Troubleshooting JavaFX App Launch Failures in Browsers
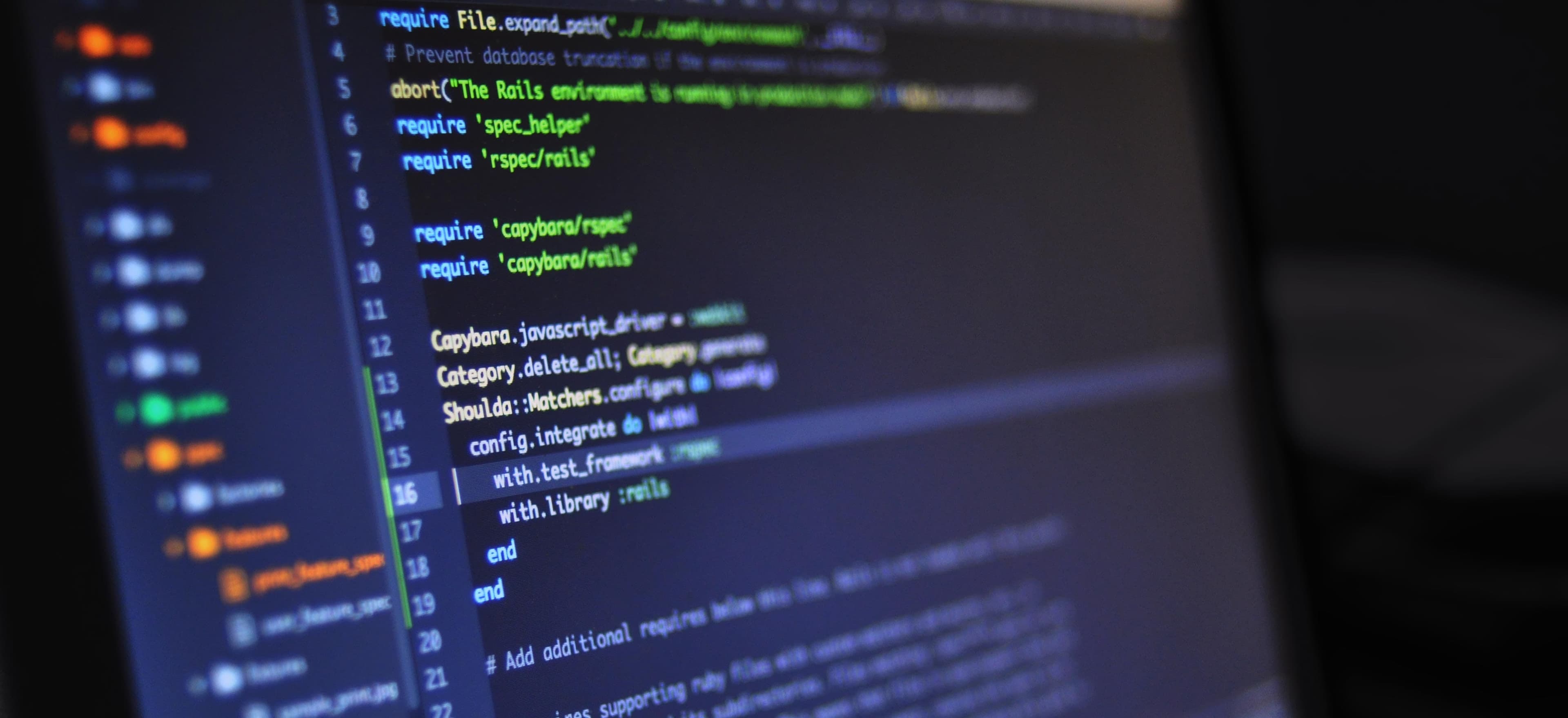
- Published on
Troubleshooting JavaFX App Launch Failures in Browsers
JavaFX provides a rich set of tools for building interactive applications, ensuring that developers can create beautiful user interfaces. However, JavaFX applications can encounter difficulties, especially when deployed in web browsers. This blog post aims to explore common issues when launching JavaFX applications in browsers and provide ways to troubleshoot these errors effectively.
Understanding JavaFX and Web Browser Deployment
JavaFX applications can be deployed as standalone applications or applications that run in a browser. The latter utilizes JavaFX's WebView component, which essentially embeds a web browser within the JavaFX application. However, the support and the environment in which these apps are executed differ significantly from a traditional desktop setting.
Why JavaFX Applications Might Fail to Launch in Browsers
Launch failures can occur due to several factors. Here are some common reasons:
- Browser Compatibility: Not all browsers support JavaFX as they used to.
- Java Version Issues: Using an inappropriate JDK version can cause problems.
- MIME Type Misconfiguration: The server needs the correct configuration for serving JavaFX applications.
Let’s delve deeper into troubleshooting these issues one by one.
Check for Browser Compatibility
First, ensure that your application is being accessed via a compatible browser. As of my last knowledge update in October 2023, most browsers have shifted away from supporting NPAPI and Java applets, which were commonly used in the past for Java applications.
What You Can Do
-
Try Different Browsers: If your application is failing in one browser, try launching it in others, such as Firefox or Chrome. However, be wary that modern versions may also refuse to support Java applet technologies altogether.
-
Consult Browser Documentation: Take time to read the latest documentation for Java or any JavaFX-specific updates pertaining to browser compatibility.
Verify Your Java Version
JavaFX is part of the Java SE distribution in Java 8 and has been decoupled in later versions. If your application was developed in an older version, it might cause issues when run in newer versions or vice versa.
Steps to Take
java -version
Executing this command will show the current Java version. Compare it with your project's required version to see if they match.
-
Upgrade or Downgrade as Necessary: If you're not running the appropriate version, install the correct JDK. Here is the link to the Oracle JDK for further assistance.
-
Update Your Project Settings: Ensure that the Java version in your IDE matches your application's runtime requirement.
Server Configuration for MIME Types
The server should be set up to correctly serve JavaFX applications, particularly in relation to MIME types. If you do not configure these correctly, browsers may not recognize the necessary files needed to run your JavaFX application.
What to Check
- MIME Types: Ensure that the server serving the JavaFX application supports the MIME type for JavaFX files. For example, you might need to add the following in your server configuration:
AddType application/x-java-jnlp-file .jnlp
AddType application/x-java-archive .jar
- Web Server Configuration Examples:
For Apache:
<IfModule mod_mime.c>
AddType application/x-java-jnlp-file .jnlp
AddType application/x-java-archive .jar
</IfModule>
For Nginx:
http {
include mime.types;
types {
application/x-java-jnlp-file jnlp;
application/x-java-archive jar;
}
}
Properly configuring these MIME types ensures that your JavaFX application loads without issues.
Proper JavaFX Package Structure
Sometimes, the issue can stem from how you structured your project. JavaFX requires specific directory layouts to load resources correctly.
Recommended Package Structure
src/
├── main/
│ ├── java/
│ │ └── com/
│ │ └── example/
│ │ └── MyApp.java
│ ├── resources/
│ │ └── fxml/
│ │ └── layout.fxml
Why This Matters
Having the correct package structure allows JavaFX to locate your resources effectively. If this is not followed, your application cannot find the FXML
, CSS
, or images, causing it to fail to launch.
Review Your Code for Exceptions
Sometimes the issue isn’t with the environment, but rather with your code itself. JavaFX applications can throw exceptions that might not surface during development but become an issue in the browser.
Tips to Debug Your Code:
- Check Console Logs: Ensure you are logging errors and can access any logs in the browser console for detailed error output.
try {
// Load your FXML
FXMLLoader loader = new FXMLLoader(getClass().getResource("layout.fxml"));
Parent root = loader.load();
} catch (IOException e) {
e.printStackTrace();
}
Incorporating logging will help you identify problems when the application fails to launch.
- Utilize Try-Catch Blocks: Use structured exception handling to gracefully handle loading failures.
Testing
If all else fails, you may want to consider developing an isolated test case that only contains the necessary components of the application. This approach will help you determine if the issue is with your specific application or with the JavaFX environment/browser configuration.
To Wrap Things Up
While JavaFX provides powerful tools for rich user interactions, deploying applications within a web browser can come with challenges. From ensuring compatibility between Java versions and browsers to server configurations, a multitude of factors can contribute to launch failures.
By methodically verifying compatibility, dependencies, server settings, and proper coding practices, you can effectively troubleshoot these issues and bring your JavaFX applications to life in the browser.
Feel free to conduct further research on JavaFX Application Deployment for more insights. If issues persist, engaging with the community through platforms like Stack Overflow can provide tailored guidance.
Happy coding!
Checkout our other articles