Understanding the Limitations of Sealed Classes in Java
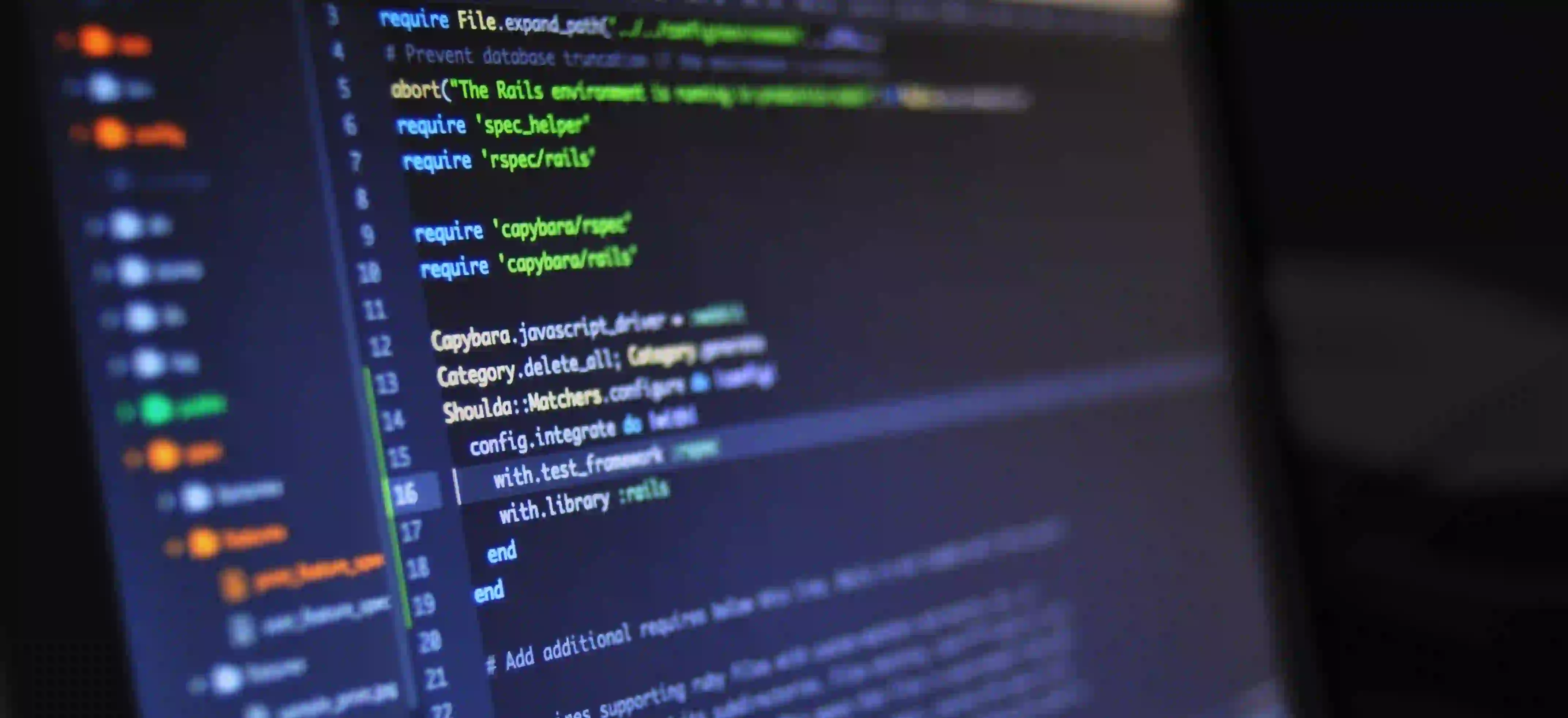
Understanding the Limitations of Sealed Classes in Java
Java's evolution over the years has brought many powerful features, including sealed classes with the introduction of Java 17. These classes enable developers to define a restricted hierarchy of subclasses, enhancing maintainability and security in codebases. However, like every feature, sealed classes come with their limitations. In this post, we'll explore what sealed classes are, their benefits, and ultimately, their limitations.
What Are Sealed Classes?
Sealed classes restrict which other classes can extend them. By declaring a class as sealed, you effectively control its inheritance, allowing only specific subclasses to extend it. This feature introduces a level of rigor to your object-oriented design, making it clear which classes are intended for inheritance.
Example of Sealed Classes
Let's consider how you declare a sealed class in Java.
public sealed class Shape permits Circle, Rectangle {
// Common attributes and methods for all shapes
public abstract double area();
}
public final class Circle extends Shape {
private final double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double area() {
return Math.PI * radius * radius;
}
}
public final class Rectangle extends Shape {
private final double length;
private final double width;
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
@Override
public double area() {
return length * width;
}
}
In the above example, Shape
is a sealed class that permits only Circle
and Rectangle
as its subclasses. This approach promotes a closed hierarchy, enhancing type safety and clarity.
Benefits of Sealed Classes
1. Enhanced Security
By restricting which classes can extend a sealed class, you reduce the risk of unexpected behavior in your application. This aspect allows greater control, ensuring that only tested and verified subclasses can modify behavior.
2. Improved Maintainability
When your class hierarchy is well-defined, it is easier to read, maintain, and evolve. Developers can quickly understand the family of subclasses associated with a sealed class without wading through a vast number of unrelated classes.
3. Clear Design Intent
Sealed classes express intent clearer. By marking a class sealed, a developer communicates that the class is not meant for free extension, aiding other developers in the understanding of the constraints around class usage.
Limitations of Sealed Classes
Despite their advantages, sealed classes come with several limitations, which we will explore next.
1. Limited Flexibility in Extension
The most apparent limitation is that once you define a sealed class, creating additional subclass types outside of the permitted ones is impossible. This limitation can cause issues in scenarios where your application evolves, and new subclasses are required.
Example
If later you need to add a Triangle
class to the hierarchy, you will face a compile-time error.
// This will cause a compile-time error
public final class Triangle extends Shape {
private final double base;
private final double height;
public Triangle(double base, double height) {
this.base = base;
this.height = height;
}
@Override
public double area() {
return 0.5 * base * height;
}
}
2. Only Inheritance Allowed at the Same Level
Sealed classes do not allow multiple levels of inheritance from subclasses. If you need a subclass of a subclass, you must explicitly permit it in the sealed class declaration. This constraint can lead to more complex code structures.
Example
Below is a modification to permit further subclassing.
public sealed class Shape permits Circle, Rectangle, Triangle {
// Shape code
}
public non-sealed class Triangle extends Shape {
// Triangle code
}
The Triangle
class is now permitted to be further subclassed, but this can reduce design clarity and intent.
3. Constructor Visibility
Sealed classes cannot have a public constructor if you want to prevent instantiation from outside classes. This means that the base class must have a restricted constructor (protected or package-private), which can lead to challenges in instantiating objects if you inadvertently need an instance of the base class itself.
Example
public sealed class Shape permits Circle, Rectangle {
protected Shape() {
// Prevents outside classes from instantiating Shape
}
// Other methods
}
4. Increased Complexity in Code Management
The start of defining sealed classes can be straightforward; however, as your codebase grows and changes, you may find that managing this structure becomes increasingly complicated. Designing early can save time later, but it requires forethought and experience.
5. Limited Tools and Frameworks Support
Though Java 17 and newer versions provided support for sealed classes, not all tools or frameworks might fully capitalize on this feature. You may face challenges in compatibility with some libraries or frameworks not yet supporting or utilizing sealed classes properly.
Wrapping Up
Understanding sealed classes in Java is essential, especially in modern application design. They offer unique benefits, especially regarding code clarity, maintainability, and security. However, their inherent limitations can pose significant challenges in rapidly evolving projects.
Being aware of these limitations enables developers to make informed architectural decisions. As a best practice, start simple by gradually incorporating sealed classes where they make sense.
For further reading and a deep dive into Java features, check out the following resources:
- Java's Sealed Classes - A Feature Overview
- Best Practices for Using Sealed Classes
Remember, choosing the right tools and techniques is all about balancing constraints and needs. Happy coding!
Feel free to adjust the complexity or focus of this blog post as needed, based on your intended audience or specific areas of interest regarding sealed classes in Java.