Overcoming Spark and Parquet Integration Challenges on S3
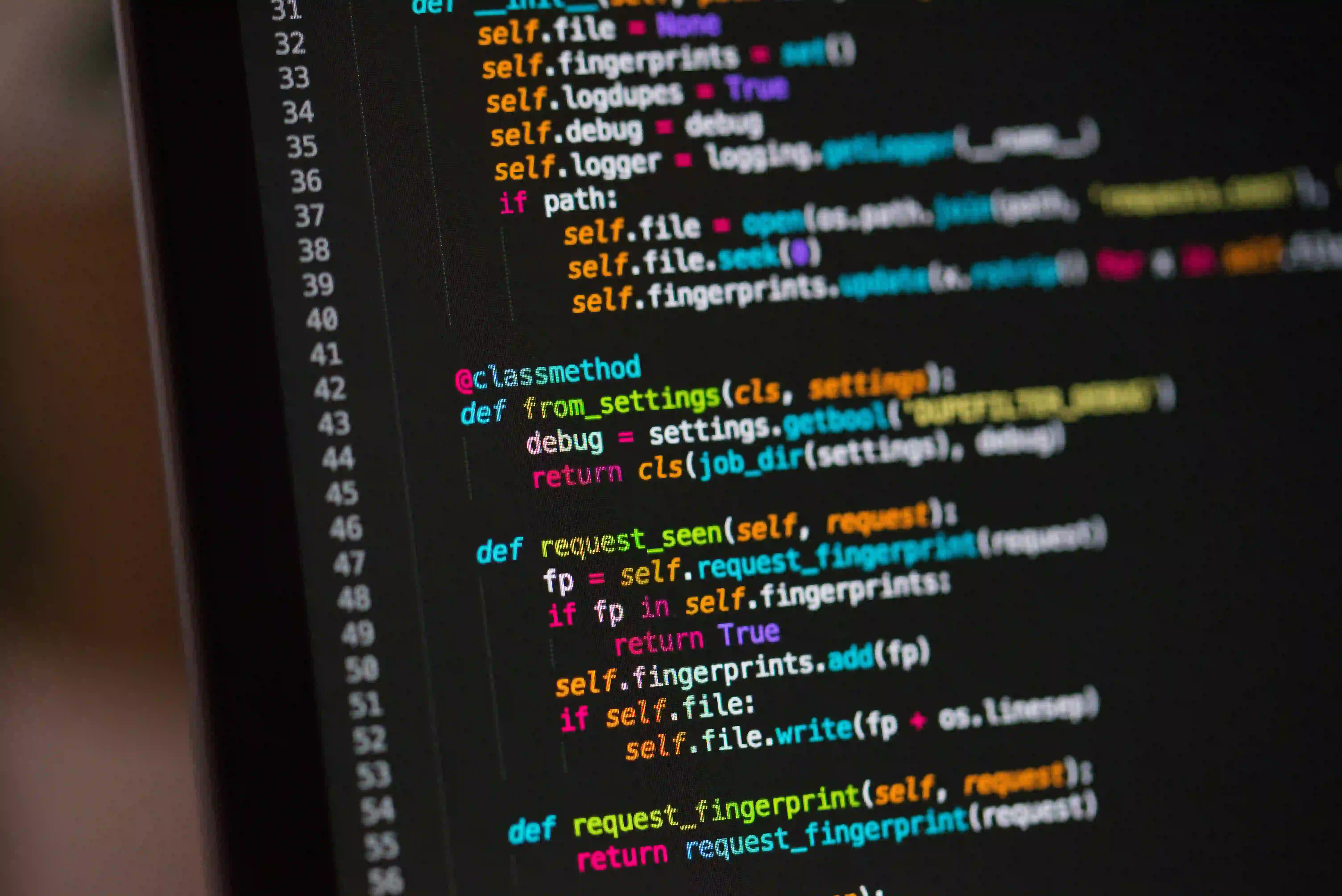
Overcoming Spark and Parquet Integration Challenges on S3
Apache Spark is a widely used distributed computing framework designed to handle big data processing with ease and efficiency. Coupled with the Parquet file format, which is optimized for efficient storage and query performance, it creates a powerful combination for data analytics. However, integrating Spark with Parquet files on Amazon S3 can present some challenges.
Why Use Spark and Parquet Together?
Before diving into the challenges, letβs understand why combining these technologies is beneficial:
-
Performance: Parquet is a columnar storage format that reduces the amount of data read during queries, further enhancing Spark's performance. This is especially critical when operating on large datasets.
-
Schema Evolution: Parquet files support schema evolution, allowing you to change the schema of your dataset over time.
-
Cost-Effectiveness: Amazon S3 is a cost-effective storage solution, particularly for scalable data solutions. You only pay for what you use, making it attractive for big data operations.
Common Integration Challenges
Despite the advantages, there are several challenges encountered when integrating Spark with Parquet on S3. Here are some of the common issues and how to overcome them.
1. Data Consistency
Challenge: Data consistency can become an issue, especially when multiple Spark jobs are reading/writing to the same Parquet file on S3 concurrently.
Solution
Utilize partitioning in your Parquet files. Partitioning allows you to subdivide your dataset into smaller, manageable segments, significantly reducing the risk of conflicting read/write operations.
For example, if you have a log dataset, consider partitioning it by date or user ID:
val logsDF = spark.read.parquet("s3://your-bucket/path/to/logs/")
logsDF.write.partitionBy("date").parquet("s3://your-bucket/output-directory/")
Here, we partition the data by date. This allows concurrent reads and writes while maintaining data integrity.
2. Configuring AWS Credentials
Challenge: Connecting to S3 requires valid AWS credentials, and misconfigurations can lead to access issues.
Solution
Configure your Spark session to include the necessary AWS access keys. Using IAM roles is a best practice for secure access.
Here's how to set up your Spark session with AWS credentials using environment variables:
import org.apache.spark.sql.SparkSession
val spark = SparkSession.builder()
.appName("SparkParquetIntegration")
.config("spark.hadoop.fs.s3a.access.key", sys.env("AWS_ACCESS_KEY_ID"))
.config("spark.hadoop.fs.s3a.secret.key", sys.env("AWS_SECRET_ACCESS_KEY"))
.getOrCreate()
This approach employs environment variables to access AWS credentials securely.
3. Performance Tuning
Challenge: You may encounter performance issues, such as slow reads/writes or excessive memory use.
Solution
Adjust the following configurations for optimal performance scaling:
-
Batch Size: Use the
spark.sql.parquet.block.size
to adjust the block size of Parquet files. -
Data Repartitioning: Repartition your DataFrame based on the size and distribution of your data.
Example:
val updatedDF = logsDF.repartition(4) // Repartition to 4 partitions
updatedDF.write.parquet("s3://your-bucket/output-directory/")
By controlling the number of partitions, you can ensure balanced workloads across your cluster.
4. Handling Schema Mismatch
Challenge: Schema mismatch issues often arise during data reads, leading to errors.
Solution
Leverage the mergeSchema
option when reading Parquet files. This tells Spark to merge the schemas of different Parquet files and use the most updated schema.
val logsDF = spark.read.option("mergeSchema", "true").parquet("s3://your-bucket/path/to/logs/")
This ensures that all Parquet files are read with the correct schema, reducing the likelihood of errors due to mismatched structures.
5. Data Cleanup and Management
Challenge: Storing large amounts of raw data can lead to s3 bucket clutter, which becomes difficult to manage over time.
Solution
Implement a regular data cleanup strategy. Use Spark jobs to manage older or unnecessary data efficiently, ensuring your S3 bucket remains organized.
import java.time.LocalDate
import java.time.format.DateTimeFormatter
val date = LocalDate.now().minusDays(30).format(DateTimeFormatter.ofPattern("yyyy-MM-dd"))
// Assume you need to delete logs older than 30 days
val oldLogsDF = spark.read.parquet("s3://your-bucket/path/to/logs/")
oldLogsDF.filter(s"date < '$date'").write.mode("overwrite").parquet("s3://your-bucket/cleanup-directory/")
This code snippet writes filtered logs to a separate directory, making it easier to manage and delete as necessary.
Best Practices
To maximize the efficiency of Spark and Parquet integration on S3, consider the following best practices:
-
Use Versioned Buckets: Enable versioning on your S3 bucket to keep track of changes over time.
-
Monitor Performance: Regularly monitor job performance and tweak Spark configurations based on observed metrics.
-
Optimize Storage Size: Parquet files' size should be balanced - ideally between 128MB and 1GB - for effective processing.
-
Leverage Databricks or EMR Integration: If feasible, consider using managed Spark services like AWS EMR or Databricks for seamless integration.
-
AWS Glue Catalog: Utilize the AWS Glue Data Catalog for managing data schemas and metadata, which provides better data governance.
The Last Word
While integrating Spark with Parquet files on Amazon S3 may present several challenges, being proactive about data management, performance tuning, and configuration can alleviate many issues. Following the recommendations outlined above will pave the way for a smoother, more efficient data processing workflow.
For more insights on best practices with Spark and Parquet, refer to the Apache Spark Documentation or check out the AWS S3 documentation.
By strategically managing these integration challenges, you can enhance your big data processing capabilities on Amazon S3, making the most out of your Spark and Parquet experience. Happy coding!