Common SSL Configuration Mistakes in Play Framework
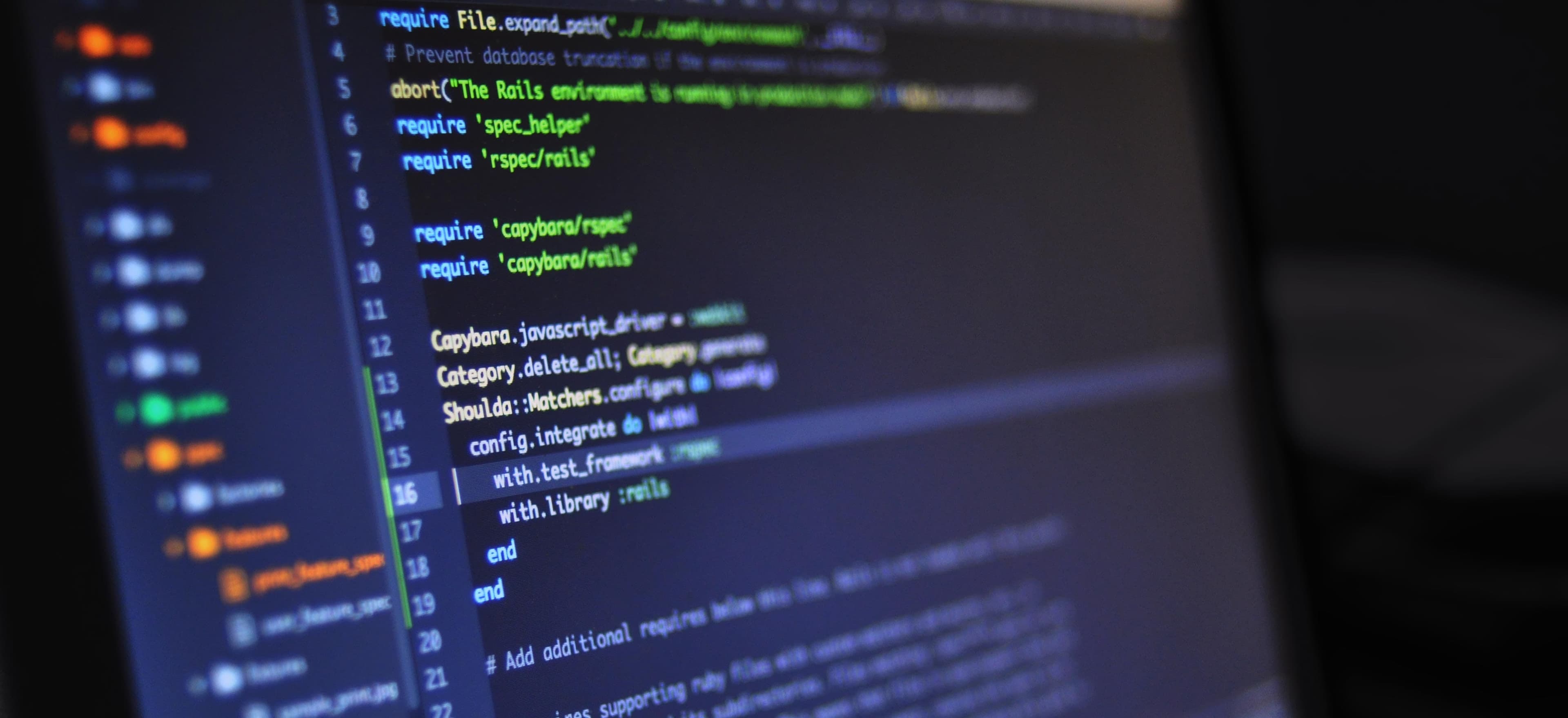
- Published on
Common SSL Configuration Mistakes in Play Framework
As developers, we understand that securing an application is not just about writing robust code; it's equally about implementing the right protocols and configurations. Secure Sockets Layer (SSL) is critical for any web application today, especially those built with the Play Framework. However, several common mistakes can jeopardize the intended level of security. In this post, we will identify and explain these mistakes to help you secure your Play Framework applications effectively.
Understanding SSL in Play Framework
SSL (or TLS, its successor) encrypts the data sent between clients and servers, ensuring that sensitive information remains confidential. Play Framework, built on Scala, provides an easy way to set up SSL. However, improper configuration can lead to vulnerabilities that adversaries can exploit.
Key Reasons to Use SSL
- Data Encryption: Protects sensitive data.
- Data Integrity: Ensures the data has not been altered.
- Authentication: Verifies the identity of users and servers.
- SEO Benefits: Google has indicated that HTTPS is a ranking factor.
Before diving into the common SSL mistakes, let's look at how to implement SSL in your Play Framework application effectively.
Implementing SSL in Play Framework
To enable SSL in your Play Framework application, you need to modify the application.conf
file and set up an HTTPS connector. Below is a sample configuration:
play.server.https.port = 9443
play.server.https.keyStore.path = "conf/keystore.jks"
play.server.https.keyStore.password = "your_keystore_password"
play.server.https.keyStore.type = "JKS"
Why This Matters: By properly setting up your keystore and SSL port, you ensure that your application can securely handle requests over HTTPS.
Common SSL Configuration Mistakes
1. Using Self-Signed Certificates in Production
Many developers opt to use self-signed certificates for ease of development. While these certificates may be acceptable in a development environment, relying on them in production can expose users to risks.
Solution: Always use certificates issued by trusted Certificate Authorities (CAs) in production. Services like Let's Encrypt can provide SSL certificates for free.
2. Neglecting to Configure HTTP Strict Transport Security (HSTS)
HSTS is a security feature that forces browsers to interact with your server only over HTTPS. Many developers forget to set this up, leaving their applications vulnerable to downgrade attacks.
How to Implement HSTS: Add the following header in your Play application:
import play.api.mvc._
class SecurityHeaders extends BaseController {
def addHSTSHeader(action: Action[AnyContent]): Action[AnyContent] = {
action.customHeaders(Seq("Strict-Transport-Security" -> "max-age=31536000; includeSubDomains"))
}
}
Why This Matters: The HSTS header tells browsers to use only HTTPS for the specified duration, helping to prevent attacks.
3. Not Redirecting HTTP to HTTPS
It's common to set up an HTTPS port without redirecting HTTP traffic. This can lead to users accidentally accessing the insecure HTTP version of the site.
Solution: Implement a redirect from HTTP to HTTPS in your Play application. You can achieve this using the following code.
def redirectHttpToHttps: Action[AnyContent] = Action { request =>
Redirect("https://" + request.host + request.uri, status = 301)
}
Why This Matters: Redirecting users ensures they always access the secure version of your application.
4. Ignoring Expiry Dates for SSL Certificates
Many developers fail to monitor the expiry date of their SSL certificates. Expired certificates lead to browser warnings and can result in loss of user trust.
Solution: Regularly check the expiry dates of your SSL certificates and set reminders for renewal. Services like SSL Labs can help you monitor your certificates.
5. Weak Cipher Suites
The choice of cipher suites can directly affect the security of your SSL connection. Utilizing outdated or weak ciphers can expose sensitive data to attackers.
To configure cipher suites in Play Framework, modify the following settings in application.conf
:
play.server.https.ciphers = ["TLS_ECDHE_RSA_WITH_AES_256_GCM_SHA384", "TLS_ECDHE_RSA_WITH_AES_128_GCM_SHA256"]
Why This Matters: Strong cipher suites ensure robust encryption, safeguarding the data that moves between your clients and server.
6. Failure to Test SSL Configuration
Assuming that your SSL configuration is correct after setting it up can lead to vulnerabilities. Regular testing is essential.
Solution: Make use of tools like Qualys SSL Labs and OpenSSL to perform tests on your SSL configurations. These tools will provide insights into your SSL health, including cipher support and configuration errors.
7. Misconfiguring the Keystore Path
One of the frequent mistakes developers make is misconfiguring the path to the keystore. An incorrect path renders SSL non-functional.
Solution: Make sure to double-check your application.conf
paths and test connectivity. If your application is deployed in a container, ensure the keystore is correctly included in your Docker image or VM environment.
Best Practices for SSL Configuration in Play Framework
- Use automated certificate renewal: Set up automated processes to renew certificates to avoid manual oversight.
- Implement logging: Keep an audit log of SSL certificate updates and configurations.
- Stay updated: Regularly look out for security advisories related to your SSL configuration. Follow updates from organizations such as the Internet Engineering Task Force (IETF).
My Closing Thoughts on the Matter
Implementing SSL in a Play Framework application is essential for ensuring a secure user experience. Be mindful of the common mistakes outlined in this post to safeguard your application effectively. By adopting best practices and maintaining vigilance, you will not only protect user data but also foster trust in your web application.
For more detailed insights into securing web applications, consider exploring resources such as OWASP's Documentation on HTTPS or Mozilla's SSL Configuration Generator.
Don't overlook the power of SSL. Let's take every step towards building secure applications today!