Troubleshooting Common @ResponseBody Issues in Spring MVC
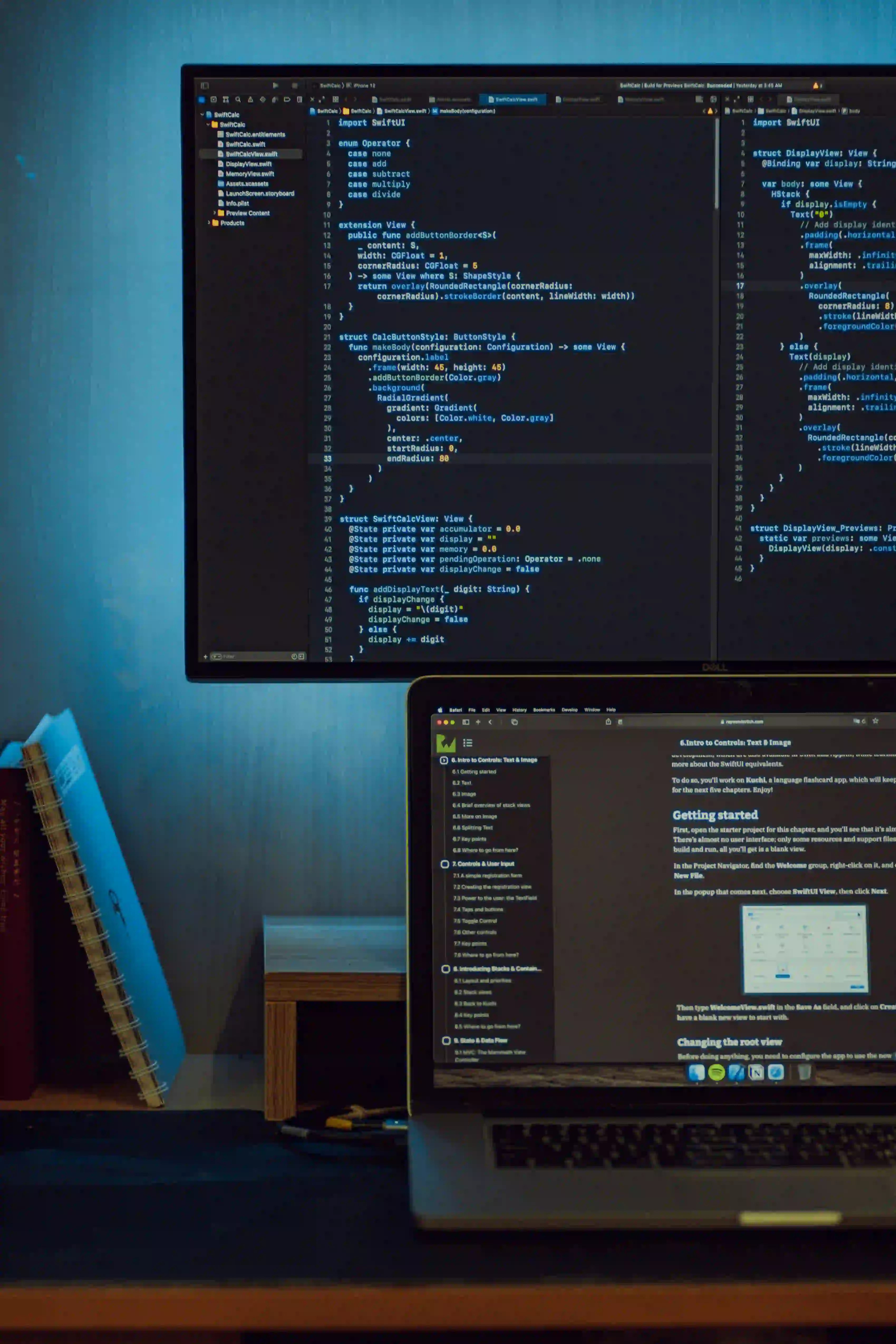
Troubleshooting Common @ResponseBody Issues in Spring MVC
In the modern web development ecosystem, REST APIs have become essential for building responsive applications. Spring MVC provides robust support for creating RESTful web services, allowing developers to serialize their Java objects into JSON or XML and send them back as responses. This is facilitated primarily through the @ResponseBody
annotation. However, while working on Spring MVC, developers often encounter various issues when using @ResponseBody
. In this blog post, we will troubleshoot these common issues and provide valuable insights for effective resolution.
Table of Contents
- Understanding @ResponseBody
- Common Issues with @ResponseBody
- Incorrect Return Type
- Missing Dependencies
- Content Negotiation Problems
- Serialization Issues
- Best Practices
- Conclusion
- Additional Resources
Understanding @ResponseBody
The @ResponseBody
annotation is a pivotal component of Spring MVC. It indicates that the return value of a method should be bound to the web response body. This essentially allows you to create RESTful endpoints that produce JSON or XML outputs, determined by the content type specified.
For instance, consider a simple controller method that returns a User
object:
@RestController
public class UserController {
@GetMapping("/user/{id}")
@ResponseBody
public User getUser(@PathVariable Long id) {
User user = userService.getUserById(id);
return user; // Will be serialized to JSON
}
}
In this example, Spring will automatically serialize the User
object to JSON format and return it to the client.
Common Issues with @ResponseBody
While @ResponseBody
adds immense functionality, it can lead to several complications. Understanding these can aid in effective troubleshooting.
Incorrect Return Type
One common issue arises when the method returns a type that cannot be serialized. For example, returning a raw List
instead of a typed List<User>
can lead to problems.
Example of the Issue:
@GetMapping("/users")
@ResponseBody
public List getUsers() { // Incorrect usage
return userService.getAllUsers(); // Will throw serialization error
}
Solution:
Specify the generic type to help Spring understand what it needs to serialize.
@GetMapping("/users")
@ResponseBody
public List<User> getUsers() { // Correct usage
return userService.getAllUsers(); // Will be serialized correctly
}
Missing Dependencies
Spring relies on certain libraries to perform JSON serialization, such as Jackson or Gson. If these dependencies are missing, calls to methods annotated with @ResponseBody
may return an error.
Solution:
Ensure that you have added the necessary dependencies in your pom.xml
if using Maven. For Jackson, the dependency looks like this:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
</dependency>
Having the correct version is crucial as well. Spring Boot usually manages these dependencies for you, but if you are configuring Spring MVC manually, ensure that relevant libraries are included.
Content Negotiation Problems
Content negotiation is the mechanism by which the client can specify the desired response format (e.g., JSON, XML). If the appropriate Content-Type
or Accept
headers are not sent in the request, the server might not respond as expected.
Example of the Issue:
GET /user/1 HTTP/1.1
Host: example.com
Accept: text/html
In this case, since the Accept
header specifies HTML, the server will attempt to return an HTML view instead of JSON.
Solution:
Make sure that your requests specify the correct Accept
header. For JSON responses, it should be:
GET /user/1 HTTP/1.1
Host: example.com
Accept: application/json
Serialization Issues
Serialization problems usually occur due to configurations or the structure of the object itself. For instance, if your model class has circular references, the JSON serialization will fail.
Example of Circular Reference:
public class User {
private Long id;
private String name;
private List<Order> orders;
// Getter/Setters
}
public class Order {
private Long id;
private User user; // Circular reference
// Getter/Setters
}
Solution:
Using annotations from libraries like Jackson, such as @JsonIgnore
, can help to manage circular references.
public class Order {
private Long id;
@JsonIgnore
private User user; // Ignore this during serialization
// Getter/Setters
}
Best Practices
To avoid issues related to @ResponseBody
, consider following these best practices:
-
Specify Return Types: Always specify the return types explicitly. This aids in clarity and avoids serialization errors.
-
Handle Exceptions Globally: Use
@ControllerAdvice
to globally handle exceptions and provide meaningful JSON responses. -
Use Proper Annotations: Leverage
@RestController
instead of@Controller
+@ResponseBody
for clarity. -
Validate Input: Ensure to validate your incoming requests for expected formats, reducing errors on serialization.
-
Document APIs: Use Swagger or Spring REST Docs to document your APIs, making it easier for consumers to understand expected structures.
In Conclusion, Here is What Matters
In summary, while @ResponseBody
in Spring MVC enhances your web services, it is essential to be cognizant of the common issues that can arise. By understanding the common pitfalls—incorrect return types, missing dependencies, content negotiation, and serialization issues—you can troubleshoot effectively and create a smoother API experience for users.
Explore the Spring documentation for more insights on developing RESTful services: Spring MVC Documentation.
Additional Resources
Feel free to download the code snippets provided in this post and start optimizing your Spring MVC applications today. Happy coding!