Common Pitfalls When Using Java S3Proxy Library
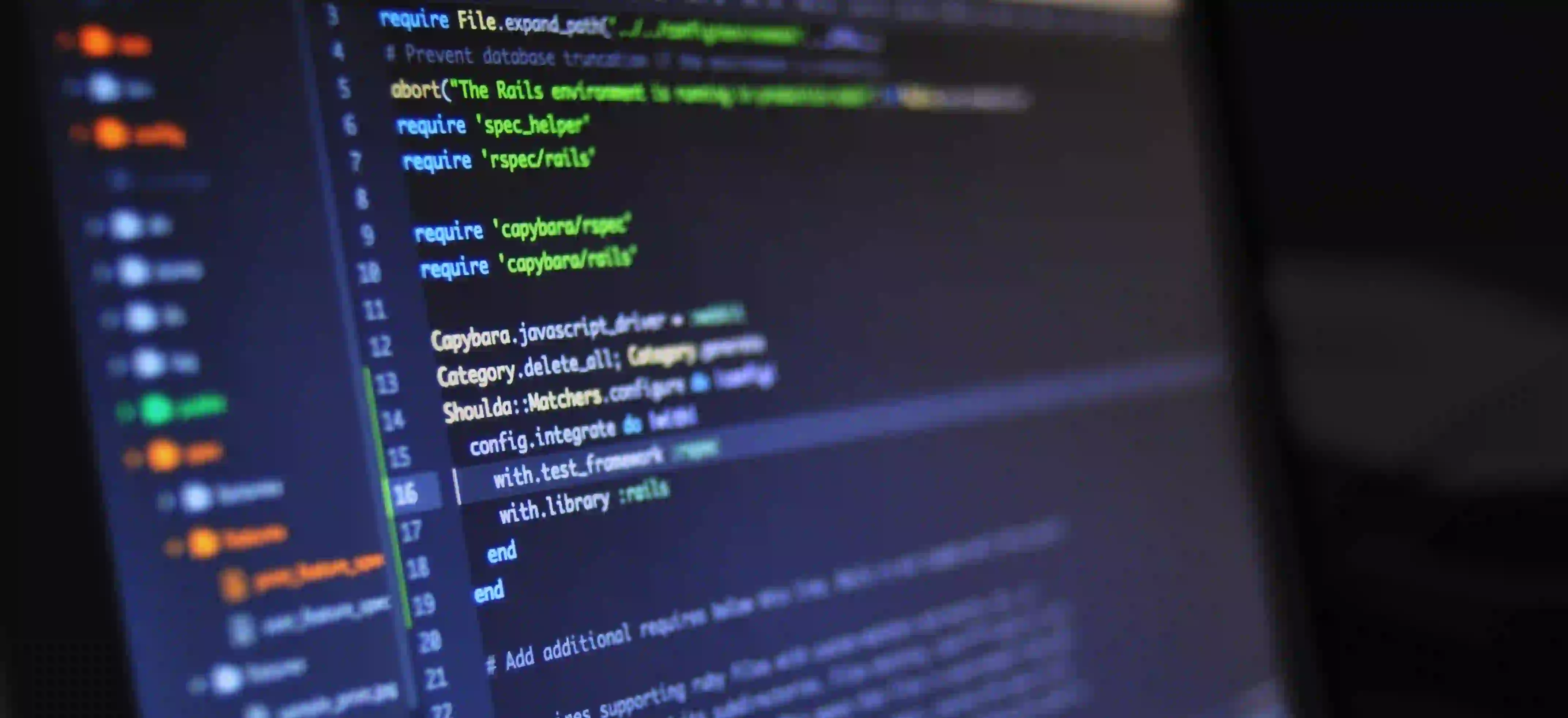
Common Pitfalls When Using the Java S3Proxy Library
When developing applications that require cloud storage solutions, you may come across various libraries designed to interact with services like Amazon Web Services (AWS) S3. One such library is the Java S3Proxy library. While it offers a robust and flexible way to integrate your Java applications with S3, there are several pitfalls developers often encounter. This blog post will explore these pitfalls and provide insights into how to avoid them.
What is S3Proxy?
Before we dive into the pitfalls, it’s beneficial to clarify what S3Proxy is. S3Proxy is an open-source tool designed to bridge between cloud storage services, like AWS S3, and your local or remote filesystem-based applications. It enables seamless access to files in cloud storage so that they can be interacted with as if they were local files. This functionality is particularly useful when working with Java-based applications, as it allows developers to utilize standard file I/O operations.
Common Pitfalls
Here are some common pitfalls developers encounter while utilizing the Java S3Proxy library.
1. Incorrect Configuration Settings
One of the most widespread issues is improper configuration of the S3Proxy. Configuration parameters are critical; without the right setup, your application won't be able to connect to the S3 service effectively.
Example
A typical configuration might look like this:
s3:
aws-zones: us-east-1
aws-endpoint: s3.amazonaws.com
aws-access-key: YOUR_ACCESS_KEY
aws-secret-key: YOUR_SECRET_KEY
bucket-name: your-bucket-name
Commentary: Always validate your configuration file's syntax and values before running your application. Misconfigured access keys or bucket names can lead to authorization errors, making debugging painful.
2. Ignoring Exception Handling
Another common pitfall is inadequate exception handling. Cloud storage operations can fail for various reasons—network issues, permission changes, etc. Ignoring these factors can lead to unforeseen application crashes.
Example
Consider an S3 upload operation:
try {
s3Client.putObject(new PutObjectRequest(bucketName, keyName, file));
} catch (AmazonServiceException e) {
System.err.println("Amazon service error: " + e.getMessage());
} catch (SdkClientException e) {
System.err.println("SDK client error: " + e.getMessage());
}
Commentary: Incorporate comprehensive exception handling to provide clear, actionable error messages. This can significantly aid debugging and improve user experience.
3. Not Using Multipart Uploads for Large Files
When uploading large files, developers sometimes opt for single-part uploads, which can be inefficient and error-prone. Instead, leveraging multipart uploads can enhance performance.
Example
Here’s how you can initiate a multipart upload:
MultipartUploadRequest multipartUploadRequest = new InitiateMultipartUploadRequest(bucketName, keyName);
String uploadId = s3Client.initiateMultipartUpload(multipartUploadRequest).getUploadId();
// Assuming you've divided your file into parts
for (Part part : parts) {
s3Client.uploadPart(new UploadPartRequest()
.withBucketName(bucketName)
.withKey(keyName)
.withUploadId(uploadId)
.withPartNumber(part.getPartNumber())
.withInputStream(part.getInputStream())
.withPartSize(part.getSize()));
}
Commentary: Multipart uploads enable you to upload files in parts, which can be retried independently if there’s a failure, making your uploads more resilient and faster.
4. Failing to Implement Versioning
Document management often requires version control. However, many developers building applications on S3 forget to enable versioning on their buckets, resulting in data loss.
Example
You can enable bucket versioning using the S3 console or programmatically:
SetBucketVersioningConfigurationRequest request = new SetBucketVersioningConfigurationRequest(bucketName, new BucketVersioningConfiguration().withStatus(BucketVersioningConfiguration.ENABLED));
s3Client.setBucketVersioningConfiguration(request);
Commentary: By enabling versioning, you can recover from accidental deletions or overwrites by retrieving previous versions of your files.
5. Overlooking Local Testing and Development
Developers frequently skip testing their code locally, especially when the application's main features rely on cloud integrations. Not testing can cause significant issues once the application is deployed.
Solution
LocalStack is a popular emulation tool that lets you run AWS services locally. Set it up to mimic an S3 environment:
docker run -d -p 4566:4566 -p 4510-4559:4510-4559 localstack/localstack
Commentary: Local testing allows you to catch issues early, reducing friction in deployment and ensuring a more stable application.
6. Using Hardcoded Credentials
Hardcoding credentials is a significant security risk. If your code is ever exposed, sensitive details like AWS access and secret keys are made vulnerable.
Example
Instead of:
String accessKey = "YOUR_ACCESS_KEY";
String secretKey = "YOUR_SECRET_KEY";
Use environment variables or AWS Identity and Access Management (IAM) roles:
String accessKey = System.getenv("AWS_ACCESS_KEY");
String secretKey = System.getenv("AWS_SECRET_KEY");
Commentary: This approach helps maintain security and simplifies credential management when deploying applications in different environments.
7. Ignoring IAM Policies
Bucketing operations often require fine-grained permissions. Developers sometimes neglect to review IAM (Identity and Access Management) policies associated with their S3 access.
Example
Make sure your IAM role includes necessary permissions like:
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": [
"s3:PutObject",
"s3:GetObject",
"s3:ListBucket"
],
"Resource": "arn:aws:s3:::your-bucket-name/*"
}
]
}
Commentary: Regularly audit IAM policies and permissions to ensure your application operates correctly and securely.
The Last Word
While the Java S3Proxy library provides a robust solution for interacting with cloud storage, it's essential to be mindful of potential pitfalls. By addressing configuration issues, implementing robust exception handling, utilizing multipart uploads, enabling versioning, conducting local tests, avoiding hardcoded credentials, and carefully managing IAM policies, you can create a more effective and secure application.
For further reading and to dive deeper into best practices for using AWS S3, you can check out AWS Documentation or S3Proxy GitHub Repository. These resources provide detailed insights and examples to help you build a scalable and secure foundation for your applications.
By remaining vigilant and aware of these common pitfalls, you can facilitate a smoother development experience and create applications that effectively leverage the power of cloud storage.