Troubleshooting JMX MBean Exposure in Spring Applications
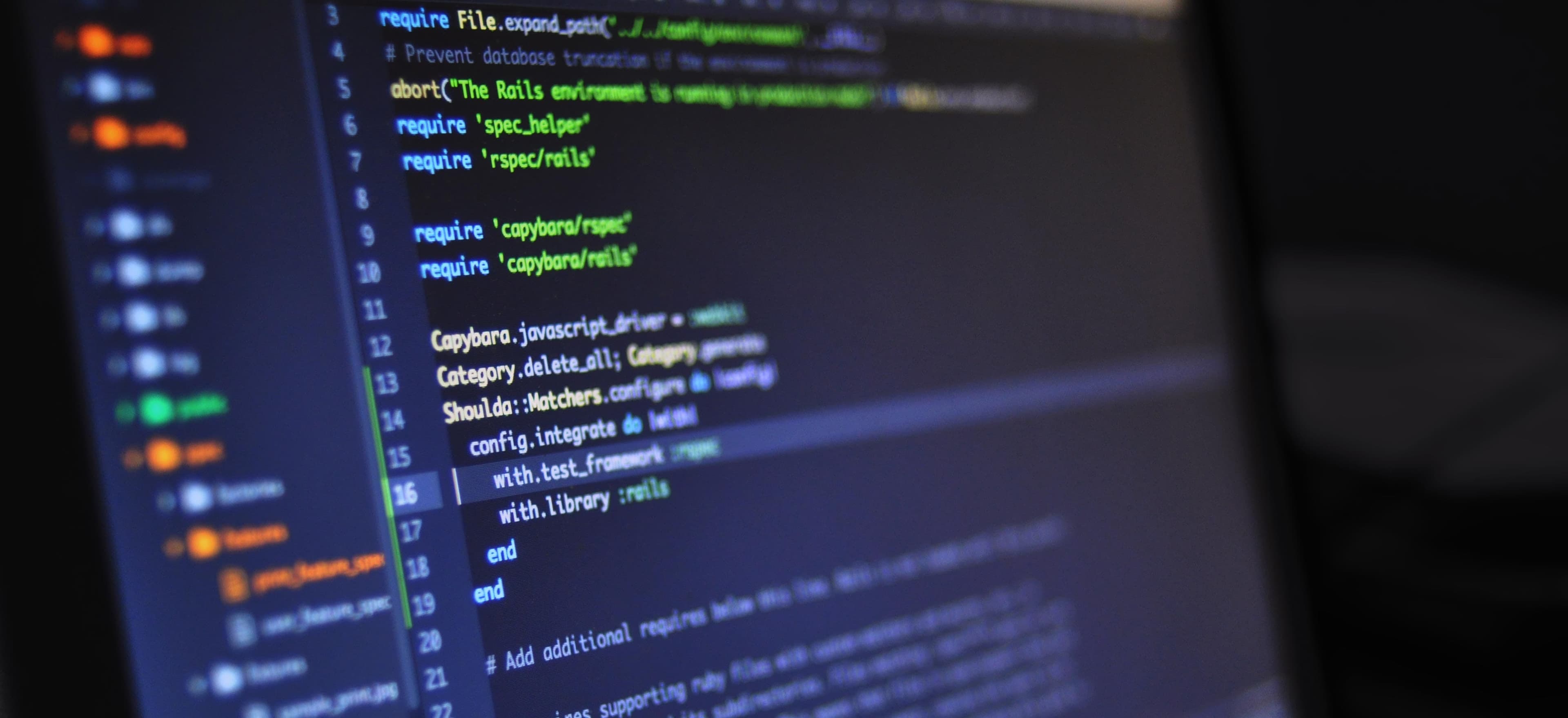
- Published on
Troubleshooting JMX MBean Exposure in Spring Applications
Java Management Extensions (JMX) is a powerful technology that provides a means for managing and monitoring Java applications. If you're developing a Spring application, you might want to expose certain beans as MBeans to monitor their state and behavior in a running application. However, there can be complexities in getting everything set up. This guide will help you troubleshoot common issues related to JMX MBean exposure in Spring applications.
Understanding JMX and MBeans
JMX is a Java technology that allows for the management of resources such as applications, system objects, and devices. An MBean (Managed Bean) is a Java object that represents a manageable resource. MBeans can be used to expose metrics, manage settings dynamically, or perform operations remotely.
Why Use JMX in Spring?
- Monitoring: Easily observe how your application’s beans are performing.
- Configuration Management: Change settings without redeploying.
- Debugging: Monitor application state without invasive measures.
Setting Up JMX in a Spring Application
Let’s look at a basic configuration of JMX in a Spring Boot application.
Maven Dependency
First, ensure you have the required dependencies in your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jmx</artifactId>
</dependency>
Application Configuration
Next, enable JMX in your application.properties
:
spring.jmx.enabled=true
spring.jmx.default-domain=my.app.domain
Exposing a Bean
To expose a bean as an MBean, annotate it with @ManagedResource
; alongside this, methods can be annotated with @ManagedOperation
or @ManagedAttribute
to control operations and attributes you want to expose.
import org.springframework.jmx.export.annotation.ManagedResource;
import org.springframework.jmx.export.annotation.ManagedOperation;
@ManagedResource(objectName = "my.app:type=MyManagedBean", description = "My Managed Bean")
public class MyManagedBean {
private int value;
@ManagedAttribute(description = "Get the current value")
public int getValue() {
return value;
}
@ManagedAttribute(description = "Set the current value")
public void setValue(int value) {
this.value = value;
}
@ManagedOperation(description = "Increment the value")
public void incrementValue() {
this.value++;
}
}
Running the Application
When you run your Spring application, the JMX MBean will be registered under “my.app:type=MyManagedBean”. You can interact with it using a JMX client, such as JConsole or VisualVM.
Common Issues in JMX MBean Exposure
Despite the straightforward setup, developers often face several common problems. Below are several typical issues and how you can resolve them.
1. MBean Not Registered
Symptoms:
- When you look for your MBean in JConsole, it's not listed.
Possible Solutions:
- Check JMX Configuration: Ensure that JMX is enabled in your
application.properties
file by verifyingspring.jmx.enabled=true
. - Class Scanning: Ensure your MBean class is scanned by Spring. If you use a specific configuration class, ensure the package scanning includes your MBean.
2. JMX Connection Issues
Symptoms:
- Unable to connect to JMX via tools like JConsole.
Possible Solutions:
- VM Options: You might need to set JMX-related VM options as follows:
-Dcom.sun.management.jmxremote
-Dcom.sun.management.jmxremote.port=12345
-Dcom.sun.management.jmxremote.authenticate=false
-Dcom.sun.management.jmxremote.ssl=false
- Make sure that the configured port is open and not blocked by firewalls.
3. Attribute Read/Write Failures
Symptoms:
- MBean attributes cannot be read or modified.
Possible Solutions:
- Check Annotations: Make sure that the methods you annotated with
@ManagedAttribute
have the correct method signatures:- It should return the data type of the attribute.
- The setter method should accept a corresponding type.
4. Exposed MBeans Not Reflecting Changes
Symptoms:
- Attribute values do not update as expected.
Possible Solutions:
- State Management: Ensure that the bean instance in your application is being managed correctly by Spring and that state changes are reflected in the attributes you've exposed.
5. MBean Object Name Issues
Symptoms:
- MBean appears with an unexpected name.
Possible Solutions:
- Define Correct Object Name: Use the
objectName
property in the@ManagedResource
annotation to set (and ensure uniqueness) of the MBean.
Integrating with Spring Boot Actuator
Spring Boot comes equipped with the Actuator module which can expose various management features via HTTP endpoints. You can enable JMX support for Actuator easily by updating your configuration:
management.endpoints.jmx.exposure.include=*
This configuration includes all Actuator endpoints in JMX, which is particularly useful for checking the health of your application, gathering metrics, and managing your beans.
Additional Resources
For those seeking to dive deeper into JMX and its integration with Spring, I recommend checking out the following links:
- Spring JMX Documentation
- JMX Overview on Oracle
Lessons Learned
JMX provides a robust framework for monitoring and managing your Spring applications. However, challenges can arise during setup and configuration. By keeping in mind the troubleshooting strategies outlined in this article, you can effectively manage and expose MBeans in your Spring applications. Remember to ensure these configurations align with your specific deployment environment for optimal performance.
With a solid understanding of JMX and the proper implementation of Spring annotations, you're well on your way to bolstering your application's robustness and observability. Happy coding!
Checkout our other articles