Mastering Java Threads: 5 Hidden Magic Tricks!
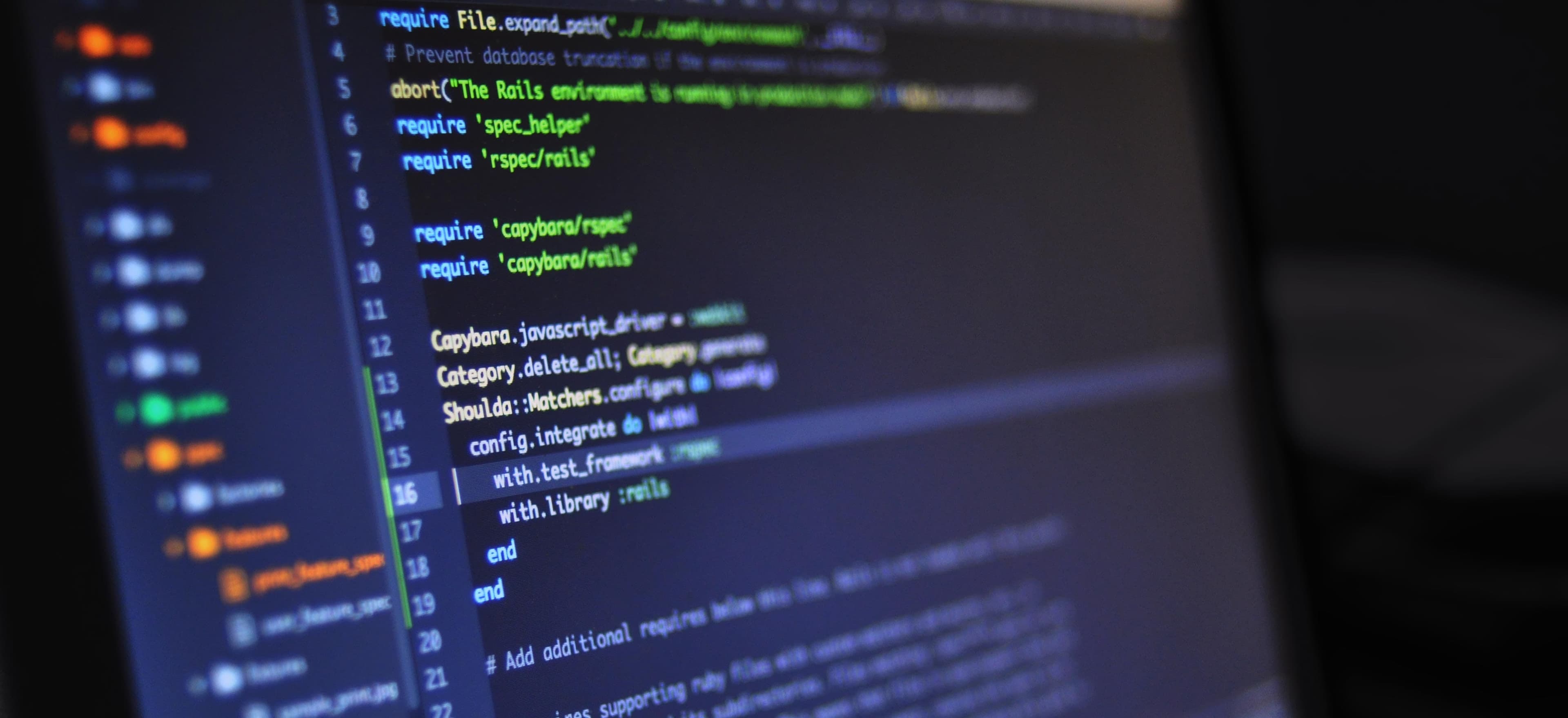
- Published on
Mastering Java Threads: 5 Hidden Magic Tricks!
Java, as one of the most widely used programming languages, has robust support for multithreading. Multithreading allows your program to perform multiple tasks concurrently, making it more efficient. In this blog post, we will explore five hidden tricks that can help you harness the power of Java threads effectively. Whether you're a beginner or an advanced user, these tips will enhance your understanding and control over threads in Java.
Table of Contents
- Understanding the Basics of Threads
- Trick 1: Using the ThreadPoolExecutor
- Trick 2: Callable and Future for Managing Results
- Trick 3: Thread Safety with Concurrent Collections
- Trick 4: The Power of Volatile Keyword
- Trick 5: Leveraging Fork/Join Framework
- Conclusion
Understanding the Basics of Threads
Before diving into the tricks, it’s essential to understand what threads are. In Java, a thread is a lightweight process that can run concurrently with other threads. The creation of threads can significantly optimize your applications, especially for I/O-bound tasks.
Java provides two primary ways to create threads:
- Extending the Thread class:
class MyThread extends Thread {
public void run() {
System.out.println("Thread is running");
}
}
This method allows you to override the run
method where the thread’s task is defined.
- Implementing the Runnable interface:
class MyRunnable implements Runnable {
public void run() {
System.out.println("Runnable is running");
}
}
The Runnable
approach is usually preferred for more complex applications because it separates the task from the thread itself.
Trick 1: Using the ThreadPoolExecutor
Why create a new thread every time when you can reuse existing ones? The ThreadPoolExecutor
provides a pool of threads that can be reused. This reduces resource consumption and improves performance.
import java.util.concurrent.Executors;
import java.util.concurrent.ExecutorService;
public class ThreadPoolExample {
public static void main(String[] args) {
ExecutorService executorService = Executors.newFixedThreadPool(3);
for (int i = 0; i < 10; i++) {
final int taskId = i;
executorService.submit(() -> {
System.out.println("Running task " + taskId);
});
}
executorService.shutdown();
}
}
Commentary
- Why use
ThreadPoolExecutor
? Creating new threads incurs significant overhead. The thread pool efficiently manages thread creation and allocation, leading to better performance, especially in applications with many short-lived tasks. - Flexible management: The pool size can be adjusted based on system resources and task requirements.
For further reading on this topic, check out Java's Concurrency in Practice.
Trick 2: Callable and Future for Managing Results
Unlike Runnable
, the Callable
interface can return results and throw exceptions. Combined with Future
, it allows you to manage and retrieve results from asynchronous computations.
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Executors;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Future;
public class CallableExample {
public static void main(String[] args) throws InterruptedException, ExecutionException {
ExecutorService executorService = Executors.newSingleThreadExecutor();
Callable<Integer> task = () -> {
Thread.sleep(1000);
return 123;
};
Future<Integer> future = executorService.submit(task);
// Do some other work here...
Integer result = future.get(); // This will block until the result is available
System.out.println("Result: " + result);
executorService.shutdown();
}
}
Commentary
- Blocking behavior: The
get()
method blocks until the task is complete. This is useful when you need to ensure that the task has finished before proceeding. - Error Management: Using
Callable
allows you to throw checked exceptions, which can be handled gracefully.
For more information on Callable
, check the Java API documentation.
Trick 3: Thread Safety with Concurrent Collections
You might have heard about the importance of thread safety in concurrent programming. Java's java.util.concurrent
package includes concurrent collections that are inherently thread-safe. Let's take a look at the ConcurrentHashMap
.
import java.util.concurrent.ConcurrentHashMap;
public class ConcurrentMapExample {
public static void main(String[] args) {
ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
Runnable task = () -> {
for (int i = 0; i < 1000; i++) {
map.put(Thread.currentThread().getName() + "-" + i, i);
}
};
Thread thread1 = new Thread(task);
Thread thread2 = new Thread(task);
thread1.start();
thread2.start();
try {
thread1.join();
thread2.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Map Size: " + map.size());
}
}
Commentary
- ConcurrentHashMap: It allows concurrent modifications without locking the entire map, which improves performance in high-concurrency situations.
- No need for manual synchronization: When using concurrent collections, you avoid the pitfalls of manual synchronization.
For a deep dive into concurrent collections, refer to Java Concurrency Tutorial.
Trick 4: The Power of Volatile Keyword
In Java, shared variables might be cached by multiple threads. The volatile
keyword ensures visibility of changes across threads but does not guarantee atomicity.
public class VolatileExample {
private static volatile boolean flag = false;
public static void main(String[] args) throws InterruptedException {
Thread writerThread = new Thread(() -> {
flag = true; // Write operation
});
Thread readerThread = new Thread(() -> {
while (!flag) {
// Busy-wait
}
System.out.println("Flag is true, exiting...");
});
readerThread.start();
Thread.sleep(1000); // Allow the reader to start first
writerThread.start();
writerThread.join();
readerThread.join();
}
}
Commentary
- Cache Coherency: The
volatile
keyword guarantees that when one thread changes the value of the variable, other threads will immediately see that change. - Avoiding accidental caching: Without
volatile
, a thread may cache the value, which could lead to inconsistencies.
For more on volatile
, see the Java Language Specification.
Trick 5: Leveraging Fork/Join Framework
Java's Fork/Join framework is designed to make parallelism easier and helps in splitting tasks into smaller tasks that can be processed concurrently.
import java.util.concurrent.RecursiveTask;
import java.util.concurrent.ForkJoinPool;
public class ForkJoinExample extends RecursiveTask<Long> {
private final long start;
private final long end;
public ForkJoinExample(long start, long end) {
this.start = start;
this.end = end;
}
@Override
protected Long compute() {
if (end - start <= 1000) { // threshold
return computeSequentially();
}
long mid = (start + end) / 2;
ForkJoinExample leftTask = new ForkJoinExample(start, mid);
ForkJoinExample rightTask = new ForkJoinExample(mid, end);
leftTask.fork(); // async
return rightTask.compute() + leftTask.join(); // sync
}
private Long computeSequentially() {
long sum = 0;
for (long i = start; i < end; i++) {
sum += i;
}
return sum;
}
public static void main(String[] args) {
ForkJoinPool pool = new ForkJoinPool();
ForkJoinExample task = new ForkJoinExample(1, 100000);
long result = pool.invoke(task);
System.out.println("Total Sum: " + result);
}
}
Commentary
- Parallelism: The Fork/Join framework does all the heavy lifting of managing threads, which simplifies parallel programming.
- Optimal performance: By breaking the problem into smaller pieces, the framework can utilize all available processor cores.
For deeper insights on the Fork/Join framework, look at the Java Concurrency documentation.
My Closing Thoughts on the Matter
Threads are a powerful feature of Java that can significantly improve the performance of your applications. By mastering these five hidden tricks, you'll not only enhance your technical skills but also write efficient, maintainable, and scalable code.
To summarize, remember to use:
- ThreadPoolExecutor for efficient task management.
- Callable and Future for handling results gracefully.
- Concurrent Collections for safe, thread-friendly data handling.
- Volatile for visibility control among threads.
- Fork/Join Framework for effective parallel processing.
Feel free to explore each topic deeper to uncover the full potential of Java's concurrency features. Happy coding!