just an afterthought.
8. Relying on Mocking Overuse
While mocking can simplify unit tests by isolating components, excessive mocking can create tests that don’t genuinely reflect how the system will behave in production.
Solution: Use mocking judiciously. Aim for integration tests that validate interactions between components while keeping mocks reserved for isolated unit tests.
9. Resistance to Change
Development teams can develop a certain inertia over time, becoming resistant to adopting TDD practices or adapting to changes in TDD methodologies.
Solution: Cultivate a growth mindset within development teams. Encourage continuous learning through workshops, team discussions, and sharing of best practices to foster a collaborative atmosphere.
Best Practices for Effective TDD
To successfully implement TDD and mitigate common pitfalls, consider these best practices:
- Write Clear and Concise Tests: Ensure your tests are understandable and cover the intended functionality.
- Focus on Minimalism: Write just enough code to pass the tests—no more, no less.
- Refactor Ruthlessly: Regularly clean up code to maintain clarity and performance.
- Engage in Pair Programming: Collaborating with another developer can enhance understanding and uncover potential pitfalls.
- Continuous Integration: Integrate TDD into your CI/CD pipeline to ensure your tests run with every build. This maintains consistent quality.
My Closing Thoughts on the Matter
Test-Driven Development can be a game-changer but presents its own challenges. By recognizing and addressing these common pitfalls, developers can greatly improve their productivity and code quality. It’s all about the right mindset and practices. Embrace TDD, focus on creating clear, maintainable code, and watch your product evolve into a robust solution.
For further insights into testing strategies and software development best practices, you might find these resources helpful:
By adopting a proactive approach and adhering to TDD principles, developers can not only avoid pitfalls but also excel in delivering high-quality software. Happy testing!