Common Pitfalls in Java Encryption: Avoid These Mistakes!
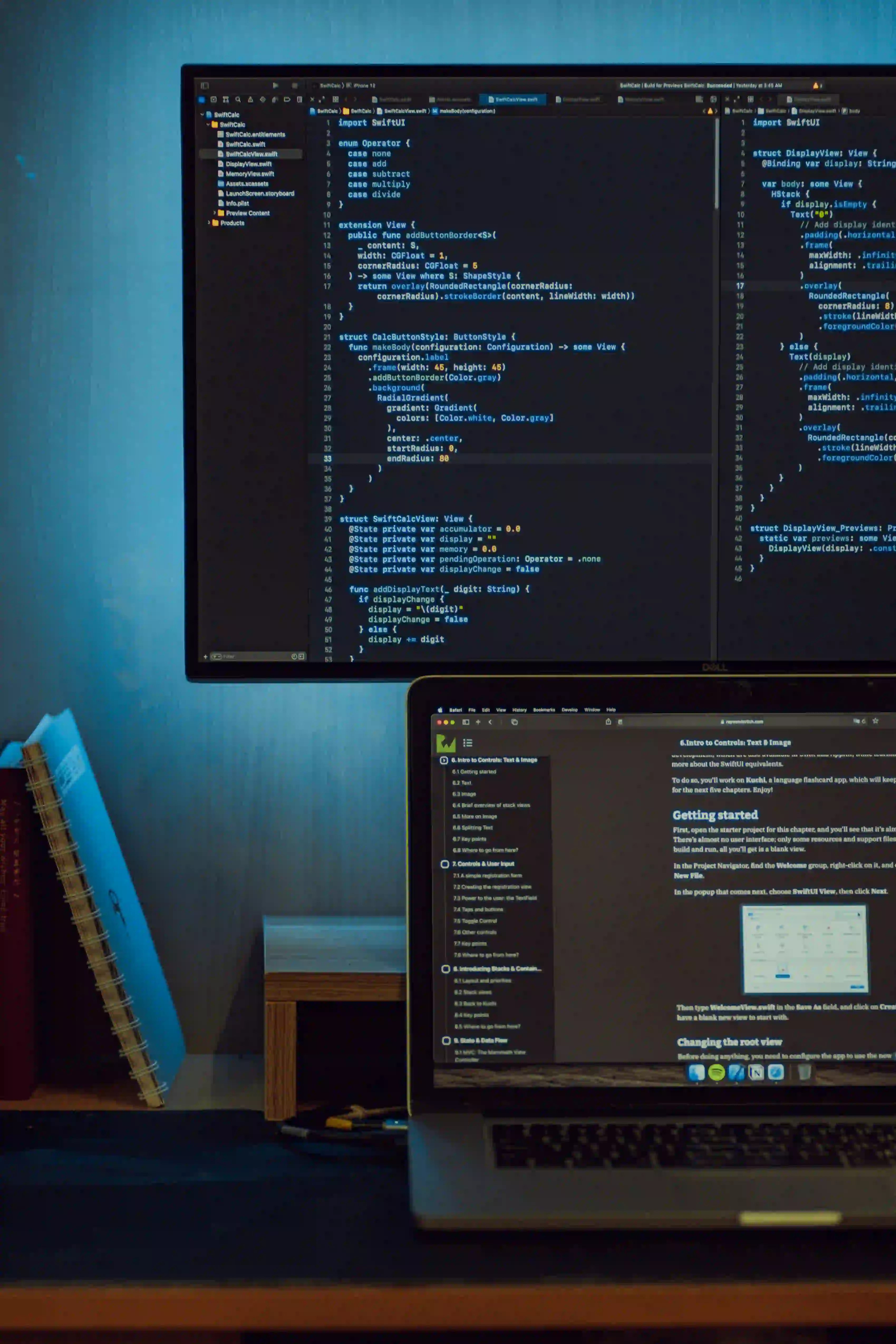
Common Pitfalls in Java Encryption: Avoid These Mistakes!
In today's digital age, securing data is more crucial than ever. One of the core components of data security is encryption. Java, with its rich set of libraries, offers robust tools for implementing encryption. However, developers often fall prey to various pitfalls that compromise the security of their applications. In this article, we'll explore common mistakes in Java encryption and provide guidance on how to avoid them.
Table of Contents
- Understanding Encryption
- Common Pitfalls in Java Encryption
- 2.1 Hardcoding Keys
- 2.2 Using Weak Algorithms
- 2.3 Improper Key Management
- 2.4 Ignoring Initialization Vectors (IV)
- 2.5 Failing to Use Secure Random
- Best Practices for Java Encryption
- Conclusion
Understanding Encryption
Encryption is a method of converting plain text into ciphertext to prevent unauthorized access. The two main types of encryption are symmetric and asymmetric:
- Symmetric Encryption: Uses the same key for both encryption and decryption.
- Asymmetric Encryption: Utilizes a pair of keys, a public key for encryption and a private key for decryption.
Java provides the javax.crypto
package, which includes classes for implementing both types of encryption. It's essential to understand the fundamental concepts of encryption before diving into implementation.
Common Pitfalls in Java Encryption
2.1 Hardcoding Keys
One of the most significant mistakes developers make is hardcoding encryption keys within the source code. This practice exposes sensitive information, making it easy for attackers to compromise the encryption.
Safer Approach
Instead, use external configuration files or secure environment variables to store keys. For example, you can use Java's Properties
class to load key information securely:
import java.util.Properties;
import java.io.InputStream;
public class KeyUtil {
public static String loadKey() {
String key = null;
try (InputStream input = KeyUtil.class.getClassLoader().getResourceAsStream("config.properties")) {
Properties prop = new Properties();
prop.load(input);
key = prop.getProperty("encryptionKey");
} catch (Exception e) {
e.printStackTrace();
}
return key;
}
}
Why: This keeps your key secure and separate from the source code, reducing the risk of exposure.
2.2 Using Weak Algorithms
Not all encryption algorithms offer the same level of security. Many developers may unknowingly utilize outdated or weak encryption methods like DES or RC4. These algorithms are vulnerable and can be broken relatively easily.
Safer Approach
Always opt for strong, modern algorithms such as AES (Advanced Encryption Standard). Here’s how you can implement AES encryption in Java:
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
public class AesEncrypt {
public static void main(String[] args) throws Exception {
KeyGenerator keyGen = KeyGenerator.getInstance("AES");
keyGen.init(128); // You can also use 192 or 256
SecretKey secretKey = keyGen.generateKey();
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
byte[] input = "Hello, World!".getBytes();
byte[] encrypted = cipher.doFinal(input);
System.out.println("Encrypted Text: " + new String(encrypted)); // Display as base64 or in hex for better readability
}
}
Why: AES is widely accepted and considered secure, making it ideal for modern applications.
2.3 Improper Key Management
Even strong encryption can be rendered useless if the keys are not managed properly. Common issues include lack of rotation, inadequate access control, and improper disposal of old keys.
Safer Approach
Implement a key management strategy that includes key rotation policies and secure key storage solutions like AWS KMS, Hashicorp Vault, or hardware security modules (HSM).
// Example of rotating keys
public void rotateKey(String oldKey, String newKey) {
// Logic for rotating keys while ensuring data availability and integrity
// This may include re-encrypting data with a new key
}
Why: Proper key management variously strengthens security by ensuring that keys are not overly exposed and outdated keys are retired.
2.4 Ignoring Initialization Vectors (IV)
When using block ciphers like AES, an IV is a critical component. Failing to use an IV or reusing it can lead to patterns in ciphertext, which attackers can exploit.
Safer Approach
Always generate a new, random IV for each encryption operation. Here’s an example of how to use an IV with AES:
import javax.crypto.spec.IvParameterSpec;
import java.security.SecureRandom;
public class AesWithIV {
public static void main(String[] args) throws Exception {
SecretKey secretKey = // Load your secret key
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
byte[] iv = new byte[16]; // AES block size
SecureRandom random = new SecureRandom();
random.nextBytes(iv);
IvParameterSpec ivParams = new IvParameterSpec(iv);
cipher.init(Cipher.ENCRYPT_MODE, secretKey, ivParams);
byte[] input = "Hello, World!".getBytes();
byte[] encrypted = cipher.doFinal(input);
System.out.println("Encrypted Text: " + new String(encrypted));
}
}
Why: An IV adds randomness to the encryption process, ensuring that the same plaintext encrypted multiple times will yield different ciphertext.
2.5 Failing to Use Secure Random
Random number generation is crucial in encryption processes, especially for key and IV generation. Using predictable random number generators can compromise your security.
Safer Approach
Utilize Java's SecureRandom
for generating secure random numbers. Always prefer SecureRandom
over Random
when working with cryptographic applications.
import java.security.SecureRandom;
public class SecureRandomExample {
public static void main(String[] args) {
SecureRandom secureRandom = new SecureRandom();
byte[] randomBytes = new byte[16];
secureRandom.nextBytes(randomBytes);
// Now use randomBytes securely
}
}
Why: SecureRandom
provides a stronger level of randomness suitable for cryptographic operations, making keys and IVs less predictable.
Best Practices for Java Encryption
After identifying common pitfalls, here are several best practices to follow when implementing encryption in Java:
-
Always Use Strong Algorithms: Stick to recognized standards like AES for symmetric encryption and RSA for asymmetric encryption.
-
Employ Salt and Hashing for Passwords: Use strong hashing algorithms with salt for user passwords instead of encrypting them directly. Libraries like Bcrypt are great for this.
-
Use HTTPS for Network Communication: Always encrypt data in transit using protocols like HTTPS, ensuring end-to-end security.
-
Regularly Update Libraries: Keep your Java cryptographic libraries up to date to leverage the latest security patches and improvements.
-
Conduct Regular Security Audits: Continuously evaluate your encryption implementation to identify vulnerabilities.
To Wrap Things Up
Encryption is a powerful tool for safeguarding data, but improper implementation can lead to serious security issues. By avoiding common pitfalls such as hardcoding keys, using weak algorithms, and neglecting proper key management practices, you can significantly enhance the security of your Java applications.
By following best practices and staying informed about the latest advancements in encryption, you can turn potential vulnerabilities into strengths. For more in-depth reading on cryptography in Java, refer to resources such as the official Java Cryptography Architecture Guide.
For further exploration, check out the Bouncy Castle Cryptography API. This library offers additional encryption capabilities that go beyond what is provided in standard Java, making it a popular choice for many developers.
Implement these guidelines, and take control of your data security today!