Resolving TinyMCE Plugin Conflicts in Java Applications
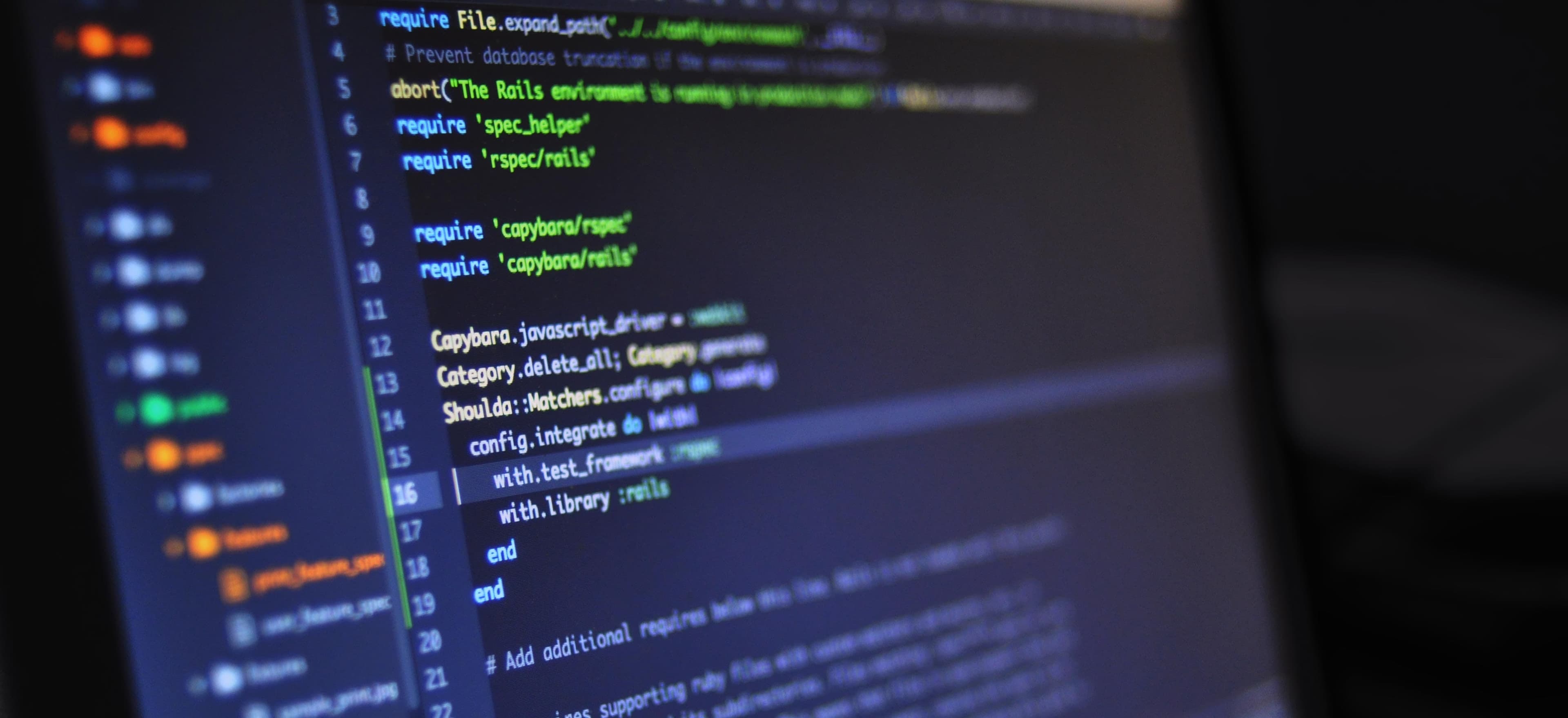
- Published on
Resolving TinyMCE Plugin Conflicts in Java Applications
In the ever-evolving world of web development, integrating rich text editors like TinyMCE can significantly enhance the user experience. However, when working with TinyMCE plugins in Java applications, developers often encounter conflicts that can impede functionality. This article will discuss how to effectively resolve these conflicts, ensuring seamless integration of TinyMCE in your Java-based web applications.
Understanding TinyMCE and Its Plugins
TinyMCE is a popular WYSIWYG editor that allows users to create content directly in their web browsers. Its versatility is largely attributed to its robust plugin system, which extends its capabilities, enabling features like media embedding, table support, and much more.
However, despite its flexibility, plugin conflicts may arise due to various reasons, such as:
- Incompatible versions: Different versions of a plugin may not work well together.
- Overlapping functionalities: Two plugins attempting to perform the same functions can lead to unexpected behavior.
- Implementation errors: Misconfigurations in your Java application may cause issues with TinyMCE.
To ensure your TinyMCE instance operates smoothly, it's crucial to troubleshoot and resolve any plugin conflicts.
Setting Up TinyMCE in a Java Application
Before delving into conflict resolution, let's quickly set up TinyMCE in a Java application.
Step 1: Include TinyMCE in Your Project
Add TinyMCE to your project by including the JS file in your HTML.
<script src="https://cdn.tiny.cloud/1/no-api-key/tinymce/6.4.1/tinymce.min.js" referrerpolicy="origin"></script>
Step 2: Initialize TinyMCE
You can initialize TinyMCE in a script tag:
tinymce.init({
selector: 'textarea#mytextarea', // Replace with your textarea ID
plugins: 'link image code',
toolbar: 'undo redo | link image | code',
});
In this example, we are initializing the editor on a specific textarea (mytextarea
) and enabling essential plugins: link, image, and code.
Step 3: Backend Configuration with Java
In a Spring Boot application, you might handle TinyMCE content submission like so:
@PostMapping("/submit")
public ResponseEntity<String> submitContent(@RequestParam("content") String content) {
// Process the content received from TinyMCE
return ResponseEntity.ok("Content submitted successfully.");
}
The above code snippet provides a simple endpoint to receive content from the TinyMCE editor. Always validate and sanitize the received HTML content before storing it in your database.
Identifying Plugin Conflicts
After setting up, you may encounter issues like non-functional buttons or unexpected behavior. Here are some signs of plugin conflicts:
- Toolbar buttons that do not respond.
- JavaScript errors in the console.
- Unexpected content rendering.
Debugging Tips
To identify the source of the conflict:
- Check the Console: Open the browser's developer tools and look for JavaScript errors.
- Isolate Plugins: Temporarily disable all plugins and re-enable them one by one.
- Consult Documentation: Ensure you are using the correct version of each plugin that is compatible with TinyMCE.
Resolving Conflicts
Here’s how to resolve common conflicts in TinyMCE plugins.
Approach 1: Updating Plugins
Ensure you are using the latest stable versions of TinyMCE and its plugins. Developers regularly release updates that fix bugs and improve compatibility.
<script src="https://cdn.tiny.cloud/1/no-api-key/tinymce/6.5.0/tinymce.min.js" referrerpolicy="origin"></script>
Approach 2: Namespace Plugin Functionality
If two plugins have overlapping functionalities, try to customize their namespace. While this may require diving into the plugin code, it can help avoid conflicts that arise from similar command names.
tinymce.init({
...,
setup: function(editor) {
editor.on('init', function() {
// Custom namespace logic
});
},
});
Approach 3: Custom Event Handling
Sometimes, you might need to handle events manually to avoid default behaviors. For instance, if one plugin interferes with another's shortcuts, custom event handling could help.
tinymce.init({
...,
setup: function(editor) {
editor.on('keydown', function(e) {
if (e.keyCode === 13) { // Enter key
e.preventDefault(); // Prevent default behavior
// Custom behavior
}
});
},
});
Referencing Existing Articles
If you encounter persistent issues, consider consulting articles such as "Troubleshooting Common Issues in TinyMCE 6 Plugins with React" for insights on how to solve common plugin problems and get a better understanding of how TinyMCE interacts with frameworks like React.
Final Considerations
Integrating TinyMCE into Java applications can greatly enhance your web interface, but plugin conflicts may pose challenges. By systematically identifying the source of conflicts, updating, namespacing functionalities, and leveraging custom event handling, you can resolve issues effectively.
For a successful implementation:
- Always keep your dependencies up-to-date.
- Test each plugin carefully.
- Use the console for troubleshooting.
By following these best practices, you can ensure a smooth and enriching user experience using TinyMCE in your Java applications. Happy coding!
Checkout our other articles