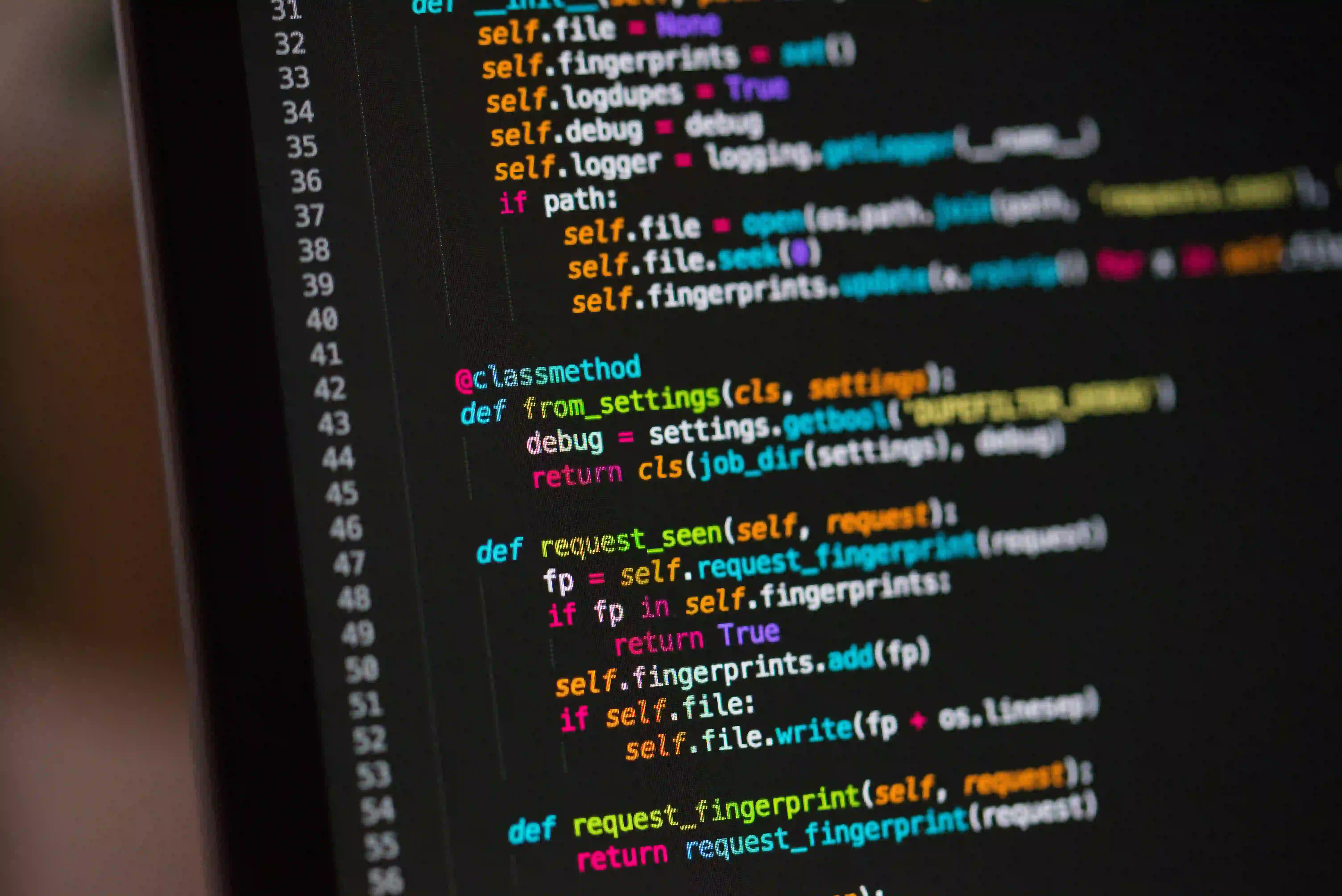
Battling WebSocket Vulnerabilities: A Security Guide
The rise of real-time web applications has led to a significant increase in the use of WebSockets. But with this growth comes the responsibility of securing these connections. In this post, we'll explore the potential vulnerabilities associated with WebSockets, practical strategies for mitigating those risks, and a few best coding practices.
Understanding WebSockets
WebSockets provide a full-duplex communication channel over a single, long-lived connection. This is ideal for applications requiring low latency and real-time updates, such as online gaming, chat applications, or live notifications. However, their persistence and continuous nature can introduce unique security challenges.
The Benefits of WebSockets
Before diving into vulnerabilities, let’s briefly highlight the benefits of using WebSockets:
- Real-time Communication: WebSockets enable instantaneous two-way communication between the client and server.
- Reduced Overhead: Unlike traditional HTTP requests, WebSocket connections minimize the data overhead, improving performance.
- Lifecycle Management: WebSockets maintain a persistent connection, which can facilitate faster message delivery.
For a deeper understanding of WebSocket communication, check out the WebSocket API documentation.
Common Vulnerabilities in WebSockets
While they offer numerous advantages, WebSockets can also expose your applications to several vulnerabilities:
1. Man-in-the-Middle (MitM) Attacks
If WebSocket connections are not secured, malicious actors can intercept and manipulate the messages being transmitted between clients and servers. This is known as a MitM attack.
Mitigation Strategy: Use Secure WebSocket connections (wss://) to encrypt the data being sent and received. This prevents unauthorized parties from viewing or manipulating the data in transit.
// Example of setting up a secure WebSocket connection in Java
import javax.websocket.ClientEndpoint;
import javax.websocket.OnMessage;
import javax.websocket.Session;
import javax.websocket.ContainerProvider;
import javax.websocket.WebSocketContainer;
// Create a secure connection to the WebSocket server
@ClientEndpoint
public class SecureWSClient {
private void connect() {
WebSocketContainer container = ContainerProvider.getWebSocketContainer();
try {
Session session = container.connectToServer(this, URI.create("wss://your-secure-websocket-server"));
// Proceed with messaging
} catch (Exception e) {
e.printStackTrace();
}
}
@OnMessage
public void onMessage(String message) {
System.out.println("Received: " + message);
}
}
2. Cross-Site WebSocket Hijacking (CSWSH)
CSWSH occurs when an attacker tricks a user's browser into making WebSocket connections to a server, which can compromise session tokens and sensitive data.
Mitigation Strategy: Implement proper origin checking in your WebSocket server. Only allow connections from trusted domains.
import javax.websocket.server.ServerEndpoint;
@ServerEndpoint(value = "/websocket", configurator = MyConfigurator.class)
public class MyWebSocketServer {
@OnOpen
public void onOpen(Session session) {
String origin = session.getRequestParameterMap().get("Origin").get(0);
if (!isOriginAllowed(origin)) {
throw new SecurityException("Origin is not allowed: " + origin);
}
// Proceed with connection
}
private boolean isOriginAllowed(String origin) {
// Check against a whitelist of origins
return "https://trusteddomain.com".equals(origin);
}
}
3. Message Injection Attacks
An attacker may send specially crafted WebSocket messages to exploit vulnerabilities in the application's message handling logic.
Mitigation Strategy: Validate all incoming WebSocket messages and handle unexpected formats gracefully. Employing rigorous input sanitization can help prevent such attacks.
@OnMessage
public void onMessage(String message, Session session) {
if (!isValidMessage(message)) {
// Log the attack attempt
System.out.println("Invalid message format: " + message);
return; // Discard the message
}
// Process the valid message
processMessage(message);
}
private boolean isValidMessage(String message) {
// Implement validation logic
return message != null && message.length() < 1000; // Example validation
}
Best Practices for WebSocket Security
Adopting security measures requires an understanding of both your technology stack and your specific application requirements. Here are best practices you should consider:
1. Use HTTPS and Secure WebSockets
Always use HTTPS for your web applications and wss:// for WebSocket connections. This ensures that data exchanged over the network is encrypted.
2. Implement Authentication and Authorization
Establish a robust authentication mechanism before allowing a user to establish a WebSocket connection. Token-based authentication (e.g., JWTs) can be effective.
3. Monitor and Log WebSocket Activity
Set up logging and monitoring to detect suspicious activities, such as unexpected connection attempts or unusual amounts of data being transmitted.
4. Limit WebSocket Access
Restrict access to WebSocket services by utilizing measures such as IP whitelisting or implementing rate limiting to safeguard against abusive behavior.
5. Regular Security Audits
Conduct regular security audits and penetration testing on your WebSocket implementations to ensure you can identify and mitigate new vulnerabilities.
Closing the Chapter
WebSockets are powerful tools for creating interactive and real-time web applications. However, with great power comes great responsibility. Understanding the various vulnerabilities and how to prevent them is essential for building secure applications.
By implementing best security practices, leveraging robust frameworks, and staying up-to-date with the latest security trends, developers can effectively safeguard their WebSocket connections.
For more detailed information on WebSocket security, you can read the OWASP WebSocket Security Cheat Sheet. This resource provides an extensive overview of potential risks and mitigation strategies.
Stay vigilant, stay secure! Your users deserve it.