Choosing Between For-Loops and Stream ForEach in Java
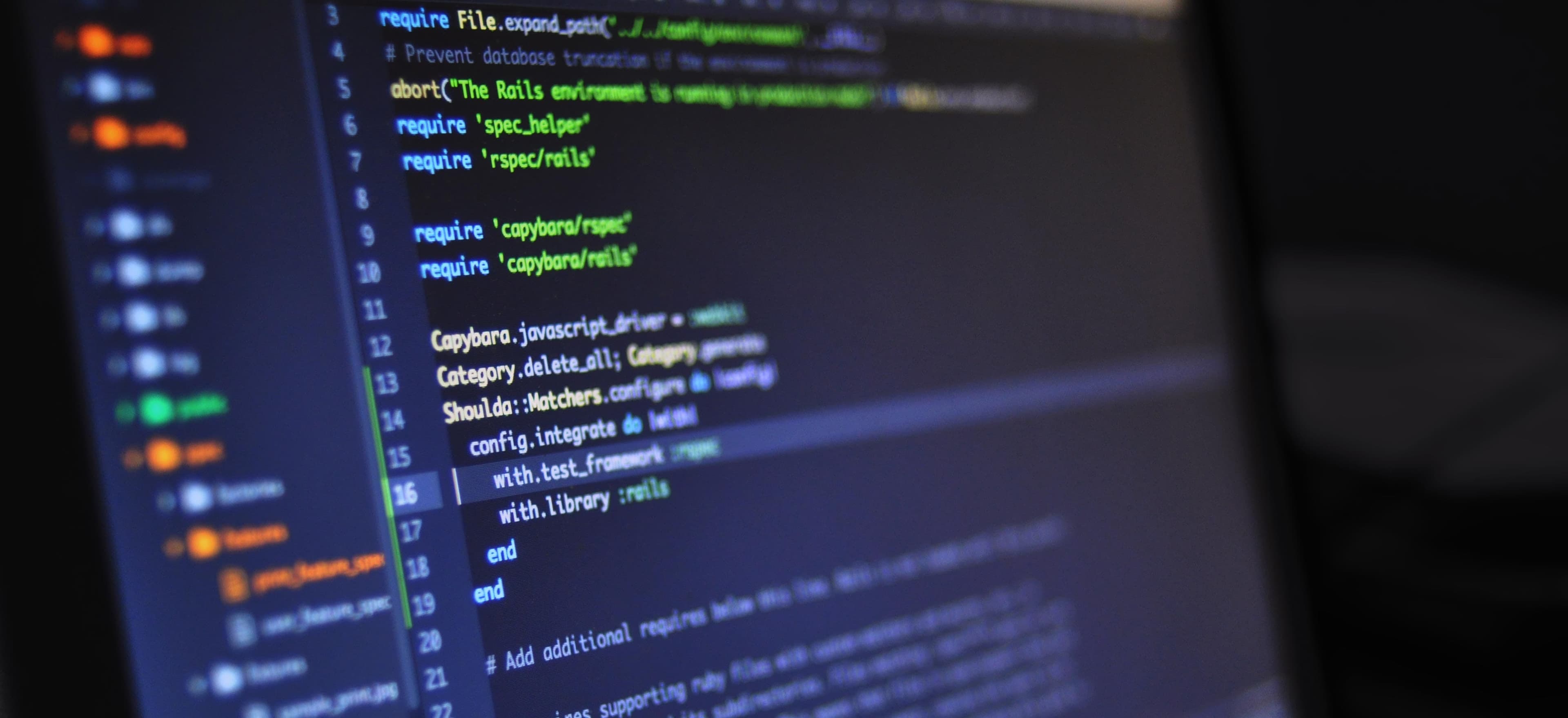
- Published on
Choosing Between For-Loops and Stream ForEach in Java
Java is a versatile programming language that offers various options for iterating over collections. Among the most common mechanisms are traditional for-loops and the more modern Stream.forEach()
method available in the Java Stream API.
In this blog post, we will explore the differences between these two techniques, their respective use cases, performance considerations, and coding examples. Let us dive into the details.
For-Loops: Classic and Straightforward
A for-loop is perhaps the most recognizable control structure in programming. In Java, a traditional for-loop iterates over a collection by manually controlling the index or iterator.
Basic Structure of a For-Loop
Here's a simple example using a for-loop to iterate over an array of integers:
int[] numbers = {1, 2, 3, 4, 5};
for (int i = 0; i < numbers.length; i++) {
System.out.println(numbers[i]);
}
Advantages of For-Loops
- Simplicity: For-loops are straightforward and easy to read, especially for beginners.
- Control: You can easily manipulate the loop counter, allowing conditional breaks and continues.
- Flexibility: For-loops allow for complex operations that may not fit into a functional style.
Disadvantages of For-Loops
- Verbosity: They often require more lines of code compared to their Stream counterparts.
- Error-Prone: Manual index management can lead to errors, resulting in
ArrayIndexOutOfBoundsException
.
Stream ForEach: Modern and Functional
The Stream API, introduced in Java 8, promotes a functional style of programming, making it easier to process collections of objects. The forEach
method allows for concise and readable code.
Basic Structure of Stream ForEach
Here’s how you can achieve the same functionality as the for-loop using Streams:
import java.util.Arrays;
public class StreamExample {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5};
Arrays.stream(numbers).forEach(System.out::println);
}
}
Advantages of Stream ForEach
- Conciseness: Shorter code is often easier to read and maintain.
- Parallel Processing: Streams can leverage multi-core architectures for potential performance gains. Using
.parallelStream()
enables this. - Declarative Style: The functional style of Streams leads to cleaner code, focusing on what needs to be done rather than how to do it.
Disadvantages of Stream ForEach
- Limited Control: The declarative approach does not allow for easy index manipulation, making complex conditions harder to implement.
- Overhead: There may be some performance overhead with Streams, especially for small collections due to setup time.
Performance Considerations
The choice between for-loops and Stream forEach
can sometimes boil down to performance.
- Small Collections: For small datasets, the difference is negligible. The readability of code may take priority.
- Large Collections: For large datasets, using the
parallelStream
can improve performance, but context matters. In cases where operations are straightforward and not computationally intensive, the clarity of a for-loop might be more advantageous.
Example of Parallel Processing
Here is an example of using parallel streams:
import java.util.List;
import java.util.Arrays;
public class ParallelStreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Using parallel stream for better performance
numbers.parallelStream().forEach(number -> {
System.out.println("Processing number: " + number);
});
}
}
In this example, each element might be processed in parallel. However, always ensure that the operation within the forEach
is thread-safe when using parallel streams.
Use Cases and Conclusion
Choosing between a for-loop and a Stream forEach
should be guided by several factors:
- Readability and Maintainability: If the operation is simple,
forEach
could be preferable for its brevity. - Complex Operations: If you involve complex iterations or manipulations, a for-loop might be the way to go.
- Performance Needs: In performance-critical applications, benchmark both to make an informed decision.
Tips for Best Practices
- For simple tasks, prefer Streams for clarity.
- If manipulating indices or conditions heavily, stick with for-loops.
- Always profile performance if in doubt, as performance can vary by context and data size.
Additional Resources
For more in-depth understanding and examples, consider exploring the following resources:
- Java Stream API Documentation
- Understanding Java for Loops
- Java Performance: Streams vs for Loops
Final Thoughts
Both for-loops and Stream forEach
have their unique strengths and weaknesses. Knowing when to use each construct can drastically improve readability and performance in your Java applications. Evaluate your specific use case, and choose the approach that best aligns with your goals. Happy coding!
Checkout our other articles