Common Pitfalls When Integrating Lucene Search
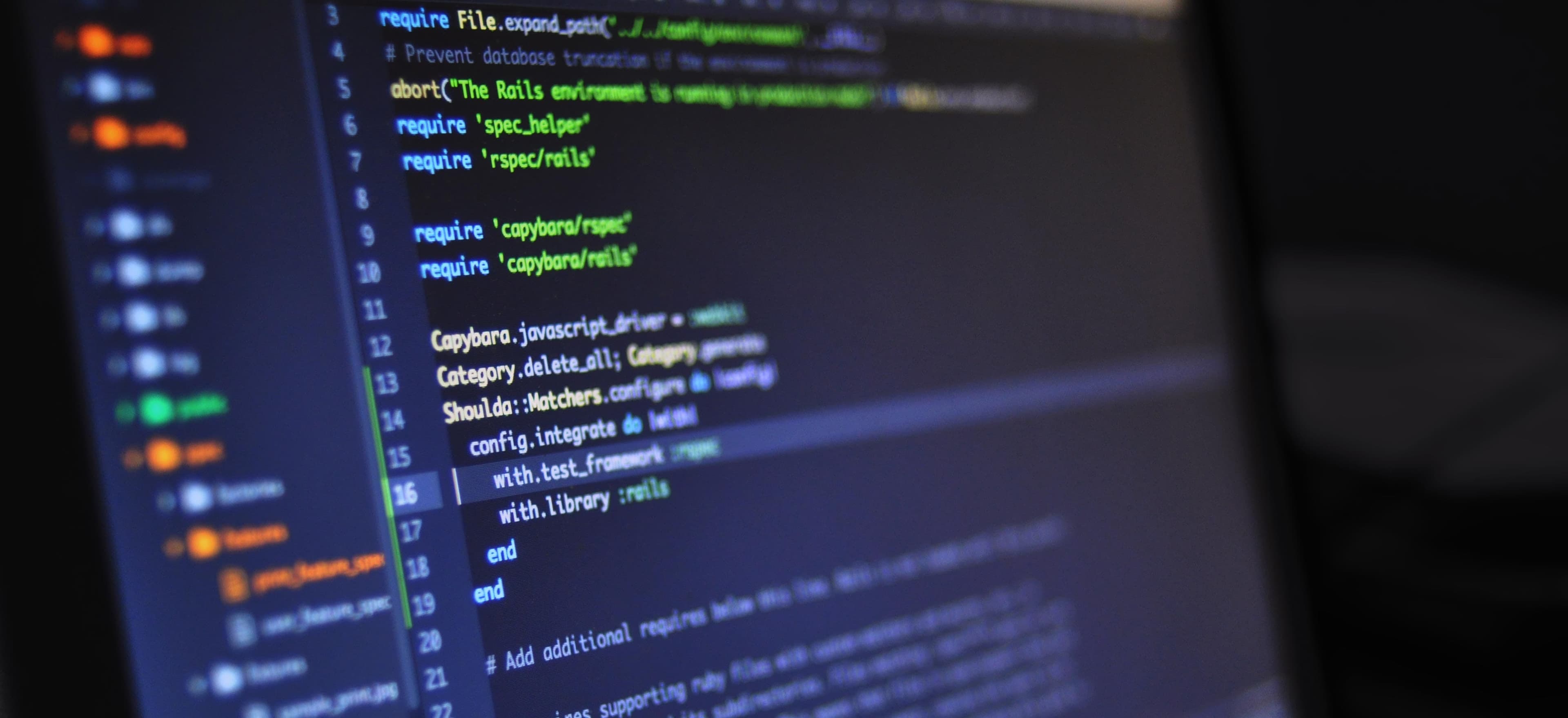
- Published on
Common Pitfalls When Integrating Lucene Search
Integrating Apache Lucene into your Java application can significantly enhance search capabilities. However, many developers encounter pitfalls that could lead to suboptimal performance and functionality. This blog post will explore these common issues and provide strategies to maneuver around them.
What is Apache Lucene?
Apache Lucene is an open-source search library written in Java. It's flexible, powerful, and designed for full-text indexing and searching capabilities. By default, it offers high scalability, allowing you to index and retrieve text data efficiently. To get started with Lucene, you should have a solid understanding of how indexing works and the basics of full-text search.
1. Poorly Designed Index Structure
The Problem
One of the most common pitfalls is failing to design an appropriate index structure. This can affect the performance drastically. A flat index may lead to ineffective searches, while an overly complex one can become hard to maintain.
The Solution
When designing your index, consider what data types and fields you'll need. Lucene uses a schema-less structure, which means that you can add fields dynamically. Still, planning your schema in advance can save time and resources later on.
Example code:
import org.apache.lucene.document.Document;
import org.apache.lucene.document.Field;
import org.apache.lucene.document.StringField;
import org.apache.lucene.document.TextField;
import org.apache.lucene.index.IndexWriter;
import org.apache.lucene.index.IndexWriterConfig;
import org.apache.lucene.store.Directory;
import org.apache.lucene.store.RAMDirectory;
public void indexDocument(IndexWriter writer, String title, String content) throws Exception {
Document doc = new Document();
// Use StringField for non-analyzed data like IDs
doc.add(new StringField("title", title, Field.Store.YES));
// Use TextField for analyzed content to allow full-text search
doc.add(new TextField("content", content, Field.Store.YES));
// Add the document to the writer
writer.addDocument(doc);
}
Why: Using StringField for non-analyzed fields (like titles) ensures that the data isn't tokenized, while TextField allows for full-text searching. This design significantly improves search accuracy and performance.
2. Ignoring Query Optimization
The Problem
After indexing your documents, it might be tempting to jump straight into writing queries. However, inefficient queries can lead to slow search responses, especially when dealing with large datasets.
The Solution
Understand the various queries that Lucene supports. Use Boolean queries judiciously and take advantage of scoring models to refine search results.
Example code:
import org.apache.lucene.queryparser.classic.QueryParser;
import org.apache.lucene.search.BooleanClause;
import org.apache.lucene.search.BooleanQuery;
import org.apache.lucene.search.Query;
public Query buildQuery(String titleQuery, String contentQuery) throws Exception {
QueryParser titleParser = new QueryParser("title", analyzer);
Query title = titleParser.parse(titleQuery);
QueryParser contentParser = new QueryParser("content", analyzer);
Query content = contentParser.parse(contentQuery);
// Combine title and content queries
BooleanQuery.Builder booleanQuery = new BooleanQuery.Builder();
booleanQuery.add(title, BooleanClause.Occur.SHOULD);
booleanQuery.add(content, BooleanClause.Occur.SHOULD);
return booleanQuery.build();
}
Why: Using a BooleanQuery
with SHOULD
clauses allows for a more flexible and targeted search. This structure can significantly improve relevance and accuracy when searching across multiple fields.
3. Lack of Proper Analyzers
The Problem
Lucene provides different analyzers to preprocess and tokenize your text. Many developers use the default analyzer without considering their dataset, which can lead to missed search opportunities.
The Solution
Choose an analyzer that fits your data best. For example, the StandardAnalyzer
is good for general purposes, whereas WhitespaceAnalyzer
might be beneficial for certain conditions.
Example code:
import org.apache.lucene.analysis.Analyzer;
import org.apache.lucene.analysis.standard.StandardAnalyzer;
public void createIndex() throws Exception {
Analyzer analyzer = new StandardAnalyzer(); // Choose appropriate analyzer
IndexWriterConfig config = new IndexWriterConfig(analyzer);
Directory index = new RAMDirectory();
IndexWriter writer = new IndexWriter(index, config);
// Now you can index documents here ...
writer.close();
}
Why: Understanding which analyzer to use allows you to tailor the tokenization process to your data, improving search performance and relevance.
4. Neglecting to Monitor Index Size
The Problem
Lucene indices can grow large over time, especially if documents are frequently added, deleted, or updated. Ignoring index size can lead to sluggish search performance and higher memory consumption.
The Solution
Implement periodic index optimization and monitor your index size regularly. Lucene has built-in methods for optimizing the indices.
Example code:
public void optimizeIndex(IndexWriter writer) throws Exception {
// Optimize the index to improve search performance
writer.forceMerge(1);
}
Why: The forceMerge
method consolidates segments in the index to improve search speed by reducing the overhead of managing multiple segments. It's a crucial step in long-term maintenance.
5. Insufficient Error Handling
The Problem
Errors will inevitably occur during the integration of any external library. Neglecting to handle these errors can lead to application crashes or, worse, inconsistent search results.
The Solution
Incorporate robust error handling using Java's exception mechanism. Always anticipate potential issues, especially when dealing with file I/O or querying.
Example code:
try {
IndexWriter writer = createWriter();
indexDocument(writer, "Test Title", "Sample content for indexing.");
writer.close();
} catch (IOException e) {
// Log and handle the error -- essential for maintaining robustness
logger.error("Indexing failure", e);
}
Why: Logging exceptions not only helps in identifying issues but also aids in debugging. Good error handling practices lead to more resilient applications.
Bringing It All Together
Integrating Apache Lucene into your Java application can dramatically enhance your search functionalities. However, the common pitfalls discussed above can hinder your progress if not addressed.
By carefully designing your index structure, optimizing your queries, selecting appropriate analyzers, monitoring index sizes, and implementing robust error handling techniques, you can significantly mitigate these issues.
For further reading on Lucene, check out the official Apache Lucene documentation. This valuable resource can help you deepen your understanding and mastery of Lucene.
Happy coding, and may your applications benefit from the powerful search capabilities that Lucene offers!
Checkout our other articles