Troubleshooting GlassFish Deployment Issues in Eclipse Juno
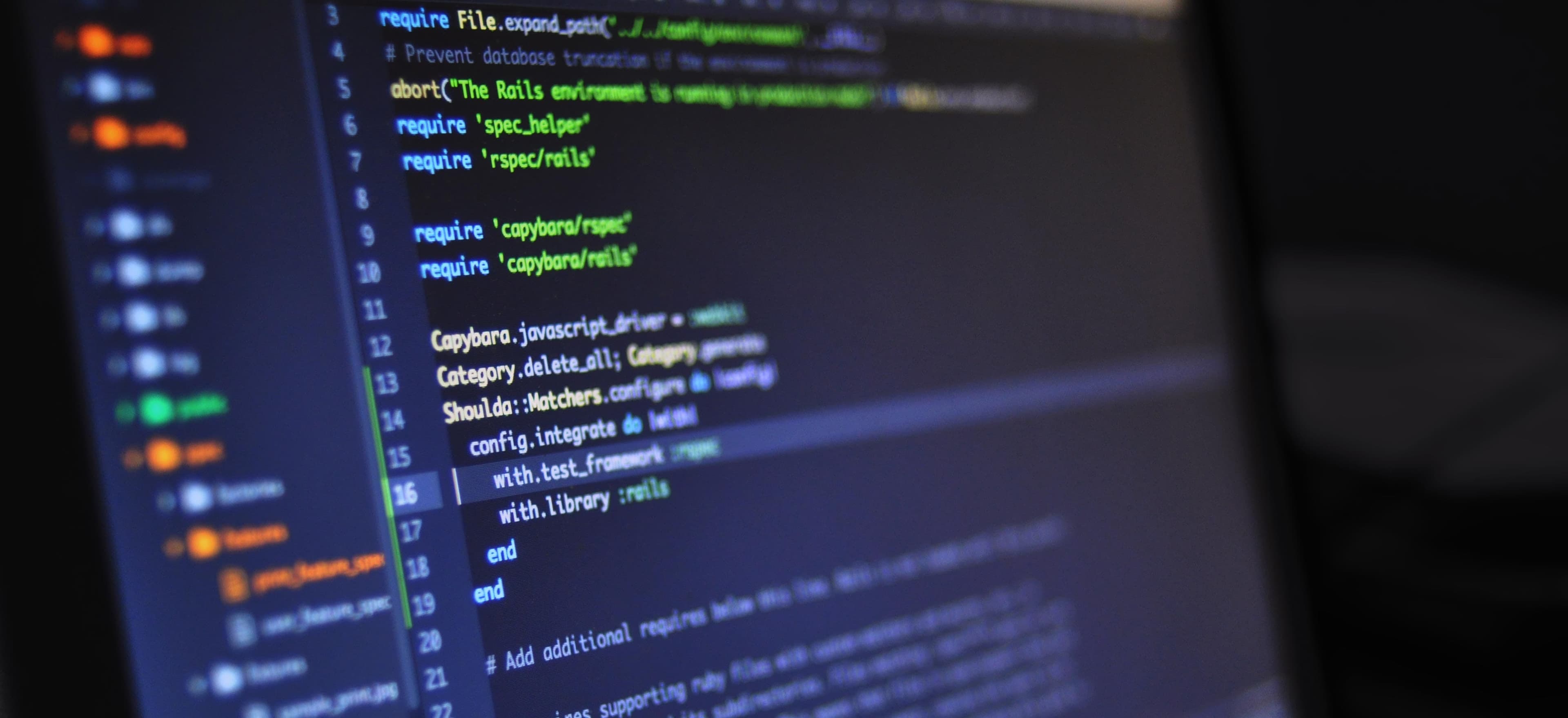
- Published on
Troubleshooting GlassFish Deployment Issues in Eclipse Juno
Deploying Java applications can often be a straightforward procedure, but when you're working with GlassFish in Eclipse Juno, you may encounter several issues. In this blog post, we’ll delve into common deployment problems, their potential causes, and how to troubleshoot them effectively. To make this more engaging, we will blend elaborate discussions with concise technical insights.
Understanding GlassFish and Eclipse Juno
Before dissecting deployment issues, let’s understand the roles GlassFish and Eclipse play in your Java development workflow.
GlassFish is an open-source application server for the Java EE platform, managing server-side applications in a robust and scalable way. On the other hand, Eclipse Juno is an Integrated Development Environment (IDE) that simplifies Java application development, offering tools for building, debugging, and testing applications.
However, the integration between GlassFish and Eclipse may not always be seamless, leading to deployment challenges.
Common Deployment Issues
Issue 1: Server Not Starting
One of the most common issues developers face is the GlassFish server not starting within Eclipse. This can stem from various factors, including port conflicts and improper configurations.
Solutions:
- Check Logs: The first step in diagnosing the problem is to check the GlassFish server log files for error messages. You can find these logs in the GlassFish directory under
glassfish/domains/domain1/logs/server.log
. - Port Conflict: Make sure that the default ports (such as 8080 for HTTP and 4848 for the Admin Console) are not being used by other applications.
- Configuration Errors: Inspect your server configuration settings in Eclipse. Go to the Servers tab, right-click your GlassFish server, and select Open. Verify your configuration settings under the Overview tab.
Example of Starting the Server
// Sample to start GlassFish server programmatically
import com.sun.enterprise.glassfish.api.*;
import org.glassfish.api.*;
public class ServerStarter {
public static void main(String[] args) {
GlassFish glassfish = GlassFish.getInstance();
glassfish.start();
System.out.println("GlassFish Server started successfully.");
}
}
The start()
function initializes the server, and it also provides an opportunity to check for potential startup errors.
Issue 2: Deployment Descriptors
Another frequent hiccup occurs when deployment descriptors (like web.xml
) are misconfigured, leading to deployment failures.
Solutions:
- Validate XML: Make sure that your
web.xml
orglassfish-web.xml
files are valid XML. Look out for typos and structural errors. - Ensure Correct Deployment: Verify that the project is marked as a Dynamic Web Project and that the runtime environment is correctly set to GlassFish.
- Examine Output Directory: Ensure that the output directory (typically
bin
ortarget
) contains your compiled classes.
Example of a Simple web.xml
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd"
version="3.1">
<servlet>
<servlet-name>HelloWorld</servlet-name>
<servlet-class>com.example.HelloWorldServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>HelloWorld</servlet-name>
<url-pattern>/hello</url-pattern>
</servlet-mapping>
</web-app>
This web.xml
file configures a simple servlet and its URL mapping. If the deployment fails, ensure that the servlet class is present in the specified path.
Issue 3: Unsatisfied Dependencies
During deployment, your application might face issues if certain dependencies are missing. This can happen if you're using web frameworks or libraries that require specific configurations.
Solutions:
- Check Maven Dependencies: If you're using Maven, make sure all dependencies are correctly defined in your
pom.xml
. Perform a Maven clean and build to ensure all libraries are downloaded.
<dependency>
<groupId>com.example</groupId>
<artifactId>my-library</artifactId>
<version>1.0-SNAPSHOT</version>
</dependency>
- Classpath Configuration: Ensure that your project’s build path includes all necessary libraries. Right-click on your project > Build Path > Configure Build Path to adjust classpath settings.
Issue 4: Invalid Deployment Assembly
A misconfigured deployment assembly can lead to deployment failures, often indicating that Eclipse is not aware of where to find your output classes.
Solution:
- Modify Deployment Assembly: Right-click your project in Eclipse, go to Properties, and then select Deployment Assembly. Ensure your source folders and libraries are correctly configured for deployment.
Example Setup
Typically, you might add entries that look something like this:
- Source Folder:
/src
- Output Folder:
/bin
This tells Eclipse where to find your compiled resources.
Bonus Tips for Better Integration
-
Run as Administrator: Sometimes, GlassFish requires administrative rights to configure and run correctly. Make sure to open Eclipse with elevated privileges.
-
Use Administrator Console: Cross-check your changes using the GlassFish Admin Console which can be accessed through http://localhost:4848. This provides a great interface for examining server settings and application status.
-
Clean and Rebuild: Don’t overlook the potential power of a clean build. Right-click the project in Eclipse and select Clean…, then rebuild the project before deployment.
Reference Links
For further understanding and troubleshooting tips, you might find the following resources helpful:
- GlassFish Documentation - Comprehensive details on GlassFish and its configurations.
- Eclipse IDE Documentation - Valuable resources for troubleshooting and maximizing the use of Eclipse.
Closing Remarks
Deploying Java applications in Eclipse Juno with GlassFish can be challenging. However, armed with the right troubleshooting strategies, you can identify and resolve deployment issues effectively.
By initially analyzing server logs, validating deployment descriptors, ensuring all dependencies are met, and configuring the deployment assembly accurately, you can overcome many common pitfalls.
Feel free to engage with the comments section below with questions, or share your experiences. Happy coding!
Checkout our other articles