Mastering Java Design Patterns: Common Pitfalls to Avoid
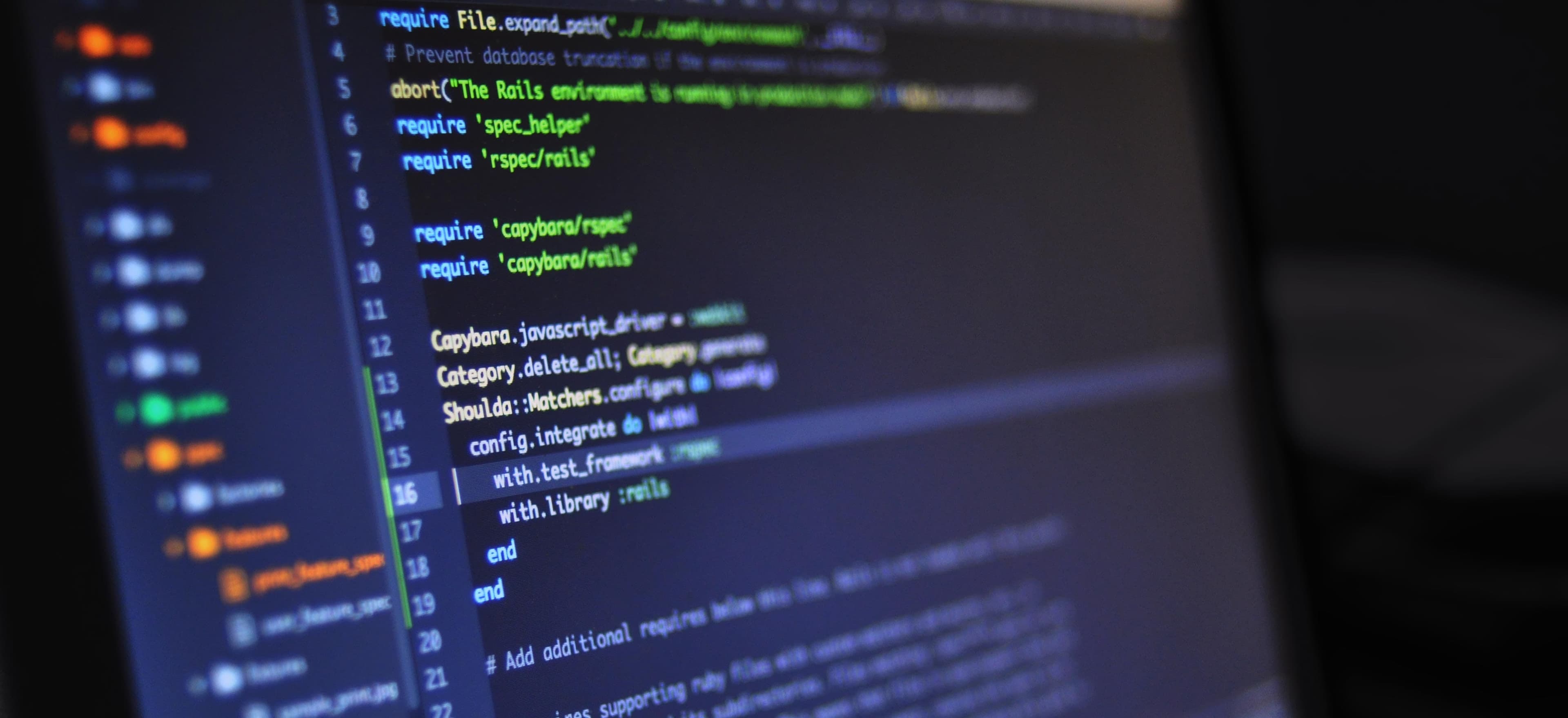
- Published on
Mastering Java Design Patterns: Common Pitfalls to Avoid
Java design patterns are proven solutions to common problems encountered in software design. They provide a template for writing code that is modular, reusable, and easy to understand. However, even seasoned developers can fall into pitfalls when applying these patterns. This blog post will explore those pitfalls and provide guidance on mastering Java design patterns effectively.
What are Design Patterns?
Design patterns are standard solutions to recurring design problems in software development. They can be categorized into three main types:
-
Creational Patterns: Focus on object creation mechanisms, trying to create objects in a manner suitable to the situation. Examples: Singleton, Factory.
-
Structural Patterns: Concerned with how classes and objects are composed to form larger structures. Examples: Adapter, Composite.
-
Behavioral Patterns: Deal with object collaboration and how responsibilities are distributed between objects. Examples: Observer, Command.
Common Pitfalls in Implementing Design Patterns
As you venture into the world of design patterns, be mindful of the following pitfalls:
1. Over-Engineering
One major pitfall developers often encounter is over-engineering the design. It is easy to get carried away with design patterns and create complex systems when simple solutions would suffice.
Why You Should Avoid It
Over-engineering leads to bloated code, making it difficult to maintain and understand. Simplicity should always be a priority in design.
// Example of over-engineering:
public interface Shape {
void draw();
}
public class Circle implements Shape {
public void draw() {
// Drawing a circle
}
}
public class Square implements Shape {
public void draw() {
// Drawing a square
}
}
public class ShapeDrawer {
public static void main(String[] args) {
Shape circle = new Circle();
Shape square = new Square();
// Complex logic to draw shapes
}
}
In this case, introducing an interface isn't necessary if the functionality is simple enough. A direct approach would be cleaner.
2. Ignoring the Context
Not all design patterns fit every situation. Using a design pattern just because it is popular or trendy can lead to poor decisions. It's crucial to assess your specific context.
Why You Should Avoid It
Ignoring the context results in applying designs that do not solve the actual problem at hand. Always justify the use of a design pattern.
// Example of fatigue in misuse:
public class DatabaseConnection {
private static DatabaseConnection instance;
private DatabaseConnection() {
// Private constructor
}
public static DatabaseConnection getInstance() {
if (instance == null) {
instance = new DatabaseConnection();
}
return instance;
}
}
public class Application {
public static void main(String[] args) {
DatabaseConnection connect = DatabaseConnection.getInstance();
// Inappropriate use leading to redundancy if it's not multi-threaded
}
}
The Singleton pattern is unnecessary if multiple instances are needed or if the application can handle connection pooling. Thus, evaluate whether a Singleton is the best choice.
3. Overusing Inheritance
Inheritance is a powerful concept in Java. However, using it to replicate patterns can create tightly coupled code, making it difficult to modify.
Why You Should Avoid It
Tightly coupled code can lead to a rigidity that hampers future development. Favor composition over inheritance when applying patterns.
// Example of overusing inheritance:
public class Animal {
public void makeSound() {
// Default sound
}
}
public class Dog extends Animal {
public void makeSound() {
// Dog barks
}
}
public class Cat extends Animal {
public void makeSound() {
// Cat meows
}
}
Instead, consider using an interface or abstract class to allow for more flexibility:
public interface Sound {
void makeSound();
}
public class Dog implements Sound {
public void makeSound() {
// Dog barks
}
}
public class Cat implements Sound {
public void makeSound() {
// Cat meows
}
}
4. Forgetting to Document
Proper documentation is vital, especially when working with design patterns. Failing to document your code can lead to misunderstandings and misusage by your team members.
Why You Should Avoid It
Well-documented code fosters collaboration and eases onboarding new developers. It serves as a guide for the usage and intent behind the chosen design patterns.
/**
* Implementation of Observer design pattern
*/
public interface Observer {
void update(String event);
}
public class ConcreteObserver implements Observer {
@Override
public void update(String event) {
// Reacting to the update
}
}
5. Not Leveraging Existing Frameworks
Often, developers overlook the power of frameworks that already implement various design patterns. Creating your own from scratch can split focus and lead to inconsistencies.
Why You Should Avoid It
Frameworks like Spring or Java EE incorporate established design patterns, allowing you to utilize their simplicity and efficiency. This is crucial for accelerated development and reduced errors.
// Utilizing Spring to implement a Singleton
@Service
public class SingletonService {
// Logic here
}
Here's a relevant Spring Design Patterns guide for further understanding.
Closing the Chapter
Java design patterns are a powerful tool for creating robust and maintainable software. However, they can turn into pitfalls if misused. To effectively master design patterns, always consider the context, document your decisions, and strive for simplicity.
In your journey, remember to continually educate yourself about best practices. This not only helps avoid common mistakes but also fosters a culture of learning within your team.
By integrating these lessons, you can avoid the common pitfalls discussed and utilize design patterns to enhance your Java applications effectively. Enjoy coding and embrace the elegance and power of design patterns!
For further reading, you might explore this comprehensive guide to Java Design Patterns which dives more deeply into practical implementations and examples.
Start implementing these concepts today and watch as your coding practices elevate to a new level!
Checkout our other articles