Mastering Optional: Avoiding Null Pointer Exceptions in Java 8
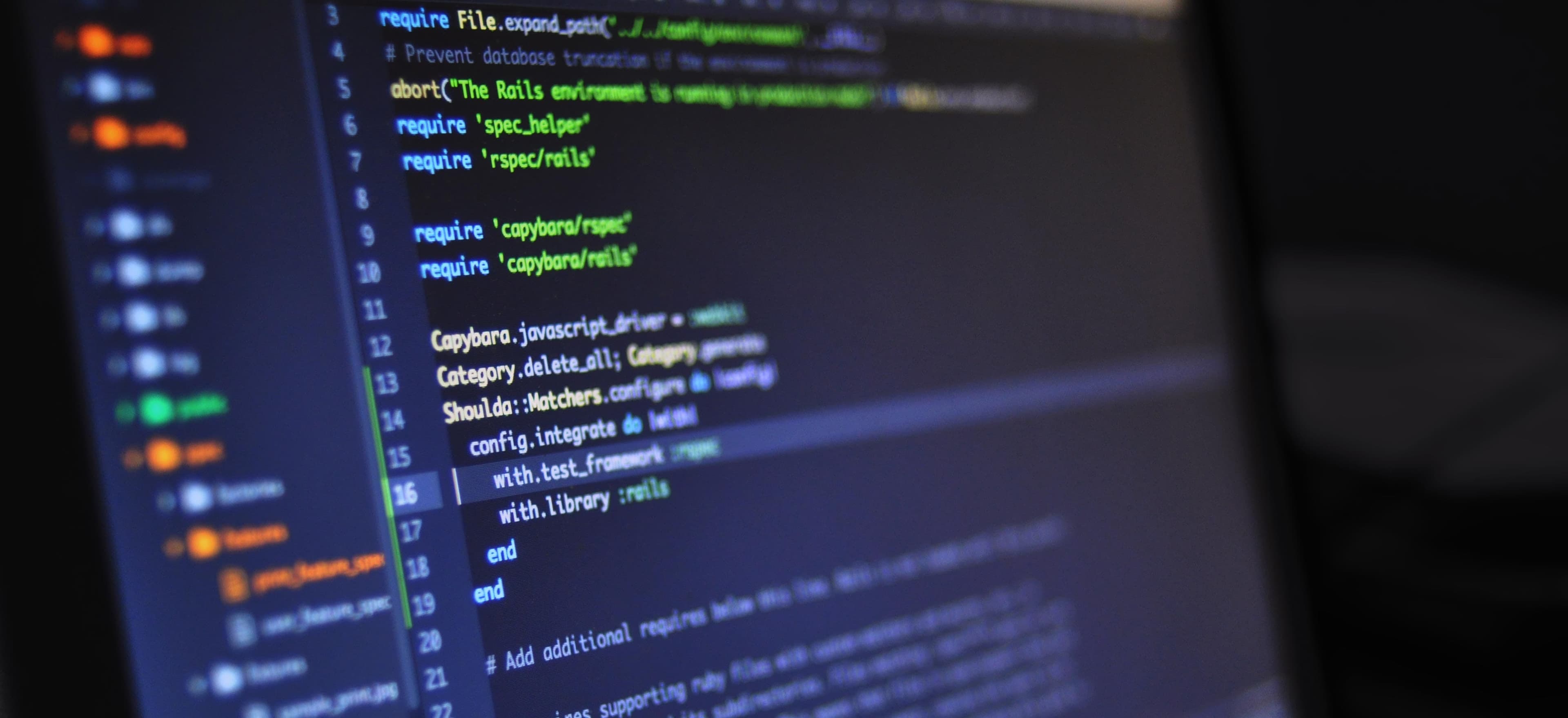
- Published on
Mastering Optional: Avoiding Null Pointer Exceptions in Java 8
In Java programming, one of the most notorious issues developers face is the dreaded Null Pointer Exception (NPE). This problem arises when you try to use an object reference that has not been initialized. With the advent of Java 8, a powerful new tool was introduced: the Optional
class. This blog aims to delve deep into the Optional
class, elucidate its advantages, and explain how it can be effectively used to avoid NPEs while providing cleaner, more readable code.
Understanding the Problem: Null Pointer Exceptions
Before we explore the solution that Optional
provides, let's examine the root cause of many Null Pointer Exceptions. Consider a typical scenario:
public String getUserEmail(User user) {
return user.getEmail();
}
In this snippet, if user
is null
, invoking getEmail()
will throw an NPE.
Consequences of NPEs
NPEs can lead to application crashes, which can result in a poor user experience and increased debugging time for developers. To better handle such situations, Java 8 introduced Optional
.
What is Optional?
Optional
is a container that may or may not contain a non-null value. By design, it prevents the use of null
directly and encourages a more declarative style of coding. This means it is explicit about whether a value is present or absent.
Syntax of Optional
The most common ways to create an Optional
object are:
- Using
Optional.of(value)
- **Using
Optional.ofNullable(value)**
- Using
Optional.empty()
Here’s how these methods work:
Optional<String> presentOpt = Optional.of("Email@example.com");
Optional<String> emptyOpt = Optional.empty();
Optional<String> nullableOpt = Optional.ofNullable(null); // This will not throw an exception
In the above code:
Optional.of(value)
will throw aNullPointerException
if the value isnull
.Optional.ofNullable(value)
safely wraps a value and returns an emptyOptional
if it'snull
.Optional.empty()
creates an emptyOptional
.
Benefits of Using Optional
- Clarity: Using
Optional
signals to the user that a value might be absent. - Method Chaining: It provides elegant and fluent APIs.
- Prevention of NPEs: It inherently limits the chances of NPE, making your code safer.
Using Optional in Practice
Let's transform our previous getUserEmail
method to use Optional
effectively.
Refactoring the Code
First, we are going to assume User
might not always be present. We modify our method to return an Optional<String>
:
public Optional<String> getUserEmail(Optional<User> user) {
return user.map(User::getEmail); // This will return an empty Optional if user is not present
}
In this code:
- The
map
function appliesgetEmail()
only if a value is present, ensuring safety against NPE.
Handling Optional Values
Now, how do we handle the possible absence of a value? Java 8 provides several methods to do that: isPresent()
, ifPresent()
, orElse()
, and orElseGet()
.
Example Usage of Optional
Optional<String> emailOpt = getUserEmail(Optional.ofNullable(user));
emailOpt.ifPresent(email -> System.out.println("User email is: " + email)); // Prints email if present
String email = emailOpt.orElse("No email provided");
System.out.println(email);
In the example above:
ifPresent
takes a lambda expression to handle the case when the value exists.orElse
provides a fallback value if theOptional
is empty.
Transformation with FlatMap
Sometimes, you need to continue chaining Optional operations; for instance, if the value itself returns an Optional
. In such cases, you can use flatMap
.
public Optional<String> getEmailDomain(Optional<User> user) {
return user.flatMap(u -> Optional.ofNullable(u.getEmail()))
.map(email -> email.substring(email.indexOf("@") + 1)); // Extract domain
}
With flatMap
, you get another level of safety by preventing a second layer of Optional
nesting. The methods of Optional
are all designed to help you avoid the pitfalls of direct null-checking.
Best Practices with Optional
- Return Optional instead of null: Prefer returning
Optional<T>
for methods that can return a nullable value. - Avoid Optional Fields: Do not use
Optional
for fields in your classes; instead, use it for method return values. - Use
Optional
only when beneficial: Don’t overuse it; for simple cases,null
may suffice.
Wrapping Up
Java 8's Optional
is a robust tool that helps developers sidestep the perils of Null Pointer Exceptions. By embracing Optional
, you can write cleaner, safer, and more manageable code. You benefit not only from improved readability but also from a declarative style that clearly communicates your intent.
To further explore Optional
and its methods, refer to the official Java Documentation.
In the ever-evolving world of software development, familiarity with constructs like Optional
is invaluable. So, your next task? Start incorporating Optional
where appropriate and watch your applications become more resilient against NPEs!
By mastering Optional
, you can significantly enhance the robustness of your Java applications. Dive deeper into Java 8 features, and stay ahead in your coding journey!
Checkout our other articles