Overcoming Challenges in Extensible Java Application Design
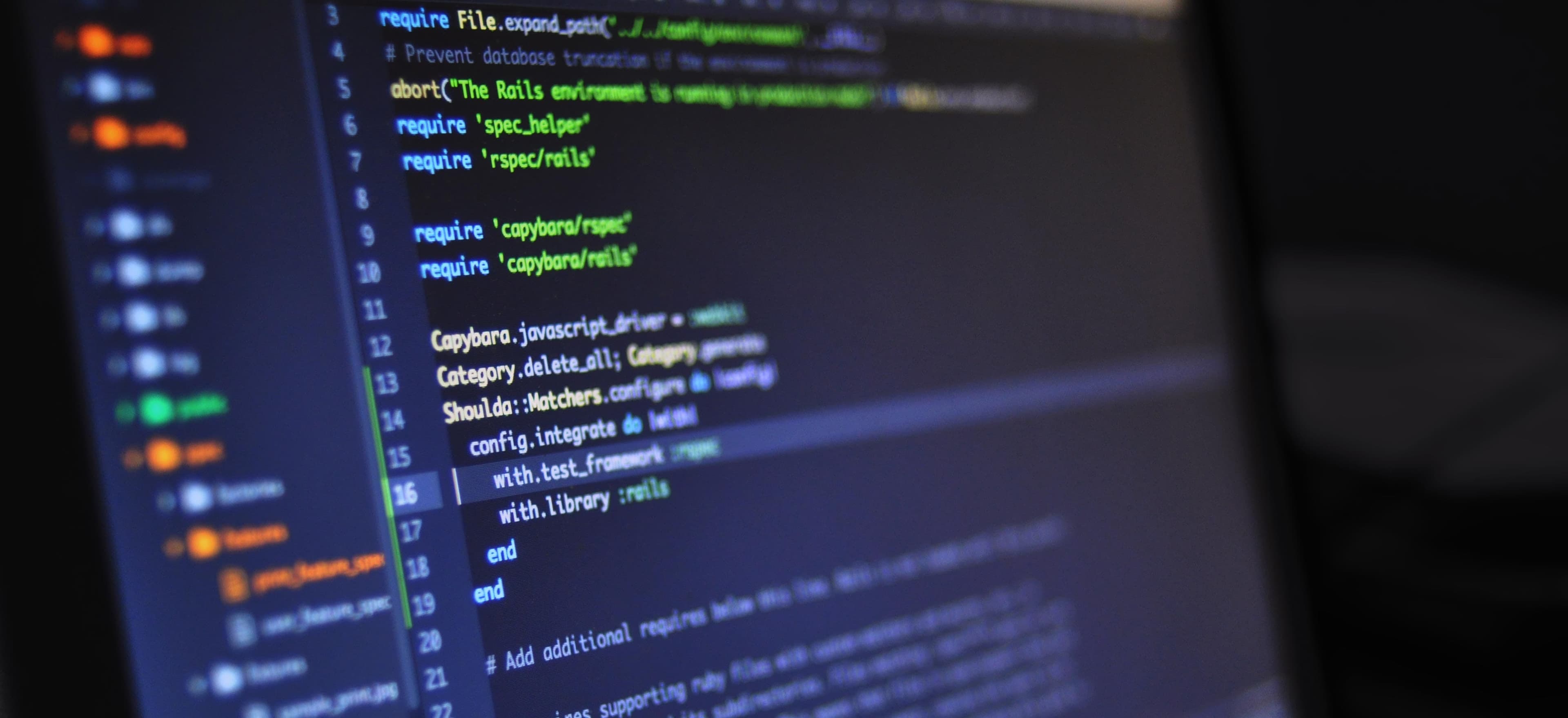
- Published on
Overcoming Challenges in Extensible Java Application Design
When developing Java applications, creating extensible systems is a priority that many developers strive to achieve. Extensibility ensures that your application can grow and adapt without requiring massive overhauls. In this blog post, we'll explore the challenges in extensible Java application design, provide actionable solutions, and present code snippets that highlight best practices.
What is Extensibility?
Extensibility refers to the capability of a software application to accommodate future growth. This may include adding new features, improving existing functionalities, or adapting to new requirements without losing integrity. In the context of Java applications, extensibility is crucial as it reduces long-term maintenance costs and improves user satisfaction.
Key Challenges in Designing Extensible Java Applications
- Tightly Coupled Architecture
- Inflexible Design Patterns
- Lack of Clear Interfaces
- Difficulties in Implementing Changes
- Insufficient Documentation
Let's delve into each challenge while discussing some solutions and strategies to overcome them.
Tightly Coupled Architecture
Tightly coupled systems make it difficult to change or extend components without affecting others. This can hinder future development.
Solution: Use Dependency Injection
By adopting Dependency Injection (DI), we can create loosely coupled components. This approach allows classes to be developed or changed independently.
// Interface for payment processing
public interface PaymentProcessor {
void processPayment(double amount);
}
// Implementation of PayPal payment processor
public class PayPalProcessor implements PaymentProcessor {
public void processPayment(double amount) {
System.out.println("Processing payment of " + amount + " through PayPal.");
}
}
// Implementation of CreditCard payment processor
public class CreditCardProcessor implements PaymentProcessor {
public void processPayment(double amount) {
System.out.println("Processing payment of " + amount + " through CreditCard.");
}
}
// Client class
public class PaymentService {
private PaymentProcessor paymentProcessor;
// Constructor for DI
public PaymentService(PaymentProcessor paymentProcessor) {
this.paymentProcessor = paymentProcessor;
}
public void makePayment(double amount) {
paymentProcessor.processPayment(amount);
}
}
// Main class to demo
public class Main {
public static void main(String[] args) {
PaymentProcessor processor = new PayPalProcessor();
PaymentService service = new PaymentService(processor);
service.makePayment(100.0);
}
}
Commentary
- Interfaces: The use of the
PaymentProcessor
interface allows for multiple implementations of payment processing. - Dependency Injection: The
PaymentService
class receives itsPaymentProcessor
as a dependency, making it easy to switch between different payment methods. - Loose Coupling: By doing this, you can easily add new payment processors without modifying the
PaymentService
.
For further reading on Dependency Injection, check out the Google Guice documentation.
Inflexible Design Patterns
Using the wrong design pattern can lead to rigid code that is resistant to change.
Solution: Strategize Design Patterns
Choose design patterns that promote flexibility. For example, the Strategy Pattern allows a family of algorithms, encapsulates each one, and makes them interchangeable.
// Context class
public class FileCompressor {
private CompressionStrategy strategy;
public FileCompressor(CompressionStrategy strategy) {
this.strategy = strategy;
}
public void compress(String fileName) {
strategy.compressFile(fileName);
}
}
// Interface for compression strategy
public interface CompressionStrategy {
void compressFile(String fileName);
}
// Implementation of ZIP compression
public class ZipCompressionStrategy implements CompressionStrategy {
public void compressFile(String fileName) {
System.out.println("Compressing " + fileName + " using ZIP.");
}
}
// Implementation of RAR compression
public class RarCompressionStrategy implements CompressionStrategy {
public void compressFile(String fileName) {
System.out.println("Compressing " + fileName + " using RAR.");
}
}
// Main class to demo
public class Main {
public static void main(String[] args) {
CompressionStrategy zipStrategy = new ZipCompressionStrategy();
FileCompressor fileCompressor = new FileCompressor(zipStrategy);
fileCompressor.compress("example.txt");
}
}
Commentary
- Strategy Pattern: This pattern enables the selection of different compression strategies at runtime, making the code adaptable and extensible.
- Extensibility: New compression strategies can be added without altering existing code.
For additional insights into design patterns, refer to the classic Gang of Four Design Patterns.
Lack of Clear Interfaces
Poorly defined interfaces can create confusion and hinder extensibility.
Solution: Define Clear and Concise Interfaces
Well-defined interfaces should be specific and only expose necessary methods.
// Car interface
public interface Car {
void start();
void stop();
}
// Implementation of ElectricCar
public class ElectricCar implements Car {
public void start() {
System.out.println("Electric car starting silently.");
}
public void stop() {
System.out.println("Electric car stopping.");
}
}
Commentary
- Clarity: The
Car
interface is straightforward, outlining exactly what a car can do. - Implementation Flexibility: New car types can be easily added without modifying existing code.
Clear interface design helps maintain a clean codebase, making future extensions more manageable.
Difficulties in Implementing Changes
Changes to code can be cumbersome when the structure is not adaptable.
Solution: Apply SOLID Principles
By following the SOLID principles (Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, Dependency Inversion), developers can create clean, maintainable code.
Example of the Single Responsibility Principle
public class Report {
public void generateReport() {
// Logic to generate the report
}
}
public class ReportPrinter {
public void print(Report report) {
// Logic to print the report
}
}
Commentary
- Separation of Concerns: The
Report
class is only responsible for generating reports, while theReportPrinter
handles printing. - Maintenance Ease: If you need to change the printing mechanism, it can be done in isolation.
For a deeper understanding of SOLID principles, visit SOLID Principles of Object-Oriented Design.
Insufficient Documentation
Bespoke solutions with little to no documentation can quickly become a nightmare for future developers.
Solution: Implement Effective Documentation Practices
- Comment Code: Briefly explain complex code segments.
- Maintain README files: Provide a high-level overview of the project, installation instructions, and common issues.
Example of Commented Code
// Generates a report and saves it
public class ReportGenerator {
// Method to create and save report
public void generate(String data) {
// Data processing logic.
}
}
Commentary
- Contextual Comments: Comments provide context to other developers, easing the onboarding process.
- Increased Maintainability: Documenting methods and classes enhances longevity and adaptability.
Conclusion
Building extensible Java applications presents various challenges, from tightly coupled architecture to documentation issues. By implementing techniques like Dependency Injection, clear interfaces, and the SOLID principles, you can overcome these challenges effectively. Ensuring your project is adaptable aids not only in future enhancements but also maintains overall code quality.
If you're looking to get started or dive deeper into extensible system design, resources like the Java Design Patterns guide can be helpful.
In summary, strive for modular, clear, and well-documented code. The resulting extensibility will not only improve the standing of your application today but also its viability in the future. Happy coding!