Mastering Java 8: Common Mistakes with Functional Interfaces
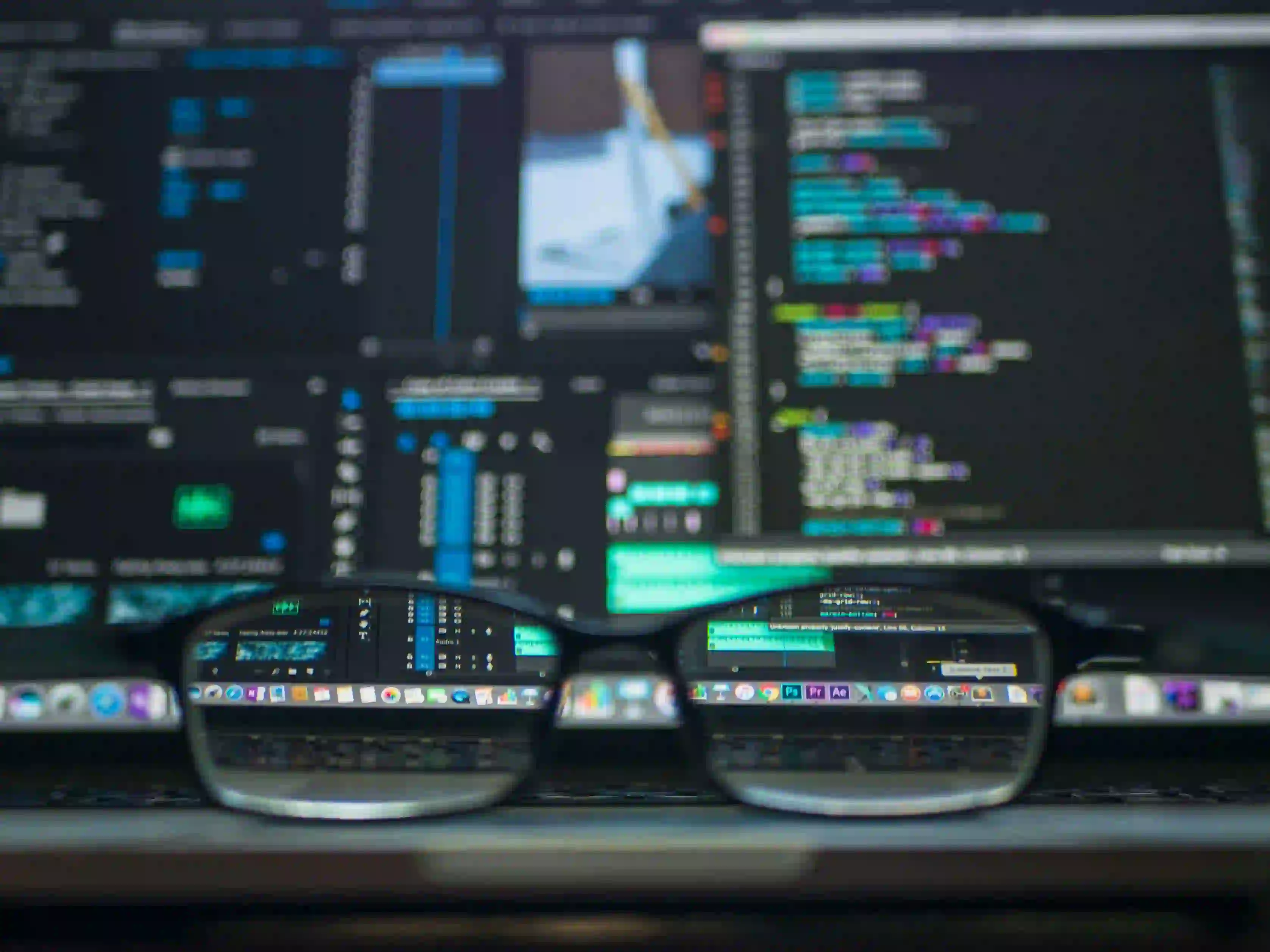
Mastering Java 8: Common Mistakes with Functional Interfaces
Java 8 introduced a paradigm shift in Java programming by introducing functional programming capabilities. One of the core components of this new approach is the use of functional interfaces. These interfaces empower developers to write cleaner, more concise code. However, along with this power comes common mistakes that can hinder the effectiveness of functional programming. This post will delve into those common pitfalls, offering guidance on how to avoid them while mastering the use of functional interfaces in Java 8.
What are Functional Interfaces?
In Java, a functional interface is an interface that contains exactly one abstract method. This characteristic makes it suitable for use with lambda expressions, providing a clear and concise way to apply functional programming principles. The @FunctionalInterface
annotation is used to indicate that an interface is intended to be functional.
Here is a simple example of a functional interface:
@FunctionalInterface
public interface MyFunctionalInterface {
void execute(String message);
}
Why Use Functional Interfaces?
Functional interfaces promote a functional style of programming that makes code more readable and easier to maintain. They allow the use of lambda expressions, simplifying the implementation of methods without the verbosity of traditional anonymous classes.
Common Mistakes with Functional Interfaces
Despite the advantages, many developers encounter pitfalls when working with functional interfaces. Let's examine these common mistakes in detail.
1. Not Using @FunctionalInterface
Annotation
Mistake: Failing to annotate your interface as a functional interface.
While it's not mandatory to use the @FunctionalInterface
annotation, doing so enhances code readability and helps catch errors during compile time. It ensures that your interface adheres to the rules of a functional interface. If you accidentally add a second abstract method, the compiler will throw an error.
@FunctionalInterface
public interface MyFunctionalInterface {
void execute(String message);
// Uncommenting the following method will cause a compiler error
// void anotherMethod();
}
Why: This annotation acts as an implicit contract between the developer and the compiler, helping you catch accidental changes that could break the intended functionality.
2. Confusing Functional Interfaces with Default Methods
Mistake: Overlooking the concept of default methods in functional interfaces.
In Java 8, functional interfaces can contain default methods, but these do not count as abstract methods. Always remember that a functional interface must have one and only one abstract method.
@FunctionalInterface
public interface MyFunctionalInterface {
void execute(String message);
default void preExecute() {
System.out.println("Preparing to execute...");
}
}
Why: Understanding this differentiation prevents confusion and ensures that you are maintaining the integrity of your functional interfaces.
3. Chaining Functional Interfaces Incorrectly
Mistake: Not chaining functional interfaces properly when multiple operations are required.
Chaining can increase readability and maintainability. Consider leveraging Java Streams, which use functional interfaces like Function
, Consumer
, and Predicate
.
import java.util.List;
import java.util.function.Predicate;
import java.util.stream.Collectors;
public class Example {
public static void main(String[] args) {
List<String> names = List.of("Alice", "Bob", "Charlie");
List<String> filteredNames = filter(names, name -> name.startsWith("A"));
System.out.println(filteredNames);
}
public static List<String> filter(List<String> names, Predicate<String> predicate) {
return names.stream()
.filter(predicate)
.collect(Collectors.toList());
}
}
Why: Using functional interfaces in a streaming context allows for more elegant and more scalable data manipulation. Chaining should be, however, done carefully to maintain readability.
4. Ignoring Null Pointer Exceptions with Optional
Mistake: Assuming that functional interfaces will safely handle null values.
Functional programming encourages the avoidance of nulls. If your functional interface can return null, be sure to use Java 8’s Optional
to avoid unnecessary NullPointerException
.
import java.util.Optional;
@FunctionalInterface
public interface MyFunction {
Optional<String> getValue();
}
public class Example {
public static void main(String[] args) {
MyFunction myFunction = () -> Optional.ofNullable(null);
System.out.println(myFunction.getValue().orElse("No Value Present"));
}
}
Why: Using Optional
allows for more expressive handling of potential null values, minimizing the risk of unexpected crashes in your application.
5. Not Leveraging Built-in Functional Interfaces
Mistake: Creating your own functional interfaces unnecessarily.
Java 8 provides a variety of built-in functional interfaces in the java.util.function
package, including Consumer
, Supplier
, Function
, Predicate
, etc. You should prefer using these pre-defined interfaces as they are well-tested, keep your code clean, and improve its readability.
import java.util.function.Consumer;
public class Example {
public static void main(String[] args) {
Consumer<String> printToConsole = System.out::println;
printToConsole.accept("Hello, World!");
}
}
Why: Utilizing built-in functional interfaces reduces boilerplate code, making your implementation more concise.
Closing Remarks
Mastering functional interfaces in Java 8 is not just about understanding how they work, but also about avoiding common pitfalls that can reduce code quality and maintainability. By using the @FunctionalInterface
annotation, understanding the inclusion of default methods, effectively chaining interfaces, managing null safety with Optional
, and embracing built-in interfaces, you can harness the true power of functional programming in Java.
Additional Resources
For further exploration of functional programming principles in Java, check out the following resources:
- Java Functional Interfaces
- Effective Java by Joshua Bloch - Chapter on Lambdas
- Java Streams Documentation
By being conscious of these common mistakes, you will be well on your way to mastering Java 8's functional interfaces, resulting in cleaner, more efficient code. Happy coding!