JIT Compilation: Balancing Performance with Complexity
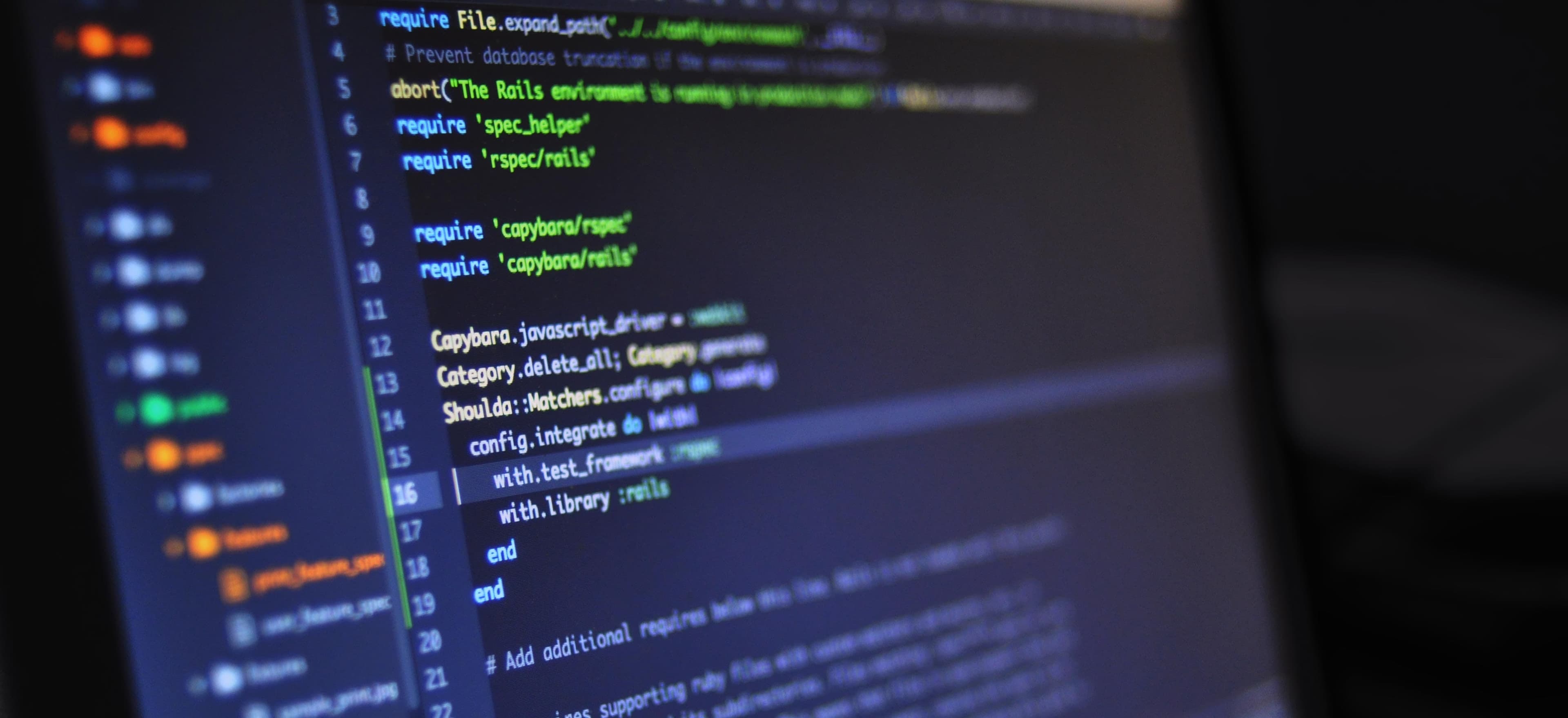
- Published on
JIT Compilation: Balancing Performance with Complexity
Just-In-Time (JIT) compilation is a powerful technique used in programming languages that run atop a virtual machine, such as Java. It acts as a bridge between high-level code and machine-level instructions. Understanding JIT compilation is critical for developers who want to optimize their applications for speed and resource efficiency. In this blog post, we will explore how JIT compilation works, its benefits and drawbacks, and practical examples to illustrate its importance.
What is JIT Compilation?
JIT compilation refers to a runtime process that translates bytecode into native machine code. Unlike traditional compilation, which occurs before execution, JIT compilation happens while the program is running. This allows for dynamic optimizations based on the current execution context, leading to increased performance for long-running applications.
How JIT Compilation Works
When you run a Java application, the Java compiler (javac) first converts the Java code (.java files) into bytecode (.class files). The Java Virtual Machine (JVM) then executes this bytecode. Here's the critical part: instead of interpreting the bytecode line by line, the JIT compiler converts frequently executed code paths into optimized machine code that the CPU can execute directly.
Below is a simplified flow of how JIT compilation works:
- Loading: The JVM loads the bytecode.
- Execution: Initially, the JVM interprets the bytecode.
- Profiling: The JIT compiler analyzes which methods are frequently called.
- Compilation: The JIT compiler converts these "hot" methods into native code.
- Execution: Subsequent calls to these methods run much faster, as they now use the native code instead of bytecode.
Example of JIT Compilation
To demonstrate the power of JIT compilation, consider the following simple Java method that calculates the sum of an array of integers:
public class ArraySum {
public static int sum(int[] arr) {
int total = 0;
for (int i = 0; i < arr.length; i++) {
total += arr[i];
}
return total;
}
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5};
System.out.println("Sum: " + sum(numbers));
}
}
Why Use JIT Compilation?
-
Performance Improvement: JIT compilation significantly boosts performance for long-running applications. If a method is called frequently, it transitions from interpreted bytecode to optimized machine code, reducing overhead substantially.
-
Dynamic Optimization: The JIT compiler can take advantage of runtime information to apply optimizations. For example, it can inline methods and eliminate redundant calculations.
-
Adaptability: The JVM can adapt to different workloads. As methods become “hot,” they are compiled, while less frequently used methods remain interpreted. This balance ensures resources are not wasted on compiling seldom-used code.
Balancing Complexity and Performance
Despite its power, JIT compilation introduces complexity. It’s essential to understand the trade-offs involved:
-
Warm-Up Time: JIT compilation requires time to compile methods and optimize. For short-lived applications, the startup cost could outweigh the benefits. Hence, JIT is highly effective for long-running applications.
-
Memory Usage: The compiled machine code resides in memory, which can increase RAM usage. For applications with limited memory resources, this might lead to performance bottlenecks.
-
Thresholds for Optimization: The JIT compiler uses various thresholds to determine when to compile a method. Understanding these thresholds helps developers make informed choices about their applications.
-
Profiling Overhead: During the profiling phase, the JVM incurs additional overhead while monitoring method execution. This can reduce performance during the initial run.
Example of HotSpot JIT Compiler
Java includes HotSpot, one of the most widely used JIT compilers. Let's look at how we can enable some of the enhancements provided by the HotSpot compiler:
java -XX:+UseCompiler -XX:CompileThreshold=1000 MyApplication.class
In this command:
- -XX:+UseCompiler: Tells the JVM to use the JIT compiler.
- -XX:CompileThreshold=1000: Sets the threshold for method compilation at 1000 invocations.
This command allows us to tweak our application's performance based on profiling criteria. Tuning these parameters can lead to significant performance improvements.
Real-World Applications of JIT Compilation
In practice, JIT compilation finds its place in various applications, from large-scale enterprise systems to gaming engines. For example, the Java-based game engines leverage JIT because many processes, such as real-time graphics rendering, are computationally intensive and repetitive. The gains made possible by JIT compilation can drastically enhance user experience by minimizing lag and boosting frame rates.
Additional Resources on JIT Compilation
To dive deeper into JIT compilation and its nuances, consider these resources:
-
Java Performance: The Definitive Guide - This book provides an in-depth look at how to enhance Java applications using JIT and other performance enhancements.
-
Understanding the Java HotSpot VM - This official guide offers insights into how the HotSpot JVM functions, including the JIT compilation process.
My Closing Thoughts on the Matter
JIT compilation is a pivotal feature of the Java ecosystem, transforming code from interpreted bytecode into optimized machine code, providing speed enhancements that significantly benefit long-running applications. Despite the complexities and trade-offs, the performance gains from JIT compilation, especially in specific scenarios, make it a vital consideration for any Java developer.
While we've provided a high-level overview here, the intricate details of JIT compilation merit ongoing exploration as Java technology continues to evolve. With the increasing demand for performance efficiency in modern applications, understanding and leveraging JIT compilation will be indispensable for developers seeking to optimize their Java applications.
By keeping abreast of advancements and best practices, you'll be well-equipped to navigate the balance between performance and complexity in your Java applications. Happy coding!
Checkout our other articles