Common Pitfalls When Implementing Specification Pattern in JPA
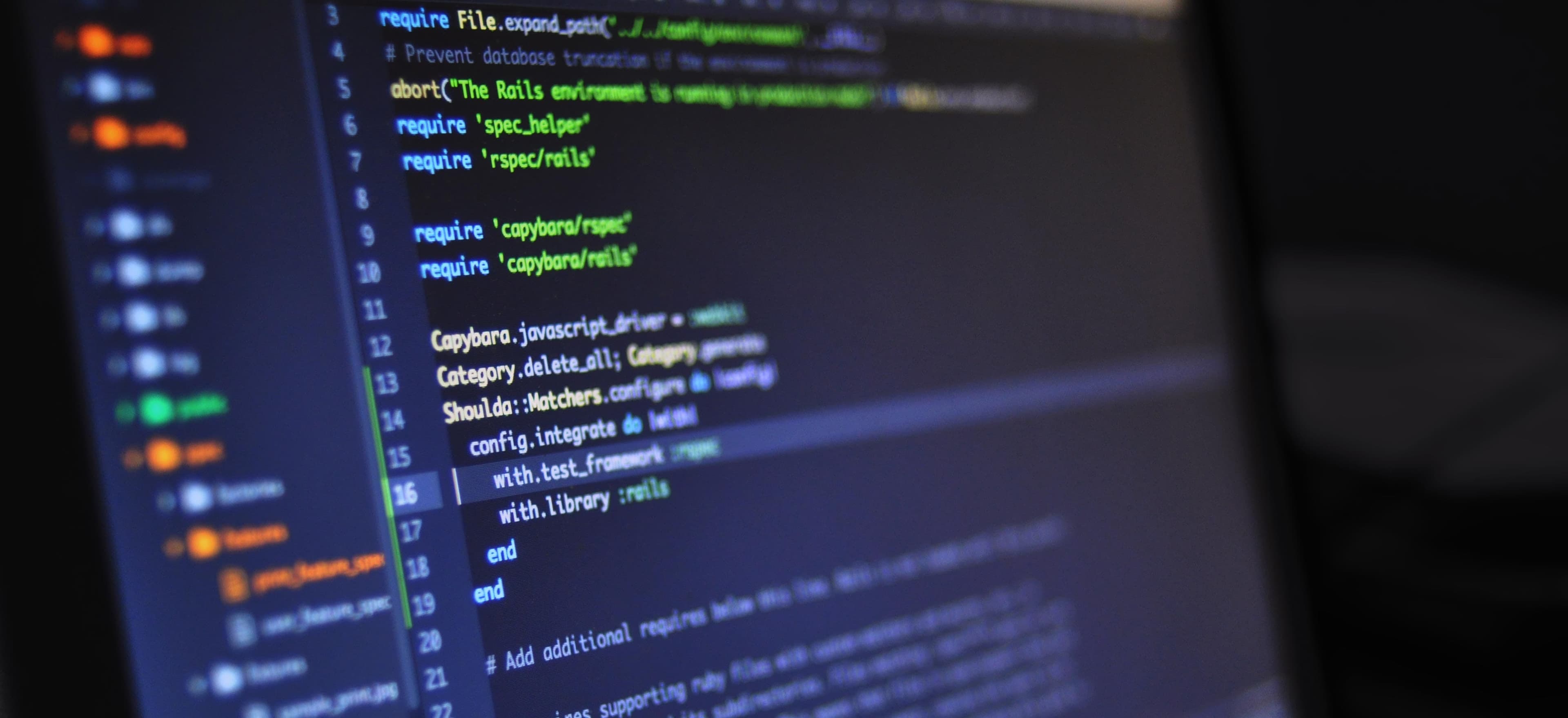
- Published on
Common Pitfalls When Implementing the Specification Pattern in JPA
The Specification Pattern is a powerful design pattern, particularly useful in Java Persistence API (JPA) environments. It provides a flexible way to encapsulate business rules and filter data. However, while implementing this pattern, developers often encounter common pitfalls that can lead to performance issues, maintenance challenges, and even bugs. In this blog post, we will explore these pitfalls and offer solutions to overcome them.
What is the Specification Pattern?
The Specification Pattern allows you to create a reusable business logic piece that can be combined to form complex queries. In JPA, this is particularly beneficial as it enables you to build dynamic queries based on varying business requirements without cluttering your code with hard-coded logic.
Basic Example of the Specification Pattern
Before diving into the pitfalls, let’s examine a basic usage example.
import org.springframework.data.jpa.domain.Specification;
public class UserSpecifications {
public static Specification<User> hasName(String name) {
return (root, query, criteriaBuilder) -> criteriaBuilder.equal(root.get("name"), name);
}
public static Specification<User> hasAgeGreaterThan(int age) {
return (root, query, criteriaBuilder) -> criteriaBuilder.greaterThan(root.get("age"), age);
}
}
In this example, we create simple specifications for filtering users by name and age. Now that we understand how to create specifications, let's explore the common pitfalls.
Common Pitfalls
1. Ignoring Performance Considerations
One of the most significant pitfalls in implementing the Specification Pattern is neglecting performance implications, especially when combining multiple specifications.
Why It Matters
Chaining multiple specifications can lead to complex SQL queries. If not optimized, these queries can degrade performance as the dataset grows. Inefficient joins, unnecessary subqueries, or Cartesian products can arise if proper care is not taken when defining specifications.
Solution
- Analyze Query Execution Plans: Regularly check the generated SQL queries. Use tools like Hibernate's query plan logger to analyze execution time and performance.
- Optimize Specifications: Group specifications thoughtfully. Sometimes you may need to rewrite them to avoid unnecessary complexity.
// Example of combining specifications
Specification<User> criteria = Specification.where(UserSpecifications.hasName("John"))
.or(UserSpecifications.hasAgeGreaterThan(30));
Here, we use the or
method to combine two specifications logically. Always ensure that your database can handle such queries efficiently.
2. Overusing Specifications
Another notable pitfall is overusing specifications, especially for simple queries that can be achieved more straightforwardly.
Why It Matters
While specifications promote code reusability, their overuse can lead to convoluted code, making it harder to read and maintain. For simple filters, using standard repository methods may be more efficient and easier to understand.
Solution
Only use specifications when benefits are apparent. For simple queries, stick to JPA repository methods. For example:
List<User> users = userRepository.findByName("John");
Reserve specifications for more complex, reusable scenarios.
3. Neglecting Joins and Relationships
Specifications don't automatically handle entity relationships, which can result in unexpected results if they're not explicitly defined.
Why It Matters
Neglecting to properly fetch and join related entities can lead to N+1 select problems, hampering performance. If a specification assumes certain data already exists in memory but it’s lazy-loaded instead, it may yield incorrect results.
Solution
- Eager Fetching: If you anticipate needing related entities, ensure they are eagerly fetched.
- Explicit Joins: Define joins explicitly within specifications.
Example:
public static Specification<User> hasRole(String role) {
return (root, query, criteriaBuilder) -> {
root.fetch("roles"); // Explicitly fetching related roles entity
return criteriaBuilder.equal(root.join("roles").get("name"), role);
};
}
This approach ensures the roles are fetched and prevent N+1 problems.
4. Failing to Handle Null Values
Many developers overlook how their specifications handle null values, which can lead to unexpected behaviors.
Why It Matters
If you're not considering null checks, such as filtering for non-existent attributes, your specifications can return incorrect results.
Solution
Use null-safe operations in your specifications:
public static Specification<User> hasEmail(String email) {
return (root, query, criteriaBuilder) -> {
if (email == null) {
return criteriaBuilder.isNull(root.get("email"));
}
return criteriaBuilder.equal(root.get("email"), email);
};
}
By adding null checks, you make your specifications robust and error-proof.
5. Not Leveraging QueryDSL or Criteria API Properly
Relying solely on String query formations or forgetting about strong typing features can diminish the benefits of using JPA Specifications.
Why It Matters
Static queries are prone to errors, making the code less readable and maintainable. The Criteria API offers a type-safe way to construct queries.
Solution
Utilize the Criteria API fully, incorporating Predicate
, CriteriaQuery
, and CriteriaBuilder
where necessary. For complex queries, consider integrating QueryDSL for a fluent API that enhances readability.
6. Hardcoding Filter Logic
In some cases, developers might hardcode filtering logic directly into their service layers rather than encapsulating this within specifications.
Why It Matters
This practice violates the Single Responsibility Principle, making the code less maintainable. Once conditions become complex, you may face increased code duplication.
Solution
Ensure that filtering logic is always defined in specifications. Create rules that can be reused across different service calls.
Example of moving the filtering logic to a specification:
public List<User> findUsersBySearchCriteria(UserSearchCriteria criteria) {
Specification<User> spec = Specification.where(null);
if (criteria.getName() != null) {
spec = spec.and(UserSpecifications.hasName(criteria.getName()));
}
if (criteria.getAge() != null) {
spec = spec.and(UserSpecifications.hasAgeGreaterThan(criteria.getAge()));
}
return userRepository.findAll(spec);
}
This approach keeps your business logic clean and manageable.
To Wrap Things Up
Implementing the Specification Pattern in JPA is a powerful technique that promotes code reuse and maintainability. However, developers must be cautious of common pitfalls, including performance issues, unnecessary complexity, handling relationships, null values, and ensuring code clarity.
By keeping in mind the solutions outlined in this article, you can leverage the Specification Pattern effectively. Always aim for simplicity, readability, and optimization in your code. With a solid understanding of these potential pitfalls, your JPA specifications will not only be more effective but will also lead to a more maintainable codebase.
For more insights on JPA and design patterns, consider reading more on Spring Data JPA and Design Patterns in Java.
Happy coding!