Struggling with Android Image Caching? Here's the Fix!
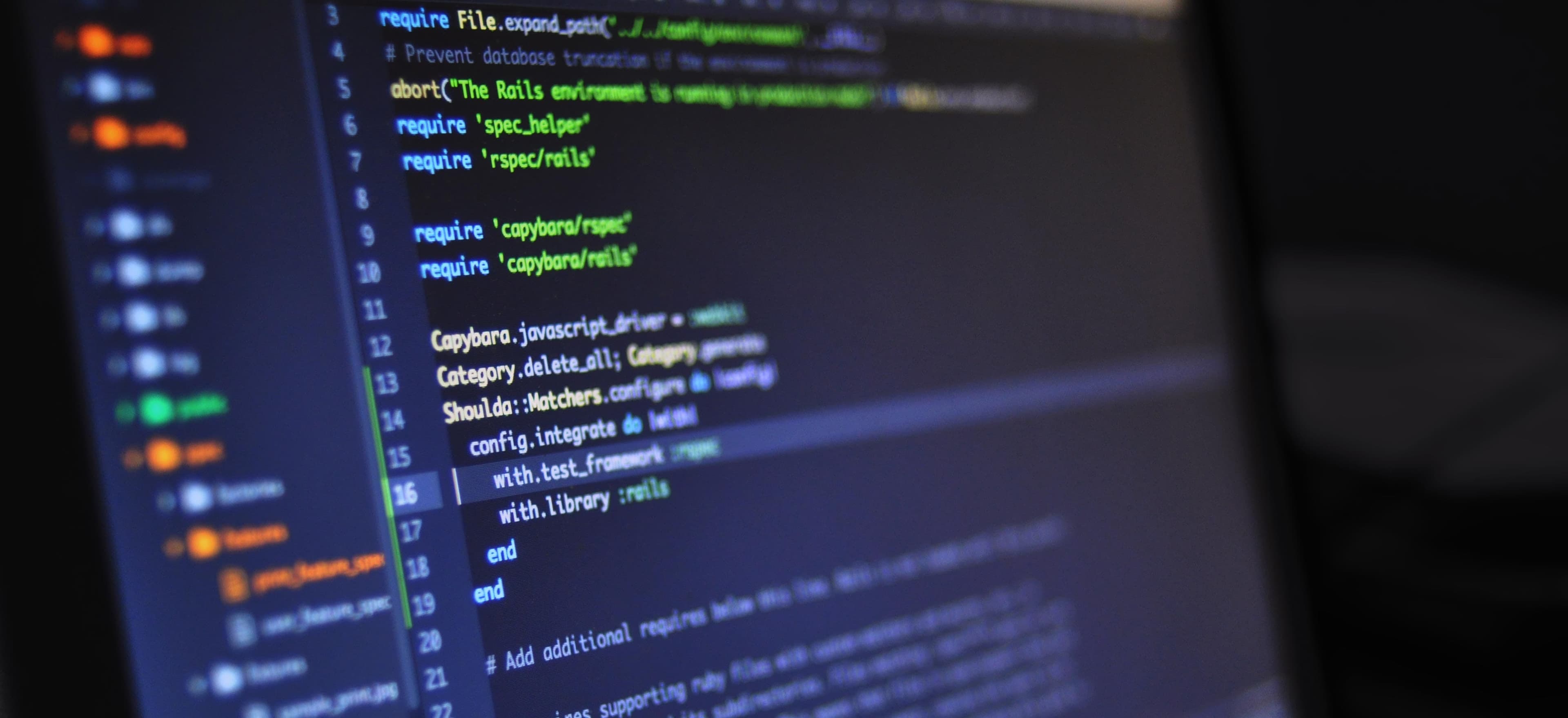
- Published on
Struggling with Android Image Caching? Here's the Fix!
Image caching is an essential aspect of mobile application development, especially for Android apps that require loading and displaying images efficiently. In this blog post, we will explore why image caching is vital, how to implement it effectively, and the best practices to enhance performance.
Why Image Caching is Important
When working with images in your Android app, performance is critical. Loading images directly from a server every time can lead to:
- Increased latency: Users have to wait longer to see images.
- Excessive data usage: Hitting the network repeatedly consumes the user’s data plan.
- Poor user experience: Stuttering or lagging interfaces frustrate users.
Image caching helps alleviate these issues by storing images locally so that they can be retrieved more quickly, reducing load times and improving overall application performance.
Understanding Image Caching Mechanisms
Image caching can be handled in two main ways:
-
Memory Caching: Images are cached in memory for quick access. This is fast, but limited by the amount of available memory.
-
Disk Caching: Images are stored on disk. This is slower but allows for greater storage capacity and persistence across app restarts.
Both methods have their place, and a balanced approach using both types of caching is often the best strategy.
Libraries for Image Caching
While you can write your own caching logic, leveraging existing libraries can save you time and ensure optimized performance. Two popular libraries for image loading and caching in Android are Glide and Picasso. Here's a quick comparative breakdown:
- Glide: Best for handling large, complex images with less memory pressure.
- Picasso: Simple to use, ideal for straightforward image loading tasks.
Implementing Image Caching with Glide
Let’s take a look at how to implement image caching using Glide—a powerful library with an uncomplicated API.
Step 1: Add Glide Dependency
First, you need to add the Glide dependency to your build.gradle
file:
dependencies {
implementation 'com.github.bumptech.glide:glide:4.12.0'
annotationProcessor 'com.github.bumptech.glide:compiler:4.12.0'
}
Step 2: Load Images with Glide
Loading images using Glide is straightforward. Here’s a simple example of loading an image from a URL into an ImageView
:
Glide.with(context)
.load("https://example.com/image.jpg") // URL of the image
.placeholder(R.drawable.placeholder) // Placeholder image while loading
.error(R.drawable.error_image) // Image to show on error
.into(imageView); // Target ImageView
Why Use These Options?
placeholder(R.drawable.placeholder)
: Displays a temporary image while your target image is being downloaded.error(R.drawable.error_image)
: Provides feedback in case the image fails to load, improving the user experience.
Customizing Glide Caching Strategy
Glide allows customization for caching strategies. You can set both memory and disk strategies:
Glide.with(context)
.load("https://example.com/image.jpg")
.diskCacheStrategy(DiskCacheStrategy.ALL) // Cache both the original and transformed images
.skipMemoryCache(false) // Use memory cache
.into(imageView);
Disk Caching Strategy Types
- DiskCacheStrategy.ALL: Caches the original image and all transformation results.
- DiskCacheStrategy.NONE: Disables disk caching.
- DiskCacheStrategy.DATA: Caches the original image only.
- DiskCacheStrategy.RESOURCE: Caches only the transformed image.
Using the right caching strategy can significantly enhance performance.
Implementing Image Caching with Picasso
If you choose to use Picasso, the implementation is similarly easy.
Step 1: Add Picasso Dependency
Include the following line in your build.gradle
file:
dependencies {
implementation 'com.squareup.picasso:picasso:2.71828'
}
Step 2: Load Images
To load an image with Picasso, the code is straightforward:
Picasso.get()
.load("https://example.com/image.jpg") // URL of the image
.placeholder(R.drawable.placeholder) // Placeholder image while loading
.error(R.drawable.error_image) // Image to show on error
.into(imageView); // Target ImageView
Advantages of Using Caching Libraries
Implementing caching via libraries like Glide or Picasso offers numerous advantages:
- Simplicity: Easy to integrate and use with minimal setup.
- Memory Management: Libraries manage memory for you.
- Performance Optimization: Built-in optimizations help enhance image loading times.
Best Practices for Image Caching
Here are some best practices to keep in mind while implementing image caching in your Android applications:
-
Use Efficient Caching Libraries: Choose libraries like Glide or Picasso that are well-optimized for Android.
-
Choose the Right Caching Strategy: Assess your application needs to choose an appropriate caching strategy.
-
Monitor Memory Usage: Regularly monitor your app's memory usage to ensure it is within acceptable limits.
-
Implement Bitmap Reuse: When using
Bitmap
objects, consider reusing instances to save memory and processing time. -
Handle Low Memory Situations: Implement logic to clear caches when the system memory is low. This helps maintain app performance.
-
Test on Various Devices: Test your implementation on different devices and Android versions to ensure consistency.
Final Thoughts
Implementing image caching is paramount for a smooth user experience in Android applications. Libraries like Glide and Picasso make it seamless to manage your images, minimizing load times and reducing data usage. By leveraging these tools and following the outlined best practices, you can enhance your app's performance and ensure users have a satisfying experience.
For more in-depth guidance, refer to Glide's official documentation and Picasso's official documentation. Happy coding!
Checkout our other articles