Debugging JSTL View Issues in Spring Controllers
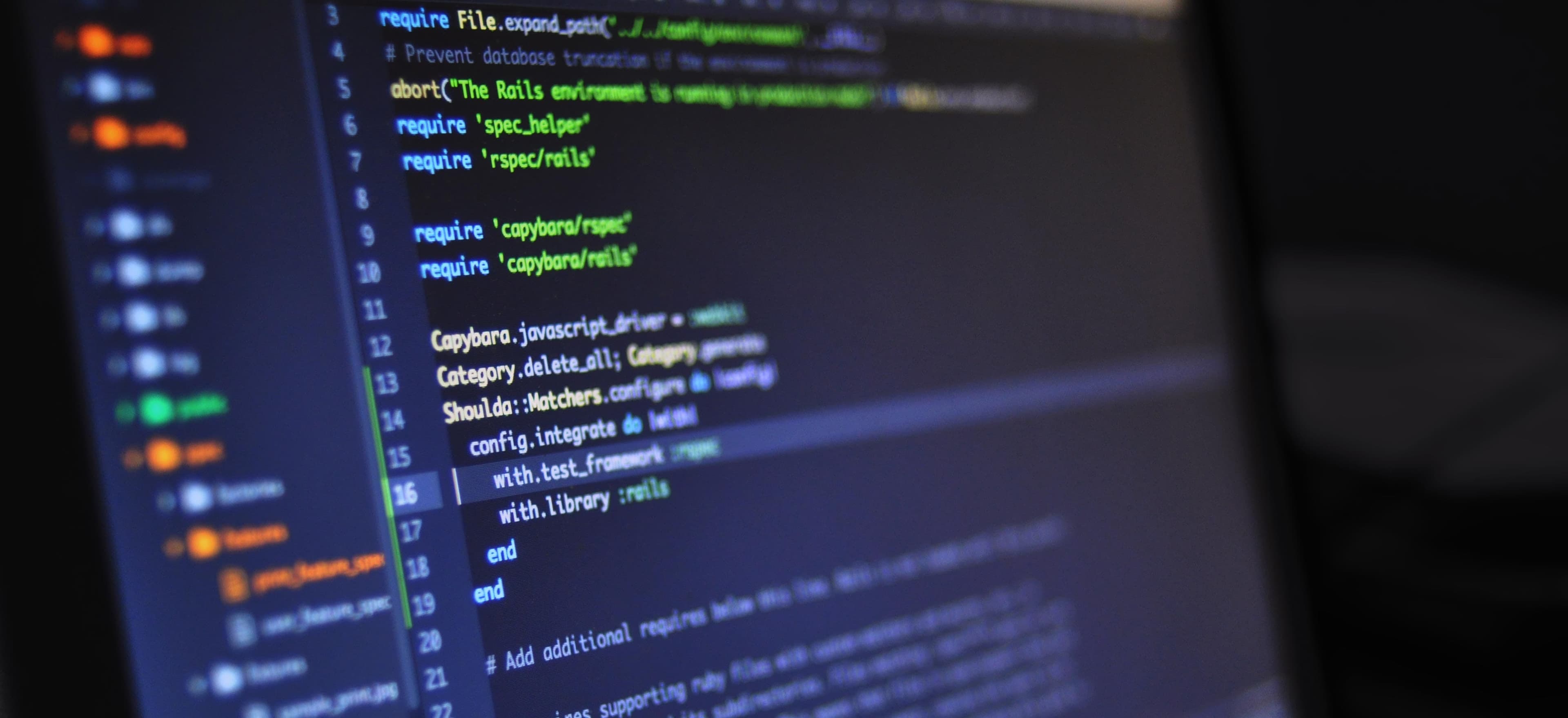
- Published on
Debugging JSTL View Issues in Spring Controllers
JavaServer Pages Standard Tag Library (JSTL) is a powerful toolkit for Java web applications, often used within Spring MVC. Many developers harness the talent of JSTL for rendering views efficiently. However, issues may crop up, creating a bump in the road for an otherwise smooth web application. This article will delve into common JSTL view issues encountered in Spring controllers, how to debug them, and best practices for effective resolution.
Understanding JSTL in Spring MVC
Before directly diving into debugging, let’s briefly discuss how JSTL integrates within Spring. Spring MVC uses the Model-View-Controller (MVC) architectural pattern where controllers handle HTTP requests, generate responses, and prepare the data to be rendered. JSTL acts in the view layer, allowing developers to create dynamic web pages with clean, readable code.
Common JSTL Tags
JSTL is known for its various tag libraries:
- Core Tags: for flow control (forEach, if, choose).
- Formatting Tags: for formatting dates and numbers.
- SQL Tags: for accessing databases (though use carefully for security reasons).
- XML Tags: for manipulating XML data.
Typical Issues with JSTL in Spring Controllers
As you develop your application, you may experience various issues involving JSTL and Spring controllers. Here are the most common ones:
- Tags Not Being Processed: This can result from missing tag libraries in your JSP files.
- EL (Expression Language) Errors: Errors when using EL syntax could indicate that the model attributes are not set correctly in the controller.
- View Resolvers Misconfiguration: If Spring is unable to find the view, it will struggle to render the JSTL tags properly.
Let's approach each of these issues methodically.
1. Tags Not Being Processed
The Issue
When JSTL tags are not processing, it may be due to missing the tag library declaration. If your JSP does not include the necessary library references, the tags will simply appear as plain text, causing confusion.
How to Fix
Ensure you have included the correct tag library declarations at the top of your JSP file. Here's an example:
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/fmt" prefix="fmt" %>
Why This Matters
Including the appropriate namespaces allows the JSP engine to recognize and process JSTL tags correctly. Without these declarations, your application cannot understand that it needs to interpret those tags, ultimately leading to incorrect or non-functional outputs.
2. EL Errors
The Issue
Expression Language is crucial for accessing data within your JSP. Errors may crop up if you try to evaluate attributes that do not exist in your model. For example:
<p>${user.name}</p>
If the user
object is not added to the model in your Spring controller, or if it does not have a name
field, this will generate an error.
How to Fix
In your Spring controller method, make sure you are model-binding correctly. For example:
@Controller
public class UserController {
@GetMapping("/user")
public String getUser(Model model) {
User user = userService.findUser();
model.addAttribute("user", user);
return "user";
}
}
Why This Matters
Ensuring that the model is populated correctly is essential for the page to display the intended data. EL errors indicate a breakdown in communication between your model and the view, signalling a need for thorough debugging.
3. View Resolvers Misconfiguration
The Issue
Sometimes, the view resolver may not be correctly configured to locate your JSP files. This could result in a 404 Not Found
error when accessing the view.
How to Check
Ensure that your Spring configuration file (like application.properties
) is set up correctly. For example, you may have something like this:
spring.mvc.view.prefix=/WEB-INF/views/
spring.mvc.view.suffix=.jsp
Why This Matters
Proper view resolver configuration ensures that Spring knows where to find your views. If configured incorrectly, it can lead to rendering issues where JSTL cannot be processed. Therefore, double-checking these settings is often the first step in resolving view-related issues.
Debugging Steps
- Check JSTL Tag Libraries: Confirm that you have imported the JSTL correctly.
- Inspect Your Controller: Ensure that the model is correctly populated and that attribute names match what you expect in your JSP.
- Look for Errors in Logs: Examine server logs for stack traces or errors that may give you clues on what went wrong.
- Debugging Tools: Use debugging tools such as breakpoints in your IDE to step through your controller code.
- Perform Unit Testing: Write unit tests for your controller methods to ensure they behave as expected.
Best Practices for Using JSTL with Spring MVC
- Declare Libraries Once: Avoid repetitive tag library declarations by declaring them in a shared layout or using a master JSP file.
- Keep Logic Minimal: Keep business logic out of your views; JSTL should be used mainly for rendering and simple control flow.
- Use Custom Tags Sparingly: While JSTL can simplify many aspects of JSP pages, don't overuse it; leverage Spring’s capabilities to handle complex logic or processing.
Final Considerations
JSTL can significantly enhance the way your Spring MVC applications deliver dynamic content. However, like anything else, it requires diligence to use effectively. By understanding common issues, taking proactive debugging steps, and adhering to best practices, you can ensure a smoother development process when integrating JSTL within your Spring controllers.
For more on the nuances of JSTL and Spring integration, check out the detailed Spring MVC documentation and the insightful JSTL guide provided by Oracle.
By staying informed and prepared, we can build clean, efficient, and robust web applications that meet user needs while minimizing frustrations from bugs and errors. Happy coding!
Checkout our other articles