“Mastering Sliding Menus: Common Animation Pitfalls!”
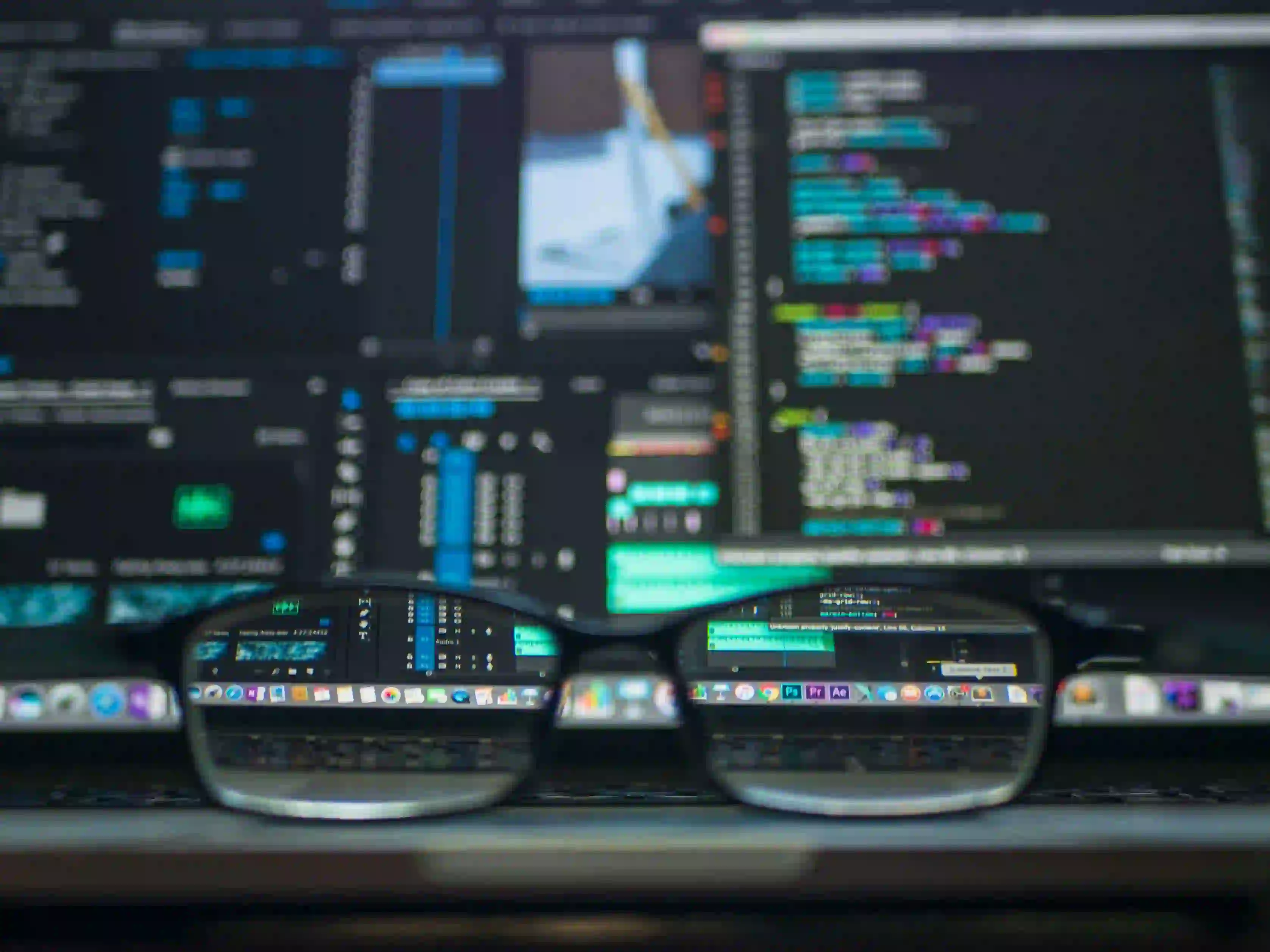
Mastering Sliding Menus: Common Animation Pitfalls
Sliding menus have become a staple in web and mobile development. When implemented effectively, they can enhance user experience by providing a seamless way to navigate applications. However, developers often encounter common pitfalls when animating these menus that can detract from their original intent. In this blog post, we'll explore these pitfalls, how to avoid them, and provide useful code snippets to better understand the nuances of sliding menu animations.
Understanding Sliding Menus
Before diving deep into the pitfalls, let’s define what a sliding menu is. A sliding menu, typically accessed with a swipe gesture or a tap, overlays content or slides in from an edge of the screen. It's an efficient way to hide secondary options, keeping the interface clean while allowing access on demand.
Why Use Sliding Menus?
- Space Efficiency: They help preserve valuable screen space.
- User Interaction: Sliding menus facilitate intuitive user interactions, particularly on mobile devices.
- Focus Control: Users can focus on the main content without distractions.
Common Pitfalls in Animation
-
Overcomplicating the Animation
Developers sometimes feel the need to make animations overly complex using different easing functions, rotations, or scale effects. While interesting, complex animations can confuse users. A natural movement, such as a simple slide-in effect, enhances usability.
Here’s a simple sliding animation implemented in Java:
☕snippet.javaimport javax.swing.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; public class SlidingMenuExample { private JFrame frame; private JPanel slidingMenu; private JButton toggleButton; public SlidingMenuExample() { frame = new JFrame("Sliding Menu Example"); slidingMenu = new JPanel(); toggleButton = new JButton("Toggle Menu"); // Setting initial position of sliding menu slidingMenu.setBounds(-200, 0, 200, 400); slidingMenu.setBackground(java.awt.Color.GRAY); frame.setSize(600, 400); frame.setLayout(null); frame.add(slidingMenu); frame.add(toggleButton); toggleButton.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { toggleMenu(); } }); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); } private void toggleMenu() { int targetX = slidingMenu.getX() == 0 ? -200 : 0; slidingMenu.setLocation(targetX, slidingMenu.getY()); } public static void main(String[] args) { new SlidingMenuExample(); } }
In this code, the sliding menu moves between two positions with a simple action. This straightforward transition keeps it user-friendly, gaining focus without overwhelming effects.
-
Ignoring Accessibility
Animations can create issues for users with certain disabilities. Fast-moving elements might not give enough time for users to react. This inconsideration leads to a poor experience and could violate accessibility guidelines.
To address this, ensure that animations can be paused or turned off. A good practice is to provide an option in the application's settings.
- Neglecting Performance
Animations using heavy graphics or excessive frame rates might slow down the application, particularly on lower-end devices. Optimize animations to maintain the app's performance.
Consider using lightweight libraries, such as Android's ViewPropertyAnimator for smooth transitions.
For example:
slidingMenu.animate()
.translationX(targetX)
.setDuration(250)
.start();
This method ensures that the animation runs efficiently, making use of the device's hardware acceleration.
- Inconsistent Animation Feedback
Users appreciate consistent feedback when they interact with an application. If the sliding menu behaves differently on different triggers (swipe vs. button press), it can confuse users.
Always standardize how the menu opens. For instance, if made clear that a swipe opens it, ensure that the button press does so in the same manner.
How to Enhance Your Sliding Menus
Use Easing Functions Wisely
Easing functions can smooth out animations, making them feel more natural. It's often best to keep it simple with an ease-out effect. This creates an impression of weight and helps the menu settle nicely into place.
Example:
slidingMenu.animate()
.translationX(targetX)
.setInterpolator(new AccelerateDecelerateInterpolator())
.setDuration(250)
.start();
Effective Duration and Delay
Short animations (around 250-300ms) keep the experience snappy. Avoid delays unless you want to call attention to something special. For sliding menus, instant user feedback is paramount.
Lessons Learned
Sliding menus can enhance navigation within an application, but only if implemented correctly. As developers, it's essential to avoid common pitfalls such as overcomplicated animations, neglecting accessibility, performance issues, and inconsistent behavior.
By prioritizing simplicity, performance, and user feedback, you can create a polished and engaging experience. For further reading on CSS transitions and animations that enhance your web applications, check out CSS Tricks and MDN Web Docs.
Master the art of sliding menus, and deliver intuitive navigation that not only impresses but also respects your users’ needs. Embrace the challenge, test thoroughly, and enjoy the process of perfecting your animations!