Overcoming Latency Issues in Model Serving for Streaming Data
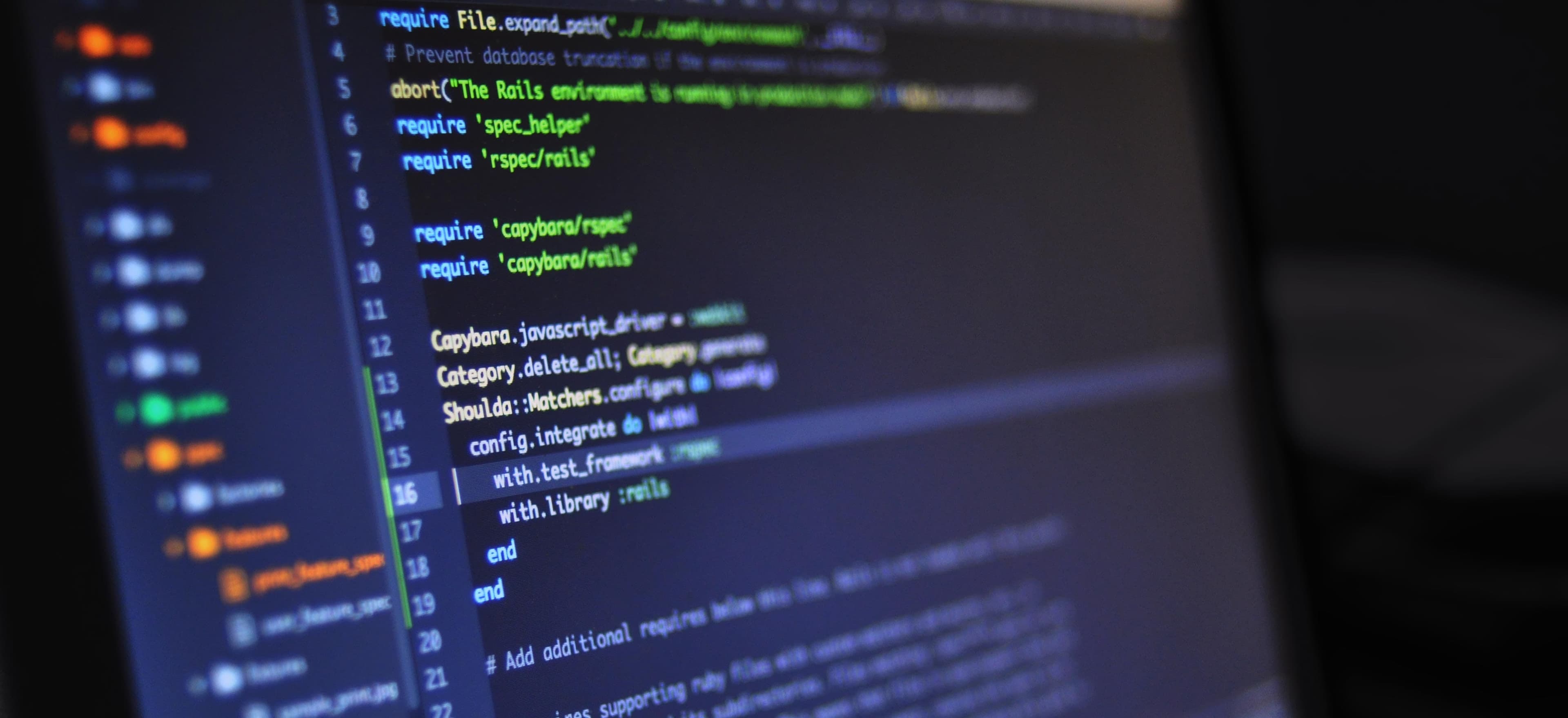
- Published on
Overcoming Latency Issues in Model Serving for Streaming Data
In today’s data-driven world, streaming data applications are becoming increasingly prevalent. From real-time analytics and fraud detection to recommendation systems, businesses are leveraging live data to drive decisions instantaneously. However, these applications often grapple with latency issues, especially when serving machine learning models. In this blog post, we will explore strategies for overcoming latency in model serving for streaming data, covering various techniques and best practices.
Understanding Latency in Model Serving
What is Latency?
Latency refers to the time taken from the moment a request is initiated to the time the response is received. In the context of model serving, latency is crucial because it directly impacts user experience and, ultimately, business outcomes. For instance, a recommendation system with high latency may frustrate users and result in lost opportunities.
Factors Contributing to Latency
- Model Complexity: Larger models with more parameters generally require more processing time.
- Data Transfer Time: Streaming data may involve delays in data transmission.
- Resource Contention: High demand for computational resources can lead to bottlenecks.
- Serialization and Deserialization: Conversion of model inputs and outputs can introduce additional overhead.
Measuring Latency
To effectively overcome latency issues, it is essential to measure them accurately. Tools such as Prometheus for monitoring and Grafana for visualization can provide insights into latency metrics. This data is integral for identifying bottlenecks and addressing them appropriately.
Strategies to Overcome Latency Issues
1. Model Optimization
Lightweight Models: If your model is too complex, consider simplifying it. Techniques such as pruning, quantization, or distillation can reduce the model's size without significantly harming its performance.
Example of Model Pruning:
import torch
import torch.nn.utils.prune as prune
# Load a pre-trained model
model = ...
# Applying pruning on the first layer
prune.random_unstructured(model.layer1, name='weight', amount=0.3)
# Checking number of non-zero parameters
count_parameters(model)
Why: Pruning removes less critical weights from the model, reducing the overall computation required during inference.
2. Asynchronous Processing
Queue-Based Systems: Using queue engines like Apache Kafka or RabbitMQ can decouple model execution from incoming data. By buffering incoming requests, you allow your model to process them at its own pace, reducing the waiting time for users.
3. Caching Results
Result Caching: If your model serves repetitive requests, consider caching results to minimize repeated computation. Tools like Redis can be invaluable here.
Example of Caching:
import redis
# Set up a Redis client
r = redis.StrictRedis(host='localhost', port=6379, db=0)
def get_prediction(data):
cache_key = f"prediction:{data}"
# Check if the result is cached
cached_result = r.get(cache_key)
if cached_result:
return cached_result.decode('utf-8')
# Otherwise, compute prediction
result = model.predict(data)
r.set(cache_key, result)
return result
Why: By caching results, you prevent the model from recalculating responses for identical inputs, speeding up response times.
4. Load Balancing
Horizontal Scaling: Implement load balancing by deploying multiple instances of your model. Tools such as Kubernetes can assist in managing load distribution efficiently.
Setting Up a Load Balancer:
apiVersion: apps/v1
kind: Deployment
metadata:
name: model-serving
spec:
replicas: 3
selector:
matchLabels:
app: model-serving
template:
metadata:
labels:
app: model-serving
spec:
containers:
- name: model-serving
image: my-model-image
Why: Load balancing effectively distributes incoming requests across several instances of your model, reducing response times.
5. Streamlined Data Processing
Preprocessing: Before passing data to your model, ensure it’s appropriately preprocessed and formatted. Use separate threads or services for this task to minimize the burden on model-serving infrastructure.
Parallel Processing Example:
from concurrent.futures import ThreadPoolExecutor
def preprocess_data(data):
# Data preprocessing steps
return processed_data
data_stream = [...] # Streaming data
with ThreadPoolExecutor(max_workers=4) as executor:
processed_stream = list(executor.map(preprocess_data, data_stream))
Why: Using multithreading for preprocessing allows you to handle batch processing simultaneously, leading to reduced latency.
6. Edge Computing
Localized Processing: If your streaming application involves IoT devices, consider employing edge computing. By processing data closer to its source, latency can be significantly minimized.
Example of Edge Deployment: You can use platforms like AWS Greengrass for deploying machine learning models to edge devices, thereby facilitating real-time decision-making directly where data is collected.
7. Continuous Monitoring and Feedback Loop
Observability: Implement an observability framework to monitor latency continuously. Tools like ELK Stack (Elasticsearch, Logstash, Kibana) can be used to visualize logs and monitor performance.
Why: Continuous monitoring allows you to identify and troubleshoot latency issues in real-time, ensuring your model continues to meet user expectations.
In Conclusion, Here is What Matters
Addressing latency in model serving for streaming data is a multifaceted challenge, but it's critical for delivering a seamless user experience. By employing strategies such as model optimization, asynchronous processing, result caching, load balancing, streamlined data processing, edge computing, and continuous monitoring, you can effectively mitigate latency and enhance the responsiveness of your applications.
As the world continues to lean into data streaming and real-time analytics, ensuring low-latency model serving becomes pivotal. By understanding the fundamentals and leveraging the right techniques, we can unlock the full potential of machine learning in a real-time environment.
For further reading, check out these resources:
- Machine Learning Model Deployment
- Real-time Stream Processing with Kafka
Let us embrace these techniques and evolve our data-serving capabilities to meet the demands of our fast-paced digital landscape.
Checkout our other articles