Common Spring MVC Errors Beginners Make and How to Fix Them
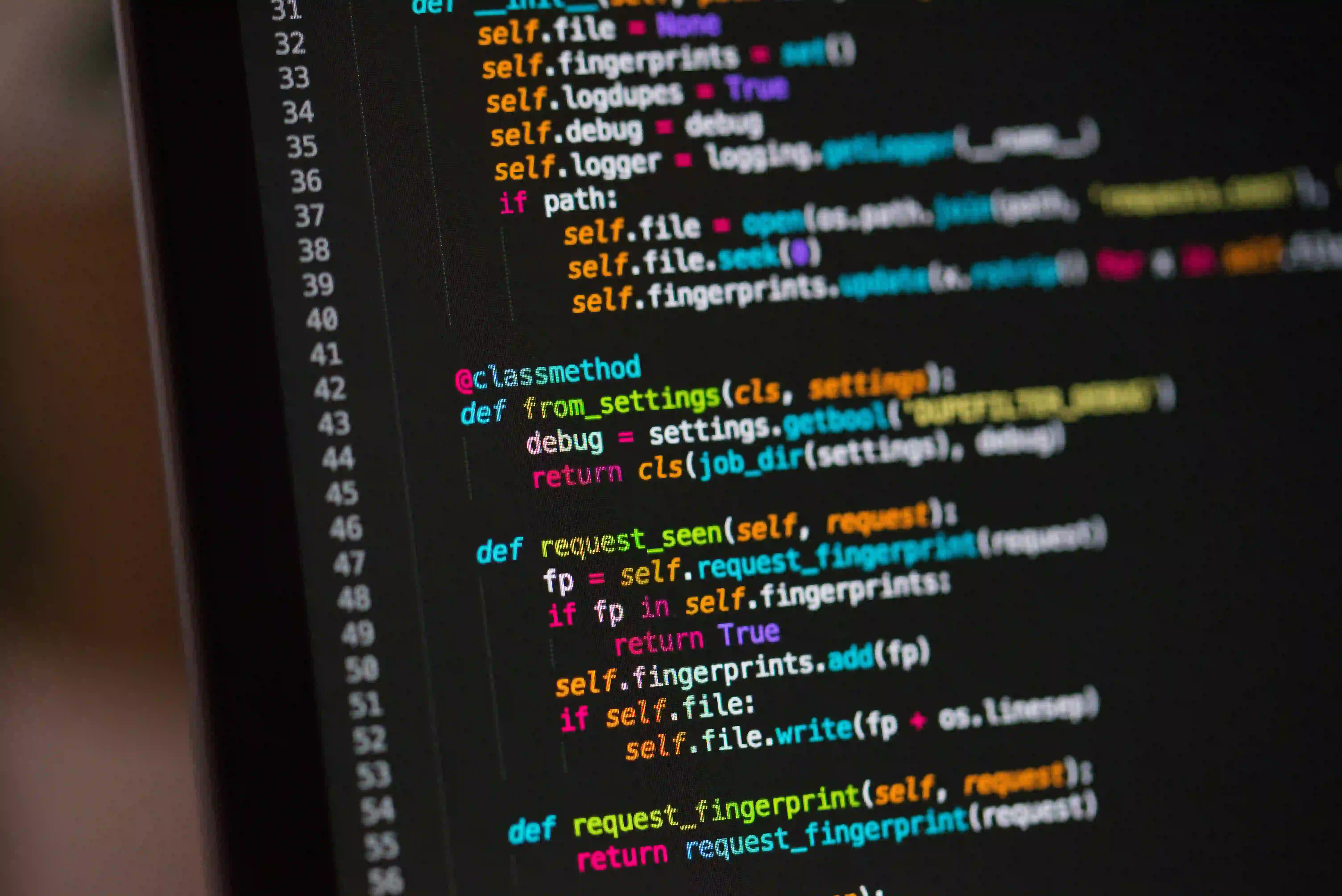
Common Spring MVC Errors Beginners Make and How to Fix Them
Spring MVC is a powerful framework for building web applications in Java. However, beginners often encounter common pitfalls that can hinder their development experience. In this post, we'll discuss some typical errors, provide solutions, and delve into the underlying rationale behind each issue.
Table of Contents
Understanding Spring MVC
Spring MVC is part of the larger Spring framework, designed for creating Java-based web applications. It follows the Model-View-Controller design pattern, separating concerns for improved management, scalability, and maintainability. This framework simplifies the process of handling requests and responses while enabling the use of several view technologies like JSP, Thymeleaf, and others.
While building applications with Spring MVC can be rewarding, it’s easy for beginners to make mistakes. Let’s explore some common errors and how to resolve them effectively.
Common Errors and Fixes
1. No Handler Found for URL
One of the most common issues beginners face in Spring MVC is the "No handler found for URL" error. This typically occurs when the URL does not match any controller mappings.
Why It Happens: 100% of your URI must correspond to the correct @RequestMapping in your controller.
Solution: To fix this, ensure that you have set up your controller correctly and that your URI pattern matches. Here’s an example:
@Controller
@RequestMapping("/greetings")
public class GreetingController {
@GetMapping("/hello")
public String hello(Model model) {
model.addAttribute("message", "Hello, Spring MVC!");
return "hello";
}
}
In this code, we defined a controller with a specific request mapping. Make sure you navigate to /greetings/hello
in your browser; otherwise, you'll receive an error indicating that no handler was found.
2. Incorrect Bean Configuration
Another common issue is related to misconfigured beans in the applicationContext.xml
file or using @Configuration incorrectly.
Why It Happens: Spring cannot create or access beans if their configuration is incorrect.
Solution: Ensure that you define beans properly and use the right annotations. For example, if you're using an @Autowired annotation, ensure that component scanning is set up correctly in your configuration.
<context:component-scan base-package="com.example.controllers"/>
For Java-based configuration, make sure your classes look like this:
@Configuration
@EnableWebMvc
public class WebConfig {
@Bean
public ViewResolver viewResolver() {
InternalResourceViewResolver resolver = new InternalResourceViewResolver();
resolver.setPrefix("/WEB-INF/views/");
resolver.setSuffix(".jsp");
return resolver;
}
}
3. Missing/Incorrect View Resolvers
If you have set up everything correctly but still encounter a blank page or errors related to views, it could be due to a missing or improperly configured view resolver.
Why It Happens: Spring MVC needs a way to translate your view names into actual views (like JSP or Thymeleaf templates) through resolvers.
Solution: Define a ViewResolver bean in your configuration:
@Bean
public ViewResolver viewResolver() {
InternalResourceViewResolver resolver = new InternalResourceViewResolver();
resolver.setPrefix("/WEB-INF/views/");
resolver.setSuffix(".jsp");
return resolver;
}
Make sure the view files are in the correct directory (/WEB-INF/views/
) with the correct file extension (.jsp
).
4. Data Binding Issues
Data binding is crucial in Spring MVC applications, especially for forms. Beginners often face issues when model attributes are not bound correctly.
Why It Happens: This can occur due to mismatching names between your form fields and your model attributes.
Solution: Ensure that the field names in your HTML form match the property names in your model class. Here’s an example:
public class User {
private String name;
private Int age;
// Getters and Setters
}
<form action="/submitUser" method="post">
Name: <input type="text" name="name" />
Age: <input type="text" name="age" />
<input type="submit" value="Submit" />
</form>
And the corresponding controller:
@PostMapping("/submitUser")
public String submitUser(@ModelAttribute User user, Model model) {
model.addAttribute("userDetails", user);
return "userResult";
}
5. CSRF Protection Errors
Cross-Site Request Forgery (CSRF) protection is enabled by default in Spring Security. Beginners might get confused when forms fail to submit or when CSRF token errors arise.
Why It Happens: The default CSRF protection expects a token to be sent along with the form submission. Failing to include this token can lead to submission errors.
Solution: Include the anti-CSRF token in your forms. Use the following tag if you’re using JSP:
<form action="/submitUser" method="post">
<input type="${_csrf.parameterName}" value="${_csrf.token}" type="hidden" />
...
</form>
The Last Word
Understanding and avoiding common errors in Spring MVC will greatly enhance your development experience and efficiency. Each of these issues stems not just from mistakes but from understanding how Spring MVC operates.
As you progress in mastering Spring MVC, keep these common pitfalls in mind: No handler found for URL, incorrect bean configurations, missing view resolvers, data binding issues, and CSRF protection errors.
With this knowledge, you'll save time and frustration in your projects, making Spring MVC an effective tool for your web development needs.
For further reading, consider checking the Spring MVC Documentation and other resources to deepen your understanding of the framework. Happy coding!