Overcoming the Complexity of Chain of Responsibility Pattern
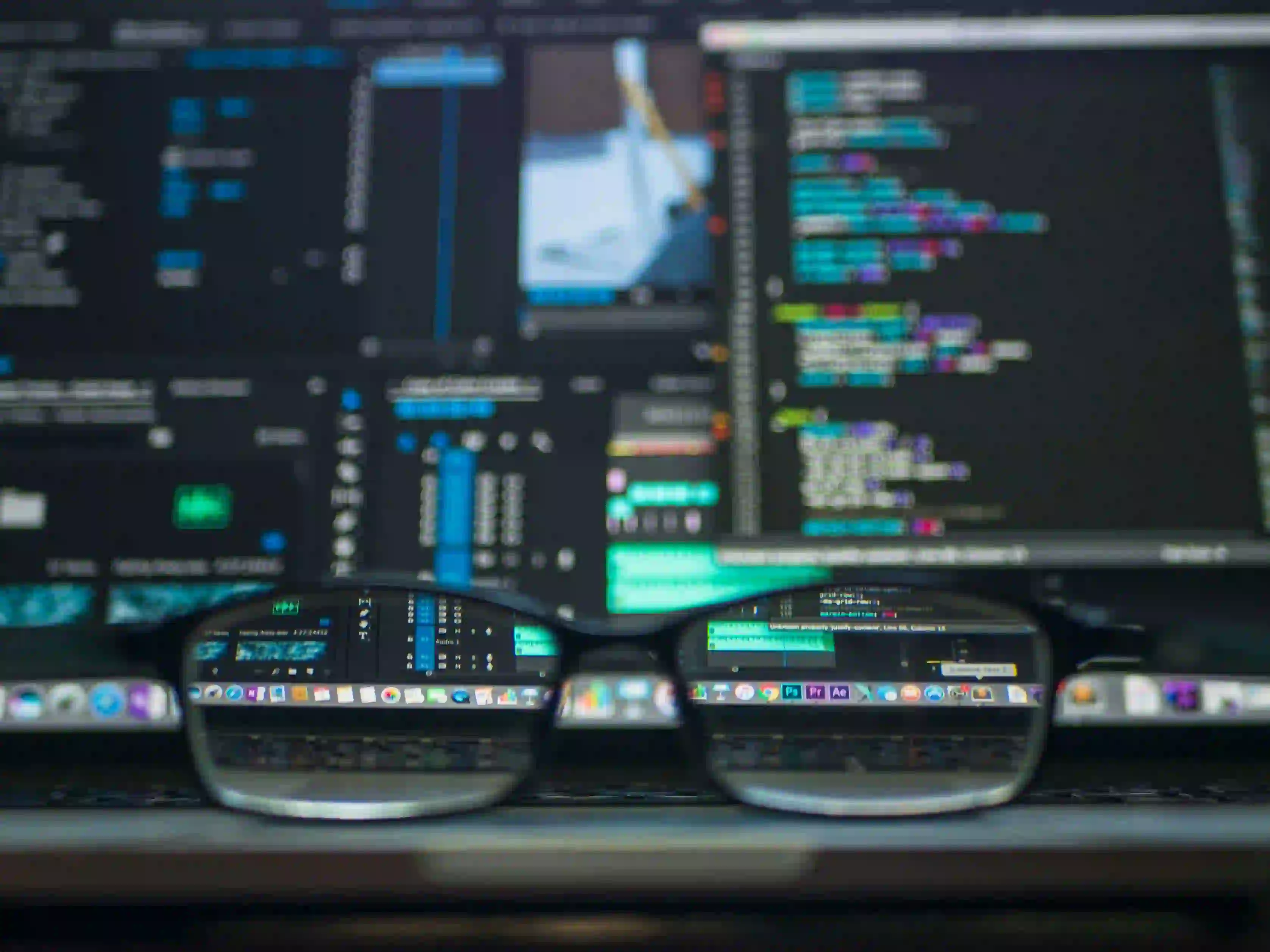
Overcoming the Complexity of the Chain of Responsibility Pattern in Java
Software design patterns are an essential part of developing maintainable and flexible code. One such pattern, the Chain of Responsibility (CoR), allows an object to pass a request along a chain of potential handlers until one of them processes the request. It embodies the principle of decoupling the sender of a request from its receivers.
While the CoR pattern offers significant advantages, it can also introduce complexity that can be challenging to manage. In this blog post, we'll explore the Chain of Responsibility pattern, its benefits, challenges, and best practices to overcome its complexity. We will include code snippets demonstrating the concept in Java, along with explanations of each element.
What is the Chain of Responsibility Pattern?
The Chain of Responsibility pattern is designed to handle requests in a flexible way without the sender needing to know which receiver will handle the request. Instead, the request is passed along a chain of handlers, where each handler can either process the request or pass it to the next handler in the chain.
Benefits of the Chain of Responsibility Pattern
- Decoupling: The sender and receivers are decoupled, allowing for easier modifications or replacement of handlers.
- Flexible Handling: You can dynamically change the chain of handlers at runtime.
- Single Responsibility: Each handler focuses on a specific type of request or functionality.
Challenges with the Chain of Responsibility Pattern
- Complexity of Configuration: As the number of handlers increases, configuring the chain can become cumbersome.
- Difficulty in Debugging: Tracing requests through multiple handlers can complicate debugging.
- Performance Issues: If not implemented carefully, it can lead to a performance overhead due to potential long chains.
Implementing the Chain of Responsibility Pattern in Java
Let’s create a simple example to illustrate the Chain of Responsibility pattern in Java. We’ll model a scenario of processing user support requests.
Step 1: Creating the Request and Handler Interfaces
First, we define a SupportRequest
class, which will represent the request, and a SupportHandler
interface that declares a method for processing the request.
public class SupportRequest {
private String requestType;
public SupportRequest(String requestType) {
this.requestType = requestType;
}
public String getRequestType() {
return requestType;
}
}
public interface SupportHandler {
void setNextHandler(SupportHandler nextHandler);
void handleRequest(SupportRequest request);
}
Why this structure?
This separation allows for flexible handler configurations. Each handler can set its successor, facilitating a chain without requiring knowledge of the handler's type.
Step 2: Implementing Concrete Handlers
Next, we create several concrete handlers, each responsible for handling specific types of support requests.
public class TechnicalSupportHandler implements SupportHandler {
private SupportHandler nextHandler;
@Override
public void setNextHandler(SupportHandler nextHandler) {
this.nextHandler = nextHandler;
}
@Override
public void handleRequest(SupportRequest request) {
if (request.getRequestType().equalsIgnoreCase("Technical")) {
System.out.println("TechnicalSupportHandler: Handling technical support request.");
} else if (nextHandler != null) {
nextHandler.handleRequest(request);
}
}
}
public class BillingSupportHandler implements SupportHandler {
private SupportHandler nextHandler;
@Override
public void setNextHandler(SupportHandler nextHandler) {
this.nextHandler = nextHandler;
}
@Override
public void handleRequest(SupportRequest request) {
if (request.getRequestType().equalsIgnoreCase("Billing")) {
System.out.println("BillingSupportHandler: Handling billing support request.");
} else if (nextHandler != null) {
nextHandler.handleRequest(request);
}
}
}
public class GeneralSupportHandler implements SupportHandler {
private SupportHandler nextHandler;
@Override
public void setNextHandler(SupportHandler nextHandler) {
this.nextHandler = nextHandler;
}
@Override
public void handleRequest(SupportRequest request) {
System.out.println("GeneralSupportHandler: Handling general support request.");
}
}
Why create specific handlers?
This promotes Single Responsibility by allowing each handler to focus only on its area of request processing, enhancing maintainability and scalability.
Step 3: Setting Up the Chain
Now that we have our handlers, we need to set them up in a chain of responsibility.
public class SupportRequestHandler {
public static void main(String[] args) {
// Creating instances of handlers
SupportHandler techHandler = new TechnicalSupportHandler();
SupportHandler billingHandler = new BillingSupportHandler();
SupportHandler generalHandler = new GeneralSupportHandler();
// Setting up the chain
techHandler.setNextHandler(billingHandler);
billingHandler.setNextHandler(generalHandler);
// Creating requests
SupportRequest technicalRequest = new SupportRequest("Technical");
SupportRequest billingRequest = new SupportRequest("Billing");
SupportRequest generalRequest = new SupportRequest("General");
// Testing the chain
techHandler.handleRequest(technicalRequest);
techHandler.handleRequest(billingRequest);
techHandler.handleRequest(generalRequest);
}
}
Why use a chain of handlers?
Setting up a chain allows requests to flow from one handler to another automatically, promoting a clean and modular code structure.
Overcoming Complexity
While implementing the CoR pattern can be straightforward, there are several strategies to handle its potential complexities:
1. Standardizing Handler Implementation
Define a base handler class with common logic. This reduces code duplication and ensures consistency among handlers.
public abstract class AbstractSupportHandler implements SupportHandler {
protected SupportHandler nextHandler;
@Override
public void setNextHandler(SupportHandler nextHandler) {
this.nextHandler = nextHandler;
}
protected void passToNext(SupportRequest request) {
if (nextHandler != null) {
nextHandler.handleRequest(request);
}
}
}
// Concrete handlers can now extend this class.
2. Limiting Chain Length
Design the system to prevent unintentional lengthy chains that may degrade performance. Consider using a factory pattern to create a handler chain dynamically based on some configuration rather than hardcoding it.
3. Error Handling and Logging
Implement a standard error handling and logging mechanism. This ensures that you can trace the request flow effectively.
public abstract class AbstractSupportHandler implements SupportHandler {
// Other existing methods
protected void log(String message) {
System.out.println(message);
}
}
4. Robust Testing
Be sure to unit test each handler individually and the chain as a whole to ensure it behaves as expected under various conditions.
Bringing It All Together
The Chain of Responsibility pattern can greatly enhance the design of your Java applications by promoting decoupling and single responsibility. However, it is crucial to recognize the complexities that can arise and take proactive measures to manage them.
By structuring your handlers well, standardizing implementations, and employing robust handling and logging strategies, you can make the most out of this pattern while keeping your code maintainable. The CoR pattern not only gives your code structure, but it also makes it more adaptable to future changes.
For more insight into design patterns in Java, refer to "Design Patterns: Elements of Reusable Object-Oriented Software", a foundational book that expands on these concepts.
Feel free to share your thoughts or any questions you have about the Chain of Responsibility pattern in the comments!