Common Pitfalls When Publishing Your Android Library
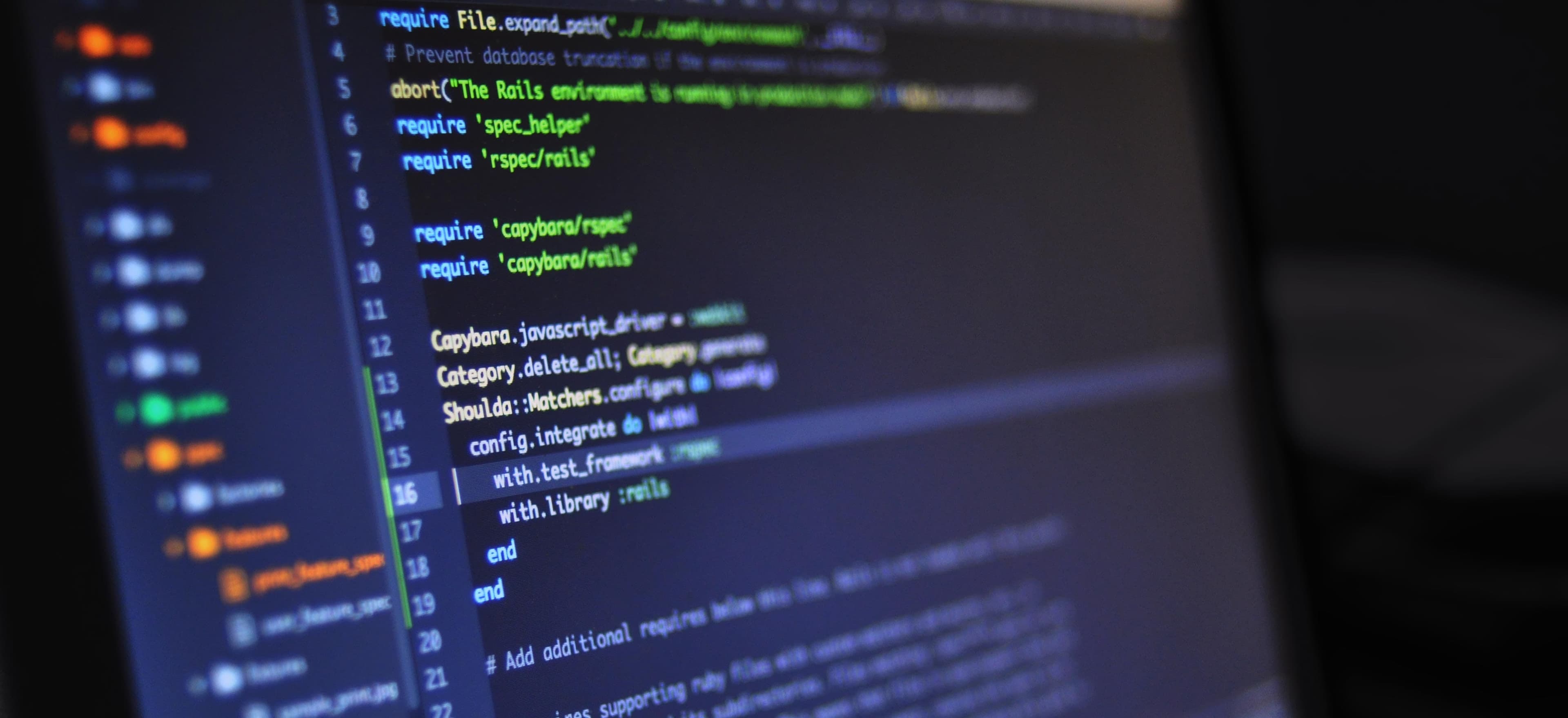
- Published on
Common Pitfalls When Publishing Your Android Library
Creating and publishing an Android library can be a rewarding experience. Whether you're building a reusable component or addressing a specific problem, libraries can significantly enhance Android development efficiency. However, there are common pitfalls that developers encounter when publishing their libraries. In this blog post, we will discuss these pitfalls, offer strategies to overcome them, and provide best practices for successful publication.
Table of Contents
Understanding Android Libraries
An Android library is a collection of reusable code that developers can integrate into their apps. Libraries facilitate code reuse and modularity, making projects more maintainable. Common types include:
- Android Library: Contains Android resources (layouts, string resources, etc.).
- Java Library: Useful for pure Java functionality.
Publishing a library means making it available to the larger developer community, often through repositories like JitPack, MavenCentral, or Google's Maven Repository.
Common Pitfalls
1. Lack of Documentation
One of the biggest pitfalls is neglecting robust documentation. A library that works perfectly but lacks proper guidance may frustrate potential users.
-
Why It’s Important: Documentation serves as a road map for users, guiding them on how to implement and utilize the library effectively.
-
Best Practices:
- Provide clear and concise README files.
- Include code snippets and examples for better comprehension.
# Example of Basic Usage
```kotlin
val myLibrary = MyLibrary()
myLibrary.performAction()
In this example, the MyLibrary is instantiated and a method performAction is called. Clear examples help developers quickly understand how to use your library.
### 2. Poor Versioning Strategy
Versioning your library might seem trivial, but it plays a crucial role in maintaining compatibility and managing upgrades.
- **Why It’s Important:** A poor versioning strategy can lead to broken builds, version conflicts, and user confusion.
- **Best Practices:**
- Use [Semantic Versioning](https://semver.org/) (MAJOR.MINOR.PATCH).
- Clearly document changes in your library's CHANGELOG.
### 3. Ignoring Dependency Management
Developers often overlook the implications of adding dependencies. Considerations about scope, versioning, and conflicts can complicate library integration.
- **Why It’s Important:** Incompatible dependencies can prevent your library from working within users' projects.
- **Best Practices:**
- Use well-defined dependency scopes.
- Avoid transitive dependencies when possible.
```groovy
dependencies {
implementation 'com.squareup.retrofit2:retrofit:2.9.0'
// Use implementation here to ensure others can't pull in transitive dependencies
}
4. Not Following Gradle Standards
Gradle is the backbone of Android build processes. Neglecting Gradle standards can lead to integration issues.
-
Why It’s Important: Adhering to these standards ensures broader compatibility and ease of use.
-
Best Practices:
- Use Gradle Plugin DSL rather than application DSL where applicable.
- Keep your build scripts tidy and organized.
apply plugin: 'com.android.library'
// Android-specific configurations
android {
compileSdkVersion 30
...
}
This structure ensures developers can easily navigate and customize their builds, fostering a better user experience.
5. Failing to Test on Multiple Devices
Testing on a single device may lead to unforeseen problems when users integrate your library into different environments.
-
Why It’s Important: Android runs on a vast range of devices with different configurations, perspectives, and capabilities.
-
Best Practices:
- Utilize Firebase Test Lab or similar services to test on a variety of devices.
- Collect feedback from beta testers who use different hardware.
Strategies for Success
To navigate these pitfalls successfully, consider the following strategies:
-
Robust Documentation: Always prioritize documentation. Invest time in writing tutorials, examples, and comprehensive API references.
-
Version Control: Implement a strong versioning system. Prepare for breaking changes and inform users in advance if a major upgrade is coming.
-
Dependency Awareness: Be cautious with dependencies. Evaluate them carefully and understand the risks before incorporating them into your library.
-
Gradle Compliance: Familiarize yourself with the latest Gradle best practices. Use official documentation as a reference.
-
Extensive Testing: Don’t skip on testing. Utilize emulators, physical devices, and community user feedback to ensure your library works flawlessly across the board.
The Last Word
Publishing an Android library can be a fulfilling endeavor, but be aware of the common pitfalls that can inhibit its success. By avoiding issues such as inadequate documentation, poor versioning, and neglecting testing, you can create a library that not only meets your needs but also serves the broader community effectively.
Moreover, actively updating your library, fostering community feedback, and maintaining clear communication can establish trust with your users, leading to wider adoption and contribution.
If you’re looking to delve deeper into Android development and library creation, consider exploring Android Developers Documentation, or join communities on platforms like Stack Overflow or GitHub for valuable insight and support.
Remember, a well-constructed library can elevate your skills as a developer and provide solutions that benefit many users across the globe. Happy coding!