Boost Membership Checks: The Power of Bloom Filters in Java
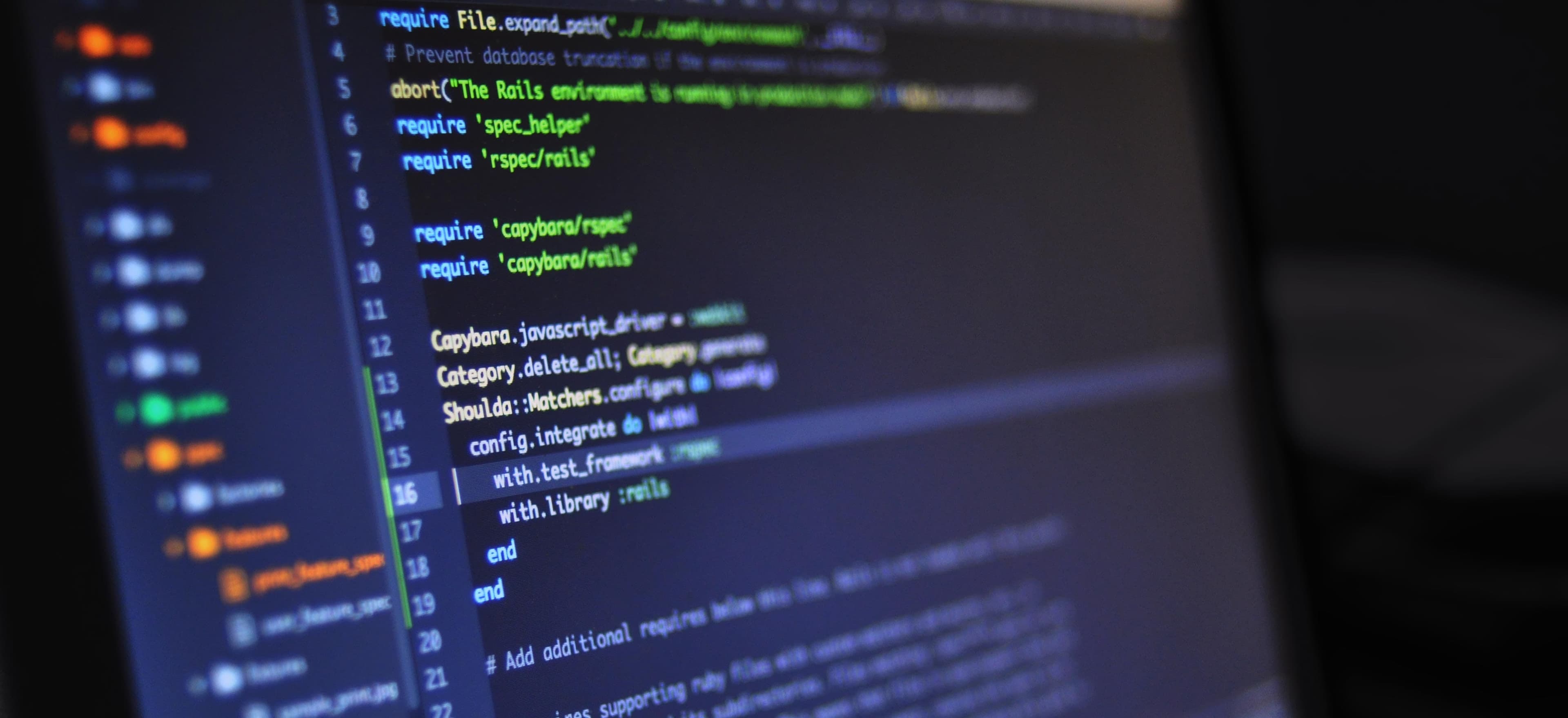
- Published on
Boost Membership Checks: The Power of Bloom Filters in Java
In the world of software development, efficient membership checking can have a significant impact on application performance and user experience. This is particularly true in systems where large sets of data need to be queried frequently—think of databases, caching mechanisms, and even recommendation systems. In this post, we’ll explore the power of Bloom Filters, an efficient probabilistic data structure that can significantly enhance membership checks.
What is a Bloom Filter?
A Bloom Filter is a space-efficient probabilistic data structure that is used to determine if an element is a member of a set. The key characteristics of a Bloom Filter are:
- Probabilistic Nature: It can definitively tell if an element is not in the set but can only probabilistically tell if an element is in the set.
- False Positives: It may produce false positives, meaning it can mistakenly indicate that an element is in the set. However, it will never produce false negatives.
Advantages of Bloom Filters
- Space Efficiency: Bloom Filters require significantly less space than storing the actual elements in a set.
- Speed: They allow for fast membership checks, which is invaluable for large datasets.
- Scalability: With minimal performance overhead, Bloom Filters can scale up efficiently with increasing data.
Where Are Bloom Filters Used?
Bloom Filters have been widely adopted in various tech stacks for purposes such as:
- Web Caching: To check whether a page exists in a cache before fetching it from the backend.
- Database Applications: In systems like Apache Cassandra and Google Bigtable, where they optimize disk lookups.
- Distributed Systems: Ensuring that data is not duplicated across nodes.
To learn more about the theoretical foundations of Bloom Filters, visit this link.
Implementing a Bloom Filter in Java
Let’s dive into the implementation details. We'll create a simple Bloom Filter in Java that supports standard operations like adding an element, checking membership, and providing a basic initialization.
Step 1: Required Libraries
We'll use Java's built-in arrays along with the Hashing
utilities found in the java.security
package to simulate multiple hash functions. If you want to implement a more sophisticated solution, consider using libraries such as Guava which has built-in support for Bloom Filters.
Step 2: Basic Bloom Filter Structure
Here's a basic implementation of a Bloom Filter:
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.BitSet;
public class BloomFilter {
private BitSet bitSet;
private int size;
private int hashCount;
public BloomFilter(int size, int hashCount) {
this.size = size;
this.hashCount = hashCount;
this.bitSet = new BitSet(size);
}
}
Why Use BitSet?
The BitSet
class allows us to create a dynamic array of bits. Each bit can represent the presence or absence of an item. This makes BitSet
an ideal choice for our Bloom Filter since it minimizes memory overhead.
Step 3: Hash Function
To demonstrate how we create hashed values for our elements, let’s implement a hashing function.
private int[] getHashes(String value) {
int[] hashes = new int[hashCount];
try {
MessageDigest md = MessageDigest.getInstance("MD5");
byte[] digest = md.digest(value.getBytes());
for (int i = 0; i < hashCount; i++) {
hashes[i] = Math.abs(digest[i % digest.length] % size);
}
} catch (NoSuchAlgorithmException e) {
throw new RuntimeException(e);
}
return hashes;
}
Commentary on Hash Functions
Using a hashing algorithm like MD5, we can generate multiple hash values for the same string. The getHashes
method produces an array of integer hash values, allowing us to set multiple bits in the BitSet
. The % size
operation ensures our indices remain within the bounds of valid bit positions.
Step 4: Adding Elements
Now let’s implement the method to add elements to our Bloom Filter.
public void add(String value) {
for (int hash : getHashes(value)) {
bitSet.set(hash);
}
}
Step 5: Checking Membership
The most critical aspect of a Bloom Filter is checking whether an element is in the set.
public boolean mightContain(String value) {
for (int hash : getHashes(value)) {
if (!bitSet.get(hash)) {
return false; // Element is definitely not in the set
}
}
return true; // Element might be in the set
}
Why the Membership Check is Efficient
This method iterates over the hash values and checks the corresponding bits in our BitSet
. If any bit is not set, we can conclude that the element is not in the set, which allows for a fast membership check with minimal computational overhead.
Putting It All Together
Here’s the complete Bloom Filter implementation:
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.BitSet;
public class BloomFilter {
private BitSet bitSet;
private int size;
private int hashCount;
public BloomFilter(int size, int hashCount) {
this.size = size;
this.hashCount = hashCount;
this.bitSet = new BitSet(size);
}
private int[] getHashes(String value) {
int[] hashes = new int[hashCount];
try {
MessageDigest md = MessageDigest.getInstance("MD5");
byte[] digest = md.digest(value.getBytes());
for (int i = 0; i < hashCount; i++) {
hashes[i] = Math.abs(digest[i % digest.length] % size);
}
} catch (NoSuchAlgorithmException e) {
throw new RuntimeException(e);
}
return hashes;
}
public void add(String value) {
for (int hash : getHashes(value)) {
bitSet.set(hash);
}
}
public boolean mightContain(String value) {
for (int hash : getHashes(value)) {
if (!bitSet.get(hash)) {
return false;
}
}
return true;
}
public static void main(String[] args) {
BloomFilter bloomFilter = new BloomFilter(100, 5);
bloomFilter.add("test@example.com");
System.out.println(bloomFilter.mightContain("test@example.com")); // true
System.out.println(bloomFilter.mightContain("unknown@example.com")); // false
}
}
Lessons Learned
Bloom Filters serve as an incredible tool for optimizing membership checks in your Java applications. By leveraging their space efficiency and fast membership checks, you can enhance performance significantly, especially when handling large datasets.
While Bloom Filters may introduce false positives, their advantages often outweigh this downside for many applications. Furthermore, extensions to the basic Bloom Filter, such as Counting Bloom Filters, can even help manage deletions at the cost of additional complexity.
To read more about the various implementations and use cases of Bloom Filters, check out this article.
Incorporating Bloom Filters into your application could be the key to improved performance. So why not give it a try? Happy coding!