Breaking Free from the Magic Setter Antipattern
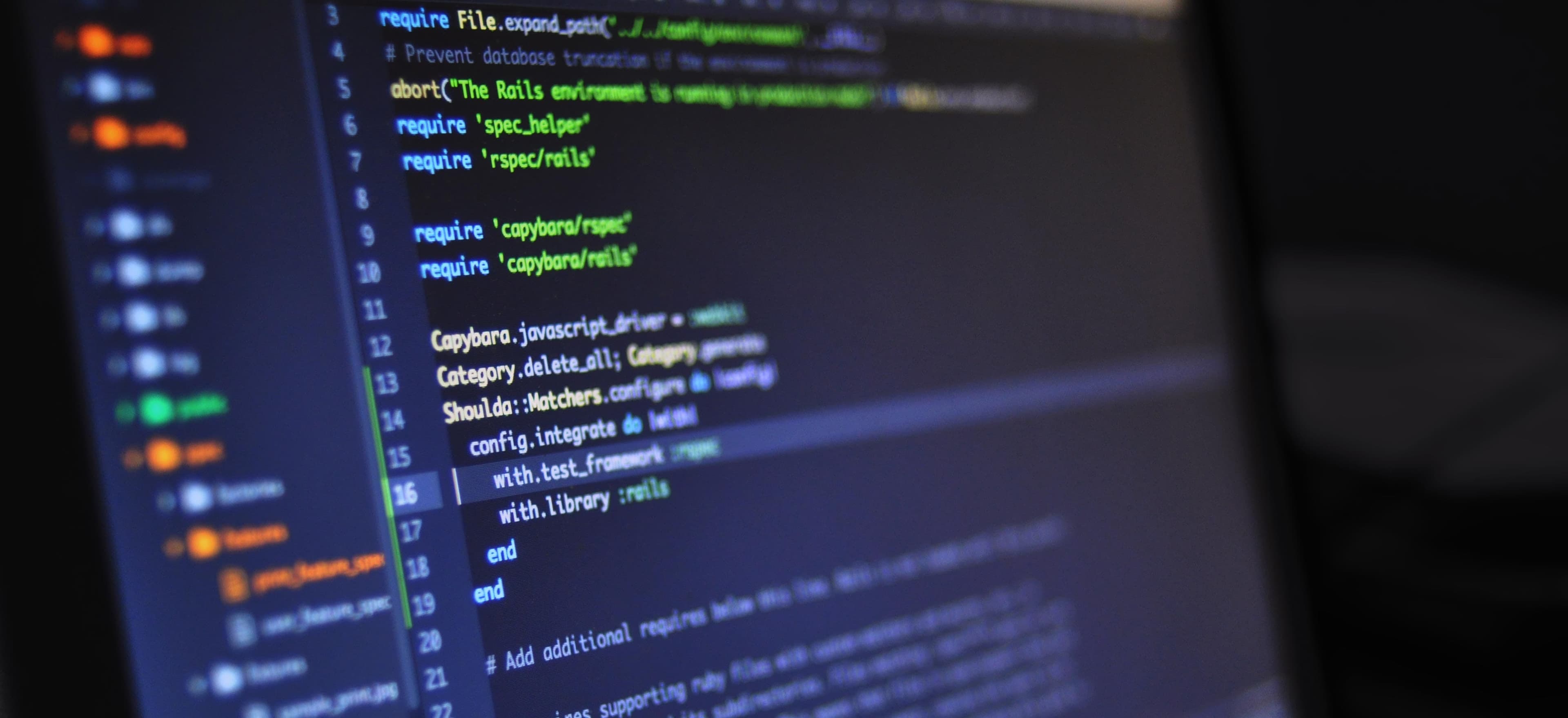
- Published on
Breaking Free from the Magic Setter Antipattern in Java
In the world of Java programming, design patterns offer solutions to common software development challenges. However, many developers inadvertently create "antipatterns," such as the Magic Setter antipattern. Understanding this antipattern and how to avoid it can elevate your code quality, improve maintainability, and lead to robust applications.
What is the Magic Setter Antipattern?
The Magic Setter antipattern occurs when a class uses setters to configure its state with minimal context. In this scenario, methods don't just set values—they might sometimes perform significant internal changes or validations implicitly. Developers using this approach may find the code less readable and harder to maintain, as it obscures the relationship between the object's state and behavior.
Characteristics of the Magic Setter Antipattern
- Implicit Logic: The setter methods perform hidden computations or validations, complicating the understanding of the object's state.
- Lack of Clarity: The class may seem simple, but there is often too much complexity hidden within the setter methods.
- Fragile Code: When changes are made, it can lead to cascading failures due to interdependent state updates amongst attributes.
Consider the following simple example that demonstrates the problem:
class UserProfile {
private String username;
private int age;
public void setUsername(String username) {
this.username = username;
// Implicit validation
if (username.length() < 3) {
throw new IllegalArgumentException("Username must be at least 3 characters.");
}
}
public void setAge(int age) {
this.age = age;
// Implicit adjustment
if (age < 18) {
this.age = 18; // Adjusting the age instead of throwing an error
}
}
}
In the above code, the setters contain logic that fails to make the purpose clear. The username is validated, while age is silently adjusted. This makes it difficult for users of the class to understand what happens when they call these methods, leading us straight into the realm of "magic."
The Dangers of Magic Setters
1. Increased Cognitive Load
As developers interact with an instance of the UserProfile, they may be unsure of the escaped behaviors tied to setters. This confusion leads to increased cognitive load, which is particularly challenging for new team members or external contributors.
2. Diminished Reusability
The tightly coupled logic embedded in setters limits how this class can be reused in other contexts. You may end up with complex test scenarios solely to account for the hidden behavior coded into the setters.
3. Difficulty in Testing
Testing becomes problematic because you must simulate all the side effects of calling a setter method. Reports can lead to a tangled web of dependencies, making unit tests verbose and harder to maintain.
Breaking Free: Refactoring the Magic Setter
Taking action to break away from this antipattern involves refactoring the code to enhance clarity, maintainability, and usability. Here are a few strategies.
1. Use Constructors for Required Fields
One effective technique is to use constructors to enforce required fields. This limits the need for setters:
class UserProfile {
private final String username;
private int age;
public UserProfile(String username, int age) {
if (username.length() < 3) {
throw new IllegalArgumentException("Username must be at least 3 characters.");
}
this.username = username;
setAge(age); // Utilize the setter for additional logic if needed
}
public void setAge(int age) {
if (age < 18) {
throw new IllegalArgumentException("Age must be at least 18");
}
this.age = age;
}
}
In this refactoring, we've moved username validation to the constructor, which clarifies that it's a required field. This design enforces immutability for username
and encapsulates the logic around age
.
2. Implement Builder Pattern
If complexity demands it or if a lot of optional fields are involved, consider using the Builder Pattern. This can provide greater clarity on how the object is constructed:
class UserProfile {
private final String username;
private final int age;
private UserProfile(Builder builder) {
this.username = builder.username;
this.age = builder.age;
}
public static class Builder {
private String username;
private int age = 18; // default age
public Builder setUsername(String username) {
if (username.length() < 3) {
throw new IllegalArgumentException("Username must be at least 3 characters.");
}
this.username = username;
return this;
}
public Builder setAge(int age) {
if (age < 18) {
throw new IllegalArgumentException("Age must be at least 18");
}
this.age = age;
return this;
}
public UserProfile build() {
return new UserProfile(this);
}
}
}
With the Builder Pattern, the customization of the UserProfile becomes explicit. Each step of the creation process is clear, making usage less error-prone and more self-explanatory.
3. Limit Side Effects
If setters are necessary, avoid embedding additional logic. Keep them straightforward to merely assign values. This maintains clarity in the code and eases the understanding of the object state.
class UserProfile {
private String username;
private int age;
public void setUsername(String username) {
this.username = username; // No validation here
}
public void setAge(int age) {
this.age = age; // Simple property setting
}
}
Incorporating clear interfaces, thorough documentation, and explicit method names all help to mitigate the magical aspects of your setters.
Closing Remarks
Magic Setters can turn manageable classes into complex, confusing entities. By recognizing the signs of this antipattern and applying the discussed strategies, developers can construct cleaner, more understandable codebases.
Creating clear constructors, using the Builder pattern, and eliminating side effects from setters are pivotal for breaking the chains of the Magic Setter antipattern. Not only does this lead to better code, but it also fosters a collaborative environment for teams working on shared codebases.
For further reading on design patterns and antipatterns in Java, you may want to look at Martin Fowler's Refactoring or Dive into GoF's classic book Design Patterns.
By leveraging these patterns and techniques, developers can work tirelessly toward building clean, maintainable, and high-quality Java applications.
Just remember: clarity first, and simplicity follows. Happy coding!