Understanding HATEOAS: Common Pitfalls to Avoid in REST APIs
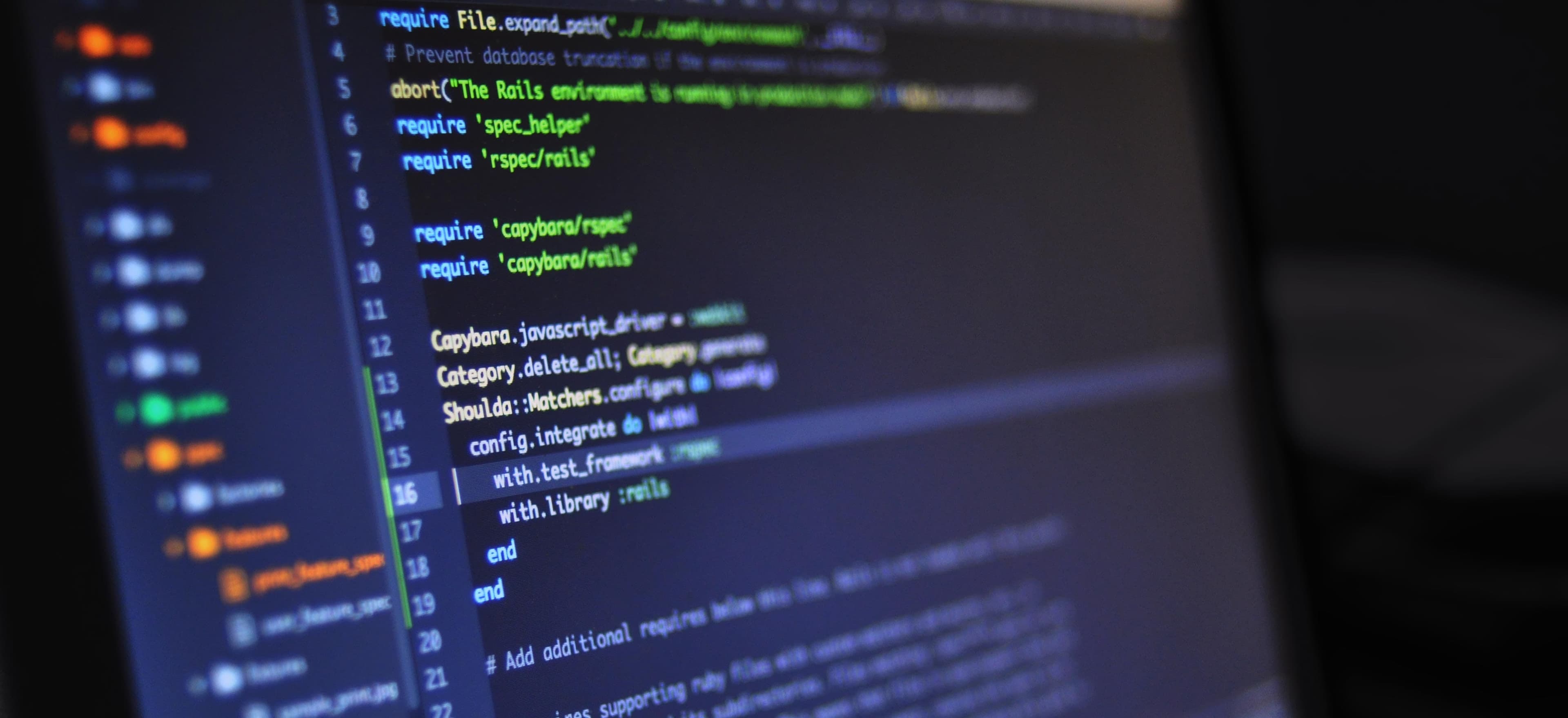
- Published on
Understanding HATEOAS: Common Pitfalls to Avoid in REST APIs
When building RESTful APIs, one crucial aspect that often gets overlooked is HATEOAS, or Hypermedia as the Engine of Application State. HATEOAS is a constraint of the REST application architecture that allows clients to dynamically navigate the application state through hypermedia links provided in the API responses.
In this blog post, we will explore the concept of HATEOAS, why it is essential for building robust APIs, and some common pitfalls to avoid when implementing it. This understanding will help you create more flexible and navigable APIs that align with RESTful principles.
What is HATEOAS?
HATEOAS is part of the larger REST architectural style, which you can learn more about here. The term itself combines hypermedia—textual representations of state transitions in an application—and the concept of states that your application can be in.
In practical terms, HATEOAS means that the server provides information in such a way that the client can discover available actions dynamically. This discovery is achieved through hyperlinks in the API responses.
Example of HATEOAS in Action
Consider the following JSON response for a user resource:
{
"id": 1,
"name": "John Doe",
"email": "john@example.com",
"_links": {
"self": {
"href": "/users/1"
},
"friends": {
"href": "/users/1/friends"
},
"posts": {
"href": "/users/1/posts"
}
}
}
In this example, the _links property provides URLs to resources related to the user. It allows the client to understand that it can fetch the user's friends and posts without needing to know the URLs ahead of time.
Why Use HATEOAS?
-
Decoupling Client and Server: One of the main benefits of HATEOAS is that it decouples the client and server, enabling you to change the API endpoints without affecting the client as long as the links in the responses are maintained.
-
Discoverability: HATEOAS promotes discoverability, allowing clients to navigate the API easily. Instead of hardcoding URLs, clients react to links provided in server responses.
-
Simplified Client Code: As clients can navigate based on hypermedia links, the client-side code becomes simpler. It can consume APIs without prior knowledge of the entire structure.
Common Pitfalls When Implementing HATEOAS
Understanding how to implement HATEOAS is crucial for avoiding certain pitfalls. Below are some common mistakes developers often make:
1. Lack of Hypermedia in Initial Responses
A common mistake is not including hypermedia links in the initial API responses. The feedback from clients should always include links that allow further actions.
Solution: Ensure that every response includes relevant _links. For example:
{
"data": {...},
"_links": {
"self": {
"href": "/data"
},
"next": {
"href": "/data?page=2"
}
}
}
2. Overloading the API with Too Many Links
Conversely, including too many links can overwhelm the client. It can become daunting to navigate through a vast number of hypermedia links.
Solution: Include only the most relevant links. For instance, you might consider separating links based on context and requesting only those that the client needs for the current operation.
3. Ignoring the Client's Perspective
API designers sometimes fail to consider how clients will interact with their APIs. Not understanding the client’s needs can lead to poor link usage.
Solution: Conduct consultations with stakeholders or potential users of the API to design the hypermedia structure tailored to their requirements.
4. Failing to Maintain Consistency
Inconsistent use of links can lead to confusion and errors. If users see varying formats or link structures for similar resources, they may struggle to comprehend how your API works.
Solution: Establish a clear standard for how to represent and construct links in your API behavior. Following JSON API specifications can help, as outlined here.
5. Not Handling Versioning Properly
As your API evolves, you may face the challenge of versioning. Simply changing the URL structure without consideration can break existing clients.
Solution: Use versioning in your links and keep your clients informed about the changes. Make sure to provide links to resources both in the old and new API versions.
{
"_links": {
"self": {
"href": "/v1/users/1"
},
"version": {
"href": "/v2/users/1"
}
}
}
6. Forgetting About Error Handling
Error scenarios can sometimes be overlooked. If a link points to a resource that does not exist or has an error, the client should receive consistent and meaningful error messages.
Solution: Include links to error responses, guiding users to next steps or details about the error. Provide useful error codes and documentation links.
{
"error": {
"message": "User not found",
"_links": {
"help": {
"href": "/docs/errors#user-not-found"
}
}
}
}
My Closing Thoughts on the Matter
HATEOAS is a powerful concept that, when implemented correctly, can greatly enhance the usability and maintainability of REST APIs. By focusing on discoverability, client-server decoupling, and clear navigation paths through hypermedia, you will create APIs that are scalable and intuitive.
Avoiding the common pitfalls outlined above will not only ensure a robust implementation of HATEOAS but also facilitate smoother collaboration between clients and servers. As you design your API, remember that flexibility and ease of use are key—always keep your users in mind.
For further reading, check out the RESTful APIs Cookbook for practical tips and implementations.
Checkout our other articles