Mastering Java 8 Optional: Avoiding Null Pointer Exceptions
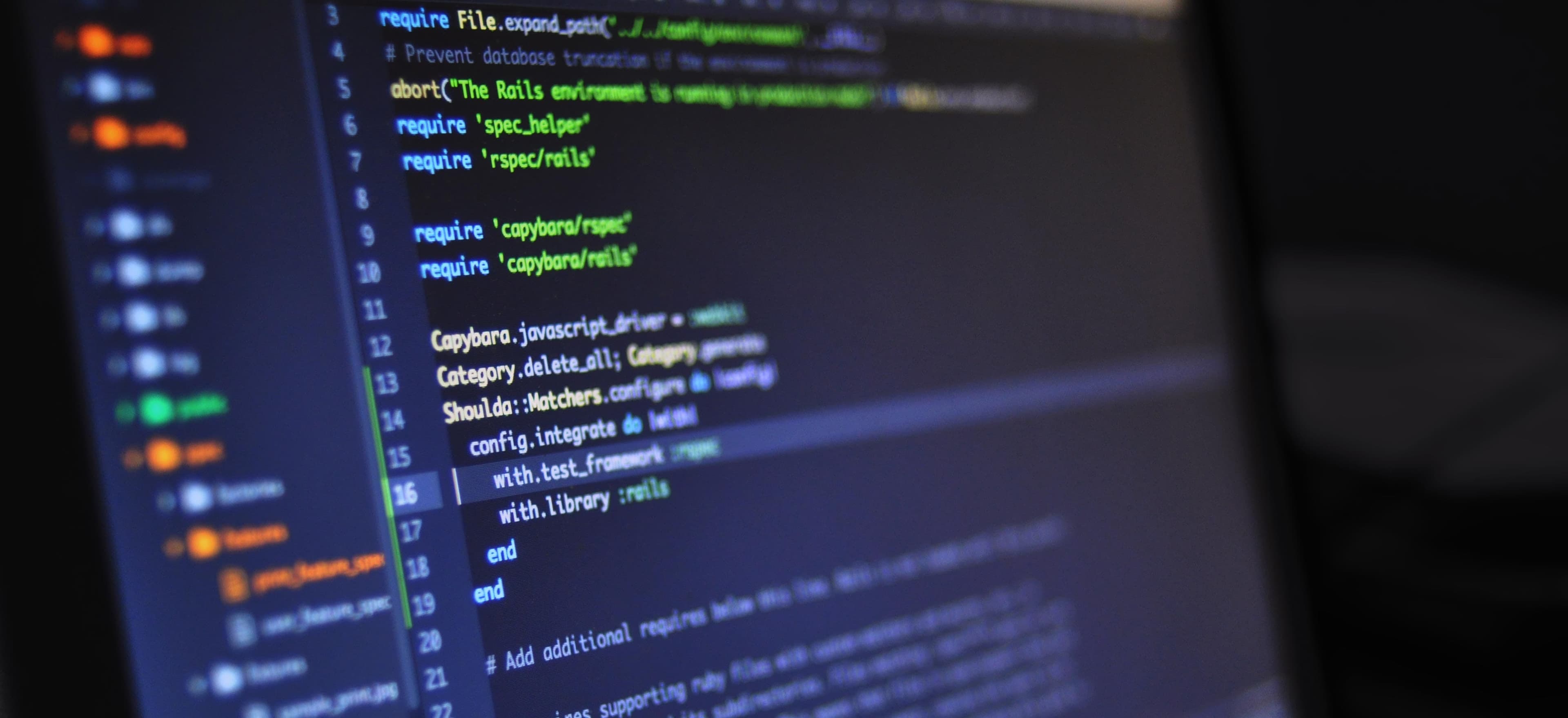
- Published on
Mastering Java 8 Optional: Avoiding Null Pointer Exceptions
Java is a powerful programming language that has built up a devoted following over the years. However, one of its most notorious issues is the infamous Null Pointer Exception (NPE). In Java 8, a game-changing solution emerged: the Optional
class. This class allows developers to express the absence of a value in a more expressive and robust way. In this blog post, we'll dive deep into Optional
, explore how to use it effectively, and demonstrate its potential to help you avoid Null Pointer Exceptions in your Java applications.
Table of Contents
- Understanding Null Pointer Exceptions
- Introducing Optional
- Creating Optional Instances
- Accessing Values Safely
- Chaining Operations with Optional
- Common Use Cases for Optional
- Best Practices and Pitfalls
- Conclusion
Understanding Null Pointer Exceptions
A Null Pointer Exception occurs when a program attempts to use an object reference that has not been initialized. For example:
String name = null;
int length = name.length(); // Throws Null Pointer Exception
The frustration of encountering NPEs is universal among developers. To prevent these issues, Java 8 introduced Optional
, a container that may or may not hold a value.
Introducing Optional
The Optional
class is a part of the java.util
package and is designed to represent a value that may or may not be present. It provides a way to refactor code and avoid the explicit use of null references. Consider the following example:
Optional<String> optionalName = Optional.ofNullable(name);
In this snippet, optionalName
can now either contain a string, or it can be empty.
Key Benefits of Optional:
- Expressive Code:
Optional
communicates intent. When you see a method that returns anOptional
, it immediately tells you that the return value might be absent. - Chaining and Functional Programming: With
Optional
, you can chain operations, enabling a more functional programming style.
Creating Optional Instances
There are several ways to instantiate an Optional
:
1. Optional.of()
This method is used when you are sure the value is non-null.
Optional<String> optionalName = Optional.of("John Doe");
2. Optional.ofNullable()
This method can accept a null value without throwing an NPE.
String name = null;
Optional<String> optionalName = Optional.ofNullable(name);
3. Optional.empty()
Use this when you want to explicitly create an empty Optional
.
Optional<String> emptyOptional = Optional.empty();
Accessing Values Safely
Accessing the value of an Optional
is straightforward, but it has to be done carefully to avoid NPEs. Here are the primary methods for doing so:
1. isPresent()
This method checks if a value is present.
if (optionalName.isPresent()) {
System.out.println("Name: " + optionalName.get());
} else {
System.out.println("No name present.");
}
2. ifPresent()
This method allows executing a piece of code if a value is present, eliminating the need for an if statement:
optionalName.ifPresent(name -> System.out.println("Name: " + name));
3. orElse() and orElseGet()
These methods provide a fallback value. Use orElseGet()
to leverage supplier logic for generating a value conditionally.
String nameOrDefault = optionalName.orElse("Default Name");
4. orElseThrow()
Instead of returning a default value, you can throw an exception if the value is not present.
String name = optionalName.orElseThrow(() -> new NoSuchElementException("No value present"));
Chaining Operations with Optional
One of the standout features of Optional
is the ability to chain operations. The map
and flatMap
methods handle the transformation of values safely.
1. Using map()
The map()
method allows you to apply a function if the value is present.
Optional<String> upperCaseName = optionalName.map(String::toUpperCase);
2. Using flatMap()
If your transformation function returns another Optional
, you can use flatMap()
to avoid nested Optional
objects.
Optional<String> result = optionalName.flatMap(name -> Optional.of(name.toUpperCase()));
Common Use Cases for Optional
The Optional
class is well-suited for a variety of situations where nullability needs to be addressed:
- Return Values: Use
Optional
for methods that might not find a corresponding value, such as search functions.
public Optional<User> findUserById(Long id) {
return userRepository.findById(id);
}
- Method Arguments: While
Optional
is not favored as a method parameter type, it can still be used if necessary to indicate that an argument may not be provided.
Best Practices and Pitfalls
While Optional
simplifies handling nulls, here are a few best practices to avoid common pitfalls:
-
Avoid Using Optional in Fields: Using
Optional
as field types can lead to performance issues and convoluted code. Stick to using it as a return type. -
Don't Overuse Optional: Use
Optional
where it provides clear benefits, such as method return values, instead of throwing unnecessary complexity into your codebase. -
Use Descriptive Names: Ensure that your variables and methods provide context. For example,
findUserById
returningOptional<User>
makes the intent clear.
Final Thoughts
The introduction of Optional
in Java 8 has significantly transformed the way developers handle nullability, leading to cleaner, more expressive, and less error-prone code. By embracing Optional
, you can effectively combat Null Pointer Exceptions and create robust applications that are easier to maintain and understand.
Next time you find yourself facing a null reference, consider whether Optional
can enhance your design. With its functional-style operations and clear semantics, it might just be the solution you need to master your Java code and ensure reliability in your applications.
For further reading on Optional
, check out the official Java Documentation, and explore more about functional programming in Java.
By understanding and applying the principles of the Optional
class, you can enhance the robustness of your applications—so go ahead, master it!